Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / HtmlElementCollection.cs / 1 / HtmlElementCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.IO; using System.Drawing; using System.Drawing.Printing; using System.Windows.Forms; using System.Security.Permissions; using System.Security; using System.Runtime.InteropServices; using System.Net; using System.Collections; namespace System.Windows.Forms { ////// /// public sealed class HtmlElementCollection : ICollection { private UnsafeNativeMethods.IHTMLElementCollection htmlElementCollection; private HtmlElement[] elementsArray; private HtmlShimManager shimManager; internal HtmlElementCollection(HtmlShimManager shimManager) { this.htmlElementCollection = null; this.elementsArray = null; this.shimManager = shimManager; } internal HtmlElementCollection(HtmlShimManager shimManager, UnsafeNativeMethods.IHTMLElementCollection elements) { this.htmlElementCollection = elements; this.elementsArray = null; this.shimManager = shimManager; Debug.Assert(this.NativeHtmlElementCollection != null, "The element collection object should implement IHTMLElementCollection"); } internal HtmlElementCollection(HtmlShimManager shimManager, HtmlElement[] array) { this.htmlElementCollection = null; this.elementsArray = array; this.shimManager = shimManager; } private UnsafeNativeMethods.IHTMLElementCollection NativeHtmlElementCollection { get { return this.htmlElementCollection; } } ///[To be supplied.] ////// /// public HtmlElement this[int index] { get { //do some bounds checking here... if (index < 0 || index >= this.Count) { throw new ArgumentOutOfRangeException("index", SR.GetString(SR.InvalidBoundArgument, "index", index, 0, this.Count - 1)); } if (this.NativeHtmlElementCollection != null) { UnsafeNativeMethods.IHTMLElement htmlElement = this.NativeHtmlElementCollection.Item((object)index, (object)0) as UnsafeNativeMethods.IHTMLElement; return (htmlElement != null) ? new HtmlElement(shimManager, htmlElement) : null; } else if (elementsArray != null) { return this.elementsArray[index]; } else { return null; } } } ///[To be supplied.] ////// /// public HtmlElement this[string elementId] { get { if (this.NativeHtmlElementCollection != null) { UnsafeNativeMethods.IHTMLElement htmlElement = this.NativeHtmlElementCollection.Item((object)elementId, (object)0) as UnsafeNativeMethods.IHTMLElement; return (htmlElement != null) ? new HtmlElement(shimManager, htmlElement) : null; } else if (elementsArray != null) { int count = this.elementsArray.Length; for (int i = 0; i < count; i++) { HtmlElement element = this.elementsArray[i]; if (element.Id == elementId) { return element; } } return null; // not found } else { return null; } } } ///[To be supplied.] ////// /// public HtmlElementCollection GetElementsByName(string name) { int count = this.Count; HtmlElement[] temp = new HtmlElement[count]; // count is the maximum # of matches int tempIndex = 0; for (int i = 0; i < count; i++) { HtmlElement element = this[i]; if (element.GetAttribute("name") == name) { temp[tempIndex] = element; tempIndex++; } } if (tempIndex == 0) { return new HtmlElementCollection(shimManager); } else { HtmlElement[] elements = new HtmlElement[tempIndex]; for (int i = 0; i < tempIndex; i++) { elements[i] = temp[i]; } return new HtmlElementCollection(shimManager, elements); } } ///[To be supplied.] ////// /// Returns the total number of elements in the collection. /// public int Count { get { if (this.NativeHtmlElementCollection != null) { return this.NativeHtmlElementCollection.GetLength(); } else if (elementsArray != null) { return this.elementsArray.Length; } else { return 0; } } } ////// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// void ICollection.CopyTo(Array dest, int index) { int count = this.Count; for (int i = 0; i < count; i++) { dest.SetValue(this[i], index++); } } /// /// public IEnumerator GetEnumerator() { HtmlElement[] htmlElements = new HtmlElement[this.Count]; ((ICollection)this).CopyTo(htmlElements, 0); return htmlElements.