Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Core / CSharp / MS / Internal / Automation / TableItemProviderWrapper.cs / 1305600 / TableItemProviderWrapper.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table Item pattern provider wrapper for WCP // // History: // 07/21/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; namespace MS.Internal.Automation { // Automation/WCP Wrapper class: Implements that UIAutomation I...Provider // interface, and calls through to a WCP AutomationPeer which implements the corresponding // I...Provider inteface. Marshalls the call from the RPC thread onto the // target AutomationPeer's context. // // Class has two major parts to it: // * Implementation of the I...Provider, which uses Dispatcher.Invoke // to call a private method (lives in second half of the class) via a delegate, // if necessary, packages any params into an object param. Return type of Invoke // must be cast from object to appropriate type. // * private methods - one for each interface entry point - which get called back // on the right context. These call through to the peer that's actually // implenting the I...Provider version of the interface. internal class TableItemProviderWrapper: MarshalByRefObject, ITableItemProvider { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TableItemProviderWrapper( AutomationPeer peer, ITableItemProvider iface ) { _peer = peer; _iface = iface; } #endregion Constructors //------------------------------------------------------ // // Interface ITableItemProvider // //----------------------------------------------------- #region Interface ITableItemProvider public int Row { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRow ), null ); } } public int Column { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumn ), null ); } } public int RowSpan { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowSpan ), null ); } } public int ColumnSpan { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnSpan ), null ); } } public IRawElementProviderSimple ContainingGrid { get { return (IRawElementProviderSimple) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetContainingGrid ), null ); } } public IRawElementProviderSimple [] GetRowHeaderItems() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowHeaderItems ), null ); } public IRawElementProviderSimple [] GetColumnHeaderItems() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnHeaderItems ), null ); } #endregion Interface ITableItemProvider //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static object Wrap( AutomationPeer peer, object iface ) { return new TableItemProviderWrapper( peer, (ITableItemProvider) iface ); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object GetRow( object unused ) { return _iface.Row; } private object GetColumn( object unused ) { return _iface.Column; } private object GetRowSpan( object unused ) { return _iface.RowSpan; } private object GetColumnSpan( object unused ) { return _iface.ColumnSpan; } private object GetContainingGrid( object unused ) { return _iface.ContainingGrid; } private object GetRowHeaderItems( object unused ) { return _iface.GetRowHeaderItems(); } private object GetColumnHeaderItems( object unused ) { return _iface.GetColumnHeaderItems(); } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; private ITableItemProvider _iface; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Table Item pattern provider wrapper for WCP // // History: // 07/21/2003 : BrendanM Ported to WCP // //--------------------------------------------------------------------------- using System; using System.Windows.Threading; using System.Windows.Media; using System.Windows.Automation; using System.Windows.Automation.Provider; using System.Windows.Automation.Peers; namespace MS.Internal.Automation { // Automation/WCP Wrapper class: Implements that UIAutomation I...Provider // interface, and calls through to a WCP AutomationPeer which implements the corresponding // I...Provider inteface. Marshalls the call from the RPC thread onto the // target AutomationPeer's context. // // Class has two major parts to it: // * Implementation of the I...Provider, which uses Dispatcher.Invoke // to call a private method (lives in second half of the class) via a delegate, // if necessary, packages any params into an object param. Return type of Invoke // must be cast from object to appropriate type. // * private methods - one for each interface entry point - which get called back // on the right context. These call through to the peer that's actually // implenting the I...Provider version of the interface. internal class TableItemProviderWrapper: MarshalByRefObject, ITableItemProvider { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private TableItemProviderWrapper( AutomationPeer peer, ITableItemProvider iface ) { _peer = peer; _iface = iface; } #endregion Constructors //------------------------------------------------------ // // Interface ITableItemProvider // //----------------------------------------------------- #region Interface ITableItemProvider public int Row { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRow ), null ); } } public int Column { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumn ), null ); } } public int RowSpan { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowSpan ), null ); } } public int ColumnSpan { get { return (int) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnSpan ), null ); } } public IRawElementProviderSimple ContainingGrid { get { return (IRawElementProviderSimple) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetContainingGrid ), null ); } } public IRawElementProviderSimple [] GetRowHeaderItems() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetRowHeaderItems ), null ); } public IRawElementProviderSimple [] GetColumnHeaderItems() { return (IRawElementProviderSimple []) ElementUtil.Invoke( _peer, new DispatcherOperationCallback( GetColumnHeaderItems ), null ); } #endregion Interface ITableItemProvider //------------------------------------------------------ // // Internal Methods // //------------------------------------------------------ #region Internal Methods internal static object Wrap( AutomationPeer peer, object iface ) { return new TableItemProviderWrapper( peer, (ITableItemProvider) iface ); } #endregion Internal Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object GetRow( object unused ) { return _iface.Row; } private object GetColumn( object unused ) { return _iface.Column; } private object GetRowSpan( object unused ) { return _iface.RowSpan; } private object GetColumnSpan( object unused ) { return _iface.ColumnSpan; } private object GetContainingGrid( object unused ) { return _iface.ContainingGrid; } private object GetRowHeaderItems( object unused ) { return _iface.GetRowHeaderItems(); } private object GetColumnHeaderItems( object unused ) { return _iface.GetColumnHeaderItems(); } #endregion Private Methods //----------------------------------------------------- // // Private Fields // //----------------------------------------------------- #region Private Fields private AutomationPeer _peer; private ITableItemProvider _iface; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
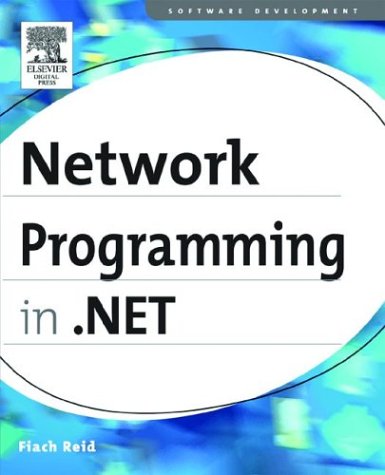
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ColumnMapTranslator.cs
- KnownBoxes.cs
- RootBrowserWindowProxy.cs
- DbConnectionInternal.cs
- StorageInfo.cs
- DBCommand.cs
- StateBag.cs
- XmlObjectSerializerWriteContextComplex.cs
- VisualTreeUtils.cs
- JavaScriptObjectDeserializer.cs
- WsiProfilesElement.cs
- LineSegment.cs
- SecurityPolicySection.cs
- UTF8Encoding.cs
- ErasingStroke.cs
- processwaithandle.cs
- ProviderConnectionPointCollection.cs
- EnlistmentTraceIdentifier.cs
- ActivityScheduledQuery.cs
- ScrollBar.cs
- ElementProxy.cs
- HybridDictionary.cs
- NavigationFailedEventArgs.cs
- FormsAuthenticationUser.cs
- xmlfixedPageInfo.cs
- SettingsProviderCollection.cs
- HeaderPanel.cs
- WebPartDisplayMode.cs
- StringInfo.cs
- Column.cs
- WebPartMenu.cs
- UDPClient.cs
- EditCommandColumn.cs
- Accessible.cs
- OracleConnectionFactory.cs
- GeneratedView.cs
- IteratorFilter.cs
- ConfigXmlWhitespace.cs
- GroupDescription.cs
- MaskedTextBox.cs
- CommandPlan.cs
- TreeWalkHelper.cs
- XmlSchemaParticle.cs
- SafeFreeMibTable.cs
- Point3DConverter.cs
- nulltextcontainer.cs
- XmlSerializerAssemblyAttribute.cs
- HtmlTitle.cs
- TextBox.cs
- ICollection.cs
- WorkflowDefinitionDispenser.cs
- FastEncoderWindow.cs
- StsCommunicationException.cs
- DataControlField.cs
- DataReceivedEventArgs.cs
- TextElementEnumerator.cs
- TextTrailingCharacterEllipsis.cs
- SemaphoreSecurity.cs
- ObjectListFieldsPage.cs
- RadioButton.cs
- PlaceHolder.cs
- DataGridPageChangedEventArgs.cs
- hwndwrapper.cs
- KeySplineConverter.cs
- MenuStrip.cs
- SiteMapNodeItem.cs
- ClientOperation.cs
- WebBrowserDocumentCompletedEventHandler.cs
- SafeMILHandle.cs
- DesigntimeLicenseContext.cs
- HttpRuntime.cs
- WebPartCollection.cs
- DoubleCollectionValueSerializer.cs
- FlowDocumentPage.cs
- TypeBrowser.xaml.cs
- ReferencedType.cs
- ObjectNotFoundException.cs
- RSAOAEPKeyExchangeDeformatter.cs
- ItemChangedEventArgs.cs
- SoapHeaderException.cs
- StylusOverProperty.cs
- ViewCellSlot.cs
- TimeSpanValidator.cs
- HandleCollector.cs
- SHA256.cs
- ValueConversionAttribute.cs
- CompilerError.cs
- NegotiateStream.cs
- EnvironmentPermission.cs
- SimpleTextLine.cs
- COM2PictureConverter.cs
- XamlTreeBuilder.cs
- DrawItemEvent.cs
- ProjectionQueryOptionExpression.cs
- IApplicationTrustManager.cs
- PeerToPeerException.cs
- WebPartDisplayMode.cs
- FillErrorEventArgs.cs
- TreeBuilderBamlTranslator.cs
- StreamGeometry.cs