Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Wmi / managed / System / Management / Qualifier.cs / 1305376 / Qualifier.cs
using System; using System.Runtime.InteropServices; using WbemClient_v1; using System.Globalization; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ////// ///Contains information about a WMI qualifier. ////// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// public class QualifierData { private ManagementBaseObject parent; //need access to IWbemClassObject pointer to be able to refresh qualifiers private string propertyOrMethodName; //remains null for object qualifiers private string qualifierName; private QualifierType qualifierType; private Object qualifierValue; private int qualifierFlavor; private IWbemQualifierSetFreeThreaded qualifierSet; internal QualifierData(ManagementBaseObject parent, string propName, string qualName, QualifierType type) { this.parent = parent; this.propertyOrMethodName = propName; this.qualifierName = qualName; this.qualifierType = type; RefreshQualifierInfo(); } //This private function is used to refresh the information from the Wmi object before returning the requested data private void RefreshQualifierInfo() { int status = (int)ManagementStatus.Failed; qualifierSet = null; switch (qualifierType) { case QualifierType.ObjectQualifier : status = parent.wbemObject.GetQualifierSet_(out qualifierSet); break; case QualifierType.PropertyQualifier : status = parent.wbemObject.GetPropertyQualifierSet_(propertyOrMethodName, out qualifierSet); break; case QualifierType.MethodQualifier : status = parent.wbemObject.GetMethodQualifierSet_(propertyOrMethodName, out qualifierSet); break; default : throw new ManagementException(ManagementStatus.Unexpected, null, null); //is this the best fit error ?? } if ((status & 0x80000000) == 0) //success { qualifierValue = null; //Make sure it's null so that we don't leak ! if (qualifierSet != null) status = qualifierSet.Get_(qualifierName, 0, ref qualifierValue, ref qualifierFlavor); } if ((status & 0xfffff000) == 0x80041000) //WMI error ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) //any failure Marshal.ThrowExceptionForHR(status); } private static object MapQualValueToWmiValue(object qualVal) { object wmiValue = System.DBNull.Value; if (null != qualVal) { if (qualVal is Array) { if ((qualVal is Int32[]) || (qualVal is Double[]) || (qualVal is String[]) || (qualVal is Boolean[])) wmiValue = qualVal; else { Array valArray = (Array)qualVal; int length = valArray.Length; //If this is an object array, we cannot use GetElementType() to determine the type //of the actual content. So we'll use the first element to type the array //Type elementType = valArray.GetType().GetElementType(); Type elementType = (length > 0 ? valArray.GetValue(0).GetType() : null); if (elementType == typeof(Int32)) { wmiValue = new Int32 [length]; for (int i = 0; i < length; i++) ((Int32[])(wmiValue))[i] = Convert.ToInt32(valArray.GetValue(i),(IFormatProvider)CultureInfo.InvariantCulture.GetFormat(typeof(System.Int32))); } else if (elementType == typeof(Double)) { wmiValue = new Double [length]; for (int i = 0; i < length; i++) ((Double[])(wmiValue))[i] = Convert.ToDouble(valArray.GetValue(i),(IFormatProvider)CultureInfo.InvariantCulture.GetFormat(typeof(System.Double))); } else if (elementType == typeof(String)) { wmiValue = new String [length]; for (int i = 0; i < length; i++) ((String[])(wmiValue))[i] = (valArray.GetValue(i)).ToString(); } else if (elementType == typeof(Boolean)) { wmiValue = new Boolean [length]; for (int i = 0; i < length; i++) ((Boolean[])(wmiValue))[i] = Convert.ToBoolean(valArray.GetValue(i),(IFormatProvider)CultureInfo.InvariantCulture.GetFormat(typeof(System.Boolean))); } else wmiValue = valArray; //should this throw ? } } else wmiValue = qualVal; } return wmiValue; } ///using System; /// using System.Management; /// /// // This sample demonstrates how to enumerate qualifiers /// // of a ManagementClass object. /// class Sample_QualifierData /// { /// public static int Main(string[] args) { /// ManagementClass diskClass = new ManagementClass("Win32_LogicalDisk"); /// diskClass.Options.UseAmendedQualifiers = true; /// QualifierData diskQualifier = diskClass.Qualifiers["Description"]; /// Console.WriteLine(diskQualifier.Name + " = " + diskQualifier.Value); /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This sample demonstrates how to enumerate qualifiers /// ' of a ManagementClass object. /// Class Sample_QualifierData /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim diskClass As New ManagementClass("win32_logicaldisk") /// diskClass.Options.UseAmendedQualifiers = True /// Dim diskQualifier As QualifierData = diskClass.Qualifiers("Description") /// Console.WriteLine(diskQualifier.Name + " = " + diskQualifier.Value) /// Return 0 /// End Function /// End Class ///
