Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / TextBoxRenderer.cs / 1305376 / TextBoxRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TextBoxRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement TextBoxElement = VisualStyleElement.TextBox.TextEdit.Normal; //cannot instantiate private TextBoxRenderer() { } ////// This is a rendering class for the TextBox control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } private static void DrawBackground(Graphics g, Rectangle bounds, TextBoxState state) { visualStyleRenderer.DrawBackground(g, bounds); if (state != TextBoxState.Disabled) { Color windowColor = visualStyleRenderer.GetColor(ColorProperty.FillColor); if (windowColor != SystemColors.Window) { Rectangle fillRect = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); //then we need to re-fill the background. using(SolidBrush brush = new SolidBrush(SystemColors.Window)) { g.FillRectangle(brush, fillRect); } } } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, TextBoxState state) { InitializeRenderer((int)state); DrawBackground(g, bounds, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, TextBoxState state) { DrawTextBox(g, bounds, textBoxText, font, TextFormatFlags.TextBoxControl, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, Rectangle textBounds, TextBoxState state) { DrawTextBox(g, bounds, textBoxText, font, textBounds, TextFormatFlags.TextBoxControl, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, TextFormatFlags flags, TextBoxState state) { InitializeRenderer((int)state); Rectangle textBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textBounds.Inflate(-2, -2); DrawTextBox(g, bounds, textBoxText, font, textBounds, flags, state); } ////// Renders a TextBox control. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, Rectangle textBounds, TextFormatFlags flags, TextBoxState state) { InitializeRenderer((int)state); DrawBackground(g, bounds, state); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, textBoxText, font, textBounds, textColor, flags); } private static void InitializeRenderer(int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. //------------------------------------------------------------------------------ ///// Renders a TextBox control. /// ///// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class TextBoxRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement TextBoxElement = VisualStyleElement.TextBox.TextEdit.Normal; //cannot instantiate private TextBoxRenderer() { } ////// This is a rendering class for the TextBox control. /// ////// /// public static bool IsSupported { get { return VisualStyleRenderer.IsSupported; // no downlevel support } } private static void DrawBackground(Graphics g, Rectangle bounds, TextBoxState state) { visualStyleRenderer.DrawBackground(g, bounds); if (state != TextBoxState.Disabled) { Color windowColor = visualStyleRenderer.GetColor(ColorProperty.FillColor); if (windowColor != SystemColors.Window) { Rectangle fillRect = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); //then we need to re-fill the background. using(SolidBrush brush = new SolidBrush(SystemColors.Window)) { g.FillRectangle(brush, fillRect); } } } } ////// Returns true if this class is supported for the current OS and user/application settings, /// otherwise returns false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, TextBoxState state) { InitializeRenderer((int)state); DrawBackground(g, bounds, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, TextBoxState state) { DrawTextBox(g, bounds, textBoxText, font, TextFormatFlags.TextBoxControl, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, Rectangle textBounds, TextBoxState state) { DrawTextBox(g, bounds, textBoxText, font, textBounds, TextFormatFlags.TextBoxControl, state); } ////// Renders a TextBox control. /// ////// /// public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, TextFormatFlags flags, TextBoxState state) { InitializeRenderer((int)state); Rectangle textBounds = visualStyleRenderer.GetBackgroundContentRectangle(g, bounds); textBounds.Inflate(-2, -2); DrawTextBox(g, bounds, textBoxText, font, textBounds, flags, state); } ////// Renders a TextBox control. