Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / WinForms / Managed / System / WinForms / PropertyGridInternal / ImmutablePropertyDescriptorGridEntry.cs / 1 / ImmutablePropertyDescriptorGridEntry.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.PropertyGridInternal { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Collections; using System.Reflection; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms; using System.Windows.Forms.Design; using System.Drawing; using System.Drawing.Design; using Microsoft.Win32; // This grid entry is used for immutable objects. An immutable object is identified // through it's TypeConverter, which returns TRUE to ShouldCreateInstance. For this case, // we never go through the property descriptor to change the value, but recreate each // time. // internal class ImmutablePropertyDescriptorGridEntry : PropertyDescriptorGridEntry { internal ImmutablePropertyDescriptorGridEntry(PropertyGrid ownerGrid, GridEntry peParent, PropertyDescriptor propInfo, bool hide) : base(ownerGrid, peParent, propInfo, hide) { } protected override bool IsPropertyReadOnly { get { return ShouldRenderReadOnly; } } public override object PropertyValue { get { return base.PropertyValue; } [SuppressMessage("Microsoft.Security", "CA2102:CatchNonClsCompliantExceptionsInGeneralHandlers")] set { // Create a new instance of the value and set it into the parent grid entry. // object owner = GetValueOwner(); GridEntry parentEntry = InstanceParentGridEntry; TypeConverter parentConverter = parentEntry.TypeConverter; PropertyDescriptorCollection props = parentConverter.GetProperties(parentEntry, owner); IDictionary values = new Hashtable(props.Count); object newObject = null; for (int i = 0; i < props.Count; i++) { if (propertyInfo.Name != null && propertyInfo.Name.Equals(props[i].Name)) { values[props[i].Name] = value; } else { values[props[i].Name] = props[i].GetValue(owner); } } try { newObject = parentConverter.CreateInstance(parentEntry, values); } catch (Exception e) { if (string.IsNullOrEmpty(e.Message)) { throw new TargetInvocationException(SR.GetString(SR.ExceptionCreatingObject, InstanceParentGridEntry.PropertyType.FullName, e.ToString()), e); } else throw; // rethrow the same exception } if (newObject != null) { parentEntry.PropertyValue = newObject; } } } internal override bool NotifyValueGivenParent(object obj, int type) { return ParentGridEntry.NotifyValue(type); } public override bool ShouldRenderReadOnly { get { return InstanceParentGridEntry.ShouldRenderReadOnly; } } private GridEntry InstanceParentGridEntry { get { GridEntry parent = this.ParentGridEntry; if (parent is CategoryGridEntry) { parent = parent.ParentGridEntry; } return parent; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- /* */ namespace System.Windows.Forms.PropertyGridInternal { using System.Runtime.Serialization.Formatters; using System.Diagnostics; using System.Diagnostics.CodeAnalysis; using System; using System.Collections; using System.Reflection; using System.ComponentModel; using System.ComponentModel.Design; using System.Windows.Forms; using System.Windows.Forms.Design; using System.Drawing; using System.Drawing.Design; using Microsoft.Win32; // This grid entry is used for immutable objects. An immutable object is identified // through it's TypeConverter, which returns TRUE to ShouldCreateInstance. For this case, // we never go through the property descriptor to change the value, but recreate each // time. // internal class ImmutablePropertyDescriptorGridEntry : PropertyDescriptorGridEntry { internal ImmutablePropertyDescriptorGridEntry(PropertyGrid ownerGrid, GridEntry peParent, PropertyDescriptor propInfo, bool hide) : base(ownerGrid, peParent, propInfo, hide) { } protected override bool IsPropertyReadOnly { get { return ShouldRenderReadOnly; } } public override object PropertyValue { get { return