Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / MS / Internal / Annotations / Serializer.cs / 1305600 / Serializer.cs
//------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Serializer performs the boot-strapping to call the public implementations // of IXmlSerializable for the Annotation object model. This would normally // be done by XmlSerializer but its slow and causes an assembly to be generated // at runtime. API-wise third-parties can still use XmlSerializer but we // choose not to for our purposes. // // History: // 08/26/2004: rruiz: Added new serializer class. // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Reflection; using System.Windows.Annotations.Storage; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; using MS.Internal; namespace MS.Internal.Annotations { ////// Serializer class for Annotation object model. All entities /// in the object model implement IXmlSerializable (or are /// contained and serialized by an entity that does). This class /// does the simple boot-strapping for serializing/deserializing /// of the object model. This lets us get by without paying the /// cost of XmlSerializer (which generates a run-time assembly). /// internal class Serializer { ////// Creates an instance of the serializer for the specified type. /// We use the type to get the default constructor and the type's /// element name and namespace. This constructor expects the /// type to be attributed with XmlRootAttribute (as all serializable /// classes in the object model are). /// /// the type to be serialized by this instance public Serializer(Type type) { Invariant.Assert(type != null); // Find the XmlRootAttribute for the type object[] attributes = type.GetCustomAttributes(false); foreach (object obj in attributes) { _attribute = obj as XmlRootAttribute; if (_attribute != null) break; } Invariant.Assert(_attribute != null, "Internal Serializer used for a type with no XmlRootAttribute."); // Get the default constructor for the type _ctor = type.GetConstructor(new Type[0]); } ////// Serializes the object to the specified XmlWriter. /// /// writer to serialize to /// object to serialize public void Serialize(XmlWriter writer, object obj) { Invariant.Assert(writer != null && obj != null); IXmlSerializable serializable = obj as IXmlSerializable; Invariant.Assert(serializable != null, "Internal Serializer used for a type that isn't IXmlSerializable."); writer.WriteStartElement(_attribute.ElementName, _attribute.Namespace); serializable.WriteXml(writer); writer.WriteEndElement(); } ////// Deserializes the next object from the reader. The /// reader is expected to be positioned on a node that /// can be deserialized into the type this serializer /// was instantiated for. /// /// reader to deserialize from ///an instance of the type this serializer was instanted /// for with values retrieved from the reader public object Deserialize(XmlReader reader) { Invariant.Assert(reader != null); IXmlSerializable serializable = (IXmlSerializable)_ctor.Invoke(new object[0]); // If this is a brand-new stream we need to jump into it if (reader.ReadState == ReadState.Initial) { reader.Read(); } serializable.ReadXml(reader); return serializable; } // XmlRootAttribute - specifies the ElementName and Namespace for // the node to read/write private XmlRootAttribute _attribute; // Constructor used to create instances when deserializing private ConstructorInfo _ctor; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //------------------------------------------------------------------------------ // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // Description: // Serializer performs the boot-strapping to call the public implementations // of IXmlSerializable for the Annotation object model. This would normally // be done by XmlSerializer but its slow and causes an assembly to be generated // at runtime. API-wise third-parties can still use XmlSerializer but we // choose not to for our purposes. // // History: // 08/26/2004: rruiz: Added new serializer class. // //----------------------------------------------------------------------------- using System; using System.Diagnostics; using System.Reflection; using System.Windows.Annotations.Storage; using System.Xml; using System.Xml.Schema; using System.Xml.Serialization; using MS.Internal; namespace MS.Internal.Annotations { ////// Serializer class for Annotation object model. All entities /// in the object model implement IXmlSerializable (or are /// contained and serialized by an entity that does). This class /// does the simple boot-strapping for serializing/deserializing /// of the object model. This lets us get by without paying the /// cost of XmlSerializer (which generates a run-time