////// ///Represents the name of the qualifier. ////// public string Name { get { return qualifierName != null ? qualifierName : ""; } } ///The name of the qualifier. ////// ///Gets or sets the value of the qualifier. ////// ///The value of the qualifier. ////// public Object Value { get { RefreshQualifierInfo(); return ValueTypeSafety.GetSafeObject(qualifierValue); } set { int status = (int)ManagementStatus.NoError; RefreshQualifierInfo(); object newValue = MapQualValueToWmiValue(value); status = qualifierSet.Put_(qualifierName, ref newValue, qualifierFlavor & ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) Marshal.ThrowExceptionForHR(status); } } ///Qualifiers can only be of the following subset of CIM /// types: ///, , /// , , , /// , , , /// . /// /// ///Gets or sets a value indicating whether the qualifier is amended. ////// ////// if this qualifier is amended; /// otherwise, . /// public bool IsAmended { get { RefreshQualifierInfo(); return ((int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_AMENDED == (qualifierFlavor & (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_AMENDED)); } set { int status = (int)ManagementStatus.NoError; RefreshQualifierInfo (); // Mask out origin bits int flavor = qualifierFlavor & ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN; if (value) flavor |= (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_AMENDED; else flavor &= ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_AMENDED; status = qualifierSet.Put_(qualifierName, ref qualifierValue, flavor); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) Marshal.ThrowExceptionForHR(status); } } ///Amended qualifiers are /// qualifiers whose value can be localized through WMI. Localized qualifiers /// reside in separate namespaces in WMI and can be merged into the basic class /// definition when retrieved. ////// ///Gets or sets a value indicating whether the qualifier has been defined locally on /// this class or has been propagated from a base class. ////// public bool IsLocal { get { RefreshQualifierInfo(); return ((int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_ORIGIN_LOCAL == (qualifierFlavor & (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN)); } } ////// if the qualifier has been defined /// locally on this class; otherwise, . /// ///Gets or sets a value indicating whether the qualifier should be propagated to instances of the /// class. ////// public bool PropagatesToInstance { get { RefreshQualifierInfo(); return ((int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_INSTANCE == (qualifierFlavor & (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_INSTANCE)); } set { int status = (int)ManagementStatus.NoError; RefreshQualifierInfo (); // Mask out origin bits int flavor = qualifierFlavor & ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN; if (value) flavor |= (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_INSTANCE; else flavor &= ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_INSTANCE; status = qualifierSet.Put_(qualifierName, ref qualifierValue, flavor); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) Marshal.ThrowExceptionForHR(status); } } ////// if this qualifier should be /// propagated to instances of the class; otherwise, . /// ///Gets or sets a value indicating whether the qualifier should be propagated to /// subclasses of the class. ////// public bool PropagatesToSubclass { get { RefreshQualifierInfo(); return ((int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_DERIVED_CLASS == (qualifierFlavor & (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_DERIVED_CLASS)); } set { int status = (int)ManagementStatus.NoError; RefreshQualifierInfo (); // Mask out origin bits int flavor = qualifierFlavor & ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN; if (value) flavor |= (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_DERIVED_CLASS; else flavor &= ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_DERIVED_CLASS; status = qualifierSet.Put_(qualifierName, ref qualifierValue, flavor); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) Marshal.ThrowExceptionForHR(status); } } ////// if the qualifier should be /// propagated to subclasses of this class; otherwise, . /// ///Gets or sets a value indicating whether the value of the qualifier can be /// overridden when propagated. ////// public bool IsOverridable { get { RefreshQualifierInfo(); return ((int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_OVERRIDABLE == (qualifierFlavor & (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_PERMISSIONS)); } set { int status = (int)ManagementStatus.NoError; RefreshQualifierInfo (); // Mask out origin bits int flavor = qualifierFlavor & ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN; if (value) flavor &= ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_NOT_OVERRIDABLE; else flavor |= (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_NOT_OVERRIDABLE; status = qualifierSet.Put_(qualifierName, ref qualifierValue, flavor); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) Marshal.ThrowExceptionForHR(status); } } }//QualifierData } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Runtime.InteropServices; using WbemClient_v1; using System.Globalization; namespace System.Management { //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// ////// if the value of the qualifier /// can be overridden when propagated; otherwise, . /// ///Contains information about a WMI qualifier. ////// //CCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCCC// public class QualifierData { private ManagementBaseObject parent; //need access to IWbemClassObject pointer to be able to refresh qualifiers private string propertyOrMethodName; //remains null for object qualifiers private string qualifierName; private QualifierType qualifierType; private Object qualifierValue; private int qualifierFlavor; private IWbemQualifierSetFreeThreaded qualifierSet; internal QualifierData(ManagementBaseObject parent, string propName, string qualName, QualifierType type) { this.parent = parent; this.propertyOrMethodName = propName; this.qualifierName = qualName; this.qualifierType = type; RefreshQualifierInfo(); } //This private function is used to refresh the information from the Wmi object before returning the requested data private void RefreshQualifierInfo() { int status = (int)ManagementStatus.Failed; qualifierSet = null; switch (qualifierType) { case QualifierType.ObjectQualifier : status = parent.wbemObject.GetQualifierSet_(out qualifierSet); break; case QualifierType.PropertyQualifier : status = parent.wbemObject.GetPropertyQualifierSet_(propertyOrMethodName, out qualifierSet); break; case QualifierType.MethodQualifier : status = parent.wbemObject.GetMethodQualifierSet_(propertyOrMethodName, out qualifierSet); break; default : throw new ManagementException(ManagementStatus.Unexpected, null, null); //is this the best fit error ?? } if ((status & 0x80000000) == 0) //success { qualifierValue = null; //Make sure it's null so that we don't leak ! if (qualifierSet != null) status = qualifierSet.Get_(qualifierName, 0, ref qualifierValue, ref qualifierFlavor); } if ((status & 0xfffff000) == 0x80041000) //WMI error ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) //any failure Marshal.ThrowExceptionForHR(status); } private static object MapQualValueToWmiValue(object qualVal) { object wmiValue = System.DBNull.Value; if (null != qualVal) { if (qualVal is Array) { if ((qualVal is Int32[]) || (qualVal is Double[]) || (qualVal is String[]) || (qualVal is Boolean[])) wmiValue = qualVal; else { Array valArray = (Array)qualVal; int length = valArray.Length; //If this is an object array, we cannot use GetElementType() to determine the type //of the actual content. So we'll use the first element to type the array //Type elementType = valArray.GetType().GetElementType(); Type elementType = (length > 0 ? valArray.GetValue(0).GetType() : null); if (elementType == typeof(Int32)) { wmiValue = new Int32 [length]; for (int i = 0; i < length; i++) ((Int32[])(wmiValue))[i] = Convert.ToInt32(valArray.GetValue(i),(IFormatProvider)CultureInfo.InvariantCulture.GetFormat(typeof(System.Int32))); } else if (elementType == typeof(Double)) { wmiValue = new Double [length]; for (int i = 0; i < length; i++) ((Double[])(wmiValue))[i] = Convert.ToDouble(valArray.GetValue(i),(IFormatProvider)CultureInfo.InvariantCulture.GetFormat(typeof(System.Double))); } else if (elementType == typeof(String)) { wmiValue = new String [length]; for (int i = 0; i < length; i++) ((String[])(wmiValue))[i] = (valArray.GetValue(i)).ToString(); } else if (elementType == typeof(Boolean)) { wmiValue = new Boolean [length]; for (int i = 0; i < length; i++) ((Boolean[])(wmiValue))[i] = Convert.ToBoolean(valArray.GetValue(i),(IFormatProvider)CultureInfo.InvariantCulture.GetFormat(typeof(System.Boolean))); } else wmiValue = valArray; //should this throw ? } } else wmiValue = qualVal; } return wmiValue; } ///using System; /// using System.Management; /// /// // This sample demonstrates how to enumerate qualifiers /// // of a ManagementClass object. /// class Sample_QualifierData /// { /// public static int Main(string[] args) { /// ManagementClass diskClass = new ManagementClass("Win32_LogicalDisk"); /// diskClass.Options.UseAmendedQualifiers = true; /// QualifierData diskQualifier = diskClass.Qualifiers["Description"]; /// Console.WriteLine(diskQualifier.Name + " = " + diskQualifier.Value); /// return 0; /// } /// } ///
///Imports System /// Imports System.Management /// /// ' This sample demonstrates how to enumerate qualifiers /// ' of a ManagementClass object. /// Class Sample_QualifierData /// Overloads Public Shared Function Main(args() As String) As Integer /// Dim diskClass As New ManagementClass("win32_logicaldisk") /// diskClass.Options.UseAmendedQualifiers = True /// Dim diskQualifier As QualifierData = diskClass.Qualifiers("Description") /// Console.WriteLine(diskQualifier.Name + " = " + diskQualifier.Value) /// Return 0 /// End Function /// End Class ///
