Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / Framework / System / Windows / Markup / Baml2006 / WpfMemberInvoker.cs / 1305600 / WpfMemberInvoker.cs
using System; using System.Collections.Generic; using System.Text; using System.Xaml.Schema; using System.Reflection; namespace System.Windows.Baml2006 { internal class WpfMemberInvoker : XamlMemberInvoker { WpfXamlMember _member; bool _hasShouldSerializeMethodBeenLookedup = false; MethodInfo _shouldSerializeMethod = null; public WpfMemberInvoker(WpfXamlMember member) : base(member) { _member = member; } public override void SetValue(object instance, object value) { DependencyObject dObject = instance as DependencyObject; if (dObject != null) { if (_member.DependencyProperty != null) { dObject.SetValue(_member.DependencyProperty, value); return; } else if (_member.RoutedEvent != null) { Delegate handler = value as Delegate; if (handler != null) { UIElement.AddHandler(dObject, _member.RoutedEvent, handler); return; } } } base.SetValue(instance, value); } public override object GetValue(object instance) { DependencyObject dObject = instance as DependencyObject; if (dObject != null && _member.DependencyProperty != null) { object result = dObject.GetValue(_member.DependencyProperty); if (result != null) { return result; } // Getter fallback: see comment on WpfXamlMember.AsContentProperty if (!_member.ApplyGetterFallback || _member.UnderlyingMember == null) { return result; } } return base.GetValue(instance); } public override ShouldSerializeResult ShouldSerializeValue(object instance) { // Look up the ShouldSerializeMethod if (!_hasShouldSerializeMethodBeenLookedup) { Type declaringType = _member.UnderlyingMember.DeclaringType; string methodName = "ShouldSerialize" + _member.Name; BindingFlags flags = BindingFlags.NonPublic | BindingFlags.Public | BindingFlags.Static; Type[] args = new Type[] { typeof(DependencyObject) }; ; if (_member.IsAttachable) { _shouldSerializeMethod = declaringType.GetMethod(methodName, flags, null, args, null); } else { flags |= BindingFlags.Instance; _shouldSerializeMethod = declaringType.GetMethod(methodName, flags, null, args, null); } _hasShouldSerializeMethodBeenLookedup = true; } // Invoke the method if we found one if (_shouldSerializeMethod != null) { bool result; var args = new object[] { instance as DependencyObject }; if (_member.IsAttachable) { result = (bool)_shouldSerializeMethod.Invoke(null, args); } else { result = (bool)_shouldSerializeMethod.Invoke(instance, args); } return result ? ShouldSerializeResult.True : ShouldSerializeResult.False; } DependencyObject dObject = instance as DependencyObject; if (dObject != null && _member.DependencyProperty != null) { // Call DO's ShouldSerializeProperty to see if the property is set. // If the property is unset, the property should not be serialized bool isPropertySet = dObject.ShouldSerializeProperty(_member.DependencyProperty); if (!isPropertySet) { return ShouldSerializeResult.False; } } return base.ShouldSerializeValue(instance); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. using System; using System.Collections.Generic; using System.Text; using System.Xaml.Schema; using System.Reflection; namespace System.Windows.Baml2006 { internal class WpfMemberInvoker : XamlMemberInvoker { WpfXamlMember _member; bool _hasShouldSerializeMethodBeenLookedup = false; MethodInfo _shouldSerializeMethod = null; public WpfMemberInvoker(WpfXamlMember member) : base(member) { _member = member; } public override void SetValue(object instance, object value) { DependencyObject dObject = instance as DependencyObject; if (dObject != null) { if (_member.DependencyProperty != null) { dObject.SetValue(_member.DependencyProperty, value); return; } else if (_member.RoutedEvent != null) { Delegate handler = value as Delegate; if (handler != null) { UIElement.AddHandler(dObject, _member.RoutedEvent, handler); return; } } } base.SetValue(instance, value); } public override object GetValue(object instance) { DependencyObject dObject = instance as DependencyObject; if (dObject != null && _member.DependencyProperty != null) { object result = dObject.GetValue(_member.DependencyProperty); if (result != null) { return result; } // Getter fallback: see comment on WpfXamlMember.AsContentProperty if (!