Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WF / RunTime / Hosting / WorkflowPersistenceService.cs / 1305376 / WorkflowPersistenceService.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.IO; using System.IO.Compression; using System.Workflow.Runtime; using System.Workflow.ComponentModel; using System.Diagnostics; namespace System.Workflow.Runtime.Hosting { ///Service for saving engine state. public abstract class WorkflowPersistenceService : WorkflowRuntimeService { ///Saves the state of a workflow instance. /// The workflow instance state to save internal protected abstract void SaveWorkflowInstanceState(Activity rootActivity, bool unlock); ////// internal protected abstract void UnlockWorkflowInstanceState(Activity rootActivity); /// Loads the state of a workflow instance. /// The unique ID of the instance to load ///The workflow instance state internal protected abstract Activity LoadWorkflowInstanceState(Guid instanceId); ///Saves the state of a completed scope. /// The completed scope to save internal protected abstract void SaveCompletedContextActivity(Activity activity); ///Loads the state of a completed scope /// The unique identifier of the completed scope ///The completed scope or null internal protected abstract Activity LoadCompletedContextActivity(Guid scopeId, Activity outerActivity); ////// /// The value of the "UnloadOnIdle" flag internal protected abstract bool UnloadOnIdle(Activity activity); static protected byte[] GetDefaultSerializedForm(Activity activity) { DateTime startTime = DateTime.Now; Byte[] result; Debug.Assert(activity != null, "Null activity"); using (MemoryStream stream = new MemoryStream(10240)) { stream.Position = 0; activity.Save(stream); using (MemoryStream compressedStream = new MemoryStream((int)stream.Length)) { using (GZipStream gzs = new GZipStream(compressedStream, CompressionMode.Compress, true)) { gzs.Write(stream.GetBuffer(), 0, (int)stream.Length); } ActivityExecutionContextInfo executionContextInfo = (ActivityExecutionContextInfo)activity.GetValue(Activity.ActivityExecutionContextInfoProperty); TimeSpan timeElapsed = DateTime.Now - startTime; WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "Serialized a {0} with id {1} to length {2}. Took {3}.", executionContextInfo, executionContextInfo.ContextGuid, compressedStream.Length, timeElapsed); result = compressedStream.GetBuffer(); Array.Resize(ref result, Convert.ToInt32(compressedStream.Length)); } } return result; } static protected Activity RestoreFromDefaultSerializedForm(Byte[] activityBytes, Activity outerActivity) { DateTime startTime = DateTime.Now; Activity state; MemoryStream stream = new MemoryStream(activityBytes); stream.Position = 0; using (GZipStream gzs = new GZipStream(stream, CompressionMode.Decompress, true)) { state = Activity.Load(gzs, outerActivity); } Debug.Assert(state != null, "invalid state recovered"); TimeSpan timeElapsed = DateTime.Now - startTime; WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "Deserialized a {0} to length {1}. Took {2}.", state, stream.Length, timeElapsed); return state; } static protected internal bool GetIsBlocked(Activity rootActivity) { return (bool)rootActivity.GetValue(WorkflowExecutor.IsBlockedProperty); } static protected internal string GetSuspendOrTerminateInfo(Activity rootActivity) { return (string)rootActivity.GetValue(WorkflowExecutor.SuspendOrTerminateInfoProperty); } static protected internal WorkflowStatus GetWorkflowStatus(Activity rootActivity) { return (WorkflowStatus)rootActivity.GetValue(WorkflowExecutor.WorkflowStatusProperty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.IO; using System.IO.Compression; using System.Workflow.Runtime; using System.Workflow.ComponentModel; using System.Diagnostics; namespace System.Workflow.Runtime.Hosting { ///Service for saving engine state. public abstract class WorkflowPersistenceService : WorkflowRuntimeService { ///Saves the state of a workflow instance. /// The workflow instance state to save internal protected abstract void SaveWorkflowInstanceState(Activity rootActivity, bool unlock); ////// internal protected abstract void UnlockWorkflowInstanceState(Activity rootActivity); /// Loads the state of a workflow instance. /// The unique ID of the instance to load ///The workflow instance state internal protected abstract Activity LoadWorkflowInstanceState(Guid instanceId); ///Saves the state of a completed scope. /// The completed scope to save internal protected abstract void SaveCompletedContextActivity(Activity activity); ///Loads the state of a completed scope /// The unique identifier of the completed scope ///The completed scope or null internal protected abstract Activity LoadCompletedContextActivity(Guid scopeId, Activity