Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / ServiceModel / System / ServiceModel / Security / InfocardInteractiveChannelInitializer.cs / 1 / InfocardInteractiveChannelInitializer.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Security { using System.Collections.Generic; using System.ServiceModel.Channels; using System.ServiceModel.Dispatcher; using System.ServiceModel.Description; using System.IdentityModel.Selectors; using System.IdentityModel.Tokens; using System.Runtime.Serialization; using System.ServiceModel.Security; public class InfocardInteractiveChannelInitializer : IInteractiveChannelInitializer { ClientCredentials credentials; Binding binding; public InfocardInteractiveChannelInitializer( ClientCredentials credentials, Binding binding ) { this.credentials = credentials; this.binding = binding; } public Binding Binding { get { return binding; } } public virtual IAsyncResult BeginDisplayInitializationUI(IClientChannel channel, AsyncCallback callback, object state) { return new GetTokenUIAsyncResult(binding, channel, this.credentials, callback, state); } public virtual void EndDisplayInitializationUI(IAsyncResult result) { GetTokenUIAsyncResult.End(result); } } internal class GetTokenUIAsyncResult :AsyncResult { IClientChannel proxy; ClientCredentials credentials; Uri relyingPartyIssuer; bool requiresInfoCard; Binding binding; static AsyncCallback callback = DiagnosticUtility.ThunkAsyncCallback(new AsyncCallback(GetTokenUIAsyncResult.Callback)); internal GetTokenUIAsyncResult(Binding binding, IClientChannel channel, ClientCredentials credentials, AsyncCallback callback, object state) : base(callback, state) { this.credentials = credentials; this.proxy = channel; this.binding = binding; this.CallBegin(true); } void CallBegin(bool completedSynchronously) { IAsyncResult result = null; Exception exception = null; try { CardSpacePolicyElement[ ] chain; SecurityTokenManager tokenManager = credentials.CreateSecurityTokenManager(); requiresInfoCard = InfoCardHelper.IsInfocardRequired(binding, credentials, tokenManager, proxy.RemoteAddress, out chain, out relyingPartyIssuer); MessageSecurityVersion bindingSecurityVersion = InfoCardHelper.GetBindingSecurityVersionOrDefault(binding); WSSecurityTokenSerializer tokenSerializer = WSSecurityTokenSerializer.DefaultInstance; result = credentials.GetInfoCardTokenCallback.BeginInvoke(requiresInfoCard, chain, tokenManager.CreateSecurityTokenSerializer(bindingSecurityVersion.SecurityTokenVersion), callback, this); } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } exception = e; } if (exception == null) { if (!result.CompletedSynchronously) { return; } this.CallEnd(result, out exception); } if (exception != null) { return; } this.CallComplete(completedSynchronously, null); } static void Callback(IAsyncResult result) { if (result.CompletedSynchronously) { return; } GetTokenUIAsyncResult outer = (GetTokenUIAsyncResult)result.AsyncState; Exception exception = null; outer.CallEnd(result, out exception); outer.CallComplete(false, exception); } void CallEnd(IAsyncResult result, out Exception exception) { try { SecurityToken token = credentials.GetInfoCardTokenCallback.EndInvoke(result); ChannelParameterCollection channelParameters = proxy.GetProperty(); if( null != channelParameters ) { channelParameters.Add( new InfoCardChannelParameter( token, relyingPartyIssuer, requiresInfoCard ) ); } exception = null; } catch (Exception e) { if (DiagnosticUtility.IsFatal(e)) { throw; } exception = e; } } void CallComplete(bool completedSynchronously, Exception exception) { this.Complete(completedSynchronously, exception); } internal static void End(IAsyncResult result) { AsyncResult.End (result); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
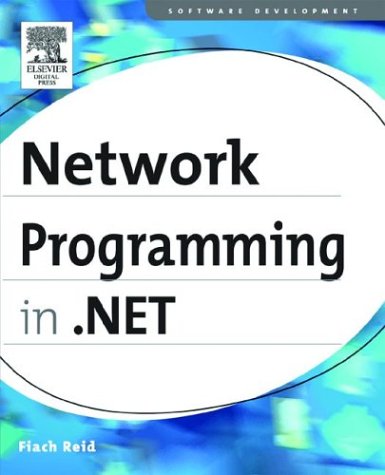
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MouseGesture.cs
- StructuredTypeEmitter.cs
- DataBindingList.cs
- UniformGrid.cs
- AddInDeploymentState.cs
- XmlDataProvider.cs
- IISUnsafeMethods.cs
- ComponentChangedEvent.cs
- QilDataSource.cs
- WinCategoryAttribute.cs
- DataMisalignedException.cs
- ChineseLunisolarCalendar.cs
- FormatSelectingMessageInspector.cs
- mediaeventshelper.cs
- MSG.cs
- QueryReaderSettings.cs
- ListViewGroup.cs
- RelationHandler.cs
- OverrideMode.cs
- FilterQueryOptionExpression.cs
- OleAutBinder.cs
- RegisteredArrayDeclaration.cs
- XmlSerializerNamespaces.cs
- EdmFunctionAttribute.cs
- OdbcCommandBuilder.cs
- RedirectionProxy.cs
- DesignBindingPicker.cs
- DataColumnPropertyDescriptor.cs
- PkcsMisc.cs
- ScriptDescriptor.cs
- HMACRIPEMD160.cs
- DecimalKeyFrameCollection.cs
- TimeoutValidationAttribute.cs
- SymbolDocumentGenerator.cs
- RestClientProxyHandler.cs
- PrincipalPermission.cs
- StatusBar.cs
- WinFormsUtils.cs
- CallbackValidatorAttribute.cs
- CannotUnloadAppDomainException.cs
- InvariantComparer.cs
- ActiveXSite.cs
- _CacheStreams.cs
- OrderedDictionaryStateHelper.cs
- OperationCanceledException.cs
- SqlParameterizer.cs
- CompilerErrorCollection.cs
- FormatterServices.cs
- AppDomainAttributes.cs
- RecordConverter.cs
- XMLUtil.cs
- ServiceMoniker.cs
- QueryRewriter.cs
- X509ChainPolicy.cs
- IApplicationTrustManager.cs
- ValueProviderWrapper.cs
- RtfControlWordInfo.cs
- ControlBindingsConverter.cs
- WebControlAdapter.cs
- ToolStripItemClickedEventArgs.cs
- DataObjectMethodAttribute.cs
- DbTransaction.cs
- CodePropertyReferenceExpression.cs
- DataGridViewCellStateChangedEventArgs.cs
- HtmlEmptyTagControlBuilder.cs
- XmlWellformedWriterHelpers.cs
- PasswordPropertyTextAttribute.cs
- DecoderBestFitFallback.cs
- MexBindingBindingCollectionElement.cs
- ControlBuilder.cs
- ObjectManager.cs
- DoubleLinkListEnumerator.cs
- SectionXmlInfo.cs
- WorkflowQueue.cs
- brushes.cs
- CompilationUnit.cs
- TreeViewImageIndexConverter.cs
- ClientApiGenerator.cs
- ThreadNeutralSemaphore.cs
- SystemException.cs
- TextSimpleMarkerProperties.cs
- BinaryKeyIdentifierClause.cs
- BrowserTree.cs
- CompositeCollection.cs
- DesigntimeLicenseContextSerializer.cs
- RtfFormatStack.cs
- Bidi.cs
- _NestedSingleAsyncResult.cs
- SqlBulkCopy.cs
- BaseValidator.cs
- TypeSystem.cs
- ToolStripDropDownClosedEventArgs.cs
- JavaScriptString.cs
- LockCookie.cs
- VSWCFServiceContractGenerator.cs
- CodeArgumentReferenceExpression.cs
- HierarchicalDataSourceConverter.cs
- InkCanvasSelection.cs
- SectionInput.cs
- PropertyMapper.cs