Code:
/ WCF / WCF / 3.5.30729.1 / untmp / Orcas / SP / ndp / cdf / src / WCF / infocard / Service / managed / Microsoft / InfoCards / ProcessManager.cs / 1 / ProcessManager.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace Microsoft.InfoCards { using System; using System.IO; using System.Text; using Microsoft.Win32; using System.Diagnostics; // Process using System.Collections.Generic; using System.Security.Principal; //WindowsIdentity using System.Runtime.CompilerServices; using System.Runtime.InteropServices; using System.ComponentModel; using Microsoft.InfoCards; using Microsoft.InfoCards.Diagnostics; using IDT = Microsoft.InfoCards.Diagnostics.InfoCardTrace; // // Summary: // Manages a collection of processes. Uses a job object where possible, and otherwise manages // the collection of processes itself. // class ProcessManager : IDisposable { const int WindowsVistaMajorVersion = 6; const string s_OnScreenKeyboardExeName = "osk"; const string s_SwitcherExeName = "msswchx"; const string s_TcserverExeName = "tcserver"; // // Job handle to use if possible. Triggered off the user being on the console session // SafeJobHandle m_hJob; // // List of processes to manage. // Listm_processList; // // The users ts session id. // uint m_userSessionId; // // The name of the trusted User Sid // private string m_trustedUserSid; // // Summary: // Construct a process manager for the given session. // public ProcessManager( int userSessionId, string trustedUserSid ) { m_hJob = null; m_userSessionId = (uint) userSessionId; m_processList = new List (); m_trustedUserSid = trustedUserSid; } internal bool IsConsoleOrVista { get { return ( m_userSessionId == NativeMethods.WTSGetActiveConsoleSessionId() || Environment.OSVersion.Version.Major >= WindowsVistaMajorVersion ) ; } } internal bool IsXPTablet { get { return ((Environment.OSVersion.Version.Major < WindowsVistaMajorVersion) && (0 != NativeMethods.GetSystemMetrics( NativeMethods.SM_TABLETPC ))); } } // Summary: // Spawns/creates the indicated process and then adds it to a job. // The job is created if needed. We add all the processes launched on // the secure desktop to a job as this allows us to easily terminate // the job when we need to switch back to the user desktop and stop/kill // all the processes we launched on the user desktop easily. // // Parameters: // hTrustedUserToken - Token with the trusted user SID allowed to be owner. // trustedUserSid - Stringized SID, used in the ACL for the process. // infocardDesktop - Desktop to launch the apps on. // userIdentity - Identity of caller. // fullpath - fullpath to the application being launched. // public void AddProcess( SafeNativeHandle hTrustedUserToken, ref string trustedUserSid, string infocardDesktop, uint userProcessId, WindowsIdentity userIdentity, string fullPath, string commandLine, bool fUseElevatedToken ) { IntPtr processHandle = IntPtr.Zero; int processId = 0; bool mustRelease = false; bool releaseJob = false; RuntimeHelpers.PrepareConstrainedRegions(); try { if( IsConsoleOrVista && null == m_hJob ) { m_hJob = Utility.CreateJobObjectWithSdHelper( trustedUserSid ); if( null == m_hJob ) { throw IDT.ThrowHelperError( new Win32Exception( Marshal.GetLastWin32Error() )); } } // // CreateJobObject will return NULL in case of failure // hTrustedUserToken.DangerousAddRef( ref mustRelease ); IntPtr trustedUserToken = hTrustedUserToken.DangerousGetHandle(); IntPtr handleJob = IntPtr.Zero; if( IsConsoleOrVista ) { m_hJob.DangerousAddRef( ref releaseJob ); handleJob = m_hJob.DangerousGetHandle(); } // // The process is assigned to the job if a job is passed in. // int error = (int) NativeMcppMethods.CreateProcessAsTrustedUserWrapper( fullPath, (null == commandLine) ? "" : commandLine, userProcessId, infocardDesktop, userIdentity.Name, m_userSessionId, ref trustedUserToken, ref processHandle, ref processId, handleJob, ref trustedUserSid, fUseElevatedToken ); if( 0 == error ) { using( SafeNativeHandle hProcess = new SafeNativeHandle( processHandle, true ) ) { if( !IsConsoleOrVista ) { m_processList.Add( Process.GetProcessById( processId ) ); } hProcess.Dispose(); IDT.TraceDebug( "ICARDACCESS:{0} launched for user {1}", fullPath, userIdentity.User.Value ); } } else { IDT.TraceDebug( "ICARDACCESS:Failed to create process with error {0}", error ); } } finally { if ( mustRelease ) { hTrustedUserToken.DangerousRelease(); } if( releaseJob ) { m_hJob.DangerousRelease(); } } } public void Dispose() { using( SystemIdentity