Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / CommandTrees / Aggregates.cs / 1305376 / Aggregates.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Data.Common; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Diagnostics; namespace System.Data.Common.CommandTrees { ////// Aggregates are pseudo-expressions. They look and feel like expressions, but /// are severely restricted in where they can appear - only in the aggregates clause /// of a group-by expression. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbAggregate { private readonly DbExpressionList _args; private readonly TypeUsage _type; internal DbAggregate(TypeUsage resultType, DbExpressionList arguments) { Debug.Assert(resultType != null, "DbAggregate.ResultType cannot be null"); Debug.Assert(arguments != null, "DbAggregate.Arguments cannot be null"); Debug.Assert(arguments.Count == 1, "DbAggregate requires a single argument"); this._type = resultType; this._args = arguments; } ////// Gets the result type of this aggregate /// public TypeUsage ResultType { get { return _type; } } ////// Gets the list of expressions that define the arguments to the aggregate. /// public IListArguments { get { return _args; } } } /// /// The aggregate type that corresponds to exposing the collection of elements that comprise a group /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbGroupAggregate : DbAggregate { internal DbGroupAggregate(TypeUsage resultType, DbExpressionList arguments) : base(resultType, arguments) { } } ////// The aggregate type that corresponds to the invocation of an aggregate function. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbFunctionAggregate : DbAggregate { private bool _distinct; EdmFunction _aggregateFunction; internal DbFunctionAggregate(TypeUsage resultType, DbExpressionList arguments, EdmFunction function, bool isDistinct) : base(resultType, arguments) { Debug.Assert(function != null, "DbFunctionAggregate.Function cannot be null"); _aggregateFunction = function; _distinct = isDistinct; } ////// Gets a value indicating whether the aggregate function is applied in a distinct fashion /// public bool Distinct { get { return _distinct; } } ////// Gets the method metadata that specifies the aggregate function to invoke. /// public EdmFunction Function { get { return _aggregateFunction; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Data.Common; using System.Collections.Generic; using System.Data.Metadata.Edm; using System.Data.Common.CommandTrees.Internal; using System.Diagnostics; namespace System.Data.Common.CommandTrees { ////// Aggregates are pseudo-expressions. They look and feel like expressions, but /// are severely restricted in where they can appear - only in the aggregates clause /// of a group-by expression. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public abstract class DbAggregate { private readonly DbExpressionList _args; private readonly TypeUsage _type; internal DbAggregate(TypeUsage resultType, DbExpressionList arguments) { Debug.Assert(resultType != null, "DbAggregate.ResultType cannot be null"); Debug.Assert(arguments != null, "DbAggregate.Arguments cannot be null"); Debug.Assert(arguments.Count == 1, "DbAggregate requires a single argument"); this._type = resultType; this._args = arguments; } ////// Gets the result type of this aggregate /// public TypeUsage ResultType { get { return _type; } } ////// Gets the list of expressions that define the arguments to the aggregate. /// public IListArguments { get { return _args; } } } /// /// The aggregate type that corresponds to exposing the collection of elements that comprise a group /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbGroupAggregate : DbAggregate { internal DbGroupAggregate(TypeUsage resultType, DbExpressionList arguments) : base(resultType, arguments) { } } ////// The aggregate type that corresponds to the invocation of an aggregate function. /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1709:IdentifiersShouldBeCasedCorrectly", MessageId = "Db")] public sealed class DbFunctionAggregate : DbAggregate { private bool _distinct; EdmFunction _aggregateFunction; internal DbFunctionAggregate(TypeUsage resultType, DbExpressionList arguments, EdmFunction function, bool isDistinct) : base(resultType, arguments) { Debug.Assert(function != null, "DbFunctionAggregate.Function cannot be null"); _aggregateFunction = function; _distinct = isDistinct; } ////// Gets a value indicating whether the aggregate function is applied in a distinct fashion /// public bool Distinct { get { return _distinct; } } ////// Gets the method metadata that specifies the aggregate function to invoke. /// public EdmFunction Function { get { return _aggregateFunction; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
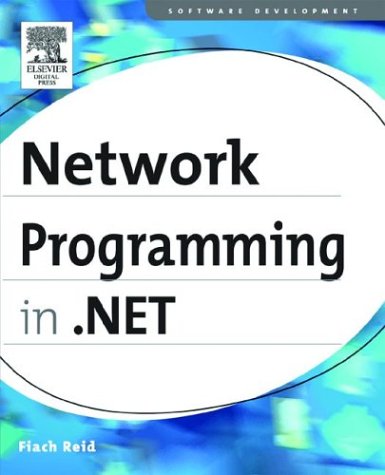
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RelationshipConstraintValidator.cs
- XPathEmptyIterator.cs
- HtmlInputFile.cs
- JapaneseLunisolarCalendar.cs
- DataTableMapping.cs
- MetadataArtifactLoaderComposite.cs
- InteropTrackingRecord.cs
- NotifyCollectionChangedEventArgs.cs
- FrameworkReadOnlyPropertyMetadata.cs
- EntityDataSourceSelectingEventArgs.cs
- HttpDebugHandler.cs
- DataKey.cs
- cookie.cs
- DocumentProperties.cs
- XPathQilFactory.cs
- Encoder.cs
- ListViewInsertEventArgs.cs
- KeyValuePairs.cs
- DataSourceXmlClassAttribute.cs
- StorageAssociationSetMapping.cs
- ColumnResizeAdorner.cs
- FileRecordSequenceHelper.cs
- TraceSource.cs
- UnsafeNativeMethods.cs
- Identity.cs
- TextSelection.cs
- XmlWriter.cs
- LicenseContext.cs
- MultiplexingDispatchMessageFormatter.cs
- Serializer.cs
- ObjectStateManagerMetadata.cs
- Paragraph.cs
- SamlAuthorizationDecisionStatement.cs
- TimelineCollection.cs
- ElementMarkupObject.cs
- DataBoundControlHelper.cs
- PriorityQueue.cs
- TreeIterators.cs
- MetabaseReader.cs
- EdmTypeAttribute.cs
- RangeValidator.cs
- MinMaxParagraphWidth.cs
- TraceAsyncResult.cs
- CheckedListBox.cs
- DrawListViewColumnHeaderEventArgs.cs
- QuotedStringWriteStateInfo.cs
- EntityDataSourceChangingEventArgs.cs
- SqlClientPermission.cs
- OdbcConnectionStringbuilder.cs
- GlyphShapingProperties.cs
- CompositionTarget.cs
- ProfilePropertySettingsCollection.cs
- TextLine.cs
- WebPartActionVerb.cs
- SettingsPropertyCollection.cs
- SQLByteStorage.cs
- PixelShader.cs
- ParallelRangeManager.cs
- Guid.cs
- GroupLabel.cs
- XmlLanguageConverter.cs
- XmlSchemaSimpleTypeRestriction.cs
- SequentialWorkflowRootDesigner.cs
- ACL.cs
- SignedXml.cs
- XmlTypeAttribute.cs
- ConfigurationValidatorAttribute.cs
- FixedDocumentPaginator.cs
- XmlDataSourceView.cs
- SmiConnection.cs
- FormViewPageEventArgs.cs
- QueueProcessor.cs
- TemplateControlParser.cs
- HandlerFactoryCache.cs
- PasswordDeriveBytes.cs
- DataGridSortCommandEventArgs.cs
- CapabilitiesSection.cs
- XhtmlBasicTextViewAdapter.cs
- NotifyCollectionChangedEventArgs.cs
- OrderByQueryOptionExpression.cs
- SqlDependencyListener.cs
- EnvelopedSignatureTransform.cs
- Condition.cs
- TextElementEnumerator.cs
- BuildManagerHost.cs
- DependencyPropertyKey.cs
- SqlClientMetaDataCollectionNames.cs
- TextReader.cs
- Baml2006ReaderFrame.cs
- BindingCompleteEventArgs.cs
- BoundingRectTracker.cs
- XmlAttributes.cs
- DrawingImage.cs
- ResourceExpressionBuilder.cs
- XmlCompatibilityReader.cs
- ListViewUpdatedEventArgs.cs
- ComponentResourceKeyConverter.cs
- DataRecordInfo.cs
- EmbeddedMailObjectsCollection.cs
- ControlIdConverter.cs