Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / Common / Utils / Boolean / KnowledgeBase.cs / 1305376 / KnowledgeBase.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Collections.ObjectModel; using System.Diagnostics; namespace System.Data.Common.Utils.Boolean { ////// Data structure supporting storage of facts and proof (resolution) of queries given /// those facts. /// /// For instance, we may know the following facts: /// /// A --> B /// A /// /// Given these facts, the knowledge base can prove the query: /// /// B /// /// through resolution. /// ///Type of leaf term identifiers in fact expressions. internal class KnowledgeBase{ private readonly List > _facts; private Vertex _knowledge; private readonly ConversionContext _context; /// /// Initialize a new knowledge base. /// internal KnowledgeBase() { _facts = new List>(); _knowledge = Vertex.One; // we know '1', but nothing else at present _context = IdentifierService .Instance.CreateConversionContext(); } /// /// Adds all facts from another knowledge base /// /// The other knowledge base internal void AddKnowledgeBase(KnowledgeBasekb) { foreach (BoolExpr fact in kb._facts) { AddFact(fact); } } /// /// Adds the given fact to this KB. /// /// Simple fact. internal virtual void AddFact(BoolExprfact) { _facts.Add(fact); Converter converter = new Converter (fact, _context); Vertex factVertex = converter.Vertex; _knowledge = _context.Solver.And(_knowledge, factVertex); } /// /// Adds the given implication to this KB, where implication is of the form: /// /// condition --> implies /// /// Condition /// Entailed expression internal void AddImplication(BoolExprcondition, BoolExpr implies) { AddFact(new Implication(condition, implies)); } /// /// Adds an equivalence to this KB, of the form: /// /// left iff. right /// /// Left operand /// Right operand internal void AddEquivalence(BoolExprleft, BoolExpr right) { AddFact(new Equivalence(left, right)); } public override string ToString() { StringBuilder builder = new StringBuilder(); builder.AppendLine("Facts:"); foreach (BoolExpr fact in _facts) { builder.Append("\t").AppendLine(fact.ToString()); } return builder.ToString(); } // Private class improving debugging output for implication facts // (fact appears as A --> B rather than !A + B) private class Implication : OrExpr { BoolExpr _condition; BoolExpr _implies; // (condition --> implies) iff. (!condition OR implies) internal Implication(BoolExpr condition, BoolExpr implies) : base(condition.MakeNegated(), implies) { _condition = condition; _implies = implies; } public override string ToString() { return StringUtil.FormatInvariant("{0} --> {1}", _condition, _implies); } } // Private class improving debugging output for equivalence facts // (fact appears as A <--> B rather than (!A + B) . (A + !B)) private class Equivalence : AndExpr { BoolExpr _left; BoolExpr _right; // (left iff. right) iff. (left --> right AND right --> left) internal Equivalence(BoolExpr left, BoolExpr right) : base(new Implication(left, right), new Implication(right, left)) { _left = left; _right = right; } public override string ToString() { return StringUtil.FormatInvariant("{0} <--> {1}", _left, _right); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- // // Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections.Generic; using System.Text; using System.Globalization; using System.Collections.ObjectModel; using System.Diagnostics; namespace System.Data.Common.Utils.Boolean { ////// Data structure supporting storage of facts and proof (resolution) of queries given /// those facts. /// /// For instance, we may know the following facts: /// /// A --> B /// A /// /// Given these facts, the knowledge base can prove the query: /// /// B /// /// through resolution. /// ///Type of leaf term identifiers in fact expressions. internal class KnowledgeBase{ private readonly List > _facts; private Vertex _knowledge; private readonly ConversionContext _context; /// /// Initialize a new knowledge base. /// internal KnowledgeBase() { _facts = new List>(); _knowledge = Vertex.One; // we know '1', but nothing else at present _context = IdentifierService .Instance.CreateConversionContext(); } /// /// Adds all facts from another knowledge base /// /// The other knowledge base internal void AddKnowledgeBase(KnowledgeBasekb) { foreach (BoolExpr fact in kb._facts) { AddFact(fact); } } /// /// Adds the given fact to this KB. /// /// Simple fact. internal