Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Net / System / Net / Mail / BufferedReadStream.cs / 1 / BufferedReadStream.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.IO; internal class BufferedReadStream : DelegatedStream { byte[] storedBuffer; int storedLength; int storedOffset; bool readMore; internal BufferedReadStream(Stream stream) : this(stream, false) { } internal BufferedReadStream(Stream stream, bool readMore) : base(stream) { this.readMore = readMore; } public override bool CanWrite { get { return false; } } public override bool CanSeek { get { return false; } } public override IAsyncResult BeginRead(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { ReadAsyncResult result = new ReadAsyncResult(this, callback, state); result.Read(buffer, offset, count); return result; } public override int EndRead(IAsyncResult asyncResult) { int read = ReadAsyncResult.End(asyncResult); return read; } public override int Read(byte[] buffer, int offset, int count) { int read = 0; if (this.storedOffset < this.storedLength) { read = Math.Min(count, this.storedLength - this.storedOffset); Buffer.BlockCopy(this.storedBuffer, this.storedOffset, buffer, offset, read); this.storedOffset += read; if (read == count || !this.readMore) { return read; } offset += read; count -= read; } return read + base.Read(buffer, offset, count); } public override int ReadByte() { if (this.storedOffset < this.storedLength) { return (int)this.storedBuffer[this.storedOffset++]; } else { return base.ReadByte(); } } internal void Push(byte[] buffer, int offset, int count) { if (count == 0) return; if (this.storedOffset == this.storedLength) { if (this.storedBuffer == null || this.storedBuffer.Length < count) { this.storedBuffer = new byte[count]; } this.storedOffset = 0; this.storedLength = count; } else { // if there's room to just insert before existing data if (count <= this.storedOffset) { this.storedOffset -= count; } // if there's room in the buffer but need to shift things over else if (count <= this.storedBuffer.Length - this.storedLength + this.storedOffset) { Buffer.BlockCopy(this.storedBuffer, this.storedOffset, this.storedBuffer, count, this.storedLength - this.storedOffset); this.storedLength += count - this.storedOffset; this.storedOffset = 0; } else { byte[] newBuffer = new byte[count + this.storedLength - this.storedOffset]; Buffer.BlockCopy(this.storedBuffer, this.storedOffset, newBuffer, count, this.storedLength - this.storedOffset); this.storedLength += count - this.storedOffset; this.storedOffset = 0; this.storedBuffer = newBuffer; } } Buffer.BlockCopy(buffer, offset, this.storedBuffer, this.storedOffset, count); } class ReadAsyncResult : LazyAsyncResult { BufferedReadStream parent; int read; static AsyncCallback onRead = new AsyncCallback(OnRead); internal ReadAsyncResult(BufferedReadStream parent, AsyncCallback callback, object state) : base(null,state,callback) { this.parent = parent; } internal void Read(byte[] buffer, int offset, int count){ if (parent.storedOffset < parent.storedLength) { this.read = Math.Min(count, parent.storedLength - parent.storedOffset); Buffer.BlockCopy(parent.storedBuffer, parent.storedOffset, buffer, offset, this.read); parent.storedOffset += this.read; if (this.read == count || !this.parent.readMore) { this.InvokeCallback(); return; } count -= this.read; offset += this.read; } IAsyncResult result = parent.BaseStream.BeginRead(buffer, offset, count, onRead, this); if (result.CompletedSynchronously) { // this.read += parent.BaseStream.EndRead(result); InvokeCallback(); } } internal