Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Ast / DebugInfoExpression.cs / 1305376 / DebugInfoExpression.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Diagnostics; using System.Dynamic.Utils; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ////// Emits or clears a sequence point for debug information. /// /// This allows the debugger to highlight the correct source code when /// debugging. /// #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.DebugInfoExpressionProxy))] #endif public class DebugInfoExpression : Expression { private readonly SymbolDocumentInfo _document; internal DebugInfoExpression(SymbolDocumentInfo document) { _document = document; } ////// Gets the static type of the expression that this ///represents. (Inherited from .) /// The public sealed override Type Type { get { return typeof(void); } } ///that represents the static type of the expression. /// Returns the node type of this ///. (Inherited from .) /// The public sealed override ExpressionType NodeType { get { return ExpressionType.DebugInfo; } } ///that represents this expression. /// Gets the start line of this public virtual int StartLine { get { throw ContractUtils.Unreachable; } } ///. /// /// Gets the start column of this public virtual int StartColumn { get { throw ContractUtils.Unreachable; } } ///. /// /// Gets the end line of this public virtual int EndLine { get { throw ContractUtils.Unreachable; } } ///. /// /// Gets the end column of this public virtual int EndColumn { get { throw ContractUtils.Unreachable; } } ///. /// /// Gets the public SymbolDocumentInfo Document { get { return _document; } } ///that represents the source file. /// /// Gets the value to indicate if the public virtual bool IsClear { get { throw ContractUtils.Unreachable; } } ///is for clearing a sequence point. /// /// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitDebugInfo(this); } } #region Specialized subclasses internal sealed class SpanDebugInfoExpression : DebugInfoExpression { private readonly int _startLine, _startColumn, _endLine, _endColumn; internal SpanDebugInfoExpression(SymbolDocumentInfo document, int startLine, int startColumn, int endLine, int endColumn) : base(document) { _startLine = startLine; _startColumn = startColumn; _endLine = endLine; _endColumn = endColumn; } public override int StartLine { get { return _startLine; } } public override int StartColumn { get { return _startColumn; } } public override int EndLine { get { return _endLine; } } public override int EndColumn { get { return _endColumn; } } public override bool IsClear { get { return false; } } protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitDebugInfo(this); } } internal sealed class ClearDebugInfoExpression : DebugInfoExpression { internal ClearDebugInfoExpression(SymbolDocumentInfo document) : base(document) { } public override bool IsClear { get { return true; } } public override int StartLine { get { return 0xfeefee; } } public override int StartColumn { get { return 0; } } public override int EndLine { get { return 0xfeefee; } } public override int EndColumn { get { return 0; } } } #endregion public partial class Expression { ////// Creates a /// Thewith the specified span. /// that represents the source file. /// The start line of this . Must be greater than 0. /// The start column of this . Must be greater than 0. /// The end line of this . Must be greater or equal than the start line. /// The end column of this . If the end line is the same as the start line, it must be greater or equal than the start column. In any case, must be greater than 0. /// An instance of public static DebugInfoExpression DebugInfo(SymbolDocumentInfo document, int startLine, int startColumn, int endLine, int endColumn) { ContractUtils.RequiresNotNull(document, "document"); if (startLine == 0xfeefee && startColumn == 0 && endLine == 0xfeefee && endColumn == 0) { return new ClearDebugInfoExpression(document); } ValidateSpan(startLine, startColumn, endLine, endColumn); return new SpanDebugInfoExpression(document, startLine, startColumn, endLine, endColumn); } ///. /// Creates a /// Thefor clearing a sequence point. /// that represents the source file. /// An instance of public static DebugInfoExpression ClearDebugInfo(SymbolDocumentInfo document) { ContractUtils.RequiresNotNull(document, "document"); return new ClearDebugInfoExpression(document); } private static void ValidateSpan(int startLine, int startColumn, int endLine, int endColumn) { if (startLine < 1) { throw Error.OutOfRange("startLine", 1); } if (startColumn < 1) { throw Error.OutOfRange("startColumn", 1); } if (endLine < 1) { throw Error.OutOfRange("endLine", 1); } if (endColumn < 1) { throw Error.OutOfRange("endColumn", 1); } if (startLine > endLine) { throw Error.StartEndMustBeOrdered(); } if (startLine == endLine && startColumn > endColumn) { throw Error.StartEndMustBeOrdered(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Diagnostics; using