lsa = new SystemIdentity( false ) ) { if( IsConsoleOrVista ) { if( null != m_hJob ) { m_hJob.Dispose(); m_hJob = null; } } else { // // Used to indicate that the on screen keyboard switcher // watching exe needs to be killed. // bool fKillSwitcher = false; for( int i = 0; i < m_processList.Count; i++ ) { Process p = m_processList[ i ]; if( !p.HasExited ) { try { if( m_userSessionId == p.SessionId ) { if( 0 == String.Compare( p.ProcessName, s_OnScreenKeyboardExeName, StringComparison.OrdinalIgnoreCase ) ) { // // We need to kill the msswchx.exe. // fKillSwitcher = true; } // // Utility will handle exceptions correctly. // Utility.KillHelper( p ); } } catch( InvalidOperationException ) { // // If the process has already terminated then, we can get // this exception. // ; } } } // // Kill switcher if needed, if we are not in a job we need // to handle this as a special case. // if( fKillSwitcher ) { foreach( Process p in Process.GetProcessesByName( s_SwitcherExeName ) ) { if( m_userSessionId == p.SessionId ) { if( !p.HasExited ) { // // Utility will handle exceptions correctly. // Utility.KillHelper( p ); } IDT.TraceDebug( "ICARDACCESS:Killed msswchx.exe on IC desktop.}" ); break; } ((IDisposable)p).Dispose(); } } m_processList.Clear(); } } // // tcserver.exe has a handle to our desktop, and will trap the user on the infocard desktop unless we explicitly kill it. // if ( IsXPTablet ) { KillTcserverInstancesForInfoCardDesktop(); } } // // Retrieves all instances of tcserver.exe that are associated with the infocard desktop and terminates them. // private void KillTcserverInstancesForInfoCardDesktop() { // // Get all instances of tcserver and check to see if the sid matches. // foreach ( Process p in Process.GetProcessesByName( s_TcserverExeName ) ) { if ( NativeMcppMethods.IsCardSpaceTcserverInstance( p.Id, m_trustedUserSid ) ) { Utility.KillHelper( p ); } ((IDisposable)p).Dispose(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
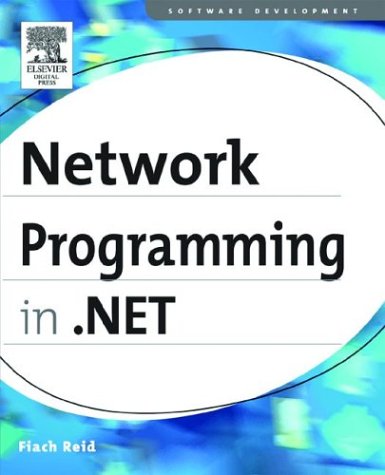
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EntityViewGenerationConstants.cs
- FixedTextView.cs
- ValidationEventArgs.cs
- ServiceAuthorizationManager.cs
- TogglePatternIdentifiers.cs
- WebPartConnectionsConnectVerb.cs
- TextTreeText.cs
- CompositeScriptReferenceEventArgs.cs
- SafeMILHandleMemoryPressure.cs
- Light.cs
- DesignConnectionCollection.cs
- AssemblyName.cs
- WebCategoryAttribute.cs
- bindurihelper.cs
- LinkUtilities.cs
- TextTrailingWordEllipsis.cs
- ColorMatrix.cs
- ResourceDisplayNameAttribute.cs
- DetailsViewRow.cs
- StatusInfoItem.cs
- EncodingNLS.cs
- _BaseOverlappedAsyncResult.cs
- ApplicationId.cs
- UnionCqlBlock.cs
- TCEAdapterGenerator.cs
- XmlDictionary.cs
- ImageConverter.cs
- UInt64Converter.cs
- EmbeddedMailObject.cs
- XmlUtil.cs
- Verify.cs
- AddingNewEventArgs.cs
- SelectorItemAutomationPeer.cs
- MetadataPropertyvalue.cs
- CompatibleComparer.cs
- ChannelServices.cs
- UnsafeNativeMethods.cs
- ITreeGenerator.cs
- PieceNameHelper.cs
- TabControlEvent.cs
- NumericUpDown.cs
- ExtensionFile.cs
- SqlCacheDependency.cs
- XmlSchemaSimpleType.cs
- ListSourceHelper.cs
- _NetRes.cs
- XPathBuilder.cs
- ColumnTypeConverter.cs
- SamlAttribute.cs
- HttpProxyTransportBindingElement.cs
- ChangesetResponse.cs
- CoTaskMemHandle.cs
- ReadOnlyPropertyMetadata.cs
- SqlRecordBuffer.cs
- sortedlist.cs
- Filter.cs
- MemberJoinTreeNode.cs
- DataRecordInternal.cs
- DispatcherHookEventArgs.cs
- validation.cs
- XmlSchemas.cs
- X509CertificateClaimSet.cs
- SafeEventLogWriteHandle.cs
- DecimalAnimationUsingKeyFrames.cs
- FontSizeConverter.cs
- UrlMappingsModule.cs
- ProfileParameter.cs
- SuppressMergeCheckAttribute.cs
- DoubleAnimationBase.cs
- _DisconnectOverlappedAsyncResult.cs
- CharacterHit.cs
- ValueCollectionParameterReader.cs
- HiddenField.cs
- EDesignUtil.cs
- Int32KeyFrameCollection.cs
- DeviceContext2.cs
- AccessDataSource.cs
- WebControlsSection.cs
- OdbcInfoMessageEvent.cs
- ExtenderProvidedPropertyAttribute.cs
- CodeValidator.cs
- DataGridRowHeaderAutomationPeer.cs
- VisualTreeHelper.cs
- HttpCachePolicy.cs
- ReadOnlyDataSourceView.cs
- DataGridPageChangedEventArgs.cs
- DateTimeUtil.cs
- DesignerHierarchicalDataSourceView.cs
- ArgumentOutOfRangeException.cs
- DoubleStorage.cs
- DefaultEventAttribute.cs
- FileVersionInfo.cs
- HttpCacheParams.cs
- PartialClassGenerationTaskInternal.cs
- DetailsViewInsertedEventArgs.cs
- RequestNavigateEventArgs.cs
- PaperSize.cs
- EditorZone.cs
- BitmapPalette.cs
- SmiMetaDataProperty.cs