static int End(IAsyncResult result) { ReadAsyncResult thisPtr = (ReadAsyncResult)result; thisPtr.InternalWaitForCompletion(); return thisPtr.read; } static void OnRead(IAsyncResult result) { if (!result.CompletedSynchronously) { ReadAsyncResult thisPtr = (ReadAsyncResult)result.AsyncState; try { thisPtr.read += thisPtr.parent.BaseStream.EndRead(result); thisPtr.InvokeCallback(); } catch (Exception e) { if (thisPtr.IsCompleted) throw; thisPtr.InvokeCallback(e); } catch { if (thisPtr.IsCompleted) throw; thisPtr.InvokeCallback(new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Net { using System; using System.IO; internal class BufferedReadStream : DelegatedStream { byte[] storedBuffer; int storedLength; int storedOffset; bool readMore; internal BufferedReadStream(Stream stream) : this(stream, false) { } internal BufferedReadStream(Stream stream, bool readMore) : base(stream) { this.readMore = readMore; } public override bool CanWrite { get { return false; } } public override bool CanSeek { get { return false; } } public override IAsyncResult BeginRead(byte[] buffer, int offset, int count, AsyncCallback callback, object state) { ReadAsyncResult result = new ReadAsyncResult(this, callback, state); result.Read(buffer, offset, count); return result; } public override int EndRead(IAsyncResult asyncResult) { int read = ReadAsyncResult.End(asyncResult); return read; } public override int Read(byte[] buffer, int offset, int count) { int read = 0; if (this.storedOffset < this.storedLength) { read = Math.Min(count, this.storedLength - this.storedOffset); Buffer.BlockCopy(this.storedBuffer, this.storedOffset, buffer, offset, read); this.storedOffset += read; if (read == count || !this.readMore) { return read; } offset += read; count -= read; } return read + base.Read(buffer, offset, count); } public override int ReadByte() { if (this.storedOffset < this.storedLength) { return (int)this.storedBuffer[this.storedOffset++]; } else { return base.ReadByte(); } } internal void Push(byte[] buffer, int offset, int count) { if (count == 0) return; if (this.storedOffset == this.storedLength) { if (this.storedBuffer == null || this.storedBuffer.Length < count) { this.storedBuffer = new byte[count]; } this.storedOffset = 0; this.storedLength = count; } else { // if there's room to just insert before existing data if (count <= this.storedOffset) { this.storedOffset -= count; } // if there's room in the buffer but need to shift things over else if (count <= this.storedBuffer.Length - this.storedLength + this.storedOffset) { Buffer.BlockCopy(this.storedBuffer, this.storedOffset, this.storedBuffer, count, this.storedLength - this.storedOffset); this.storedLength += count - this.storedOffset; this.storedOffset = 0; } else { byte[] newBuffer = new byte[count + this.storedLength - this.storedOffset]; Buffer.BlockCopy(this.storedBuffer, this.storedOffset, newBuffer, count, this.storedLength - this.storedOffset); this.storedLength += count - this.storedOffset; this.storedOffset = 0; this.storedBuffer = newBuffer; } } Buffer.BlockCopy(buffer, offset, this.storedBuffer, this.storedOffset, count); } class ReadAsyncResult : LazyAsyncResult { BufferedReadStream parent; int read; static AsyncCallback onRead = new AsyncCallback(OnRead); internal ReadAsyncResult(BufferedReadStream parent, AsyncCallback callback, object state) : base(null,state,callback) { this.parent = parent; } internal void Read(byte[] buffer, int offset, int count){ if (parent.storedOffset < parent.storedLength) { this.read = Math.Min(count, parent.storedLength - parent.storedOffset); Buffer.BlockCopy(parent.storedBuffer, parent.storedOffset, buffer, offset, this.read); parent.storedOffset += this.read; if (this.read == count || !this.parent.readMore) { this.InvokeCallback(); return; } count -= this.read; offset += this.read; } IAsyncResult result = parent.BaseStream.BeginRead(buffer, offset, count, onRead, this); if (result.CompletedSynchronously) { // this.read += parent.BaseStream.EndRead(result); InvokeCallback(); } } internal static int End(IAsyncResult result) { ReadAsyncResult thisPtr = (ReadAsyncResult)result; thisPtr.InternalWaitForCompletion(); return thisPtr.read; } static void OnRead(IAsyncResult result) { if (!result.CompletedSynchronously) { ReadAsyncResult thisPtr = (ReadAsyncResult)result.AsyncState; try { thisPtr.read += thisPtr.parent.BaseStream.EndRead(result); thisPtr.InvokeCallback(); } catch (Exception e) { if (thisPtr.IsCompleted) throw; thisPtr.InvokeCallback(e); } catch { if (thisPtr.IsCompleted) throw; thisPtr.InvokeCallback(new Exception(SR.GetString(SR.net_nonClsCompliantException))); } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
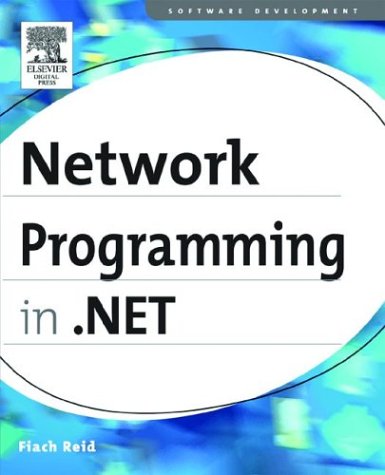
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BaseTemplateCodeDomTreeGenerator.cs
- Input.cs
- EnumerableCollectionView.cs
- WindowsGraphics.cs
- InputLanguageSource.cs
- AttributeEmitter.cs
- DesignerMetadata.cs
- ActivityExecutorDelegateInfo.cs
- TableRowGroupCollection.cs
- WindowsStatic.cs
- TextContainer.cs
- ProcessModuleCollection.cs
- WindowsPen.cs
- Buffer.cs
- NodeLabelEditEvent.cs
- PresentationAppDomainManager.cs
- AssociationSet.cs
- SafeNativeMethods.cs
- _KerberosClient.cs
- RuntimeArgument.cs
- CompilerResults.cs
- Glyph.cs
- NGCPageContentCollectionSerializerAsync.cs
- DataPointer.cs
- TdsParserStaticMethods.cs
- Drawing.cs
- CompressedStack.cs
- StickyNote.cs
- VolatileResourceManager.cs
- HideDisabledControlAdapter.cs
- TrackingMemoryStream.cs
- _LocalDataStoreMgr.cs
- DoWorkEventArgs.cs
- UxThemeWrapper.cs
- DrawingVisualDrawingContext.cs
- QilChoice.cs
- WebScriptEnablingBehavior.cs
- SafeCloseHandleCritical.cs
- ApplicationManager.cs
- GenericUriParser.cs
- SQLDecimalStorage.cs
- XsltException.cs
- KeyMatchBuilder.cs
- Int64AnimationUsingKeyFrames.cs
- FlatButtonAppearance.cs
- TypeSchema.cs
- XmlSerializer.cs
- StateRuntime.cs
- CssStyleCollection.cs
- SslStreamSecurityBindingElement.cs
- TypeValidationEventArgs.cs
- XmlAttributeAttribute.cs
- CultureNotFoundException.cs
- Matrix.cs
- CommandField.cs
- SecurityTokenSerializer.cs
- NavigationWindowAutomationPeer.cs
- MD5CryptoServiceProvider.cs
- OutgoingWebResponseContext.cs
- UnsafeMethods.cs
- LabelLiteral.cs
- ObjectRef.cs
- AssemblyResourceLoader.cs
- KerberosRequestorSecurityToken.cs
- ExpressionBuilder.cs
- XmlHierarchicalEnumerable.cs
- RoutedEvent.cs
- ChangeNode.cs
- SelectionEditingBehavior.cs
- StylusPointPropertyId.cs
- StylusCaptureWithinProperty.cs
- DataControlField.cs
- HostingEnvironmentException.cs
- SamlAssertionKeyIdentifierClause.cs
- OpacityConverter.cs
- CounterSet.cs
- ContentOperations.cs
- BuildManagerHost.cs
- BamlStream.cs
- TextSpanModifier.cs
- SizeConverter.cs
- IdleTimeoutMonitor.cs
- DependencySource.cs
- CodeDomExtensionMethods.cs
- _NegotiateClient.cs
- SecurityHeaderElementInferenceEngine.cs
- AttributeCollection.cs
- TextEndOfParagraph.cs
- TableColumn.cs
- UserControlParser.cs
- XPathNode.cs
- NotFiniteNumberException.cs
- JsonGlobals.cs
- ButtonColumn.cs
- FindCriteria.cs
- DebugController.cs
- RegularExpressionValidator.cs
- SqlFunctions.cs
- XmlAttribute.cs
- DBCommand.cs