Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Server / System / Data / Services / Providers / RootProjectionNode.cs / 1305376 / RootProjectionNode.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Represents the root of the projection tree // for queries with $expand and/or $select. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { #region Namespaces using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; #endregion ///Internal class which implements the root of the projection tree. ///This class is used to carry information required by our V1 providers /// to able able to fall back to the V1 behavior of using the [DebuggerDisplay("RootProjectionNode {PropertyName}")] internal class RootProjectionNode : ExpandedProjectionNode { #region Private fields ///interface. The collection of expand paths. ///Used by V1 providers to pass the V1 way of representing description /// of expands in the query to the private readonly List. expandPaths; /// The base resource type for all entities in this query. ///This is usually the base resource type of the resource set as well, /// but it can happen that it's a derived type of the resource set base type. private readonly ResourceType baseResourceType; #endregion #region Constructor ///Creates new root node for the projection tree. /// The resource set of the root level of the query. /// The ordering info for this node. null means no ordering to be applied. /// The filter for this node. null means no filter to be applied. /// Number of results to skip. null means no results to be skipped. /// Maximum number of results to return. null means return all available results. /// Maximum number of expected results. Hint that the provider should return /// at least maxResultsExpected + 1 results (if available). /// The list of expanded paths. /// The resource type for all entities in this query. internal RootProjectionNode( ResourceSetWrapper resourceSetWrapper, OrderingInfo orderingInfo, Expression filter, int? skipCount, int? takeCount, int? maxResultsExpected, ListexpandPaths, ResourceType baseResourceType) : base( String.Empty, null, resourceSetWrapper, orderingInfo, filter, skipCount, takeCount, maxResultsExpected) { Debug.Assert(baseResourceType != null, "baseResourceType != null"); this.expandPaths = expandPaths; this.baseResourceType = baseResourceType; } #endregion #region Internal properties /// The resource type in which all the entities expanded by this segment will be of. ///This is usually the resource type of the internal override ResourceType ResourceType { get { return this.baseResourceType; } } ///for this node, /// but it can also be a derived type of that resource type. /// This can happen if navigation property points to a resource set but uses a derived type. /// It can also happen if service operation returns entities from a given resource set /// but it returns derived types. The collection of expand paths. ///Used by V1 providers to pass the V1 way of representing description /// of expands in the query to the internal List. ExpandPaths { get { return this.expandPaths; } } /// Flag which is set when the ExpandPaths property should be used to determine the expanded /// properties to serialize instead of using the ProjectedNode tree. ///This flag is set if the old IExpandProvider was used to process expansions and thus it could have /// modified the ExpandPaths, in which case the serialization needs to use that to comply with the provider. /// Note that this can never be set to true if projections where used in the query, so in that case /// there's no possiblity for the ExpandPaths to differ from the ProjectedNode tree. /// If projections are in place, we only apply the expansion removal caused by "*" projection expressions /// to the tree and leave the ExpandPaths unaffected, as they should not be used in that case. internal bool UseExpandPathsForSerialization { get; set; } ///Flag used to mark that projections were used in the query. internal bool ProjectionsSpecified { get; set; } ///Returns true if there are any expansions in this tree. internal bool ExpansionsSpecified { get; set; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Represents the root of the projection tree // for queries with $expand and/or $select. // // // @owner [....] //--------------------------------------------------------------------- namespace System.Data.Services.Providers { #region Namespaces using System; using System.Collections.Generic; using System.Diagnostics; using System.Linq; using System.Linq.Expressions; #endregion ///Internal class which implements the root of the projection tree. ///This class is used to carry information required by our V1 providers /// to able able to fall back to the V1 behavior of using the [DebuggerDisplay("RootProjectionNode {PropertyName}")] internal class RootProjectionNode : ExpandedProjectionNode { #region Private fields ///interface. The collection of expand paths. ///Used by V1 providers to pass the V1 way of representing description /// of expands in the query to the private readonly List. expandPaths; /// The base resource type for all entities in this query. ///This is usually the base resource type of the resource set as well, /// but it can happen that it's a derived type of the resource set base type. private readonly ResourceType baseResourceType; #endregion #region Constructor ///Creates new root node for the projection