base.PropertyValue; } [SuppressMessage("Microsoft.Security", "CA2102:CatchNonClsCompliantExceptionsInGeneralHandlers")] set { // Create a new instance of the value and set it into the parent grid entry. // object owner = GetValueOwner(); GridEntry parentEntry = InstanceParentGridEntry; TypeConverter parentConverter = parentEntry.TypeConverter; PropertyDescriptorCollection props = parentConverter.GetProperties(parentEntry, owner); IDictionary values = new Hashtable(props.Count); object newObject = null; for (int i = 0; i < props.Count; i++) { if (propertyInfo.Name != null && propertyInfo.Name.Equals(props[i].Name)) { values[props[i].Name] = value; } else { values[props[i].Name] = props[i].GetValue(owner); } } try { newObject = parentConverter.CreateInstance(parentEntry, values); } catch (Exception e) { if (string.IsNullOrEmpty(e.Message)) { throw new TargetInvocationException(SR.GetString(SR.ExceptionCreatingObject, InstanceParentGridEntry.PropertyType.FullName, e.ToString()), e); } else throw; // rethrow the same exception } if (newObject != null) { parentEntry.PropertyValue = newObject; } } } internal override bool NotifyValueGivenParent(object obj, int type) { return ParentGridEntry.NotifyValue(type); } public override bool ShouldRenderReadOnly { get { return InstanceParentGridEntry.ShouldRenderReadOnly; } } private GridEntry InstanceParentGridEntry { get { GridEntry parent = this.ParentGridEntry; if (parent is CategoryGridEntry) { parent = parent.ParentGridEntry; } return parent; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
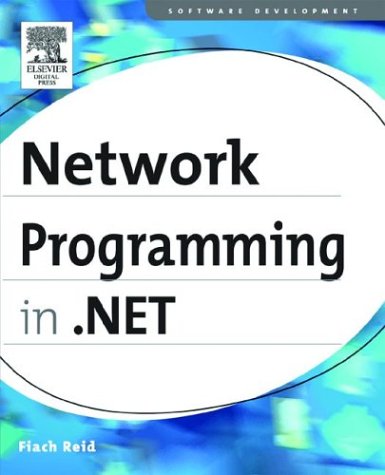
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SelectedPathEditor.cs
- StaticTextPointer.cs
- ViewUtilities.cs
- Stack.cs
- XamlFilter.cs
- WizardStepCollectionEditor.cs
- Helper.cs
- CacheHelper.cs
- DictionaryEntry.cs
- CounterSampleCalculator.cs
- Container.cs
- BridgeDataRecord.cs
- Visual.cs
- PlanCompilerUtil.cs
- Predicate.cs
- StateChangeEvent.cs
- JpegBitmapDecoder.cs
- OutputScopeManager.cs
- DetailsViewUpdateEventArgs.cs
- AliasGenerator.cs
- BaseParaClient.cs
- FontStretchConverter.cs
- HyperLinkColumn.cs
- _FixedSizeReader.cs
- UncommonField.cs
- DateBoldEvent.cs
- EventLogPermissionEntryCollection.cs
- SEHException.cs
- Activity.cs
- DefaultAssemblyResolver.cs
- Process.cs
- KeyPressEvent.cs
- ControlBuilder.cs
- PropertyValue.cs
- WriteTimeStream.cs
- XmlComment.cs
- cookie.cs
- SynchronizedInputHelper.cs
- ShowExpandedMultiValueConverter.cs
- GuidTagList.cs
- Inflater.cs
- FormattedTextSymbols.cs
- DecimalStorage.cs
- BridgeDataRecord.cs
- XmlSerializerFactory.cs
- DependencyObjectProvider.cs
- AnnotationResourceChangedEventArgs.cs
- RefExpr.cs
- ResXBuildProvider.cs
- XpsFilter.cs
- EventProviderTraceListener.cs
- SelectorAutomationPeer.cs
- ValueUnavailableException.cs
- DatagridviewDisplayedBandsData.cs
- PersonalizationState.cs
- PieceNameHelper.cs
- SafeEventHandle.cs
- TextTreeUndoUnit.cs
- CompressedStack.cs
- ConfigPathUtility.cs
- PtsCache.cs
- PopOutPanel.cs
- HorizontalAlignConverter.cs
- BitmapEffectInput.cs
- TreeNode.cs
- Parser.cs
- BaseTemplatedMobileComponentEditor.cs
- MailMessage.cs
- MarshalByValueComponent.cs
- SqlProviderManifest.cs
- Part.cs
- NavigatingCancelEventArgs.cs
- CodeAttributeArgumentCollection.cs
- TimelineClockCollection.cs
- FieldNameLookup.cs
- DbgUtil.cs
- XamlDesignerSerializationManager.cs
- EntityContainerAssociationSetEnd.cs
- XmlSchemaIdentityConstraint.cs
- FileCodeGroup.cs
- DesignerDataStoredProcedure.cs
- mda.cs
- EqualityComparer.cs
- XmlSchemaDatatype.cs
- TextRenderer.cs
- DnsPermission.cs
- PolyBezierSegmentFigureLogic.cs
- ConfigLoader.cs
- WorkflowDesigner.cs
- JsonFormatGeneratorStatics.cs
- MenuAdapter.cs
- Point3DCollectionConverter.cs
- ToolBarTray.cs
- ControlAdapter.cs
- InvalidDataException.cs
- StateMachineWorkflow.cs
- XamlContextStack.cs
- StructuredCompositeActivityDesigner.cs
- AudioLevelUpdatedEventArgs.cs
- WhitespaceSignificantCollectionAttribute.cs