Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ContextMenuStrip.cs / 1305376 / ContextMenuStrip.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Drawing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ///this class is just a conceptual wrapper around ToolStripDropDownMenu. [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultEvent("Opening"), SRDescription(SR.DescriptionContextMenuStrip) ] public class ContextMenuStrip : ToolStripDropDownMenu { /// /// Summary of ContextMenuStrip. /// public ContextMenuStrip(IContainer container) : base() { // this constructor ensures ContextMenuStrip is disposed properly since its not parented to the form. if (container == null) { throw new ArgumentNullException("container"); } container.Add(this); } public ContextMenuStrip(){ } protected override void Dispose(bool disposing) { base.Dispose(disposing); } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ContextMenuStripSourceControlDescr) ] public Control SourceControl { [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] get { return SourceControlInternal; } } // minimal Clone implementation for DGV support only. internal ContextMenuStrip Clone() { // VERY limited support for cloning. ContextMenuStrip contextMenuStrip = new ContextMenuStrip(); // copy over events contextMenuStrip.Events.AddHandlers(this.Events); contextMenuStrip.AutoClose = AutoClose; contextMenuStrip.AutoSize = AutoSize; contextMenuStrip.Bounds = Bounds; contextMenuStrip.ImageList = ImageList; contextMenuStrip.ShowCheckMargin = ShowCheckMargin; contextMenuStrip.ShowImageMargin = ShowImageMargin; // copy over relevant properties for (int i = 0; i < Items.Count; i++) { ToolStripItem item = Items[i]; if (item is ToolStripSeparator) { contextMenuStrip.Items.Add(new ToolStripSeparator()); } else if (item is ToolStripMenuItem) { ToolStripMenuItem menuItem = item as ToolStripMenuItem; contextMenuStrip.Items.Add(menuItem.Clone()); } } return contextMenuStrip; } // internal overload so we know whether or not to show mnemonics. internal void ShowInternal(Control source, Point location, bool isKeyboardActivated) { Show(source, location); // if we were activated by keyboard - show mnemonics. if (isKeyboardActivated) { ToolStripManager.ModalMenuFilter.Instance.ShowUnderlines = true; } } internal void ShowInTaskbar(int x, int y) { // we need to make ourselves a topmost window WorkingAreaConstrained = false; Rectangle bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveLeft); Rectangle screenBounds = Screen.FromRectangle(bounds).Bounds; if (bounds.Y < screenBounds.Y) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.BelowLeft); } else if (bounds.X < screenBounds.X) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveRight); } bounds = WindowsFormsUtils.ConstrainToBounds(screenBounds, bounds); Show(bounds.X, bounds.Y); } protected override void SetVisibleCore(bool visible) { if (!visible) { WorkingAreaConstrained = true; } base.SetVisibleCore(visible); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.ComponentModel; using System.Drawing; using System.Runtime.InteropServices; using System.Security; using System.Security.Permissions; ///this class is just a conceptual wrapper around ToolStripDropDownMenu. [ ComVisible(true), ClassInterface(ClassInterfaceType.AutoDispatch), DefaultEvent("Opening"), SRDescription(SR.DescriptionContextMenuStrip) ] public class ContextMenuStrip : ToolStripDropDownMenu { /// /// Summary of ContextMenuStrip. /// public ContextMenuStrip(IContainer container) : base() { // this constructor ensures ContextMenuStrip is disposed properly since its not parented to the form. if (container == null) { throw new ArgumentNullException("container"); } container.Add(this); } public ContextMenuStrip(){ } protected override void Dispose(bool disposing) { base.Dispose(disposing); } [ Browsable(false), DesignerSerializationVisibility(DesignerSerializationVisibility.Hidden), SRDescription(SR.ContextMenuStripSourceControlDescr) ] public Control SourceControl { [UIPermission(SecurityAction.Demand, Window=UIPermissionWindow.AllWindows)] get { return SourceControlInternal; } } // minimal Clone implementation for DGV support only. internal ContextMenuStrip Clone() { // VERY limited support for cloning. ContextMenuStrip