Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / Microsoft / Scripting / Ast / SymbolDocumentInfo.cs / 1305376 / SymbolDocumentInfo.cs
/* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Dynamic.Utils; namespace System.Linq.Expressions { ////// Stores information needed to emit debugging symbol information for a /// source file, in particular the file name and unique language identifier. /// public class SymbolDocumentInfo { private readonly string _fileName; internal SymbolDocumentInfo(string fileName) { ContractUtils.RequiresNotNull(fileName, "fileName"); _fileName = fileName; } ////// The source file name. /// public string FileName { get { return _fileName; } } ////// Returns the language's unique identifier, if any. /// public virtual Guid Language { get { return Guid.Empty; } } ////// Returns the language vendor's unique identifier, if any. /// public virtual Guid LanguageVendor { get { return Guid.Empty; } } ////// Returns the document type's unique identifier, if any. /// Defaults to the guid for a text file. /// public virtual Guid DocumentType { get { return Compiler.SymbolGuids.DocumentType_Text; } } } internal sealed class SymbolDocumentWithGuids : SymbolDocumentInfo { private readonly Guid _language; private readonly Guid _vendor; private readonly Guid _documentType; internal SymbolDocumentWithGuids(string fileName, ref Guid language) : base(fileName) { _language = language; _documentType = Compiler.SymbolGuids.DocumentType_Text; } internal SymbolDocumentWithGuids(string fileName, ref Guid language, ref Guid vendor) : base(fileName) { _language = language; _vendor = vendor; _documentType = Compiler.SymbolGuids.DocumentType_Text; } internal SymbolDocumentWithGuids(string fileName, ref Guid language, ref Guid vendor, ref Guid documentType) : base(fileName) { _language = language; _vendor = vendor; _documentType = documentType; } public override Guid Language { get { return _language; } } public override Guid LanguageVendor { get { return _vendor; } } public override Guid DocumentType { get { return _documentType; } } } public partial class Expression { ////// Creates an instance of /// A. /// to set the equal to. /// A public static SymbolDocumentInfo SymbolDocument(string fileName) { return new SymbolDocumentInfo(fileName); } ///that has the property set to the specified value. /// Creates an instance of /// A. /// to set the equal to. /// A to set the equal to. /// A public static SymbolDocumentInfo SymbolDocument(string fileName, Guid language) { return new SymbolDocumentWithGuids(fileName, ref language); } ///that has the /// and properties set to the specified value. /// Creates an instance of /// A. /// to set the equal to. /// A to set the equal to. /// A to set the equal to. /// A public static SymbolDocumentInfo SymbolDocument(string fileName, Guid language, Guid languageVendor) { return new SymbolDocumentWithGuids(fileName, ref language, ref languageVendor); } ///that has the /// and /// and properties set to the specified value. /// Creates an instance of /// A. /// to set the equal to. /// A to set the equal to. /// A to set the equal to. /// A to set the equal to. /// A public static SymbolDocumentInfo SymbolDocument(string fileName, Guid language, Guid languageVendor, Guid documentType) { return new SymbolDocumentWithGuids(fileName, ref language, ref languageVendor, ref documentType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. /* **************************************************************************** * * Copyright (c) Microsoft Corporation. * * This source code is subject to terms and conditions of the Microsoft Public License. A * copy of the license can be found in the License.html file at the root of this distribution. If * you cannot locate the Microsoft Public License, please send an email to * dlr@microsoft.com. By using this source code in any fashion, you are agreeing to be bound * by the terms of the Microsoft Public License. * * You must not remove this notice, or any other, from this software. * * * ***************************************************************************/ using System.Dynamic.Utils; namespace System.Linq.Expressions { ///that has the /// and /// and /// and properties set to the specified value. /// Stores information needed to emit debugging symbol information for a /// source file, in particular the file name and unique language identifier. /// public class SymbolDocumentInfo { private readonly string _fileName; internal SymbolDocumentInfo(string fileName) { ContractUtils.RequiresNotNull(fileName, "fileName"); _fileName = fileName; } ////// The source file name. /// public string FileName { get { return _fileName; } } ////// Returns the language's unique identifier, if any. /// public virtual Guid Language { get { return Guid.Empty; } } ////// Returns the language vendor's unique identifier, if any. /// public virtual Guid LanguageVendor { get { return Guid.Empty; } } ////// Returns the document type's unique identifier, if any. /// Defaults to the guid for a text file. /// public virtual Guid DocumentType { get { return Compiler.SymbolGuids.DocumentType_Text; } } } internal sealed class SymbolDocumentWithGuids : SymbolDocumentInfo { private readonly Guid _language; private readonly Guid _vendor; private readonly Guid _documentType; internal SymbolDocumentWithGuids(string fileName, ref Guid language) : base(fileName) { _language = language; _documentType = Compiler.SymbolGuids.DocumentType_Text; } internal SymbolDocumentWithGuids(string fileName, ref Guid language, ref Guid vendor) : base(fileName) { _language = language; _vendor = vendor; _documentType = Compiler.SymbolGuids.DocumentType_Text; } internal SymbolDocumentWithGuids(string fileName, ref Guid language, ref Guid vendor, ref Guid documentType) : base(fileName) { _language = language; _vendor = vendor; _documentType = documentType; } public override Guid Language { get { return _language; } } public override Guid LanguageVendor { get { return _vendor; } } public override Guid DocumentType { get { return _documentType; } } } public partial class Expression { ////// Creates an instance of /// A. /// to set the equal to. /// A public static SymbolDocumentInfo SymbolDocument(string fileName) { return new SymbolDocumentInfo(fileName); } ///that has the property set to the specified value. /// Creates an instance of /// A. /// to set the equal to. /// A to set the equal to. /// A public static SymbolDocumentInfo SymbolDocument(string fileName, Guid language) { return new SymbolDocumentWithGuids(fileName, ref language); } ///that has the /// and properties set to the specified value. /// Creates an instance of /// A. /// to set the equal to. /// A to set the equal to. /// A to set the equal to. /// A public static SymbolDocumentInfo SymbolDocument(string fileName, Guid language, Guid languageVendor) { return new SymbolDocumentWithGuids(fileName, ref language, ref languageVendor); } ///that has the /// and /// and properties set to the specified value. /// Creates an instance of /// A. /// to set the equal to. /// A to set the equal to. /// A to set the equal to. /// A to set the equal to. /// A public static SymbolDocumentInfo SymbolDocument(string fileName, Guid language, Guid languageVendor, Guid documentType) { return new SymbolDocumentWithGuids(fileName, ref language, ref languageVendor, ref documentType); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.that has the /// and /// and /// and properties set to the specified value.
