Code:
/ Net / Net / 3.5.50727.3053 / DEVDIV / depot / DevDiv / releases / whidbey / netfxsp / ndp / fx / src / Xml / System / Xml / schema / XmlSchemaInfo.cs / 1 / XmlSchemaInfo.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Xml; using System.Collections; namespace System.Xml.Schema { ///public class XmlSchemaInfo : IXmlSchemaInfo { bool isDefault; bool isNil; XmlSchemaElement schemaElement; XmlSchemaAttribute schemaAttribute; XmlSchemaType schemaType; XmlSchemaSimpleType memberType; XmlSchemaValidity validity; XmlSchemaContentType contentType; public XmlSchemaInfo() { Clear(); } internal XmlSchemaInfo(XmlSchemaValidity validity) : this() { this.validity = validity; } public XmlSchemaValidity Validity { get { return validity; } set { validity = value; } } public bool IsDefault { get { return isDefault; } set { isDefault = value; } } public bool IsNil { get { return isNil; } set { isNil = value; } } public XmlSchemaSimpleType MemberType { get { return memberType; } set { memberType = value; } } public XmlSchemaType SchemaType { get { return schemaType; } set { schemaType = value; if (schemaType != null) { //Member type will not change its content type contentType = schemaType.SchemaContentType; } else { contentType = XmlSchemaContentType.Empty; } } } public XmlSchemaElement SchemaElement { get { return schemaElement; } set { schemaElement = value; if (value != null) { //Setting non-null SchemaElement means SchemaAttribute should be null schemaAttribute = null; } } } public XmlSchemaAttribute SchemaAttribute { get { return schemaAttribute; } set { schemaAttribute = value; if (value != null) { //Setting non-null SchemaAttribute means SchemaElement should be null schemaElement = null; } } } public XmlSchemaContentType ContentType { get { return contentType; } set { contentType = value; } } internal XmlSchemaType XmlType { get { if (memberType != null) { return memberType; } return schemaType; } } internal bool HasDefaultValue { get { return schemaElement != null && schemaElement.ElementDecl.DefaultValueTyped != null; } } internal bool IsUnionType { get { if (schemaType == null || schemaType.Datatype == null) { return false; } return schemaType.Datatype.Variety == XmlSchemaDatatypeVariety.Union; } } internal void Clear() { isNil = false; isDefault = false; schemaType = null; schemaElement = null; schemaAttribute = null; memberType = null; validity = XmlSchemaValidity.NotKnown; contentType = XmlSchemaContentType.Empty; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //[....] //----------------------------------------------------------------------------- using System.Xml; using System.Collections; namespace System.Xml.Schema { ///public class XmlSchemaInfo : IXmlSchemaInfo { bool isDefault; bool isNil; XmlSchemaElement schemaElement; XmlSchemaAttribute schemaAttribute; XmlSchemaType schemaType; XmlSchemaSimpleType memberType; XmlSchemaValidity validity; XmlSchemaContentType contentType; public XmlSchemaInfo() { Clear(); } internal XmlSchemaInfo(XmlSchemaValidity validity) : this() { this.validity = validity; } public XmlSchemaValidity Validity { get { return validity; } set { validity = value; } } public bool IsDefault { get { return isDefault; } set { isDefault = value; } } public bool IsNil { get { return isNil; } set { isNil = value; } } public XmlSchemaSimpleType MemberType { get { return memberType; } set { memberType = value; } } public XmlSchemaType SchemaType { get { return schemaType; } set { schemaType = value; if (schemaType != null) { //Member type will not change its content type contentType = schemaType.SchemaContentType; } else { contentType = XmlSchemaContentType.Empty; } } } public XmlSchemaElement SchemaElement { get { return schemaElement; } set { schemaElement = value; if (value != null) { //Setting non-null SchemaElement means SchemaAttribute should be null schemaAttribute = null; } } } public XmlSchemaAttribute SchemaAttribute { get { return schemaAttribute; } set { schemaAttribute = value; if (value != null) { //Setting non-null SchemaAttribute means SchemaElement should be null schemaElement = null; } } } public XmlSchemaContentType ContentType { get { return contentType; } set { contentType = value; } } internal XmlSchemaType XmlType { get { if (memberType != null) { return memberType; } return schemaType; } } internal bool HasDefaultValue { get { return schemaElement != null && schemaElement.ElementDecl.DefaultValueTyped != null; } } internal bool IsUnionType { get { if (schemaType == null || schemaType.Datatype == null) { return false; } return schemaType.Datatype.Variety == XmlSchemaDatatypeVariety.Union; } } internal void Clear() { isNil = false; isDefault = false; schemaType = null; schemaElement = null; schemaAttribute = null; memberType = null; validity = XmlSchemaValidity.NotKnown; contentType = XmlSchemaContentType.Empty; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
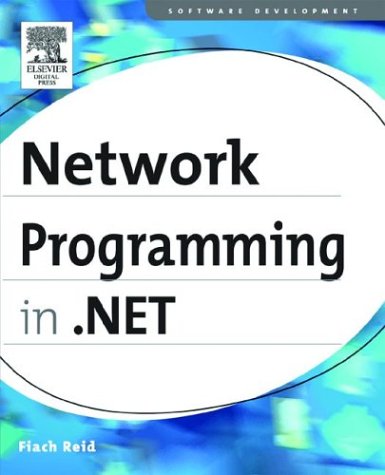
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextSelectionProcessor.cs
- UseAttributeSetsAction.cs
- SingleConverter.cs
- CollectionViewGroupRoot.cs
- SiteMembershipCondition.cs
- StringReader.cs
- NamespaceCollection.cs
- StateManagedCollection.cs
- BindingListCollectionView.cs
- EnumValAlphaComparer.cs
- DataBoundControlDesigner.cs
- AuthenticationService.cs
- NeutralResourcesLanguageAttribute.cs
- SecurityTokenAuthenticator.cs
- ObjectStateManagerMetadata.cs
- TypeForwardedToAttribute.cs
- Metadata.cs
- StateWorkerRequest.cs
- XmlTextAttribute.cs
- HasCopySemanticsAttribute.cs
- ScrollViewerAutomationPeer.cs
- Figure.cs
- Logging.cs
- APCustomTypeDescriptor.cs
- GeneratedContractType.cs
- XsdValidatingReader.cs
- ScriptManagerProxy.cs
- FileLogRecord.cs
- Effect.cs
- Attributes.cs
- FieldDescriptor.cs
- RTLAwareMessageBox.cs
- WindowsMenu.cs
- ThicknessAnimationBase.cs
- CombinedGeometry.cs
- WindowPatternIdentifiers.cs
- JavascriptXmlWriterWrapper.cs
- GregorianCalendar.cs
- EncryptedReference.cs
- SiteMapProvider.cs
- EncoderExceptionFallback.cs
- PanelStyle.cs
- DetailsViewDeleteEventArgs.cs
- ControlDesigner.cs
- DataGridViewUtilities.cs
- RadioButtonList.cs
- RowSpanVector.cs
- ImageMapEventArgs.cs
- Base64Stream.cs
- Int32Collection.cs
- WebConfigurationFileMap.cs
- CharAnimationUsingKeyFrames.cs
- ControlCollection.cs
- DataGridViewRowStateChangedEventArgs.cs
- ScrollItemProviderWrapper.cs
- DataBindingHandlerAttribute.cs
- PageMediaType.cs
- KnownBoxes.cs
- _UncName.cs
- basemetadatamappingvisitor.cs
- XmlDictionaryReaderQuotas.cs
- XsdDuration.cs
- SendParametersContent.cs
- ColumnMapVisitor.cs
- ContentPlaceHolder.cs
- XPathSelectionIterator.cs
- GlobalizationAssembly.cs
- ErrorFormatterPage.cs
- Compiler.cs
- TypeDescriptionProvider.cs
- Stylesheet.cs
- CqlIdentifiers.cs
- EntityObject.cs
- RectangleF.cs
- PlainXmlDeserializer.cs
- DocumentGridContextMenu.cs
- EtwProvider.cs
- Helpers.cs
- XmlAttributeCollection.cs
- PerspectiveCamera.cs
- PageSettings.cs
- MDIWindowDialog.cs
- SevenBitStream.cs
- SpeechEvent.cs
- SByteConverter.cs
- WmlLinkAdapter.cs
- FontFamilyIdentifier.cs
- DynamicMetaObjectBinder.cs
- BinaryUtilClasses.cs
- contentDescriptor.cs
- PropertyValueChangedEvent.cs
- FamilyMapCollection.cs
- DataGridViewTextBoxColumn.cs
- Encoder.cs
- FontConverter.cs
- EntityDataSourceSelectingEventArgs.cs
- SerializationInfo.cs
- CacheMode.cs
- DebugInfoGenerator.cs
- PerfCounters.cs