Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / infocard / Client / System / IdentityModel / Selectors / SafeLibraryHandle.cs / 1305376 / SafeLibraryHandle.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Selectors { using System; using System.IO; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using System.Runtime.CompilerServices; using Microsoft.InfoCards.Diagnostics; using Microsoft.Win32; using System.Security.Permissions; using Microsoft.Win32.SafeHandles; // // Summary: // A class to wrap a library handle for reliability. // When LoadLibrary returns, the runtime stores the resulting IntPtr // into the already created SafeLibraryHandle. The runtime guarantees that // this operation is atomic, meaning that if the P/Invoke method successfully returns, // the IntPtr will be stored safely inside the SafeHandle. Once inside the SafeHandle, // even if an asynchronous exception occurs and prevents LoadLibrary's SafeLibraryHandle return // value from being stored, the relevant IntPtr is already stored within a managed object // whose critical finalizer will ensure its proper release. // [ SecurityPermission( SecurityAction.LinkDemand, UnmanagedCode=true ) ] class SafeLibraryHandle : SafeHandleZeroOrMinusOneIsInvalid { private SafeLibraryHandle() : base( true ) { } protected override bool ReleaseHandle() { #pragma warning suppress 56523 return FreeLibrary( handle ); } [DllImport( "kernel32.dll", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true, CallingConvention = CallingConvention.StdCall )] internal static extern SafeLibraryHandle LoadLibraryW( [MarshalAs( UnmanagedType.LPWStr )] string dllname ); [DllImport( "kernel32.dll", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true, CallingConvention = CallingConvention.StdCall )] internal static extern bool FreeLibrary( IntPtr hModule ); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.IdentityModel.Selectors { using System; using System.IO; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.Runtime.InteropServices; using System.Runtime.ConstrainedExecution; using System.Runtime.CompilerServices; using Microsoft.InfoCards.Diagnostics; using Microsoft.Win32; using System.Security.Permissions; using Microsoft.Win32.SafeHandles; // // Summary: // A class to wrap a library handle for reliability. // When LoadLibrary returns, the runtime stores the resulting IntPtr // into the already created SafeLibraryHandle. The runtime guarantees that // this operation is atomic, meaning that if the P/Invoke method successfully returns, // the IntPtr will be stored safely inside the SafeHandle. Once inside the SafeHandle, // even if an asynchronous exception occurs and prevents LoadLibrary's SafeLibraryHandle return // value from being stored, the relevant IntPtr is already stored within a managed object // whose critical finalizer will ensure its proper release. // [ SecurityPermission( SecurityAction.LinkDemand, UnmanagedCode=true ) ] class SafeLibraryHandle : SafeHandleZeroOrMinusOneIsInvalid { private SafeLibraryHandle() : base( true ) { } protected override bool ReleaseHandle() { #pragma warning suppress 56523 return FreeLibrary( handle ); } [DllImport( "kernel32.dll", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true, CallingConvention = CallingConvention.StdCall )] internal static extern SafeLibraryHandle LoadLibraryW( [MarshalAs( UnmanagedType.LPWStr )] string dllname ); [DllImport( "kernel32.dll", CharSet = CharSet.Unicode, ExactSpelling = true, SetLastError = true, CallingConvention = CallingConvention.StdCall )] internal static extern bool FreeLibrary( IntPtr hModule ); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
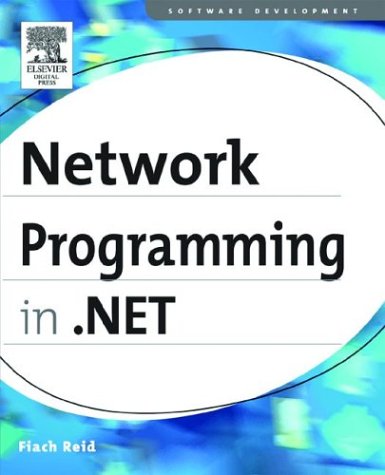
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ProtocolsConfigurationHandler.cs
- CookieHandler.cs
- TypeConverterHelper.cs
- ServiceOperationParameter.cs
- _NegoState.cs
- WindowsRichEditRange.cs
- DiscoveryClientProtocol.cs
- DataGridViewCellCancelEventArgs.cs
- ExpressionBuilder.cs
- EndpointNotFoundException.cs
- MissingMethodException.cs
- Rotation3DKeyFrameCollection.cs
- Automation.cs
- DefaultAsyncDataDispatcher.cs
- DeviceSpecificDialogCachedState.cs
- Vector.cs
- EmptyEnumerator.cs
- ContractMapping.cs
- TextParaLineResult.cs
- TextBox.cs
- SoapAttributeOverrides.cs
- AmbiguousMatchException.cs
- JsonFormatWriterGenerator.cs
- CryptoKeySecurity.cs
- SettingsProperty.cs
- UnionCodeGroup.cs
- _CookieModule.cs
- EventProviderWriter.cs
- ClientOptions.cs
- BindingMAnagerBase.cs
- FacetChecker.cs
- TrackingServices.cs
- ExpressionTable.cs
- QilFactory.cs
- ObjectRef.cs
- InputProcessorProfiles.cs
- RowBinding.cs
- XmlName.cs
- SpotLight.cs
- StylusShape.cs
- EditorPartChrome.cs
- MetadataCache.cs
- SymbolType.cs
- EntityDataSourceWrapperCollection.cs
- ConfigurationSection.cs
- CompositeControl.cs
- MeshGeometry3D.cs
- ActivityBuilderHelper.cs
- UIPermission.cs
- DataColumnPropertyDescriptor.cs
- GeneralTransform2DTo3D.cs
- AvTraceFormat.cs
- DateRangeEvent.cs
- JsonByteArrayDataContract.cs
- EntryWrittenEventArgs.cs
- ModelPerspective.cs
- BStrWrapper.cs
- AesManaged.cs
- RijndaelManagedTransform.cs
- TextServicesManager.cs
- CodeBlockBuilder.cs
- MemberInfoSerializationHolder.cs
- DispatcherHooks.cs
- AuthenticationModeHelper.cs
- Certificate.cs
- ViewStateModeByIdAttribute.cs
- EnterpriseServicesHelper.cs
- WCFModelStrings.Designer.cs
- SqlServer2KCompatibilityCheck.cs
- ConfigurationValues.cs
- Expression.cs
- SemanticKeyElement.cs
- GlyphRun.cs
- WebOperationContext.cs
- SelectionHighlightInfo.cs
- TypeLoadException.cs
- TiffBitmapDecoder.cs
- TransactionState.cs
- HttpCookie.cs
- X509ChainPolicy.cs
- EntityDataSourceStatementEditor.cs
- NavigationService.cs
- ManagedFilter.cs
- XPathItem.cs
- CreateParams.cs
- ThumbAutomationPeer.cs
- CompoundFileStorageReference.cs
- ToolTipAutomationPeer.cs
- StylusSystemGestureEventArgs.cs
- SharedPersonalizationStateInfo.cs
- Constants.cs
- InfoCardArgumentException.cs
- DataSet.cs
- XmlUrlResolver.cs
- EnumValAlphaComparer.cs
- SerializationObjectManager.cs
- SoapClientProtocol.cs
- ProfileEventArgs.cs
- Constants.cs
- SrgsItemList.cs