////// ///Represents the name of the qualifier. ////// public string Name { get { return qualifierName != null ? qualifierName : ""; } } ///The name of the qualifier. ////// ///Gets or sets the value of the qualifier. ////// ///The value of the qualifier. ////// public Object Value { get { RefreshQualifierInfo(); return ValueTypeSafety.GetSafeObject(qualifierValue); } set { int status = (int)ManagementStatus.NoError; RefreshQualifierInfo(); object newValue = MapQualValueToWmiValue(value); status = qualifierSet.Put_(qualifierName, ref newValue, qualifierFlavor & ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) Marshal.ThrowExceptionForHR(status); } } ///Qualifiers can only be of the following subset of CIM /// types: ///, , /// , , , /// , , , /// . /// /// ///Gets or sets a value indicating whether the qualifier is amended. ////// ////// if this qualifier is amended; /// otherwise, . /// public bool IsAmended { get { RefreshQualifierInfo(); return ((int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_AMENDED == (qualifierFlavor & (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_AMENDED)); } set { int status = (int)ManagementStatus.NoError; RefreshQualifierInfo (); // Mask out origin bits int flavor = qualifierFlavor & ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN; if (value) flavor |= (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_AMENDED; else flavor &= ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_AMENDED; status = qualifierSet.Put_(qualifierName, ref qualifierValue, flavor); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) Marshal.ThrowExceptionForHR(status); } } ///Amended qualifiers are /// qualifiers whose value can be localized through WMI. Localized qualifiers /// reside in separate namespaces in WMI and can be merged into the basic class /// definition when retrieved. ////// ///Gets or sets a value indicating whether the qualifier has been defined locally on /// this class or has been propagated from a base class. ////// public bool IsLocal { get { RefreshQualifierInfo(); return ((int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_ORIGIN_LOCAL == (qualifierFlavor & (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN)); } } ////// if the qualifier has been defined /// locally on this class; otherwise, . /// ///Gets or sets a value indicating whether the qualifier should be propagated to instances of the /// class. ////// public bool PropagatesToInstance { get { RefreshQualifierInfo(); return ((int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_INSTANCE == (qualifierFlavor & (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_INSTANCE)); } set { int status = (int)ManagementStatus.NoError; RefreshQualifierInfo (); // Mask out origin bits int flavor = qualifierFlavor & ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN; if (value) flavor |= (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_INSTANCE; else flavor &= ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_INSTANCE; status = qualifierSet.Put_(qualifierName, ref qualifierValue, flavor); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) Marshal.ThrowExceptionForHR(status); } } ////// if this qualifier should be /// propagated to instances of the class; otherwise, . /// ///Gets or sets a value indicating whether the qualifier should be propagated to /// subclasses of the class. ////// public bool PropagatesToSubclass { get { RefreshQualifierInfo(); return ((int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_DERIVED_CLASS == (qualifierFlavor & (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_DERIVED_CLASS)); } set { int status = (int)ManagementStatus.NoError; RefreshQualifierInfo (); // Mask out origin bits int flavor = qualifierFlavor & ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN; if (value) flavor |= (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_DERIVED_CLASS; else flavor &= ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_FLAG_PROPAGATE_TO_DERIVED_CLASS; status = qualifierSet.Put_(qualifierName, ref qualifierValue, flavor); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) Marshal.ThrowExceptionForHR(status); } } ////// if the qualifier should be /// propagated to subclasses of this class; otherwise, . /// ///Gets or sets a value indicating whether the value of the qualifier can be /// overridden when propagated. ////// public bool IsOverridable { get { RefreshQualifierInfo(); return ((int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_OVERRIDABLE == (qualifierFlavor & (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_PERMISSIONS)); } set { int status = (int)ManagementStatus.NoError; RefreshQualifierInfo (); // Mask out origin bits int flavor = qualifierFlavor & ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_MASK_ORIGIN; if (value) flavor &= ~(int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_NOT_OVERRIDABLE; else flavor |= (int)tag_WBEM_FLAVOR_TYPE.WBEM_FLAVOR_NOT_OVERRIDABLE; status = qualifierSet.Put_(qualifierName, ref qualifierValue, flavor); if ((status & 0xfffff000) == 0x80041000) ManagementException.ThrowWithExtendedInfo((ManagementStatus)status); else if ((status & 0x80000000) != 0) Marshal.ThrowExceptionForHR(status); } } }//QualifierData } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved./// if the value of the qualifier /// can be overridden when propagated; otherwise, .