Link Menu
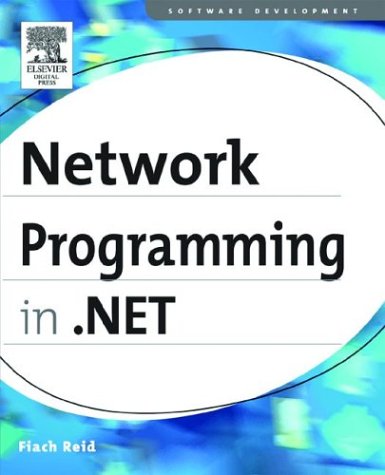
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- EventWaitHandle.cs
- Trace.cs
- OdbcDataAdapter.cs
- xamlnodes.cs
- _NtlmClient.cs
- DataGridTextBoxColumn.cs
- DataObjectFieldAttribute.cs
- ExcludeFromCodeCoverageAttribute.cs
- XmlProcessingInstruction.cs
- EndPoint.cs
- InstanceDataCollectionCollection.cs
- DataObjectSettingDataEventArgs.cs
- Logging.cs
- DataRowView.cs
- TextTrailingCharacterEllipsis.cs
- StatusBarItemAutomationPeer.cs
- GlyphElement.cs
- DataSvcMapFile.cs
- DataKey.cs
- CodeDelegateInvokeExpression.cs
- FacetValueContainer.cs
- UnicodeEncoding.cs
- thaishape.cs
- XhtmlBasicSelectionListAdapter.cs
- GenericPrincipal.cs
- StatusCommandUI.cs
- AuthenticationModuleElement.cs
- StructureChangedEventArgs.cs
- WebResourceAttribute.cs
- XPathScanner.cs
- DecimalConstantAttribute.cs
- BitArray.cs
- ToolTipService.cs
- NamedPermissionSet.cs
- basemetadatamappingvisitor.cs
- TextFormatter.cs
- ProfileInfo.cs
- PageParserFilter.cs
- SynchronizationLockException.cs
- CollaborationHelperFunctions.cs
- TextSelectionProcessor.cs
- CacheAxisQuery.cs
- InvocationExpression.cs
- DeploymentSectionCache.cs
- Marshal.cs
- ObjectParameter.cs
- InvalidOleVariantTypeException.cs
- IconBitmapDecoder.cs
- AssociationSetEnd.cs
- ObjectDataSourceFilteringEventArgs.cs
- BinaryMethodMessage.cs
- CompositeFontParser.cs
- FontConverter.cs
- BindingsCollection.cs
- SortFieldComparer.cs
- JsonCollectionDataContract.cs
- RootBuilder.cs
- BitmapImage.cs
- NotifyParentPropertyAttribute.cs
- SubpageParaClient.cs
- InstanceLockTracking.cs
- EntityDataSourceViewSchema.cs
- SystemNetHelpers.cs
- RegularExpressionValidator.cs
- ResourceReferenceExpressionConverter.cs
- Vector3D.cs
- ResizeGrip.cs
- StylusPoint.cs
- UIElement.cs
- GACIdentityPermission.cs
- SendOperation.cs
- SafeArrayTypeMismatchException.cs
- CodeAttributeDeclarationCollection.cs
- EventMappingSettingsCollection.cs
- CommandSet.cs
- OracleBinary.cs
- WebBrowserHelper.cs
- ExtenderProvidedPropertyAttribute.cs
- DefaultTextStore.cs
- GroupBox.cs
- NameTable.cs
- MailAddressParser.cs
- WorkflowDesignerMessageFilter.cs
- TiffBitmapDecoder.cs
- HelloMessage11.cs
- HttpApplicationFactory.cs
- MeasurementDCInfo.cs
- ObjectConverter.cs
- InfoCardHelper.cs
- KeyConstraint.cs
- WebChannelFactory.cs
- XmlElement.cs
- CollectionViewGroupRoot.cs
- AsymmetricSecurityProtocolFactory.cs
- TextBreakpoint.cs
- MetafileHeader.cs
- DesignTimeTemplateParser.cs
- Pair.cs
- HTTPNotFoundHandler.cs
- FunctionImportElement.cs