virtual void AddFact(BoolExprfact) { _facts.Add(fact); Converter converter = new Converter (fact, _context); Vertex factVertex = converter.Vertex; _knowledge = _context.Solver.And(_knowledge, factVertex); } /// /// Adds the given implication to this KB, where implication is of the form: /// /// condition --> implies /// /// Condition /// Entailed expression internal void AddImplication(BoolExprcondition, BoolExpr implies) { AddFact(new Implication(condition, implies)); } /// /// Adds an equivalence to this KB, of the form: /// /// left iff. right /// /// Left operand /// Right operand internal void AddEquivalence(BoolExprleft, BoolExpr right) { AddFact(new Equivalence(left, right)); } public override string ToString() { StringBuilder builder = new StringBuilder(); builder.AppendLine("Facts:"); foreach (BoolExpr fact in _facts) { builder.Append("\t").AppendLine(fact.ToString()); } return builder.ToString(); } // Private class improving debugging output for implication facts // (fact appears as A --> B rather than !A + B) private class Implication : OrExpr { BoolExpr _condition; BoolExpr _implies; // (condition --> implies) iff. (!condition OR implies) internal Implication(BoolExpr condition, BoolExpr implies) : base(condition.MakeNegated(), implies) { _condition = condition; _implies = implies; } public override string ToString() { return StringUtil.FormatInvariant("{0} --> {1}", _condition, _implies); } } // Private class improving debugging output for equivalence facts // (fact appears as A <--> B rather than (!A + B) . (A + !B)) private class Equivalence : AndExpr { BoolExpr _left; BoolExpr _right; // (left iff. right) iff. (left --> right AND right --> left) internal Equivalence(BoolExpr left, BoolExpr right) : base(new Implication(left, right), new Implication(right, left)) { _left = left; _right = right; } public override string ToString() { return StringUtil.FormatInvariant("{0} <--> {1}", _left, _right); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
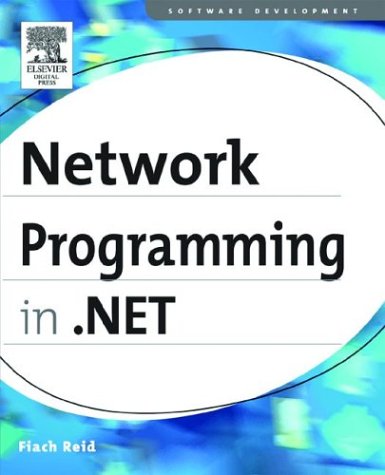
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AttachmentCollection.cs
- UnsafeNativeMethods.cs
- EndpointDiscoveryBehavior.cs
- dataprotectionpermission.cs
- HtmlButton.cs
- SafeNativeMethods.cs
- DataTemplateKey.cs
- TemplatedMailWebEventProvider.cs
- URI.cs
- TransformConverter.cs
- SqlUDTStorage.cs
- DomNameTable.cs
- SafeLibraryHandle.cs
- UserNameServiceElement.cs
- SqlDataSourceStatusEventArgs.cs
- SqlNotificationEventArgs.cs
- RadioButtonRenderer.cs
- ProxyElement.cs
- WebCategoryAttribute.cs
- DispatcherFrame.cs
- SecurityContextKeyIdentifierClause.cs
- _KerberosClient.cs
- MetaModel.cs
- ReferenceSchema.cs
- TransactionFlowOption.cs
- TableRowGroup.cs
- ZipIOLocalFileDataDescriptor.cs
- SHA384Managed.cs
- StatusStrip.cs
- CompilationUnit.cs
- Crypto.cs
- ArgIterator.cs
- HttpClientCertificate.cs
- TableColumnCollection.cs
- ProfessionalColorTable.cs
- SafeProcessHandle.cs
- NullableFloatSumAggregationOperator.cs
- DrawingVisual.cs
- SqlCachedBuffer.cs
- KnownBoxes.cs
- PlanCompiler.cs
- LabelTarget.cs
- GeneralTransform3D.cs
- ReflectionPermission.cs
- ISO2022Encoding.cs
- RemoteWebConfigurationHostStream.cs
- JsonFormatGeneratorStatics.cs
- Pkcs7Signer.cs
- CollectionAdapters.cs
- _HTTPDateParse.cs
- TemplateEditingVerb.cs
- SubstitutionList.cs
- RowParagraph.cs
- BinHexEncoder.cs
- FilterQueryOptionExpression.cs
- MenuTracker.cs
- RichTextBoxConstants.cs
- DataRow.cs
- XmlNodeComparer.cs
- UInt64Converter.cs
- DBCSCodePageEncoding.cs
- SoundPlayer.cs
- BindToObject.cs
- CustomLineCap.cs
- RegexCharClass.cs
- WebPermission.cs
- CodePropertyReferenceExpression.cs
- BitStream.cs
- TdsRecordBufferSetter.cs
- TimeSpanStorage.cs
- DoubleLink.cs
- ScrollProviderWrapper.cs
- KerberosReceiverSecurityToken.cs
- QuaternionRotation3D.cs
- CurrencyManager.cs
- SafeFileMapViewHandle.cs
- Win32SafeHandles.cs
- VisualState.cs
- ThaiBuddhistCalendar.cs
- DataMember.cs
- DataGridColumn.cs
- ResourceDisplayNameAttribute.cs
- CompilationSection.cs
- XmlFormatWriterGenerator.cs
- PartialCachingControl.cs
- FilteredAttributeCollection.cs
- HitTestResult.cs
- CounterSetInstance.cs
- FunctionImportMapping.cs
- ItemCollectionEditor.cs
- Color.cs
- JournalEntryListConverter.cs
- HTMLTagNameToTypeMapper.cs
- MemberRelationshipService.cs
- ThreadAttributes.cs
- DefaultParameterValueAttribute.cs
- HttpCookieCollection.cs
- RawTextInputReport.cs
- AvTrace.cs
- DataRow.cs