Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Web / System / Web / Services / Discovery / DiscoveryDocument.cs / 1305376 / DiscoveryDocument.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Services.Discovery { using System.Xml.Serialization; using System.Xml; using System.IO; using System; using System.Text; using System.Collections; using System.Web.Services.Configuration; ////// /// [XmlRoot("discovery", Namespace=DiscoveryDocument.Namespace)] public sealed class DiscoveryDocument { ///[To be supplied.] ////// /// public const string Namespace = "http://schemas.xmlsoap.org/disco/"; private ArrayList references = new ArrayList(); ///[To be supplied.] ////// /// public DiscoveryDocument() { } // NOTE, [....]: This property is not really ignored by the xml serializer. Instead, // the attributes that would go here are configured in WebServicesConfiguration's // DiscoveryDocumentSerializer property. ///[To be supplied.] ////// /// [XmlIgnore] public IList References { get { return references; } } ///[To be supplied.] ////// /// public static DiscoveryDocument Read(Stream stream) { XmlTextReader r = new XmlTextReader(stream); r.WhitespaceHandling = WhitespaceHandling.Significant; r.XmlResolver = null; r.DtdProcessing = DtdProcessing.Prohibit; return Read(r); } ///[To be supplied.] ////// /// public static DiscoveryDocument Read(TextReader reader) { XmlTextReader r = new XmlTextReader(reader); r.WhitespaceHandling = WhitespaceHandling.Significant; r.XmlResolver = null; r.DtdProcessing = DtdProcessing.Prohibit; return Read(r); } ///[To be supplied.] ////// /// public static DiscoveryDocument Read(XmlReader xmlReader) { return (DiscoveryDocument) WebServicesSection.Current.DiscoveryDocumentSerializer.Deserialize(xmlReader); } ///[To be supplied.] ////// /// public static bool CanRead(XmlReader xmlReader) { return WebServicesSection.Current.DiscoveryDocumentSerializer.CanDeserialize(xmlReader); } ///[To be supplied.] ////// /// public void Write(TextWriter writer) { XmlTextWriter xmlWriter = new XmlTextWriter(writer); xmlWriter.Formatting = Formatting.Indented; xmlWriter.Indentation = 2; Write(xmlWriter); } ///[To be supplied.] ////// /// public void Write(Stream stream) { TextWriter writer = new StreamWriter(stream, new UTF8Encoding(false)); Write(writer); } ///[To be supplied.] ////// /// public void Write(XmlWriter writer) { XmlSerializer serializer = WebServicesSection.Current.DiscoveryDocumentSerializer; XmlSerializerNamespaces ns = new XmlSerializerNamespaces(); serializer.Serialize(writer, this, ns); } } // This is a special serializer that hardwires to the generated // ServiceDescriptionSerializer. To regenerate the serializer // Turn on KEEPTEMPFILES // Restart server // Run disco as follows // disco[To be supplied.] ///?disco // Goto windows temp dir (usually \winnt\temp) // and get the latest generated .cs file // Change namespace to 'System.Web.Services.Discovery' // Change class names to DiscoveryDocumentSerializationWriter // and DiscoveryDocumentSerializationReader // Make the classes internal // Ensure the public Write method is Write10_discovery (If not // change Serialize to call the new one) // Ensure the public Read method is Read11_discovery (If not // change Deserialize to call the new one) internal class DiscoveryDocumentSerializer : XmlSerializer { protected override XmlSerializationReader CreateReader() { return new DiscoveryDocumentSerializationReader(); } protected override XmlSerializationWriter CreateWriter() { return