contextMenuStrip = new ContextMenuStrip(); // copy over events contextMenuStrip.Events.AddHandlers(this.Events); contextMenuStrip.AutoClose = AutoClose; contextMenuStrip.AutoSize = AutoSize; contextMenuStrip.Bounds = Bounds; contextMenuStrip.ImageList = ImageList; contextMenuStrip.ShowCheckMargin = ShowCheckMargin; contextMenuStrip.ShowImageMargin = ShowImageMargin; // copy over relevant properties for (int i = 0; i < Items.Count; i++) { ToolStripItem item = Items[i]; if (item is ToolStripSeparator) { contextMenuStrip.Items.Add(new ToolStripSeparator()); } else if (item is ToolStripMenuItem) { ToolStripMenuItem menuItem = item as ToolStripMenuItem; contextMenuStrip.Items.Add(menuItem.Clone()); } } return contextMenuStrip; } // internal overload so we know whether or not to show mnemonics. internal void ShowInternal(Control source, Point location, bool isKeyboardActivated) { Show(source, location); // if we were activated by keyboard - show mnemonics. if (isKeyboardActivated) { ToolStripManager.ModalMenuFilter.Instance.ShowUnderlines = true; } } internal void ShowInTaskbar(int x, int y) { // we need to make ourselves a topmost window WorkingAreaConstrained = false; Rectangle bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveLeft); Rectangle screenBounds = Screen.FromRectangle(bounds).Bounds; if (bounds.Y < screenBounds.Y) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.BelowLeft); } else if (bounds.X < screenBounds.X) { bounds = CalculateDropDownLocation(new Point(x,y), ToolStripDropDownDirection.AboveRight); } bounds = WindowsFormsUtils.ConstrainToBounds(screenBounds, bounds); Show(bounds.X, bounds.Y); } protected override void SetVisibleCore(bool visible) { if (!visible) { WorkingAreaConstrained = true; } base.SetVisibleCore(visible); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
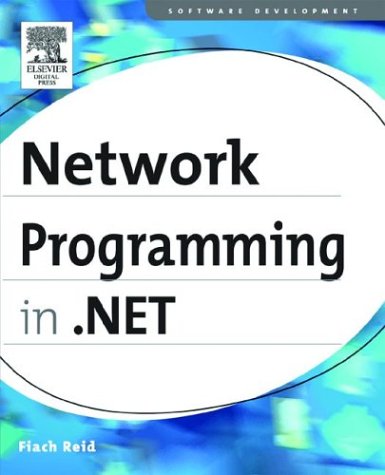
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ManualResetEvent.cs
- Hashtable.cs
- ResourceContainer.cs
- SystemIcmpV6Statistics.cs
- File.cs
- CalendarAutomationPeer.cs
- BrowserTree.cs
- XmlILTrace.cs
- MaterialCollection.cs
- ButtonBase.cs
- DoubleLink.cs
- InvalidComObjectException.cs
- CookieProtection.cs
- WebResourceUtil.cs
- ElementProxy.cs
- MarkerProperties.cs
- PersistChildrenAttribute.cs
- ContainerUIElement3D.cs
- WindowClosedEventArgs.cs
- MultiSelectRootGridEntry.cs
- XmlAttributes.cs
- ToolStripContainer.cs
- LinkLabelLinkClickedEvent.cs
- ArithmeticLiteral.cs
- DataObjectFieldAttribute.cs
- SmtpReplyReaderFactory.cs
- DrawingVisualDrawingContext.cs
- StringConcat.cs
- InvalidOperationException.cs
- ActivityDesignerHelper.cs
- FontFamily.cs
- TokenBasedSetEnumerator.cs
- Boolean.cs
- ReadOnlyDictionary.cs
- ConfigsHelper.cs
- DataColumnCollection.cs
- Tablet.cs
- OdbcFactory.cs
- Attributes.cs
- WebPartVerb.cs
- ArrayHelper.cs
- BuildProviderUtils.cs
- ClientClassGenerator.cs
- DbConnectionStringBuilder.cs
- FlowLayoutSettings.cs
- OdbcConnection.cs
- ErrorInfoXmlDocument.cs
- SpoolingTaskBase.cs
- EventManager.cs
- TargetParameterCountException.cs
- XPathNodeHelper.cs
- ContentElement.cs
- LinkLabelLinkClickedEvent.cs
- NativeActivityContext.cs
- SystemSounds.cs
- DocumentOrderQuery.cs
- CompositeScriptReferenceEventArgs.cs
- XmlSecureResolver.cs
- PointLight.cs
- LogFlushAsyncResult.cs
- EventPrivateKey.cs
- PingOptions.cs
- HtmlTitle.cs
- SequenceQuery.cs
- XPathSelfQuery.cs
- XmlElement.cs
- WebDescriptionAttribute.cs
- DataViewListener.cs
- ConfigXmlReader.cs
- TreeNodeStyleCollection.cs
- httpapplicationstate.cs
- DropShadowBitmapEffect.cs
- HttpApplication.cs
- DictionaryEntry.cs
- BigIntegerStorage.cs
- DragDropHelper.cs
- SupportsPreviewControlAttribute.cs
- SctClaimSerializer.cs
- FixedSOMPageElement.cs
- DocumentPageViewAutomationPeer.cs
- NetTcpSection.cs
- SQLString.cs
- ConnectionManagementSection.cs
- TextEndOfParagraph.cs
- AggregateNode.cs
- ProcessMonitor.cs
- ArgumentValidation.cs
- SecureConversationVersion.cs
- InputElement.cs
- SplitContainer.cs
- DetailsViewPageEventArgs.cs
- DetailsViewPageEventArgs.cs
- DragDeltaEventArgs.cs
- CharacterMetrics.cs
- InputLangChangeEvent.cs
- SharedPersonalizationStateInfo.cs
- ResolvedKeyFrameEntry.cs
- Internal.cs
- XmlBoundElement.cs
- PackageProperties.cs