Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / SendingRequestEventArgs.cs / 1305376 / SendingRequestEventArgs.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Event args for the event fired before executing a web request. Gives a // chance to customize or replace the request object to be used. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Diagnostics; ////// Event args for the event fired before executing a web request. Gives a /// chance to customize or replace the request object to be used. /// public class SendingRequestEventArgs : EventArgs { ///The web request reported through this event #if ASTORIA_LIGHT [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1823:AvoidUnusedPrivateFields", Justification = "Not used in Silverlight")] #endif private System.Net.WebRequest request; ///The request header collection. private System.Net.WebHeaderCollection requestHeaders; ////// Constructor /// /// The request reported through this event /// The request header collection. internal SendingRequestEventArgs(System.Net.WebRequest request, System.Net.WebHeaderCollection requestHeaders) { // In Silverlight the request object is not accesible #if ASTORIA_LIGHT Debug.Assert(null == request, "non-null request in SL."); #else Debug.Assert(null != request, "null request"); #endif Debug.Assert(null != requestHeaders, "null requestHeaders"); this.request = request; this.requestHeaders = requestHeaders; } #if !ASTORIA_LIGHT // Data.Services http stack ///The web request reported through this event. The handler may modify or replace it. public System.Net.WebRequest Request { get { return this.request; } set { Util.CheckArgumentNull(value, "value"); if (!(value is System.Net.HttpWebRequest)) { throw Error.Argument(Strings.Context_SendingRequestEventArgsNotHttp, "value"); } this.request = value; this.requestHeaders = value.Headers; } } #endif ///The request header collection. public System.Net.WebHeaderCollection RequestHeaders { get { return this.requestHeaders; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Event args for the event fired before executing a web request. Gives a // chance to customize or replace the request object to be used. // //--------------------------------------------------------------------- namespace System.Data.Services.Client { using System; using System.Diagnostics; ////// Event args for the event fired before executing a web request. Gives a /// chance to customize or replace the request object to be used. /// public class SendingRequestEventArgs : EventArgs { ///The web request reported through this event #if ASTORIA_LIGHT [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Performance", "CA1823:AvoidUnusedPrivateFields", Justification = "Not used in Silverlight")] #endif private System.Net.WebRequest request; ///The request header collection. private System.Net.WebHeaderCollection requestHeaders; ////// Constructor /// /// The request reported through this event /// The request header collection. internal SendingRequestEventArgs(System.Net.WebRequest request, System.Net.WebHeaderCollection requestHeaders) { // In Silverlight the request object is not accesible #if ASTORIA_LIGHT Debug.Assert(null == request, "non-null request in SL."); #else Debug.Assert(null != request, "null request"); #endif Debug.Assert(null != requestHeaders, "null requestHeaders"); this.request = request; this.requestHeaders = requestHeaders; } #if !ASTORIA_LIGHT // Data.Services http stack ///The web request reported through this event. The handler may modify or replace it. public System.Net.WebRequest Request { get { return this.request; } set { Util.CheckArgumentNull(value, "value"); if (!(value is System.Net.HttpWebRequest)) { throw Error.Argument(Strings.Context_SendingRequestEventArgsNotHttp, "value"); } this.request = value; this.requestHeaders = value.Headers; } } #endif ///The request header collection. public System.Net.WebHeaderCollection RequestHeaders { get { return this.requestHeaders; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
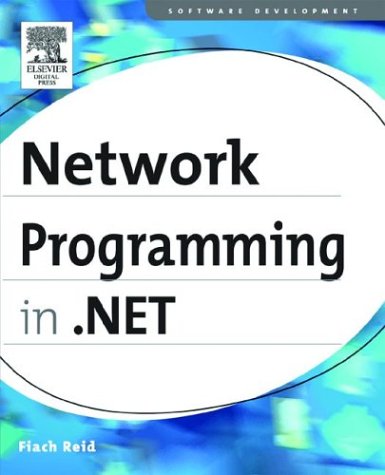
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LineInfo.cs
- ChangesetResponse.cs
- AssemblyResourceLoader.cs
- Rotation3D.cs
- SafeNativeMethods.cs
- IndexOutOfRangeException.cs
- CommandID.cs
- CodeObjectCreateExpression.cs
- BCLDebug.cs
- CreateUserErrorEventArgs.cs
- ApplicationServiceHelper.cs
- PropertyBuilder.cs
- ArgIterator.cs
- MiniAssembly.cs
- basecomparevalidator.cs
- DataObjectAttribute.cs
- EndpointInfo.cs
- FormViewDeletedEventArgs.cs
- WebPartVerb.cs
- MsmqIntegrationReceiveParameters.cs
- ImportFileRequest.cs
- SiteMapNodeItem.cs
- base64Transforms.cs
- HelpProvider.cs
- SqlDataSourceParameterParser.cs
- AspCompat.cs
- RequestTimeoutManager.cs
- AnonymousIdentificationModule.cs
- WebPartZoneAutoFormat.cs
- CheckBoxAutomationPeer.cs
- CalendarAutomationPeer.cs
- RegexWorker.cs
- IdentityManager.cs
- BaseInfoTable.cs
- XmlSchemaCollection.cs
- ToolStripMenuItem.cs
- XamlSerializer.cs
- ScaleTransform3D.cs
- CrossAppDomainChannel.cs
- SignedInfo.cs
- Style.cs
- NextPreviousPagerField.cs
- ArrayWithOffset.cs
- InteropBitmapSource.cs
- ServiceSecurityAuditElement.cs
- OdbcPermission.cs
- TableRow.cs
- TabItemAutomationPeer.cs
- SqlRewriteScalarSubqueries.cs
- FormsIdentity.cs
- HistoryEventArgs.cs
- DPCustomTypeDescriptor.cs
- SchemeSettingElementCollection.cs
- AccessKeyManager.cs
- ToolStripManager.cs
- HttpDebugHandler.cs
- DebuggerService.cs
- BitmapSizeOptions.cs
- PublisherMembershipCondition.cs
- ConstructorExpr.cs
- MdImport.cs
- ObjectPersistData.cs
- TagNameToTypeMapper.cs
- TcpChannelListener.cs
- EventPrivateKey.cs
- DataGridRowClipboardEventArgs.cs
- RemoteDebugger.cs
- FontUnit.cs
- XmlByteStreamWriter.cs
- UnknownWrapper.cs
- NoPersistProperty.cs
- Int32KeyFrameCollection.cs
- LifetimeServices.cs
- GradientStop.cs
- ProgressiveCrcCalculatingStream.cs
- PackagingUtilities.cs
- DecoderReplacementFallback.cs
- Utils.cs
- Configuration.cs
- WindowsStatic.cs
- CngKeyBlobFormat.cs
- TextSpan.cs
- CacheManager.cs
- OLEDB_Util.cs
- PointConverter.cs
- DataFormats.cs
- QuadTree.cs
- SizeAnimation.cs
- XmlDataLoader.cs
- WrappedIUnknown.cs
- RemotingException.cs
- QilNode.cs
- BitmapDownload.cs
- EmptyImpersonationContext.cs
- XmlSiteMapProvider.cs
- ArgumentsParser.cs
- DocumentReference.cs
- PageParserFilter.cs
- XmlAttributeAttribute.cs
- OutputCacheModule.cs