new DiscoveryDocumentSerializationWriter(); } public override bool CanDeserialize(System.Xml.XmlReader xmlReader){ return xmlReader.IsStartElement("discovery", "http://schemas.xmlsoap.org/disco/"); } protected override void Serialize(Object objectToSerialize, XmlSerializationWriter writer){ ((DiscoveryDocumentSerializationWriter)writer).Write10_discovery(objectToSerialize); } protected override object Deserialize(XmlSerializationReader reader){ return ((DiscoveryDocumentSerializationReader)reader).Read10_discovery(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Web.Services.Discovery { using System.Xml.Serialization; using System.Xml; using System.IO; using System; using System.Text; using System.Collections; using System.Web.Services.Configuration; ////// /// [XmlRoot("discovery", Namespace=DiscoveryDocument.Namespace)] public sealed class DiscoveryDocument { ///[To be supplied.] ////// /// public const string Namespace = "http://schemas.xmlsoap.org/disco/"; private ArrayList references = new ArrayList(); ///[To be supplied.] ////// /// public DiscoveryDocument() { } // NOTE, [....]: This property is not really ignored by the xml serializer. Instead, // the attributes that would go here are configured in WebServicesConfiguration's // DiscoveryDocumentSerializer property. ///[To be supplied.] ////// /// [XmlIgnore] public IList References { get { return references; } } ///[To be supplied.] ////// /// public static DiscoveryDocument Read(Stream stream) { XmlTextReader r = new XmlTextReader(stream); r.WhitespaceHandling = WhitespaceHandling.Significant; r.XmlResolver = null; r.DtdProcessing = DtdProcessing.Prohibit; return Read(r); } ///[To be supplied.] ////// /// public static DiscoveryDocument Read(TextReader reader) { XmlTextReader r = new XmlTextReader(reader); r.WhitespaceHandling = WhitespaceHandling.Significant; r.XmlResolver = null; r.DtdProcessing = DtdProcessing.Prohibit; return Read(r); } ///[To be supplied.] ////// /// public static DiscoveryDocument Read(XmlReader xmlReader) { return (DiscoveryDocument) WebServicesSection.Current.DiscoveryDocumentSerializer.Deserialize(xmlReader); } ///[To be supplied.] ////// /// public static bool CanRead(XmlReader xmlReader) { return WebServicesSection.Current.DiscoveryDocumentSerializer.CanDeserialize(xmlReader); } ///[To be supplied.] ////// /// public void Write(TextWriter writer) { XmlTextWriter xmlWriter = new XmlTextWriter(writer); xmlWriter.Formatting = Formatting.Indented; xmlWriter.Indentation = 2; Write(xmlWriter); } ///[To be supplied.] ////// /// public void Write(Stream stream) { TextWriter writer = new StreamWriter(stream, new UTF8Encoding(false)); Write(writer); } ///[To be supplied.] ////// /// public void Write(XmlWriter writer) { XmlSerializer serializer = WebServicesSection.Current.DiscoveryDocumentSerializer; XmlSerializerNamespaces ns = new XmlSerializerNamespaces(); serializer.Serialize(writer, this, ns); } } // This is a special serializer that hardwires to the generated // ServiceDescriptionSerializer. To regenerate the serializer // Turn on KEEPTEMPFILES // Restart server // Run disco as follows // disco[To be supplied.] ///?disco // Goto windows temp dir (usually \winnt\temp) // and get the latest generated .cs file // Change namespace to 'System.Web.Services.Discovery' // Change class names to DiscoveryDocumentSerializationWriter // and DiscoveryDocumentSerializationReader // Make the classes internal // Ensure the public Write method is Write10_discovery (If not // change Serialize to call the new one) // Ensure the public Read method is Read11_discovery (If not // change Deserialize to call the new one) internal class DiscoveryDocumentSerializer : XmlSerializer { protected override XmlSerializationReader CreateReader() { return new DiscoveryDocumentSerializationReader(); } protected override XmlSerializationWriter CreateWriter() { return new DiscoveryDocumentSerializationWriter(); } public override bool CanDeserialize(System.Xml.XmlReader xmlReader){ return xmlReader.IsStartElement("discovery", "http://schemas.xmlsoap.org/disco/"); } protected override void Serialize(Object objectToSerialize, XmlSerializationWriter writer){ ((DiscoveryDocumentSerializationWriter)writer).Write10_discovery(objectToSerialize); } protected override object Deserialize(XmlSerializationReader reader){ return ((DiscoveryDocumentSerializationReader)reader).Read10_discovery(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
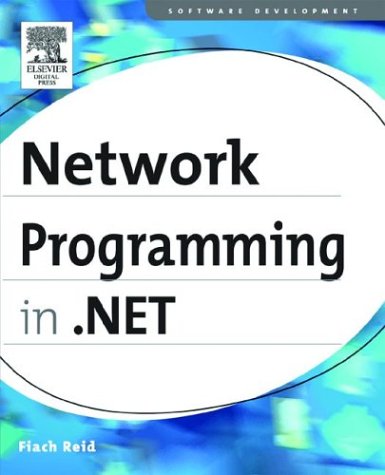
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- tooltip.cs
- ObjectStateFormatter.cs
- AsmxEndpointPickerExtension.cs
- ProviderCollection.cs
- CqlLexer.cs
- ConfigXmlDocument.cs
- GridViewDeleteEventArgs.cs
- HostingPreferredMapPath.cs
- StylusLogic.cs
- NonClientArea.cs
- DrawingVisual.cs
- ToolBarTray.cs
- ObjectTypeMapping.cs
- DbModificationCommandTree.cs
- TimeSpanMinutesConverter.cs
- DependencyProperty.cs
- EmissiveMaterial.cs
- DependencyObjectType.cs
- RegexCompilationInfo.cs
- Int16.cs
- SAPICategories.cs
- ObjectManager.cs
- ConstantSlot.cs
- KeyValuePairs.cs
- ActionItem.cs
- CodeDomConfigurationHandler.cs
- ConsoleKeyInfo.cs
- BitmapEffectInput.cs
- IndexedEnumerable.cs
- ExpressionBindings.cs
- SplineKeyFrames.cs
- PerfService.cs
- TableCell.cs
- HttpApplicationFactory.cs
- BypassElementCollection.cs
- Transform.cs
- StaticExtension.cs
- BoolExpressionVisitors.cs
- TimeSpanSecondsConverter.cs
- HtmlInputText.cs
- ConfigurationValidatorBase.cs
- DataStorage.cs
- SectionXmlInfo.cs
- TypeConverters.cs
- WarningException.cs
- RegisteredExpandoAttribute.cs
- OracleRowUpdatedEventArgs.cs
- ProviderCollection.cs
- ByteKeyFrameCollection.cs
- RestHandlerFactory.cs
- BamlResourceContent.cs
- NotFiniteNumberException.cs
- ConnectionProviderAttribute.cs
- _LazyAsyncResult.cs
- PerformanceCounterPermissionEntry.cs
- MetadataItemEmitter.cs
- HtmlInputRadioButton.cs
- OdbcConnectionString.cs
- QilList.cs
- ObjectFactoryCodeDomTreeGenerator.cs
- MenuItem.cs
- QuadraticBezierSegment.cs
- Compiler.cs
- CollectionViewGroupInternal.cs
- ServiceModelSecurityTokenRequirement.cs
- AsnEncodedData.cs
- LOSFormatter.cs
- LogConverter.cs
- RegionIterator.cs
- ValueProviderWrapper.cs
- ControlBuilderAttribute.cs
- ToolStripHighContrastRenderer.cs
- OleDbDataAdapter.cs
- BamlRecordReader.cs
- GeneralTransformGroup.cs
- DragCompletedEventArgs.cs
- Exception.cs
- RightsManagementPermission.cs
- DynamicRenderer.cs
- CodeTypeDeclaration.cs
- StickyNoteHelper.cs
- RetrieveVirtualItemEventArgs.cs
- UserNamePasswordValidator.cs
- DataService.cs
- AuthenticationModuleElementCollection.cs
- cookieexception.cs
- RootDesignerSerializerAttribute.cs
- InvalidOperationException.cs
- DetailsViewDeleteEventArgs.cs
- ObjectViewEntityCollectionData.cs
- TreeBuilderXamlTranslator.cs
- GuidelineSet.cs
- BaseTemplateBuildProvider.cs
- ActivityTypeDesigner.xaml.cs
- MetafileEditor.cs
- SectionVisual.cs
- PropertyValueUIItem.cs
- ClaimTypes.cs
- JsonFormatMapping.cs
- validationstate.cs