Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Sys / System / Configuration / SettingsPropertyCollection.cs / 1305376 / SettingsPropertyCollection.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Runtime.Serialization; using System.Configuration.Provider; using System.Globalization; using System.IO; using System.Runtime.Serialization.Formatters.Binary; using System.Xml.Serialization; using System.ComponentModel; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public class SettingsPropertyCollection : IEnumerable, ICloneable, ICollection { private Hashtable _Hashtable = null; private bool _ReadOnly = false; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public SettingsPropertyCollection() { _Hashtable = new Hashtable(10, StringComparer.CurrentCultureIgnoreCase); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Add(SettingsProperty property) { if (_ReadOnly) throw new NotSupportedException(); OnAdd(property); _Hashtable.Add(property.Name, property); try { OnAddComplete(property); } catch { _Hashtable.Remove(property.Name); throw; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Remove(string name) { if (_ReadOnly) throw new NotSupportedException(); SettingsProperty toRemove = (SettingsProperty)_Hashtable[name]; if (toRemove == null) return; OnRemove(toRemove); _Hashtable.Remove(name); try { OnRemoveComplete(toRemove); } catch { _Hashtable.Add(name, toRemove); throw; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public SettingsProperty this[string name] { get { return _Hashtable[name] as SettingsProperty; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public IEnumerator GetEnumerator() { return _Hashtable.Values.GetEnumerator(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public object Clone() { return new SettingsPropertyCollection(_Hashtable); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void SetReadOnly() { if (_ReadOnly) return; _ReadOnly = true; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Clear() { if (_ReadOnly) throw new NotSupportedException(); OnClear(); _Hashtable.Clear(); OnClearComplete(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // On* Methods for deriving classes to override. These have // been modeled after the CollectionBase class but have // been stripped of their "index" parameters as there is no // visible index to this collection. protected virtual void OnAdd(SettingsProperty property) {} protected virtual void OnAddComplete(SettingsProperty property) {} protected virtual void OnClear() {} protected virtual void OnClearComplete() {} protected virtual void OnRemove(SettingsProperty property) {} protected virtual void OnRemoveComplete(SettingsProperty property) {} //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // ICollection interface public int Count { get { return _Hashtable.Count; } } public bool IsSynchronized { get { return false; } } public object SyncRoot { get { return this; } } public void CopyTo(Array array, int index) { _Hashtable.Values.CopyTo(array, index); } ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// private SettingsPropertyCollection(Hashtable h) { _Hashtable = (Hashtable)h.Clone(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Configuration { using System.Collections; using System.Collections.Specialized; using System.Runtime.Serialization; using System.Configuration.Provider; using System.Globalization; using System.IO; using System.Runtime.Serialization.Formatters.Binary; using System.Xml.Serialization; using System.ComponentModel; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public class SettingsPropertyCollection : IEnumerable, ICloneable, ICollection { private Hashtable _Hashtable = null; private bool _ReadOnly = false; //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public SettingsPropertyCollection() { _Hashtable = new Hashtable(10, StringComparer.CurrentCultureIgnoreCase); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Add(SettingsProperty property) { if (_ReadOnly) throw new NotSupportedException(); OnAdd(property); _Hashtable.Add(property.Name, property); try { OnAddComplete(property); } catch { _Hashtable.Remove(property.Name); throw; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Remove(string name) { if (_ReadOnly) throw new NotSupportedException(); SettingsProperty toRemove = (SettingsProperty)_Hashtable[name]; if (toRemove == null) return; OnRemove(toRemove); _Hashtable.Remove(name); try { OnRemoveComplete(toRemove); } catch { _Hashtable.Add(name, toRemove); throw; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public SettingsProperty this[string name] { get { return _Hashtable[name] as SettingsProperty; } } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public IEnumerator GetEnumerator() { return _Hashtable.Values.GetEnumerator(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public object Clone() { return new SettingsPropertyCollection(_Hashtable); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void SetReadOnly() { if (_ReadOnly) return; _ReadOnly = true; } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// public void Clear() { if (_ReadOnly) throw new NotSupportedException(); OnClear(); _Hashtable.Clear(); OnClearComplete(); } //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // On* Methods for deriving classes to override. These have // been modeled after the CollectionBase class but have // been stripped of their "index" parameters as there is no // visible index to this collection. protected virtual void OnAdd(SettingsProperty property) {} protected virtual void OnAddComplete(SettingsProperty property) {} protected virtual void OnClear() {} protected virtual void OnClearComplete() {} protected virtual void OnRemove(SettingsProperty property) {} protected virtual void OnRemoveComplete(SettingsProperty property) {} //////////////////////////////////////////////////////////// //////////////////////////////////////////////////////////// // ICollection interface public int Count { get { return _Hashtable.Count; } } public bool IsSynchronized { get { return false; } } public object SyncRoot { get { return this; } } public void CopyTo(Array array, int index) { _Hashtable.Values.CopyTo(array, index); } ///////////////////////////////////////////////////////////////////////////// ///////////////////////////////////////////////////////////////////////////// private SettingsPropertyCollection(Hashtable h) { _Hashtable = (Hashtable)h.Clone(); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
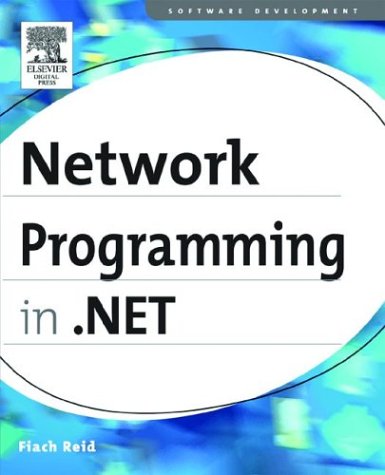
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TemplateControlBuildProvider.cs
- ParseChildrenAsPropertiesAttribute.cs
- ResourceKey.cs
- NavigationProperty.cs
- CompilerResults.cs
- HtmlInputSubmit.cs
- InvalidEnumArgumentException.cs
- XPathArrayIterator.cs
- LocalizableAttribute.cs
- MemberInfoSerializationHolder.cs
- StructureChangedEventArgs.cs
- oledbmetadatacollectionnames.cs
- Button.cs
- InputElement.cs
- IconBitmapDecoder.cs
- BindToObject.cs
- TextCollapsingProperties.cs
- XmlNamespaceManager.cs
- PersistenceTypeAttribute.cs
- StreamingContext.cs
- COM2ExtendedUITypeEditor.cs
- DesignerPerfEventProvider.cs
- SafeNativeMethodsOther.cs
- PropertyKey.cs
- CodeDOMProvider.cs
- Content.cs
- NavigateEvent.cs
- ModelItemDictionary.cs
- contentDescriptor.cs
- Point4D.cs
- InheritanceContextChangedEventManager.cs
- Matrix.cs
- ReflectTypeDescriptionProvider.cs
- TerminateSequence.cs
- CodeTypeReference.cs
- LexicalChunk.cs
- PresentationAppDomainManager.cs
- BlobPersonalizationState.cs
- CanonicalFontFamilyReference.cs
- TextCompositionManager.cs
- XmlComment.cs
- JoinSymbol.cs
- StyleCollection.cs
- InputProviderSite.cs
- Control.cs
- WebContentFormatHelper.cs
- DbParameterHelper.cs
- ServiceEndpointElementCollection.cs
- DockPattern.cs
- Image.cs
- TreeNodeBindingCollection.cs
- XmlSchemaObject.cs
- EntityDataSourceViewSchema.cs
- MissingMemberException.cs
- ErrorFormatter.cs
- ZipIORawDataFileBlock.cs
- DatagridviewDisplayedBandsData.cs
- XmlAttribute.cs
- SHA512Managed.cs
- Expression.cs
- ExpressionNode.cs
- GeneralTransform3DCollection.cs
- EventLogEntryCollection.cs
- PackageDigitalSignatureManager.cs
- MemoryPressure.cs
- ViewManagerAttribute.cs
- ChannelTracker.cs
- infer.cs
- SubclassTypeValidator.cs
- ExportOptions.cs
- TypeUnloadedException.cs
- TextDecoration.cs
- PropertyPushdownHelper.cs
- SecurityRuntime.cs
- SendMailErrorEventArgs.cs
- OdbcEnvironmentHandle.cs
- ResXFileRef.cs
- StylusDevice.cs
- MessagingDescriptionAttribute.cs
- Native.cs
- ConfigXmlWhitespace.cs
- InternalConfigSettingsFactory.cs
- __Error.cs
- SizeAnimationBase.cs
- SemanticResultValue.cs
- WsdlBuildProvider.cs
- TypeElementCollection.cs
- Intellisense.cs
- SecurityHeaderTokenResolver.cs
- Quaternion.cs
- TreeWalker.cs
- ConfigXmlReader.cs
- COM2ICategorizePropertiesHandler.cs
- SqlClientWrapperSmiStreamChars.cs
- WorkflowOperationInvoker.cs
- MarkupWriter.cs
- List.cs
- ObjectViewFactory.cs
- XamlStyleSerializer.cs
- KeyedCollection.cs