assembly). /// internal class Serializer { ////// Creates an instance of the serializer for the specified type. /// We use the type to get the default constructor and the type's /// element name and namespace. This constructor expects the /// type to be attributed with XmlRootAttribute (as all serializable /// classes in the object model are). /// /// the type to be serialized by this instance public Serializer(Type type) { Invariant.Assert(type != null); // Find the XmlRootAttribute for the type object[] attributes = type.GetCustomAttributes(false); foreach (object obj in attributes) { _attribute = obj as XmlRootAttribute; if (_attribute != null) break; } Invariant.Assert(_attribute != null, "Internal Serializer used for a type with no XmlRootAttribute."); // Get the default constructor for the type _ctor = type.GetConstructor(new Type[0]); } ////// Serializes the object to the specified XmlWriter. /// /// writer to serialize to /// object to serialize public void Serialize(XmlWriter writer, object obj) { Invariant.Assert(writer != null && obj != null); IXmlSerializable serializable = obj as IXmlSerializable; Invariant.Assert(serializable != null, "Internal Serializer used for a type that isn't IXmlSerializable."); writer.WriteStartElement(_attribute.ElementName, _attribute.Namespace); serializable.WriteXml(writer); writer.WriteEndElement(); } ////// Deserializes the next object from the reader. The /// reader is expected to be positioned on a node that /// can be deserialized into the type this serializer /// was instantiated for. /// /// reader to deserialize from ///an instance of the type this serializer was instanted /// for with values retrieved from the reader public object Deserialize(XmlReader reader) { Invariant.Assert(reader != null); IXmlSerializable serializable = (IXmlSerializable)_ctor.Invoke(new object[0]); // If this is a brand-new stream we need to jump into it if (reader.ReadState == ReadState.Initial) { reader.Read(); } serializable.ReadXml(reader); return serializable; } // XmlRootAttribute - specifies the ElementName and Namespace for // the node to read/write private XmlRootAttribute _attribute; // Constructor used to create instances when deserializing private ConstructorInfo _ctor; } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
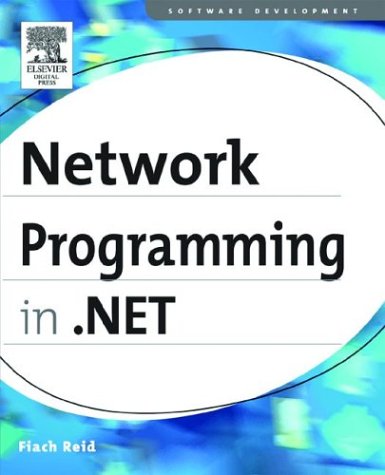
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RectAnimationUsingKeyFrames.cs
- SerializationAttributes.cs
- MetadataResolver.cs
- TransportReplyChannelAcceptor.cs
- IdentityValidationException.cs
- StateMachine.cs
- NavigationProperty.cs
- StickyNote.cs
- Win32SafeHandles.cs
- ScriptingWebServicesSectionGroup.cs
- EUCJPEncoding.cs
- PasswordTextNavigator.cs
- Shape.cs
- ElementUtil.cs
- ExpressionCopier.cs
- ListView.cs
- ByteStack.cs
- DetailsViewInsertEventArgs.cs
- ToolZone.cs
- DataBoundLiteralControl.cs
- XmlIgnoreAttribute.cs
- WebBrowserContainer.cs
- ReferentialConstraint.cs
- SchemaElement.cs
- ArraySet.cs
- TemplateAction.cs
- NamespaceInfo.cs
- HtmlHead.cs
- XamlSerializerUtil.cs
- GridViewRowEventArgs.cs
- LinearGradientBrush.cs
- TextTreeRootTextBlock.cs
- DataGridViewLinkCell.cs
- HMACSHA384.cs
- InkCanvas.cs
- WebReferenceCollection.cs
- AnnotationHelper.cs
- GridView.cs
- MappingSource.cs
- ReferenceEqualityComparer.cs
- RenderDataDrawingContext.cs
- TypeElement.cs
- HttpResponseInternalWrapper.cs
- ValidationHelper.cs
- WebPartMenuStyle.cs
- DataServiceRequest.cs
- EmptyElement.cs
- WindowsFont.cs
- PropertyChangeTracker.cs
- X509ClientCertificateAuthentication.cs
- LocatorGroup.cs
- wgx_commands.cs
- CallTemplateAction.cs
- EncoderExceptionFallback.cs
- dataSvcMapFileLoader.cs
- SplineKeyFrames.cs
- MimeParameters.cs
- PagerSettings.cs
- NamespaceExpr.cs
- WS2007FederationHttpBindingElement.cs
- AnimationStorage.cs
- QilTernary.cs
- HorizontalAlignConverter.cs
- TabRenderer.cs
- BitmapEffectCollection.cs
- brushes.cs
- ReflectTypeDescriptionProvider.cs
- OpenFileDialog.cs
- ExtensionDataObject.cs
- _SingleItemRequestCache.cs
- InternalTransaction.cs
- StateWorkerRequest.cs
- XhtmlBasicValidatorAdapter.cs
- ModuleBuilderData.cs
- DataColumnMappingCollection.cs
- Query.cs
- OdbcStatementHandle.cs
- XmlTextWriter.cs
- _Semaphore.cs
- WorkItem.cs
- SoapUnknownHeader.cs
- DbProviderSpecificTypePropertyAttribute.cs
- SecurityRuntime.cs
- RangeValueProviderWrapper.cs
- OdbcParameterCollection.cs
- XmlName.cs
- Condition.cs
- Subtree.cs
- ControlCodeDomSerializer.cs
- WinHttpWebProxyFinder.cs
- XmlObjectSerializerWriteContextComplexJson.cs
- VerificationAttribute.cs
- ElementHost.cs
- UserControl.cs
- COM2ExtendedUITypeEditor.cs
- FontClient.cs
- MemberAccessException.cs
- StylesEditorDialog.cs
- SQLMoney.cs
- SrgsGrammarCompiler.cs