System.Dynamic.Utils; #if SILVERLIGHT using System.Core; #endif namespace System.Linq.Expressions { ///for clearning a sequence point. /// Emits or clears a sequence point for debug information. /// /// This allows the debugger to highlight the correct source code when /// debugging. /// #if !SILVERLIGHT [DebuggerTypeProxy(typeof(Expression.DebugInfoExpressionProxy))] #endif public class DebugInfoExpression : Expression { private readonly SymbolDocumentInfo _document; internal DebugInfoExpression(SymbolDocumentInfo document) { _document = document; } ////// Gets the static type of the expression that this ///represents. (Inherited from .) /// The public sealed override Type Type { get { return typeof(void); } } ///that represents the static type of the expression. /// Returns the node type of this ///. (Inherited from .) /// The public sealed override ExpressionType NodeType { get { return ExpressionType.DebugInfo; } } ///that represents this expression. /// Gets the start line of this public virtual int StartLine { get { throw ContractUtils.Unreachable; } } ///. /// /// Gets the start column of this public virtual int StartColumn { get { throw ContractUtils.Unreachable; } } ///. /// /// Gets the end line of this public virtual int EndLine { get { throw ContractUtils.Unreachable; } } ///. /// /// Gets the end column of this public virtual int EndColumn { get { throw ContractUtils.Unreachable; } } ///. /// /// Gets the public SymbolDocumentInfo Document { get { return _document; } } ///that represents the source file. /// /// Gets the value to indicate if the public virtual bool IsClear { get { throw ContractUtils.Unreachable; } } ///is for clearing a sequence point. /// /// Dispatches to the specific visit method for this node type. /// protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitDebugInfo(this); } } #region Specialized subclasses internal sealed class SpanDebugInfoExpression : DebugInfoExpression { private readonly int _startLine, _startColumn, _endLine, _endColumn; internal SpanDebugInfoExpression(SymbolDocumentInfo document, int startLine, int startColumn, int endLine, int endColumn) : base(document) { _startLine = startLine; _startColumn = startColumn; _endLine = endLine; _endColumn = endColumn; } public override int StartLine { get { return _startLine; } } public override int StartColumn { get { return _startColumn; } } public override int EndLine { get { return _endLine; } } public override int EndColumn { get { return _endColumn; } } public override bool IsClear { get { return false; } } protected internal override Expression Accept(ExpressionVisitor visitor) { return visitor.VisitDebugInfo(this); } } internal sealed class ClearDebugInfoExpression : DebugInfoExpression { internal ClearDebugInfoExpression(SymbolDocumentInfo document) : base(document) { } public override bool IsClear { get { return true; } } public override int StartLine { get { return 0xfeefee; } } public override int StartColumn { get { return 0; } } public override int EndLine { get { return 0xfeefee; } } public override int EndColumn { get { return 0; } } } #endregion public partial class Expression { ////// Creates a /// Thewith the specified span. /// that represents the source file. /// The start line of this . Must be greater than 0. /// The start column of this . Must be greater than 0. /// The end line of this . Must be greater or equal than the start line. /// The end column of this . If the end line is the same as the start line, it must be greater or equal than the start column. In any case, must be greater than 0. /// An instance of public static DebugInfoExpression DebugInfo(SymbolDocumentInfo document, int startLine, int startColumn, int endLine, int endColumn) { ContractUtils.RequiresNotNull(document, "document"); if (startLine == 0xfeefee && startColumn == 0 && endLine == 0xfeefee && endColumn == 0) { return new ClearDebugInfoExpression(document); } ValidateSpan(startLine, startColumn, endLine, endColumn); return new SpanDebugInfoExpression(document, startLine, startColumn, endLine, endColumn); } ///. /// Creates a /// Thefor clearing a sequence point. /// that represents the source file. /// An instance of public static DebugInfoExpression ClearDebugInfo(SymbolDocumentInfo document) { ContractUtils.RequiresNotNull(document, "document"); return new ClearDebugInfoExpression(document); } private static void ValidateSpan(int startLine, int startColumn, int endLine, int endColumn) { if (startLine < 1) { throw Error.OutOfRange("startLine", 1); } if (startColumn < 1) { throw Error.OutOfRange("startColumn", 1); } if (endLine < 1) { throw Error.OutOfRange("endLine", 1); } if (endColumn < 1) { throw Error.OutOfRange("endColumn", 1); } if (startLine > endLine) { throw Error.StartEndMustBeOrdered(); } if (startLine == endLine && startColumn > endColumn) { throw Error.StartEndMustBeOrdered(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.for clearning a sequence point.
