Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / xsp / System / Extensions / UI / WebControls / Expressions / ExpressionHelper.cs / 1305376 / ExpressionHelper.cs
#if ORYX_VNEXT namespace Microsoft.Web.Data.UI.WebControls.Expressions { #else namespace System.Web.UI.WebControls.Expressions { #endif using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; internal static class ExpressionHelper { public static Expression GetValue(Expression exp) { Type realType = GetUnderlyingType(exp.Type); if (realType == exp.Type) { return exp; } return Expression.Convert(exp, realType); } public static Type GetUnderlyingType(Type type) { // Get the type from Nullable types if (type.IsGenericType && type.GetGenericTypeDefinition() == typeof(Nullable<>)) { return type.GetGenericArguments()[0]; } return type; } public static object BuildObjectValue(object value, Type type) { #if ORYX_VNEXT return Microsoft.Web.Data.UI.WebControls.DataSourceHelper.BuildObjectValue(value, type, String.Empty); #else return System.Web.UI.WebControls.DataSourceHelper.BuildObjectValue(value, type, String.Empty); #endif } public static Expression CreatePropertyExpression(Expression parameterExpression, string propertyName) { if (parameterExpression == null) { return null; } if (String.IsNullOrEmpty(propertyName)) { return null; } Expression propExpression = null; string[] props = propertyName.Split('.'); foreach (var p in props) { if (propExpression == null) { propExpression = Expression.PropertyOrField(parameterExpression, p); } else { propExpression = Expression.PropertyOrField(propExpression, p); } } return propExpression; } public static IQueryable Where(this IQueryable source, LambdaExpression lambda) { return Call(source, "Where", lambda, source.ElementType); } public static IQueryable Call(this IQueryable source, string queryMethod, Type[] genericArgs, params Expression[] arguments) { if (source == null) { throw new ArgumentNullException("source"); } return source.Provider.CreateQuery( Expression.Call( typeof(Queryable), queryMethod, genericArgs, arguments)); } public static IQueryable Call(this IQueryable source, string queryableMethod, LambdaExpression lambda, params Type[] genericArgs) { if (source == null) { throw new ArgumentNullException("source"); } return source.Provider.CreateQuery( Expression.Call( typeof(Queryable), queryableMethod, genericArgs, source.Expression, Expression.Quote(lambda))); } public static Expression Or(IEnumerableexpressions) { Expression orExpression = null; foreach (Expression e in expressions) { if (e == null) { continue; } if (orExpression == null) { orExpression = e; } else { orExpression = Expression.OrElse(orExpression, e); } } return orExpression; } public static Expression And(IEnumerable expressions) { Expression andExpression = null; foreach (Expression e in expressions) { if (e == null) { continue; } if (andExpression == null) { andExpression = e; } else { andExpression = Expression.AndAlso(andExpression, e); } } return andExpression; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. #if ORYX_VNEXT namespace Microsoft.Web.Data.UI.WebControls.Expressions { #else namespace System.Web.UI.WebControls.Expressions { #endif using System; using System.Collections.Generic; using System.Linq; using System.Linq.Expressions; internal static class ExpressionHelper { public static Expression GetValue(Expression exp) { Type realType = GetUnderlyingType(exp.Type); if (realType == exp.Type) { return exp; } return Expression.Convert(exp, realType); } public static Type GetUnderlyingType(Type type) { // Get the type from Nullable types if (type.IsGenericType && type.GetGenericTypeDefinition() == typeof(Nullable<>)) { return type.GetGenericArguments()[0]; } return type; } public static object BuildObjectValue(object value, Type type) { #if ORYX_VNEXT return Microsoft.Web.Data.UI.WebControls.DataSourceHelper.BuildObjectValue(value, type, String.Empty); #else return System.Web.UI.WebControls.DataSourceHelper.BuildObjectValue(value, type, String.Empty); #endif } public static Expression CreatePropertyExpression(Expression parameterExpression, string propertyName) { if (parameterExpression == null) { return null; } if (String.IsNullOrEmpty(propertyName)) { return null; } Expression propExpression = null; string[] props = propertyName.Split('.'); foreach (var p in props) { if (propExpression == null) { propExpression = Expression.PropertyOrField(parameterExpression, p); } else { propExpression = Expression.PropertyOrField(propExpression, p); } } return propExpression; } public static IQueryable Where(this IQueryable source, LambdaExpression lambda) { return Call(source, "Where", lambda, source.ElementType); } public static IQueryable Call(this IQueryable source, string queryMethod, Type[] genericArgs, params Expression[] arguments) { if (source == null) { throw new ArgumentNullException("source"); } return source.Provider.CreateQuery( Expression.Call( typeof(Queryable), queryMethod, genericArgs, arguments)); } public static IQueryable Call(this IQueryable source, string queryableMethod, LambdaExpression lambda, params Type[] genericArgs) { if (source == null) { throw new ArgumentNullException("source"); } return source.Provider.CreateQuery( Expression.Call( typeof(Queryable), queryableMethod, genericArgs, source.Expression, Expression.Quote(lambda))); } public static Expression Or(IEnumerable expressions) { Expression orExpression = null; foreach (Expression e in expressions) { if (e == null) { continue; } if (orExpression == null) { orExpression = e; } else { orExpression = Expression.OrElse(orExpression, e); } } return orExpression; } public static Expression And(IEnumerable expressions) { Expression andExpression = null; foreach (Expression e in expressions) { if (e == null) { continue; } if (andExpression == null) { andExpression = e; } else { andExpression = Expression.AndAlso(andExpression, e); } } return andExpression; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
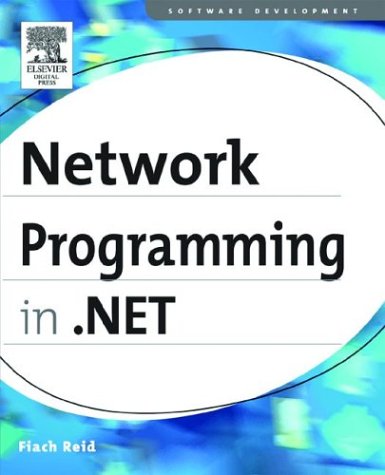
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MD5.cs
- PnrpPermission.cs
- ObfuscateAssemblyAttribute.cs
- DataRelationCollection.cs
- FunctionParameter.cs
- OdbcHandle.cs
- Function.cs
- ipaddressinformationcollection.cs
- IntAverageAggregationOperator.cs
- DefaultTextStoreTextComposition.cs
- TextTreeNode.cs
- MediaEntryAttribute.cs
- SqlSupersetValidator.cs
- EntityDataSourceWizardForm.cs
- CellConstantDomain.cs
- MaskDesignerDialog.cs
- Enum.cs
- UIElementParagraph.cs
- StorageBasedPackageProperties.cs
- X509Certificate2Collection.cs
- ValidationErrorEventArgs.cs
- ProfileEventArgs.cs
- XmlDocumentSurrogate.cs
- TypeRestriction.cs
- PrimaryKeyTypeConverter.cs
- NameValuePermission.cs
- TTSEngineProxy.cs
- SafeHandles.cs
- ContextBase.cs
- ControlDesignerState.cs
- DataSetViewSchema.cs
- AvtEvent.cs
- SystemIPInterfaceStatistics.cs
- SchemaTypeEmitter.cs
- RepeaterItemCollection.cs
- PreProcessor.cs
- DispatcherTimer.cs
- IPEndPointCollection.cs
- HtmlToClrEventProxy.cs
- CellLabel.cs
- MetafileHeader.cs
- AuthorizationPolicyTypeElementCollection.cs
- CodeAccessPermission.cs
- TextEndOfParagraph.cs
- CompleteWizardStep.cs
- OutOfMemoryException.cs
- JsonClassDataContract.cs
- DrawingVisualDrawingContext.cs
- TreePrinter.cs
- DefaultValueConverter.cs
- ResourceCategoryAttribute.cs
- XmlDocumentFragment.cs
- ToolStripSeparator.cs
- X509InitiatorCertificateClientElement.cs
- RangeValidator.cs
- DependencyObjectProvider.cs
- Atom10FormatterFactory.cs
- ParagraphVisual.cs
- Helpers.cs
- PermissionSetEnumerator.cs
- DesignerSerializationManager.cs
- XmlSchemaProviderAttribute.cs
- EpmContentDeSerializer.cs
- WebReferenceOptions.cs
- SoapRpcMethodAttribute.cs
- ColorPalette.cs
- PropertyGroupDescription.cs
- EventArgs.cs
- DocumentViewerAutomationPeer.cs
- ActivityTrace.cs
- TaskHelper.cs
- AudioStateChangedEventArgs.cs
- BindingNavigatorDesigner.cs
- RuntimeConfigLKG.cs
- OleDbPermission.cs
- MatrixKeyFrameCollection.cs
- XmlStreamStore.cs
- WebPartEditorCancelVerb.cs
- StylusPlugInCollection.cs
- MatrixCamera.cs
- HitTestFilterBehavior.cs
- Simplifier.cs
- SQLByteStorage.cs
- CodeFieldReferenceExpression.cs
- EntityDataSourceDataSelection.cs
- DoubleUtil.cs
- safelinkcollection.cs
- EncodingNLS.cs
- DirectoryRedirect.cs
- NetworkInformationException.cs
- Listbox.cs
- Rights.cs
- TableAutomationPeer.cs
- DataGridViewRowPrePaintEventArgs.cs
- TokenBasedSetEnumerator.cs
- ZoneLinkButton.cs
- PackageFilter.cs
- SchemaMapping.cs
- CriticalFinalizerObject.cs
- BinHexEncoder.cs