Link Menu
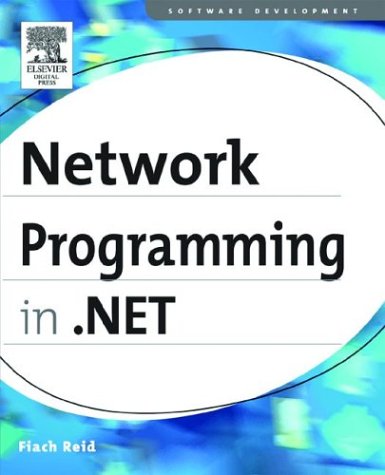
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SqlDataReaderSmi.cs
- JavaScriptObjectDeserializer.cs
- Control.cs
- HtmlEncodedRawTextWriter.cs
- SecurityException.cs
- Viewport2DVisual3D.cs
- TypeUtil.cs
- HostedBindingBehavior.cs
- Path.cs
- ProjectionPruner.cs
- Expression.cs
- Triangle.cs
- WSDualHttpSecurityElement.cs
- Aggregates.cs
- MachineKeySection.cs
- ResourcesBuildProvider.cs
- FilterQuery.cs
- DropShadowBitmapEffect.cs
- XmlAttributeAttribute.cs
- xamlnodes.cs
- TranslateTransform3D.cs
- ExeContext.cs
- FieldNameLookup.cs
- BitmapCodecInfo.cs
- PolicyLevel.cs
- IpcClientManager.cs
- BinarySerializer.cs
- BitmapMetadataBlob.cs
- DataGridViewSelectedCellsAccessibleObject.cs
- SoapAttributeOverrides.cs
- IFlowDocumentViewer.cs
- DataGridViewColumnConverter.cs
- DataStreams.cs
- ConcurrentQueue.cs
- BaseTemplateCodeDomTreeGenerator.cs
- SrgsSubset.cs
- DataGridPageChangedEventArgs.cs
- ObjRef.cs
- SessionParameter.cs
- TypeKeyValue.cs
- DiffuseMaterial.cs
- OleDbTransaction.cs
- LineInfo.cs
- NetNamedPipeBindingElement.cs
- KerberosSecurityTokenProvider.cs
- ReferencedType.cs
- CodeIdentifier.cs
- RenderData.cs
- DBDataPermission.cs
- ScrollBar.cs
- AssemblyBuilder.cs
- ConfigurationErrorsException.cs
- HttpProfileGroupBase.cs
- CollectionBase.cs
- ServicePoint.cs
- FacetDescription.cs
- FormViewPageEventArgs.cs
- EditorZone.cs
- TreeNode.cs
- Converter.cs
- FieldNameLookup.cs
- PropertyDescriptorComparer.cs
- RadioButton.cs
- OpacityConverter.cs
- HttpConfigurationSystem.cs
- Classification.cs
- DataGridCellItemAutomationPeer.cs
- processwaithandle.cs
- XmlSchemaAll.cs
- NotCondition.cs
- XPathNavigatorReader.cs
- StrokeNodeEnumerator.cs
- DecoderFallback.cs
- ObjectStateEntryOriginalDbUpdatableDataRecord.cs
- WindowCollection.cs
- SqlCacheDependencyDatabaseCollection.cs
- XPathDocumentNavigator.cs
- CLSCompliantAttribute.cs
- TemplateBindingExpressionConverter.cs
- LineGeometry.cs
- SqlConnectionFactory.cs
- TableCell.cs
- DocumentStatusResources.cs
- BaseDataList.cs
- ProfileInfo.cs
- WebFormsRootDesigner.cs
- OpenFileDialog.cs
- XmlILModule.cs
- PanelStyle.cs
- PhysicalOps.cs
- EnumBuilder.cs
- SecurityCriticalDataForSet.cs
- SingleConverter.cs
- DataGridPageChangedEventArgs.cs
- OperationAbortedException.cs
- FamilyMap.cs
- DbgUtil.cs
- PathGeometry.cs
- WebBrowsableAttribute.cs
- Accessors.cs