outerActivity); ////// /// The value of the "UnloadOnIdle" flag internal protected abstract bool UnloadOnIdle(Activity activity); static protected byte[] GetDefaultSerializedForm(Activity activity) { DateTime startTime = DateTime.Now; Byte[] result; Debug.Assert(activity != null, "Null activity"); using (MemoryStream stream = new MemoryStream(10240)) { stream.Position = 0; activity.Save(stream); using (MemoryStream compressedStream = new MemoryStream((int)stream.Length)) { using (GZipStream gzs = new GZipStream(compressedStream, CompressionMode.Compress, true)) { gzs.Write(stream.GetBuffer(), 0, (int)stream.Length); } ActivityExecutionContextInfo executionContextInfo = (ActivityExecutionContextInfo)activity.GetValue(Activity.ActivityExecutionContextInfoProperty); TimeSpan timeElapsed = DateTime.Now - startTime; WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "Serialized a {0} with id {1} to length {2}. Took {3}.", executionContextInfo, executionContextInfo.ContextGuid, compressedStream.Length, timeElapsed); result = compressedStream.GetBuffer(); Array.Resize(ref result, Convert.ToInt32(compressedStream.Length)); } } return result; } static protected Activity RestoreFromDefaultSerializedForm(Byte[] activityBytes, Activity outerActivity) { DateTime startTime = DateTime.Now; Activity state; MemoryStream stream = new MemoryStream(activityBytes); stream.Position = 0; using (GZipStream gzs = new GZipStream(stream, CompressionMode.Decompress, true)) { state = Activity.Load(gzs, outerActivity); } Debug.Assert(state != null, "invalid state recovered"); TimeSpan timeElapsed = DateTime.Now - startTime; WorkflowTrace.Host.TraceEvent(TraceEventType.Information, 0, "Deserialized a {0} to length {1}. Took {2}.", state, stream.Length, timeElapsed); return state; } static protected internal bool GetIsBlocked(Activity rootActivity) { return (bool)rootActivity.GetValue(WorkflowExecutor.IsBlockedProperty); } static protected internal string GetSuspendOrTerminateInfo(Activity rootActivity) { return (string)rootActivity.GetValue(WorkflowExecutor.SuspendOrTerminateInfoProperty); } static protected internal WorkflowStatus GetWorkflowStatus(Activity rootActivity) { return (WorkflowStatus)rootActivity.GetValue(WorkflowExecutor.WorkflowStatusProperty); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
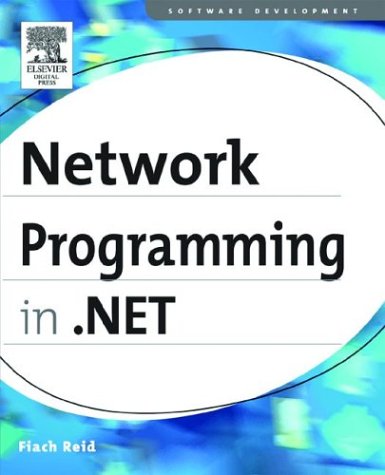
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TypeConvertions.cs
- XmlSchemaSubstitutionGroup.cs
- CompiledRegexRunnerFactory.cs
- BitmapDownload.cs
- CompiledXpathExpr.cs
- SoundPlayerAction.cs
- EndpointIdentityExtension.cs
- TypedTableHandler.cs
- PrintDocument.cs
- Attachment.cs
- PolicyException.cs
- QueryCoreOp.cs
- BooleanSwitch.cs
- JsonClassDataContract.cs
- UserControl.cs
- PriorityRange.cs
- SQLDoubleStorage.cs
- FlowDocument.cs
- Underline.cs
- MetabaseSettingsIis7.cs
- GlyphRunDrawing.cs
- CatalogPartChrome.cs
- IndexedEnumerable.cs
- TimelineGroup.cs
- QilInvoke.cs
- MultiView.cs
- TrackingMemoryStream.cs
- MimeTypePropertyAttribute.cs
- DoubleUtil.cs
- WebPartDescription.cs
- ZipArchive.cs
- OdbcErrorCollection.cs
- Profiler.cs
- MembershipValidatePasswordEventArgs.cs
- DataGridViewAccessibleObject.cs
- SqlConnectionManager.cs
- BooleanAnimationUsingKeyFrames.cs
- DataControlFieldHeaderCell.cs
- DelegateSerializationHolder.cs
- HuffmanTree.cs
- PageHandlerFactory.cs
- CodeAssignStatement.cs
- BinaryObjectReader.cs
- DeclarativeCatalogPart.cs
- RealProxy.cs
- PtsHost.cs
- ActivityStatusChangeEventArgs.cs
- XmlSerializationWriter.cs
- DocumentViewerBase.cs
- BooleanExpr.cs
- NamespaceCollection.cs
- SQLBytes.cs
- Scripts.cs
- RotateTransform.cs
- AttributeUsageAttribute.cs
- AuthorizationSection.cs
- BindingExpressionBase.cs
- WpfPayload.cs
- Thickness.cs
- TextControl.cs
- FormsAuthenticationUserCollection.cs
- MultipleViewPattern.cs
- OdbcEnvironment.cs
- ProcessThread.cs
- XmlnsCache.cs
- ComponentResourceKeyConverter.cs
- TemplatedMailWebEventProvider.cs
- DataColumnPropertyDescriptor.cs
- MenuBase.cs
- ParsedAttributeCollection.cs
- WebRequest.cs
- EndPoint.cs
- httpstaticobjectscollection.cs
- ConsoleTraceListener.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- StylusCollection.cs
- CreatingCookieEventArgs.cs
- IssuanceLicense.cs
- DashStyle.cs
- Message.cs
- ControlUtil.cs
- ColumnReorderedEventArgs.cs
- OleServicesContext.cs
- SecurityElement.cs
- ToolStripRenderer.cs
- DoubleConverter.cs
- IPPacketInformation.cs
- SplitContainer.cs
- DataGridRow.cs
- validationstate.cs
- WithStatement.cs
- XmlSchemaSimpleTypeUnion.cs
- PropertyConverter.cs
- ButtonField.cs
- SelectedGridItemChangedEvent.cs
- XmlAttributeHolder.cs
- ClipboardData.cs
- ControlFilterExpression.cs
- SqlBooleanizer.cs
- WS2007FederationHttpBindingElement.cs