Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / Common / AuthoringOM / Compiler / CodeGeneration / CodeGenerationManager.cs / 1305376 / CodeGenerationManager.cs
namespace System.Workflow.ComponentModel.Compiler { using System; using System.ComponentModel.Design.Serialization; using System.Collections; using System.Collections.Generic; #region CodeGenerationManager public sealed class CodeGenerationManager : IServiceProvider { private Hashtable hashOfGenerators = new Hashtable(); private IServiceProvider serviceProvider = null; private ContextStack context = null; public CodeGenerationManager(IServiceProvider serviceProvider) { this.serviceProvider = serviceProvider; } public ContextStack Context { get { if (this.context == null) this.context = new ContextStack(); return this.context; } } #region IServiceProvider Members public object GetService(Type serviceType) { if (this.serviceProvider == null) return null; return this.serviceProvider.GetService(serviceType); } #endregion public ActivityCodeGenerator[] GetCodeGenerators(Type type) { if (type == null) throw new ArgumentNullException("type"); if (this.hashOfGenerators.Contains(type)) return ((List)this.hashOfGenerators[type]).ToArray(); List generators = new List (); // Return validators for other types such as Bind, XmolDocument, etc. foreach (ActivityCodeGenerator generator in ComponentDispenser.CreateComponents(type, typeof(ActivityCodeGeneratorAttribute))) { generators.Add(generator); } this.hashOfGenerators[type] = generators; return generators.ToArray(); } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. namespace System.Workflow.ComponentModel.Compiler { using System; using System.ComponentModel.Design.Serialization; using System.Collections; using System.Collections.Generic; #region CodeGenerationManager public sealed class CodeGenerationManager : IServiceProvider { private Hashtable hashOfGenerators = new Hashtable(); private IServiceProvider serviceProvider = null; private ContextStack context = null; public CodeGenerationManager(IServiceProvider serviceProvider) { this.serviceProvider = serviceProvider; } public ContextStack Context { get { if (this.context == null) this.context = new ContextStack(); return this.context; } } #region IServiceProvider Members public object GetService(Type serviceType) { if (this.serviceProvider == null) return null; return this.serviceProvider.GetService(serviceType); } #endregion public ActivityCodeGenerator[] GetCodeGenerators(Type type) { if (type == null) throw new ArgumentNullException("type"); if (this.hashOfGenerators.Contains(type)) return ((List )this.hashOfGenerators[type]).ToArray(); List generators = new List (); // Return validators for other types such as Bind, XmolDocument, etc. foreach (ActivityCodeGenerator generator in ComponentDispenser.CreateComponents(type, typeof(ActivityCodeGeneratorAttribute))) { generators.Add(generator); } this.hashOfGenerators[type] = generators; return generators.ToArray(); } } #endregion } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
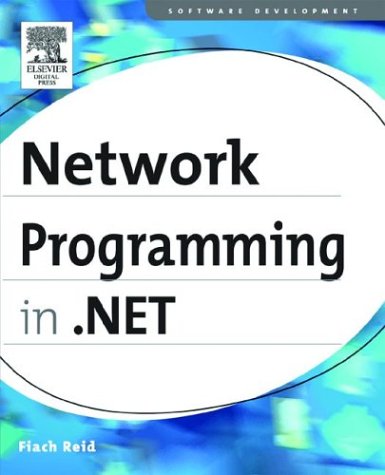
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ManagementEventArgs.cs
- BaseProcessProtocolHandler.cs
- GACIdentityPermission.cs
- FormsAuthentication.cs
- WebScriptEnablingBehavior.cs
- TextBoxAutoCompleteSourceConverter.cs
- Encoder.cs
- FileRecordSequence.cs
- PrintPreviewDialog.cs
- CodeSubDirectory.cs
- TextBox.cs
- CompilerScopeManager.cs
- SqlInternalConnectionSmi.cs
- MetadataSerializer.cs
- UnsafeNativeMethods.cs
- WebPartDeleteVerb.cs
- SqlDataReaderSmi.cs
- DBBindings.cs
- Rectangle.cs
- RegexCapture.cs
- WebContentFormatHelper.cs
- CompleteWizardStep.cs
- Rect3D.cs
- OfTypeExpression.cs
- TextDecoration.cs
- GroupItemAutomationPeer.cs
- InfocardInteractiveChannelInitializer.cs
- WebReferencesBuildProvider.cs
- ShaderRenderModeValidation.cs
- CryptoStream.cs
- ListViewSelectEventArgs.cs
- ToolStripSeparatorRenderEventArgs.cs
- Int64KeyFrameCollection.cs
- SequenceDesigner.xaml.cs
- SchemaImporter.cs
- ResourceWriter.cs
- BooleanStorage.cs
- DoubleCollectionConverter.cs
- ToolStripTextBox.cs
- Metadata.cs
- IIS7UserPrincipal.cs
- UrlAuthorizationModule.cs
- StrongName.cs
- TextParaClient.cs
- DisplayMemberTemplateSelector.cs
- TextAdaptor.cs
- ContainerParaClient.cs
- ServiceContractDetailViewControl.cs
- SharedConnectionWorkflowTransactionService.cs
- CurrentChangingEventManager.cs
- SimpleHandlerBuildProvider.cs
- EraserBehavior.cs
- securitycriticaldata.cs
- DataGridViewSelectedCellCollection.cs
- SecurityPermission.cs
- XPathNode.cs
- Accessible.cs
- CodeTypeDelegate.cs
- _UriTypeConverter.cs
- RepeatBehavior.cs
- DataServiceBehavior.cs
- WebPartZone.cs
- DateTimeOffset.cs
- DataGridViewColumnDividerDoubleClickEventArgs.cs
- WmpBitmapEncoder.cs
- DbProviderFactories.cs
- TextDecorationCollectionConverter.cs
- ChildChangedEventArgs.cs
- ModelEditingScope.cs
- BufferedMessageWriter.cs
- DataGridCommandEventArgs.cs
- ObjectNavigationPropertyMapping.cs
- PrintPageEvent.cs
- AtomMaterializerLog.cs
- complextypematerializer.cs
- BamlLocalizableResourceKey.cs
- GPRECT.cs
- Relationship.cs
- Oci.cs
- DataServiceResponse.cs
- Vertex.cs
- RangeValidator.cs
- MissingSatelliteAssemblyException.cs
- ContextQuery.cs
- CallbackValidatorAttribute.cs
- VSWCFServiceContractGenerator.cs
- BitmapMetadataEnumerator.cs
- SqlDataSourceView.cs
- DataListItemEventArgs.cs
- BuiltInExpr.cs
- Span.cs
- TableCellCollection.cs
- Trace.cs
- UnsafeNativeMethodsTablet.cs
- ReadOnlyDataSource.cs
- ConfigurationCollectionAttribute.cs
- SerializationSectionGroup.cs
- RemoteWebConfigurationHostServer.cs
- CollectionConverter.cs
- PropertyCollection.cs