tree. /// The resource set of the root level of the query. /// The ordering info for this node. null means no ordering to be applied. /// The filter for this node. null means no filter to be applied. /// Number of results to skip. null means no results to be skipped. /// Maximum number of results to return. null means return all available results. /// Maximum number of expected results. Hint that the provider should return /// at least maxResultsExpected + 1 results (if available). /// The list of expanded paths. /// The resource type for all entities in this query. internal RootProjectionNode( ResourceSetWrapper resourceSetWrapper, OrderingInfo orderingInfo, Expression filter, int? skipCount, int? takeCount, int? maxResultsExpected, ListexpandPaths, ResourceType baseResourceType) : base( String.Empty, null, resourceSetWrapper, orderingInfo, filter, skipCount, takeCount, maxResultsExpected) { Debug.Assert(baseResourceType != null, "baseResourceType != null"); this.expandPaths = expandPaths; this.baseResourceType = baseResourceType; } #endregion #region Internal properties /// The resource type in which all the entities expanded by this segment will be of. ///This is usually the resource type of the internal override ResourceType ResourceType { get { return this.baseResourceType; } } ///for this node, /// but it can also be a derived type of that resource type. /// This can happen if navigation property points to a resource set but uses a derived type. /// It can also happen if service operation returns entities from a given resource set /// but it returns derived types. The collection of expand paths. ///Used by V1 providers to pass the V1 way of representing description /// of expands in the query to the internal List. ExpandPaths { get { return this.expandPaths; } } /// Flag which is set when the ExpandPaths property should be used to determine the expanded /// properties to serialize instead of using the ProjectedNode tree. ///This flag is set if the old IExpandProvider was used to process expansions and thus it could have /// modified the ExpandPaths, in which case the serialization needs to use that to comply with the provider. /// Note that this can never be set to true if projections where used in the query, so in that case /// there's no possiblity for the ExpandPaths to differ from the ProjectedNode tree. /// If projections are in place, we only apply the expansion removal caused by "*" projection expressions /// to the tree and leave the ExpandPaths unaffected, as they should not be used in that case. internal bool UseExpandPathsForSerialization { get; set; } ///Flag used to mark that projections were used in the query. internal bool ProjectionsSpecified { get; set; } ///Returns true if there are any expansions in this tree. internal bool ExpansionsSpecified { get; set; } #endregion } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
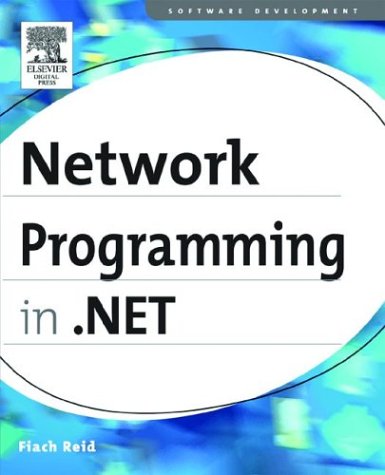
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SectionRecord.cs
- WebPartTransformerCollection.cs
- ObjectRef.cs
- Effect.cs
- DesignerDataStoredProcedure.cs
- DataGridViewUtilities.cs
- InsufficientMemoryException.cs
- MenuItemStyle.cs
- TraceInternal.cs
- ProfilePropertySettingsCollection.cs
- CorrelationManager.cs
- CaseInsensitiveComparer.cs
- StylusLogic.cs
- StrokeCollection.cs
- SourceItem.cs
- AnnotationService.cs
- ProxyElement.cs
- SafeSecurityHelper.cs
- CompiledELinqQueryState.cs
- TCEAdapterGenerator.cs
- OracleCommand.cs
- RtfNavigator.cs
- SmiMetaDataProperty.cs
- ConstrainedDataObject.cs
- PackageFilter.cs
- Column.cs
- DataServiceKeyAttribute.cs
- InvalidPrinterException.cs
- ViewEventArgs.cs
- CodeCompiler.cs
- GCHandleCookieTable.cs
- IUnknownConstantAttribute.cs
- AccessDataSource.cs
- PlainXmlSerializer.cs
- NoClickablePointException.cs
- AssemblyAttributesGoHere.cs
- DispatcherFrame.cs
- Form.cs
- AspNetPartialTrustHelpers.cs
- DataGridViewRowStateChangedEventArgs.cs
- DBConnectionString.cs
- _SslStream.cs
- CopyNamespacesAction.cs
- DispatcherExceptionEventArgs.cs
- AbstractSvcMapFileLoader.cs
- ProvideValueServiceProvider.cs
- ProfessionalColorTable.cs
- Point.cs
- FrameworkElementFactoryMarkupObject.cs
- RSAPKCS1KeyExchangeFormatter.cs
- SmiRequestExecutor.cs
- SafeSystemMetrics.cs
- QueryableFilterRepeater.cs
- CollectionConverter.cs
- LOSFormatter.cs
- ImageKeyConverter.cs
- HttpListenerContext.cs
- DataBoundLiteralControl.cs
- SuppressIldasmAttribute.cs
- DataDocumentXPathNavigator.cs
- DataTableNewRowEvent.cs
- HierarchicalDataSourceControl.cs
- SkipStoryboardToFill.cs
- SamlAttributeStatement.cs
- FixedHighlight.cs
- RoutedEventConverter.cs
- AdornerHitTestResult.cs
- ActivationWorker.cs
- CompressStream.cs
- OuterGlowBitmapEffect.cs
- HttpStreamFormatter.cs
- UserControlBuildProvider.cs
- DetailsViewModeEventArgs.cs
- TextHidden.cs
- InputScopeManager.cs
- BevelBitmapEffect.cs
- WebPartManager.cs
- SelectionEditingBehavior.cs
- SelectQueryOperator.cs
- CommonGetThemePartSize.cs
- Dispatcher.cs
- ZoneMembershipCondition.cs
- BufferAllocator.cs
- CqlQuery.cs
- AssemblyHash.cs
- CodeExporter.cs
- DBDataPermission.cs
- MouseEventArgs.cs
- ExecutionEngineException.cs
- XMLDiffLoader.cs
- TextPenaltyModule.cs
- MailWriter.cs
- ADMembershipUser.cs
- DataTableTypeConverter.cs
- ConnectionPoolManager.cs
- DbCommandTree.cs
- WindowsStatusBar.cs
- XmlNullResolver.cs
- ObjectManager.cs
- SafeHandles.cs