Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / GDI / TextRenderer.cs / 1305376 / TextRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Internal; using System; using System.Drawing; using System.Windows.Forms.Internal; using System.Diagnostics; ////// /// public sealed class TextRenderer { //cannot instantiate private TextRenderer() { } ////// This class provides API for drawing GDI text. /// ///public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText( text, wf, bounds, foreColor, GetIntTextFormatFlags( flags ) ); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText(text, wf, bounds, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } private static IntTextFormatFlags GetIntTextFormatFlags(TextFormatFlags flags) { if( ((uint)flags & WindowsGraphics.GdiUnsupportedFlagMask) == 0 ) { return (IntTextFormatFlags) flags; } // Clear TextRenderer custom flags. IntTextFormatFlags windowsGraphicsSupportedFlags = (IntTextFormatFlags) ( ((uint)flags) & ~WindowsGraphics.GdiUnsupportedFlagMask ); return windowsGraphicsSupportedFlags; } /// MeasureText wrappers. public static Size MeasureText(string text, Font font ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf); } } public static Size MeasureText(string text, Font font, Size proposedSize ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, WindowsGraphicsCacheManager.GetWindowsFont(font), proposedSize); } } public static Size MeasureText(string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } public static Size MeasureText(IDeviceContext dc, string text, Font font) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf, proposedSize); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using (WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper(dc, flags)) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wgr.WindowsGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } } internal static Color DisabledTextColor(Color backColor) { //Theme specs -- if the backcolor is darker than Control, we use // ControlPaint.Dark(backcolor). Otherwise we use ControlDark. // see VS#357226 Color disabledTextForeColor = SystemColors.ControlDark; if (ControlPaint.IsDarker(backColor, SystemColors.Control)) { disabledTextForeColor = ControlPaint.Dark(backColor); } return disabledTextForeColor; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ // // Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System.Internal; using System; using System.Drawing; using System.Windows.Forms.Internal; using System.Diagnostics; ////// /// public sealed class TextRenderer { //cannot instantiate private TextRenderer() { } ////// This class provides API for drawing GDI text. /// ///public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, pt, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Point pt, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wgr.WindowsGraphics.DrawText(text, wf, pt, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { wg.DrawText(text, wf, bounds, foreColor, backColor); } } } finally { dc.ReleaseHdc(); } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText( text, wf, bounds, foreColor, GetIntTextFormatFlags( flags ) ); } } } /// public static void DrawText(IDeviceContext dc, string text, Font font, Rectangle bounds, Color foreColor, Color backColor, TextFormatFlags flags) { if (dc == null) { throw new ArgumentNullException("dc"); } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using( WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper( dc, flags )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont( font, fontQuality )) { wgr.WindowsGraphics.DrawText(text, wf, bounds, foreColor, backColor, GetIntTextFormatFlags(flags)); } } } private static IntTextFormatFlags GetIntTextFormatFlags(TextFormatFlags flags) { if( ((uint)flags & WindowsGraphics.GdiUnsupportedFlagMask) == 0 ) { return (IntTextFormatFlags) flags; } // Clear TextRenderer custom flags. IntTextFormatFlags windowsGraphicsSupportedFlags = (IntTextFormatFlags) ( ((uint)flags) & ~WindowsGraphics.GdiUnsupportedFlagMask ); return windowsGraphicsSupportedFlags; } /// MeasureText wrappers. public static Size MeasureText(string text, Font font ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf); } } public static Size MeasureText(string text, Font font, Size proposedSize ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, WindowsGraphicsCacheManager.GetWindowsFont(font), proposedSize); } } public static Size MeasureText(string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (string.IsNullOrEmpty(text)) { return Size.Empty; } using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font)) { return WindowsGraphicsCacheManager.MeasurementGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } public static Size MeasureText(IDeviceContext dc, string text, Font font) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); IntPtr hdc = dc.GetHdc(); try { using( WindowsGraphics wg = WindowsGraphics.FromHdc( hdc )) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wg.MeasureText(text, wf, proposedSize); } } } finally { dc.ReleaseHdc(); } } public static Size MeasureText(IDeviceContext dc, string text, Font font, Size proposedSize, TextFormatFlags flags ) { if (dc == null) { throw new ArgumentNullException("dc"); } if (string.IsNullOrEmpty(text)) { return Size.Empty; } WindowsFontQuality fontQuality = WindowsFont.WindowsFontQualityFromTextRenderingHint(dc as Graphics); using (WindowsGraphicsWrapper wgr = new WindowsGraphicsWrapper(dc, flags)) { using (WindowsFont wf = WindowsGraphicsCacheManager.GetWindowsFont(font, fontQuality)) { return wgr.WindowsGraphics.MeasureText(text, wf, proposedSize, GetIntTextFormatFlags(flags)); } } } internal static Color DisabledTextColor(Color backColor) { //Theme specs -- if the backcolor is darker than Control, we use // ControlPaint.Dark(backcolor). Otherwise we use ControlDark. // see VS#357226 Color disabledTextForeColor = SystemColors.ControlDark; if (ControlPaint.IsDarker(backColor, SystemColors.Control)) { disabledTextForeColor = ControlPaint.Dark(backColor); } return disabledTextForeColor; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
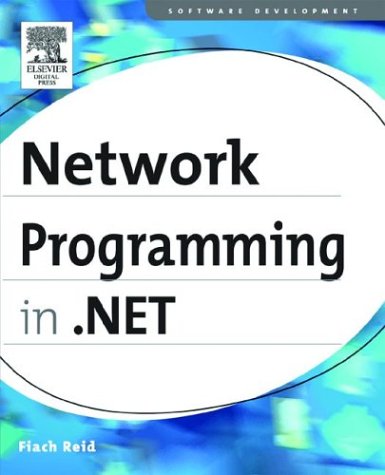
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextTreePropertyUndoUnit.cs
- LineGeometry.cs
- Types.cs
- RequiredAttributeAttribute.cs
- BinHexEncoder.cs
- FileSystemEventArgs.cs
- XmlBinaryReader.cs
- PasswordValidationException.cs
- CardSpaceShim.cs
- Clipboard.cs
- GroupStyle.cs
- ColorTransform.cs
- Propagator.Evaluator.cs
- SplitterEvent.cs
- TreeViewHitTestInfo.cs
- GetPageNumberCompletedEventArgs.cs
- SqlCacheDependency.cs
- PrePrepareMethodAttribute.cs
- Encoding.cs
- ExternalCalls.cs
- HttpCookiesSection.cs
- HwndHost.cs
- SecurityDescriptor.cs
- ByteAnimationBase.cs
- SubclassTypeValidatorAttribute.cs
- CompiledRegexRunnerFactory.cs
- ObfuscateAssemblyAttribute.cs
- DocumentPageViewAutomationPeer.cs
- LinkClickEvent.cs
- VisualTreeFlattener.cs
- DataGridViewUtilities.cs
- fixedPageContentExtractor.cs
- DeclarativeCatalogPart.cs
- DateTimeFormat.cs
- XmlKeywords.cs
- StringToken.cs
- DbDataSourceEnumerator.cs
- SplineKeyFrames.cs
- ContentAlignmentEditor.cs
- BuilderInfo.cs
- JobCollate.cs
- ButtonRenderer.cs
- DataGridViewCellStyleConverter.cs
- WhiteSpaceTrimStringConverter.cs
- InputQueue.cs
- ImageMapEventArgs.cs
- ImpersonateTokenRef.cs
- SecureStringHasher.cs
- CodeDirectionExpression.cs
- QueryInterceptorAttribute.cs
- TemplateBindingExtension.cs
- AuthenticateEventArgs.cs
- ByteConverter.cs
- ReplyChannel.cs
- SqlLiftWhereClauses.cs
- GridItem.cs
- RelationshipType.cs
- WinEventQueueItem.cs
- SqlCacheDependencyDatabaseCollection.cs
- HostedHttpRequestAsyncResult.cs
- Function.cs
- DataGridViewRowHeightInfoNeededEventArgs.cs
- SessionEndingEventArgs.cs
- XpsFilter.cs
- ConsumerConnectionPoint.cs
- PageFunction.cs
- ParseElement.cs
- ValidationError.cs
- WindowsClaimSet.cs
- SimpleExpression.cs
- StringBlob.cs
- WindowsListViewGroupSubsetLink.cs
- SymbolUsageManager.cs
- WinCategoryAttribute.cs
- DateTimePicker.cs
- TableLayoutStyleCollection.cs
- HTTPRemotingHandler.cs
- ClientRuntimeConfig.cs
- HtmlMeta.cs
- TypeDescriptor.cs
- AsmxEndpointPickerExtension.cs
- ClearCollection.cs
- DateBoldEvent.cs
- ImpersonationContext.cs
- WebService.cs
- EventMappingSettings.cs
- TreeViewDesigner.cs
- ListViewUpdateEventArgs.cs
- StreamMarshaler.cs
- ProfileBuildProvider.cs
- ButtonPopupAdapter.cs
- SafeLocalMemHandle.cs
- EtwTrace.cs
- CodeGeneratorOptions.cs
- EntityFrameworkVersions.cs
- RelativeSource.cs
- XmlNode.cs
- SurrogateSelector.cs
- Util.cs
- PageVisual.cs