Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / Log / System / IO / Log / FileLogRecordEnumerator.cs / 1 / FileLogRecordEnumerator.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IO.Log { using System; using System.Collections; using System.Collections.Generic; internal class FileLogRecordEnumerable : IEnumerable{ LogRecordEnumeratorType logRecordEnum; SimpleFileLog log; SequenceNumber start; bool enumRestartAreas; internal FileLogRecordEnumerable( SimpleFileLog log, SequenceNumber start, LogRecordEnumeratorType logRecordEnum, bool enumRestartAreas) { this.log = log; this.start = start; this.logRecordEnum = logRecordEnum; this.enumRestartAreas = enumRestartAreas; } IEnumerator IEnumerable.GetEnumerator() { return this.GetEnumerator(); } public IEnumerator GetEnumerator() { return new FileLogRecordEnumerator( log, start, logRecordEnum, enumRestartAreas); } } internal class FileLogRecordEnumerator : IEnumerator { FileLogRecordStream stream = null; FileLogRecord record = null; bool enumStarted = false; SequenceNumber start; SequenceNumber current; LogRecordEnumeratorType logRecordEnum; SimpleFileLog log; bool disposed = false; bool enumRestartAreas; internal FileLogRecordEnumerator( SimpleFileLog log, SequenceNumber start, LogRecordEnumeratorType logRecordEnum, bool enumRestartAreas) { this.log = log; this.start = start; this.current = start; this.logRecordEnum = logRecordEnum; this.enumRestartAreas = enumRestartAreas; } object IEnumerator.Current { get { return this.Current; } } public LogRecord Current { get { if (this.disposed) #pragma warning suppress 56503 throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ObjectDisposed()); // IEnumerable interface contract for "current" member can throw InvalidOperationException. Suppressing this warning. if (!this.enumStarted) #pragma warning suppress 56503 throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.EnumNotStarted()); if (this.record == null) #pragma warning suppress 56503 throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.EnumEnded()); return this.record; } } public bool MoveNext() { if (this.disposed) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ObjectDisposed()); if (this.current == SequenceNumber.Invalid) return false; if (!this.enumStarted) { this.enumStarted = true; } else { switch (this.logRecordEnum) { case LogRecordEnumeratorType.Next: this.current = this.stream.NextLsn; break; case LogRecordEnumeratorType.Previous: this.current = this.stream.Header.PreviousLsn; break; case LogRecordEnumeratorType.User: this.current = this.stream.Header.NextUndoLsn; break; } } SequenceNumber first; SequenceNumber last; log.GetLogLimits(out first, out last); if (this.current < first || last < this.current || this.current == SequenceNumber.Invalid) { this.record = null; return false; } this.stream = new FileLogRecordStream(this.log, this.current); if (!this.enumRestartAreas && this.stream.Header.IsRestartArea) { if (this.logRecordEnum == LogRecordEnumeratorType.Next) { // Move to the next record after restart area. return MoveNext(); } else { // We have hit a restart area. // Restart areas have special values for prev and next undo in the header. // Cannot enumerate further. this.record = null; return false; } } this.record = new FileLogRecord(this.stream); return true; } public void Reset() { if (this.disposed) throw DiagnosticUtility.ExceptionUtility.ThrowHelperError(Error.ObjectDisposed()); this.enumStarted = false; this.current = this.start; this.record = null; } public void Dispose() { this.disposed = true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
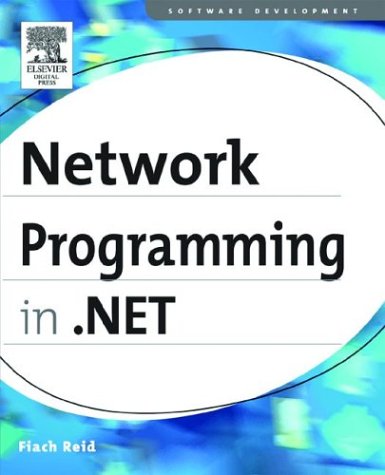
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AnimationStorage.cs
- EnumValAlphaComparer.cs
- CriticalHandle.cs
- XsdValidatingReader.cs
- DbQueryCommandTree.cs
- WrappingXamlSchemaContext.cs
- UrlPropertyAttribute.cs
- DockingAttribute.cs
- OLEDB_Util.cs
- ElapsedEventArgs.cs
- XmlSchemaAnnotation.cs
- SecurityToken.cs
- ServiceMemoryGates.cs
- ResourceReader.cs
- GridViewSelectEventArgs.cs
- ErrorView.xaml.cs
- MetadataCacheItem.cs
- SQLDouble.cs
- OdbcCommandBuilder.cs
- EntityParameter.cs
- ConfigUtil.cs
- DynamicQueryStringParameter.cs
- FontFamily.cs
- QilStrConcatenator.cs
- TdsParserSessionPool.cs
- IsolationInterop.cs
- SerializationException.cs
- StrongNameUtility.cs
- Instrumentation.cs
- BufferedWebEventProvider.cs
- ChannelSinkStacks.cs
- MessageSecurityOverMsmqElement.cs
- cookieexception.cs
- EntityStoreSchemaFilterEntry.cs
- FileNameEditor.cs
- __Filters.cs
- AudioFileOut.cs
- SHA256Managed.cs
- DataViewListener.cs
- RSAPKCS1SignatureFormatter.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- SimpleExpression.cs
- CompiledXpathExpr.cs
- ControlAdapter.cs
- SQLBinary.cs
- SqlDelegatedTransaction.cs
- UnsafeNativeMethodsPenimc.cs
- SlipBehavior.cs
- UnsafeNativeMethods.cs
- HelpInfo.cs
- AuthorizationRule.cs
- SettingsPropertyIsReadOnlyException.cs
- SessionStateItemCollection.cs
- GeometryGroup.cs
- ScrollEvent.cs
- StateWorkerRequest.cs
- ConfigXmlCDataSection.cs
- GreenMethods.cs
- WebServiceMethodData.cs
- IgnoreFlushAndCloseStream.cs
- hresults.cs
- PrePostDescendentsWalker.cs
- HandlerBase.cs
- XmlBinaryReader.cs
- ViewBase.cs
- SqlParameterCollection.cs
- LogManagementAsyncResult.cs
- OleDbConnectionInternal.cs
- MasterPageBuildProvider.cs
- WindowsStreamSecurityBindingElement.cs
- _AutoWebProxyScriptEngine.cs
- DependencyPropertyKind.cs
- QuaternionValueSerializer.cs
- SelectQueryOperator.cs
- DataSet.cs
- CodeTypeDelegate.cs
- BypassElement.cs
- InheritanceContextChangedEventManager.cs
- Interlocked.cs
- HttpStreamMessage.cs
- AssemblyNameProxy.cs
- TextSearch.cs
- TypeDescriptionProviderAttribute.cs
- AppDomainFactory.cs
- ModifiableIteratorCollection.cs
- Evaluator.cs
- BulletedListEventArgs.cs
- LabelAutomationPeer.cs
- CompiledELinqQueryState.cs
- SqlErrorCollection.cs
- DataGridViewColumnHeaderCell.cs
- GroupBox.cs
- MessagingDescriptionAttribute.cs
- Normalization.cs
- CompareValidator.cs
- BoolExpr.cs
- SerializationFieldInfo.cs
- MouseButtonEventArgs.cs
- TraceLevelStore.cs
- JsonStringDataContract.cs