Link Menu
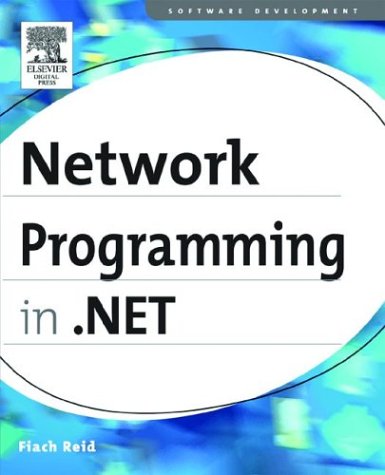
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- COM2PropertyPageUITypeConverter.cs
- XmlAnyElementAttributes.cs
- GlobalAllocSafeHandle.cs
- RowUpdatingEventArgs.cs
- RadioButton.cs
- IProvider.cs
- CompositionTarget.cs
- EdmItemError.cs
- ModelItemImpl.cs
- Events.cs
- ReadingWritingEntityEventArgs.cs
- TextWriterTraceListener.cs
- DesignTimeType.cs
- DataGridTextBoxColumn.cs
- RegexCompilationInfo.cs
- PackageStore.cs
- ValuePattern.cs
- PropertySegmentSerializer.cs
- EventData.cs
- ExpandedWrapper.cs
- DecimalSumAggregationOperator.cs
- XmlValidatingReaderImpl.cs
- X509IssuerSerialKeyIdentifierClause.cs
- StatusBar.cs
- XmlReaderSettings.cs
- PerSessionInstanceContextProvider.cs
- LinearGradientBrush.cs
- TimeSpanMinutesConverter.cs
- RowUpdatedEventArgs.cs
- PageCatalogPart.cs
- XmlSerializerNamespaces.cs
- EmptyImpersonationContext.cs
- OracleBoolean.cs
- DomainConstraint.cs
- ZoneButton.cs
- TextEmbeddedObject.cs
- InputLangChangeRequestEvent.cs
- CopyNamespacesAction.cs
- MobileResource.cs
- RoleServiceManager.cs
- PersonalizationStateQuery.cs
- Vector3DAnimationUsingKeyFrames.cs
- XmlSchemaSequence.cs
- HatchBrush.cs
- WeakEventManager.cs
- DoubleSumAggregationOperator.cs
- MetafileHeaderWmf.cs
- NetworkAddressChange.cs
- ProcessModuleCollection.cs
- JsonReader.cs
- ReceiveReply.cs
- ConnectAlgorithms.cs
- Model3D.cs
- VarRefManager.cs
- SimpleTextLine.cs
- PointAnimationBase.cs
- Identity.cs
- FixedTextBuilder.cs
- TabControl.cs
- DataGridViewCellMouseEventArgs.cs
- ImportCatalogPart.cs
- AvTraceFormat.cs
- ResponseBodyWriter.cs
- SettingsPropertyCollection.cs
- SizeF.cs
- FileStream.cs
- MbpInfo.cs
- PowerEase.cs
- CorrelationTokenTypeConvertor.cs
- Trace.cs
- BamlRecordReader.cs
- DataStreams.cs
- AutoResetEvent.cs
- HwndProxyElementProvider.cs
- HMACSHA1.cs
- BitmapDecoder.cs
- DataGridViewBindingCompleteEventArgs.cs
- ZipIOExtraField.cs
- InternalCompensate.cs
- _IPv6Address.cs
- ConfigurationManagerHelperFactory.cs
- ToolbarAUtomationPeer.cs
- RemoteWebConfigurationHost.cs
- ComboBoxRenderer.cs
- DesignSurfaceServiceContainer.cs
- BindingNavigator.cs
- SiteOfOriginPart.cs
- Stack.cs
- FontStretch.cs
- Ticks.cs
- DockAndAnchorLayout.cs
- IERequestCache.cs
- Color.cs
- TableLayoutStyleCollection.cs
- VectorConverter.cs
- CommandField.cs
- MenuItem.cs
- BindingSource.cs
- ItemCheckEvent.cs
- TabletDeviceInfo.cs