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawTextBox(Graphics g, Rectangle bounds, string textBoxText, Font font, Rectangle textBounds, TextFormatFlags flags, TextBoxState state) { InitializeRenderer((int)state); DrawBackground(g, bounds, state); Color textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); TextRenderer.DrawText(g, textBoxText, font, textBounds, textColor, flags); } private static void InitializeRenderer(int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(TextBoxElement.ClassName, TextBoxElement.Part, state); } else { visualStyleRenderer.SetParameters(TextBoxElement.ClassName, TextBoxElement.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Renders a TextBox control. /// ///
Link Menu
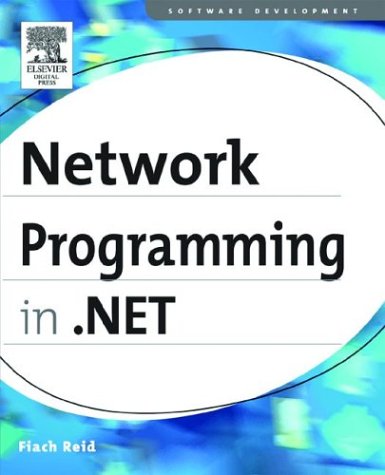
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ScaleTransform3D.cs
- Attributes.cs
- SystemIPInterfaceProperties.cs
- PrintDialog.cs
- TextEditorParagraphs.cs
- DesignerForm.cs
- ImageAnimator.cs
- MutableAssemblyCacheEntry.cs
- FixedPageProcessor.cs
- BlockCollection.cs
- IsolatedStorageFileStream.cs
- ConfigurationElementCollection.cs
- UiaCoreApi.cs
- TableParagraph.cs
- RenderContext.cs
- AutomationIdentifierGuids.cs
- DeferredReference.cs
- RemotingService.cs
- PeerOutputChannel.cs
- HashCryptoHandle.cs
- ContextActivityUtils.cs
- peernodeimplementation.cs
- BufferAllocator.cs
- regiisutil.cs
- EntryWrittenEventArgs.cs
- FormatterServices.cs
- Win32.cs
- HostedElements.cs
- ZipIORawDataFileBlock.cs
- DashStyle.cs
- ToolboxItem.cs
- DataExchangeServiceBinder.cs
- XPathDocumentNavigator.cs
- DesigntimeLicenseContext.cs
- ProcessHostConfigUtils.cs
- XmlSchemaNotation.cs
- DesignerLabelAdapter.cs
- wgx_commands.cs
- StylusPlugInCollection.cs
- BinaryCommonClasses.cs
- ProfileInfo.cs
- SqlTypesSchemaImporter.cs
- XmlSerializationGeneratedCode.cs
- PolicyException.cs
- UserPreferenceChangingEventArgs.cs
- HandlerBase.cs
- BamlLocalizer.cs
- GeneratedView.cs
- ClientTargetSection.cs
- ConsumerConnectionPoint.cs
- Parser.cs
- Composition.cs
- RSAOAEPKeyExchangeDeformatter.cs
- VoiceInfo.cs
- RevocationPoint.cs
- TextRangeProviderWrapper.cs
- ToggleProviderWrapper.cs
- SignatureToken.cs
- PartManifestEntry.cs
- WorkflowDesignerMessageFilter.cs
- SafeRightsManagementSessionHandle.cs
- DataException.cs
- CurrentTimeZone.cs
- ComplexBindingPropertiesAttribute.cs
- GridItemCollection.cs
- BoundPropertyEntry.cs
- LogReserveAndAppendState.cs
- Screen.cs
- WebPartsPersonalizationAuthorization.cs
- Trace.cs
- StateBag.cs
- SerialReceived.cs
- SqlClientWrapperSmiStream.cs
- CallbackValidatorAttribute.cs
- CompModHelpers.cs
- PersonalizationStateInfo.cs
- FacetValues.cs
- ClaimComparer.cs
- InfiniteIntConverter.cs
- DataPagerFieldItem.cs
- QueryableFilterUserControl.cs
- XmlSchemaAnnotation.cs
- FlowchartStart.xaml.cs
- RequestStatusBarUpdateEventArgs.cs
- PeerContact.cs
- ColumnResult.cs
- LiteralControl.cs
- SearchForVirtualItemEventArgs.cs
- _IPv6Address.cs
- GeneratedView.cs
- Simplifier.cs
- LineServices.cs
- DebuggerService.cs
- EnumBuilder.cs
- DataGridState.cs
- QueryOptionExpression.cs
- LookupNode.cs
- WindowsButton.cs
- _NegoStream.cs
- BindingContext.cs