_member.ApplyGetterFallback || _member.UnderlyingMember == null) { return result; } } return base.GetValue(instance); } public override ShouldSerializeResult ShouldSerializeValue(object instance) { // Look up the ShouldSerializeMethod if (!_hasShouldSerializeMethodBeenLookedup) { Type declaringType = _member.UnderlyingMember.DeclaringType; string methodName = "ShouldSerialize" + _member.Name; BindingFlags flags = BindingFlags.NonPublic | BindingFlags.Public | BindingFlags.Static; Type[] args = new Type[] { typeof(DependencyObject) }; ; if (_member.IsAttachable) { _shouldSerializeMethod = declaringType.GetMethod(methodName, flags, null, args, null); } else { flags |= BindingFlags.Instance; _shouldSerializeMethod = declaringType.GetMethod(methodName, flags, null, args, null); } _hasShouldSerializeMethodBeenLookedup = true; } // Invoke the method if we found one if (_shouldSerializeMethod != null) { bool result; var args = new object[] { instance as DependencyObject }; if (_member.IsAttachable) { result = (bool)_shouldSerializeMethod.Invoke(null, args); } else { result = (bool)_shouldSerializeMethod.Invoke(instance, args); } return result ? ShouldSerializeResult.True : ShouldSerializeResult.False; } DependencyObject dObject = instance as DependencyObject; if (dObject != null && _member.DependencyProperty != null) { // Call DO's ShouldSerializeProperty to see if the property is set. // If the property is unset, the property should not be serialized bool isPropertySet = dObject.ShouldSerializeProperty(_member.DependencyProperty); if (!isPropertySet) { return ShouldSerializeResult.False; } } return base.ShouldSerializeValue(instance); } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
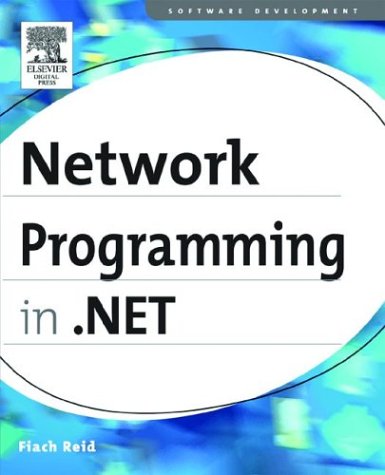
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- Vector3DCollection.cs
- DebugView.cs
- TableLayoutSettingsTypeConverter.cs
- SmiContext.cs
- IconConverter.cs
- ReliableSessionBindingElement.cs
- EditorZoneBase.cs
- _LocalDataStoreMgr.cs
- SqlCommandBuilder.cs
- MissingManifestResourceException.cs
- WsatConfiguration.cs
- _BasicClient.cs
- StreamSecurityUpgradeInitiatorAsyncResult.cs
- DesignerVerb.cs
- EventHandlerService.cs
- NavigationProperty.cs
- DispatcherSynchronizationContext.cs
- SoapSchemaMember.cs
- DataGridColumnCollection.cs
- DateTimeValueSerializerContext.cs
- DoubleAnimationUsingKeyFrames.cs
- CommandID.cs
- ArrangedElementCollection.cs
- ContextProperty.cs
- WebBrowserUriTypeConverter.cs
- BaseTemplatedMobileComponentEditor.cs
- Matrix.cs
- DesignerDataStoredProcedure.cs
- QuadraticBezierSegment.cs
- GreaterThan.cs
- WebBrowsableAttribute.cs
- CaseStatement.cs
- RemoteWebConfigurationHostServer.cs
- HttpCachePolicyElement.cs
- SystemDropShadowChrome.cs
- TraceXPathNavigator.cs
- ChannelAcceptor.cs
- RolePrincipal.cs
- PointAnimation.cs
- WindowHideOrCloseTracker.cs
- TiffBitmapEncoder.cs
- Msec.cs
- CalendarData.cs
- PolyLineSegment.cs
- SafeNativeMethods.cs
- ListViewCancelEventArgs.cs
- DetectEofStream.cs
- SafeNativeMethods.cs
- ResetableIterator.cs
- PageFunction.cs
- Point4D.cs
- ComponentDispatcherThread.cs
- DocumentGrid.cs
- ListViewHitTestInfo.cs
- ProtectedConfigurationSection.cs
- ShaderEffect.cs
- DSACryptoServiceProvider.cs
- NumberFunctions.cs
- ReferencedAssembly.cs
- WebRequestModuleElement.cs
- CompilationLock.cs
- SemaphoreFullException.cs
- DynamicRendererThreadManager.cs
- X509UI.cs
- CSharpCodeProvider.cs
- DelegatingConfigHost.cs
- SqlUserDefinedTypeAttribute.cs
- ArraySortHelper.cs
- SourceElementsCollection.cs
- DataSourceView.cs
- XPSSignatureDefinition.cs
- Calendar.cs
- SourceSwitch.cs
- StateMachineWorkflowInstance.cs
- DivideByZeroException.cs
- PointAnimationUsingKeyFrames.cs
- CanonicalFontFamilyReference.cs
- FormsAuthenticationModule.cs
- EntityContainerAssociationSet.cs
- SubtreeProcessor.cs
- ReadOnlyMetadataCollection.cs
- ComponentManagerBroker.cs
- FrameworkElementFactory.cs
- FileLogRecordHeader.cs
- XmlNodeChangedEventManager.cs
- Gdiplus.cs
- TextSchema.cs
- CaseCqlBlock.cs
- PropertyDescriptorCollection.cs
- DbInsertCommandTree.cs
- FamilyCollection.cs
- Dump.cs
- ALinqExpressionVisitor.cs
- sitestring.cs
- Path.cs
- Activity.cs
- EntryIndex.cs
- RouteItem.cs
- ConfigurationSection.cs
- ResourceKey.cs