Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Tokens / WindowsSecurityToken.cs / 1 / WindowsSecurityToken.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Tokens { using System.Collections.Generic; using System.Collections.ObjectModel; using System.Security.Principal; using System.IdentityModel.Claims; using System.IdentityModel.Policy; using System.IdentityModel.Tokens; public class WindowsSecurityToken : SecurityToken, IDisposable { string id; DateTime effectiveTime; DateTime expirationTime; WindowsIdentity windowsIdentity; bool disposed = false; public WindowsSecurityToken(WindowsIdentity windowsIdentity) : this(windowsIdentity, SecurityUniqueId.Create().Value) { } public WindowsSecurityToken(WindowsIdentity windowsIdentity, string id) { DateTime effectiveTime = DateTime.UtcNow; Initialize(id, effectiveTime, DateTime.UtcNow.AddHours(10), windowsIdentity, true); } protected WindowsSecurityToken() { } protected void Initialize(string id, DateTime effectiveTime, DateTime expirationTime, WindowsIdentity windowsIdentity, bool clone) { if (windowsIdentity == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("windowsIdentity"); if (id == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("id"); this.id = id; this.effectiveTime = effectiveTime; this.expirationTime = expirationTime; this.windowsIdentity = clone ? SecurityUtils.CloneWindowsIdentityIfNecessary(windowsIdentity) : windowsIdentity; } public override string Id { get { return this.id; } } public override DateTime ValidFrom { get { return this.effectiveTime; } } public override DateTime ValidTo { get { return this.expirationTime; } } public virtual WindowsIdentity WindowsIdentity { get { ThrowIfDisposed(); return this.windowsIdentity; } } public override ReadOnlyCollectionSecurityKeys { get { return EmptyReadOnlyCollection .Instance; } } public virtual void Dispose() { if (!this.disposed) { this.disposed = true; if (this.windowsIdentity != null) { this.windowsIdentity.Dispose(); this.windowsIdentity = null; } } } protected void ThrowIfDisposed() { if (this.disposed) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(new ObjectDisposedException(this.GetType().FullName)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
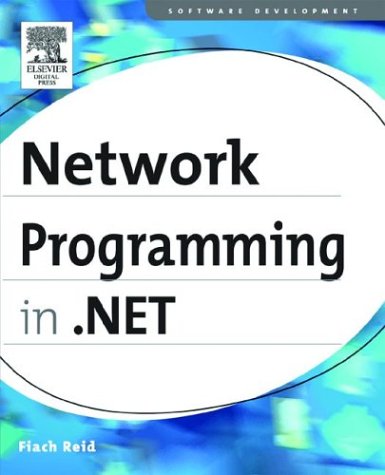
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- COM2ExtendedUITypeEditor.cs
- BooleanToVisibilityConverter.cs
- DataBinding.cs
- MemberMaps.cs
- GacUtil.cs
- MailWebEventProvider.cs
- formatter.cs
- TransactedReceiveScope.cs
- RangeValuePattern.cs
- PointCollectionConverter.cs
- IdentityManager.cs
- VerticalConnector.xaml.cs
- DrawingGroupDrawingContext.cs
- ConfigurationErrorsException.cs
- Variant.cs
- CacheChildrenQuery.cs
- OdbcTransaction.cs
- SoapCodeExporter.cs
- EdmToObjectNamespaceMap.cs
- ResourcePermissionBaseEntry.cs
- BidOverLoads.cs
- NetNamedPipeSecurityMode.cs
- EntityConnectionStringBuilder.cs
- GroupBox.cs
- WeakEventManager.cs
- Transform3D.cs
- TraceRecord.cs
- AppDomainFactory.cs
- entitydatasourceentitysetnameconverter.cs
- EntityDataSourceQueryBuilder.cs
- Point4D.cs
- SiteOfOriginContainer.cs
- EntityTemplateFactory.cs
- ClusterRegistryConfigurationProvider.cs
- DataTableClearEvent.cs
- CommandPlan.cs
- FastEncoderWindow.cs
- WmlCommandAdapter.cs
- BuildResult.cs
- SimpleMailWebEventProvider.cs
- TokenBasedSetEnumerator.cs
- ApplicationBuildProvider.cs
- Point3DCollection.cs
- IgnoreSectionHandler.cs
- AsyncSerializedWorker.cs
- BitmapEffectInput.cs
- Ipv6Element.cs
- ReflectionServiceProvider.cs
- BasicCellRelation.cs
- Operator.cs
- GridViewColumn.cs
- SectionVisual.cs
- SafeReversePInvokeHandle.cs
- Rect.cs
- AutomationProperty.cs
- ISCIIEncoding.cs
- BaseEntityWrapper.cs
- Matrix.cs
- Variable.cs
- SectionXmlInfo.cs
- XmlSchemaObject.cs
- VerificationException.cs
- CacheMemory.cs
- BinHexEncoding.cs
- ParameterCollection.cs
- CssClassPropertyAttribute.cs
- ComponentDispatcher.cs
- AuthorizationRule.cs
- ContainerActivationHelper.cs
- ContextMenuStrip.cs
- ClientScriptManagerWrapper.cs
- RichTextBox.cs
- ConnectionInterfaceCollection.cs
- MetadataResolver.cs
- StandardOleMarshalObject.cs
- Line.cs
- Vector3DCollectionConverter.cs
- TemplateXamlTreeBuilder.cs
- ADConnectionHelper.cs
- MSAAWinEventWrap.cs
- XXXOnTypeBuilderInstantiation.cs
- IndexedGlyphRun.cs
- WorkflowTransactionOptions.cs
- counter.cs
- ByeMessageCD1.cs
- FormView.cs
- Socket.cs
- COM2ExtendedTypeConverter.cs
- DataSetMappper.cs
- SqlClientWrapperSmiStream.cs
- MouseOverProperty.cs
- PlaceHolder.cs
- RenderDataDrawingContext.cs
- BamlLocalizabilityResolver.cs
- AuthenticationException.cs
- CommentEmitter.cs
- ToolboxDataAttribute.cs
- ErrorStyle.cs
- HScrollBar.cs
- SimpleType.cs