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.ComponentModel; using System.Diagnostics; using System.IO; using System.Drawing; using System.Drawing.Printing; using System.Windows.Forms; using System.Security.Permissions; using System.Security; using System.Runtime.InteropServices; using System.Net; using System.Collections; namespace System.Windows.Forms { ////// /// public sealed class HtmlElementCollection : ICollection { private UnsafeNativeMethods.IHTMLElementCollection htmlElementCollection; private HtmlElement[] elementsArray; private HtmlShimManager shimManager; internal HtmlElementCollection(HtmlShimManager shimManager) { this.htmlElementCollection = null; this.elementsArray = null; this.shimManager = shimManager; } internal HtmlElementCollection(HtmlShimManager shimManager, UnsafeNativeMethods.IHTMLElementCollection elements) { this.htmlElementCollection = elements; this.elementsArray = null; this.shimManager = shimManager; Debug.Assert(this.NativeHtmlElementCollection != null, "The element collection object should implement IHTMLElementCollection"); } internal HtmlElementCollection(HtmlShimManager shimManager, HtmlElement[] array) { this.htmlElementCollection = null; this.elementsArray = array; this.shimManager = shimManager; } private UnsafeNativeMethods.IHTMLElementCollection NativeHtmlElementCollection { get { return this.htmlElementCollection; } } ///[To be supplied.] ////// /// public HtmlElement this[int index] { get { //do some bounds checking here... if (index < 0 || index >= this.Count) { throw new ArgumentOutOfRangeException("index", SR.GetString(SR.InvalidBoundArgument, "index", index, 0, this.Count - 1)); } if (this.NativeHtmlElementCollection != null) { UnsafeNativeMethods.IHTMLElement htmlElement = this.NativeHtmlElementCollection.Item((object)index, (object)0) as UnsafeNativeMethods.IHTMLElement; return (htmlElement != null) ? new HtmlElement(shimManager, htmlElement) : null; } else if (elementsArray != null) { return this.elementsArray[index]; } else { return null; } } } ///[To be supplied.] ////// /// public HtmlElement this[string elementId] { get { if (this.NativeHtmlElementCollection != null) { UnsafeNativeMethods.IHTMLElement htmlElement = this.NativeHtmlElementCollection.Item((object)elementId, (object)0) as UnsafeNativeMethods.IHTMLElement; return (htmlElement != null) ? new HtmlElement(shimManager, htmlElement) : null; } else if (elementsArray != null) { int count = this.elementsArray.Length; for (int i = 0; i < count; i++) { HtmlElement element = this.elementsArray[i]; if (element.Id == elementId) { return element; } } return null; // not found } else { return null; } } } ///[To be supplied.] ////// /// public HtmlElementCollection GetElementsByName(string name) { int count = this.Count; HtmlElement[] temp = new HtmlElement[count]; // count is the maximum # of matches int tempIndex = 0; for (int i = 0; i < count; i++) { HtmlElement element = this[i]; if (element.GetAttribute("name") == name) { temp[tempIndex] = element; tempIndex++; } } if (tempIndex == 0) { return new HtmlElementCollection(shimManager); } else { HtmlElement[] elements = new HtmlElement[tempIndex]; for (int i = 0; i < tempIndex; i++) { elements[i] = temp[i]; } return new HtmlElementCollection(shimManager, elements); } } ///[To be supplied.] ////// /// Returns the total number of elements in the collection. /// public int Count { get { if (this.NativeHtmlElementCollection != null) { return this.NativeHtmlElementCollection.GetLength(); } else if (elementsArray != null) { return this.elementsArray.Length; } else { return 0; } } } ////// bool ICollection.IsSynchronized { get { return false; } } /// /// object ICollection.SyncRoot { get { return this; } } /// /// void ICollection.CopyTo(Array dest, int index) { int count = this.Count; for (int i = 0; i < count; i++) { dest.SetValue(this[i], index++); } } /// /// public IEnumerator GetEnumerator() { HtmlElement[] htmlElements = new HtmlElement[this.Count]; ((ICollection)this).CopyTo(htmlElements, 0); return htmlElements.GetEnumerator(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
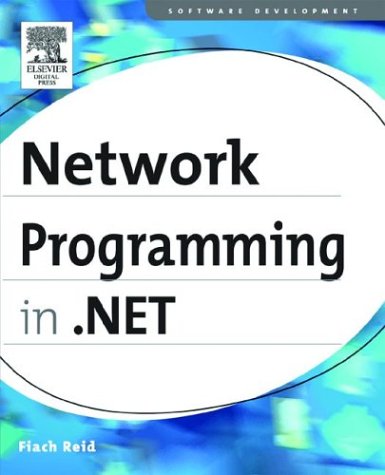
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextEffect.cs
- WaitHandle.cs
- ObjectPersistData.cs
- TypeSystemHelpers.cs
- OdbcConnectionHandle.cs
- RegionIterator.cs
- RowTypePropertyElement.cs
- WebBrowser.cs
- SiteOfOriginPart.cs
- SessionIDManager.cs
- ListManagerBindingsCollection.cs
- NetSectionGroup.cs
- GridEntryCollection.cs
- XmlDesignerDataSourceView.cs
- CommandManager.cs
- ComponentDispatcherThread.cs
- ConfigsHelper.cs
- DesignerRegion.cs
- DocumentEventArgs.cs
- DatatypeImplementation.cs
- HttpResponse.cs
- BooleanStorage.cs
- ManagementPath.cs
- _ReceiveMessageOverlappedAsyncResult.cs
- TreeViewItemAutomationPeer.cs
- DataTableNameHandler.cs
- StrokeFIndices.cs
- ProfileService.cs
- AppendHelper.cs
- XmlMessageFormatter.cs
- SortKey.cs
- HotSpot.cs
- UntrustedRecipientException.cs
- Binding.cs
- AppDomainProtocolHandler.cs
- ImportCatalogPart.cs
- TextElementEnumerator.cs
- NativeMethods.cs
- DesignerActionKeyboardBehavior.cs
- TimerEventSubscription.cs
- FileSystemInfo.cs
- cookiecollection.cs
- DataGridTableCollection.cs
- CustomUserNameSecurityTokenAuthenticator.cs
- References.cs
- _NestedMultipleAsyncResult.cs
- CompilerHelpers.cs
- ProgressBarHighlightConverter.cs
- HttpCapabilitiesSectionHandler.cs
- ImageFormatConverter.cs
- IInstanceTable.cs
- PropertyInfoSet.cs
- DataSourceSerializationException.cs
- FormsAuthenticationConfiguration.cs
- SubstitutionList.cs
- DataTableTypeConverter.cs
- SpeechRecognitionEngine.cs
- State.cs
- IntegrationExceptionEventArgs.cs
- ExpanderAutomationPeer.cs
- PrintDialogDesigner.cs
- CompilerError.cs
- ReadOnlyCollectionBase.cs
- basecomparevalidator.cs
- DataBindingExpressionBuilder.cs
- ParserExtension.cs
- GacUtil.cs
- BamlReader.cs
- Help.cs
- ExecutionEngineException.cs
- TdsParserStaticMethods.cs
- RootProfilePropertySettingsCollection.cs
- PanelStyle.cs
- XmlDictionaryReaderQuotasElement.cs
- FtpCachePolicyElement.cs
- XmlSerializationWriter.cs
- AutomationPropertyInfo.cs
- QueryContinueDragEvent.cs
- ThreadInterruptedException.cs
- Attributes.cs
- ControlTemplate.cs
- ProcessManager.cs
- CellNormalizer.cs
- FunctionImportMapping.cs
- StringComparer.cs
- EntityDataSourceDesignerHelper.cs
- InternalPermissions.cs
- RouteItem.cs
- XmlWhitespace.cs
- StructuredCompositeActivityDesigner.cs
- SqlConnectionFactory.cs
- MarkedHighlightComponent.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- SafeWaitHandle.cs
- Tile.cs
- DataGrid.cs
- SupportingTokenSpecification.cs
- IsolatedStorageFileStream.cs
- XPathChildIterator.cs
- UnaryNode.cs