Code:
/ 4.0 / 4.0 / DEVDIV_TFS / Dev10 / Releases / RTMRel / wpf / src / UIAutomation / UIAutomationClient / System / Windows / Automation / ItemContainerPattern.cs / 1305600 / ItemContainerPattern.cs
//---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for ItemContainer Pattern // // History: // 10/22/2008 : [....] - created // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; using System.Runtime.InteropServices; using System.Globalization; namespace System.Windows.Automation { ////// Represents Containers that maintains items and support item look up by propety value. /// #if (INTERNAL_COMPILE) internal class ItemContainerPattern: BasePattern #else public class ItemContainerPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private ItemContainerPattern(AutomationElement el, SafePatternHandle hPattern) : base(el, hPattern) { _hPattern = hPattern; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///ItemContainer pattern public static readonly AutomationPattern Pattern = ItemContainerPatternIdentifiers.Pattern; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Find item by specified property/value. It will return /// placeholder which depending upon it's virtualization state may /// or may not have the information of the complete peer/Wrapper. /// /// Throws ArgumentException if the property requested is not one that the /// container supports searching over. Supports Name property, AutomationId, /// IsSelected and ControlType. /// /// This method is expected to be relatively slow, since it may need to /// traverse multiple objects in order to find a matching one. /// When used in a loop to return multiple items, no specific order is /// defined so long as each item is returned only once (ie. loop should /// terminate). This method is also item-centric, not UI-centric, so items /// with multiple UI representations need only be returned once. /// /// A special propertyId of 0 means ‘match all items’. This can be used /// with startAfter=NULL to get the first item, and then to get successive /// items. /// /// this represents the item after which the container wants to begin search /// corresponds to property for whose value it want to search over. /// value to be searched for, for specified property ///The first item which matches the searched criterion, if no item matches, it returns null public AutomationElement FindItemByProperty(AutomationElement startAfter, AutomationProperty property, object value) { SafeNodeHandle hNode; // Invalidate the "value" passed against the "property" before passing it to UIACore, Don't invalidate if search is being done for "null" property // FindItemByProperty supports find for null property. if (property != null) { value = PropertyValueValidateAndMap(property, value); } if (startAfter != null) { if (property != null) hNode = UiaCoreApi.ItemContainerPattern_FindItemByProperty(_hPattern, startAfter.RawNode, property.Id, value); else hNode = UiaCoreApi.ItemContainerPattern_FindItemByProperty(_hPattern, startAfter.RawNode, 0, null); } else { if (property != null) hNode = UiaCoreApi.ItemContainerPattern_FindItemByProperty(_hPattern, new SafeNodeHandle(), property.Id, value); else hNode = UiaCoreApi.ItemContainerPattern_FindItemByProperty(_hPattern, new SafeNodeHandle(), 0, null); } AutomationElement wrappedElement = AutomationElement.Wrap(hNode); return wrappedElement; } #endregion Public Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object PropertyValueValidateAndMap(AutomationProperty property, object value) { AutomationPropertyInfo info; if (!Schema.GetPropertyInfo(property, out info)) { throw new ArgumentException(SR.Get(SRID.UnsupportedProperty)); } // Check type is appropriate: NotSupported is allowed against any property, // null is allowed for any reference type (ie not for value types), otherwise // type must be assignable from expected type. Type expectedType = info.Type; if (value != AutomationElement.NotSupported && ((value == null && expectedType.IsValueType) || (value != null && !expectedType.IsAssignableFrom(value.GetType())))) { throw new ArgumentException(SR.Get(SRID.PropertyConditionIncorrectType, property.ProgrammaticName, expectedType.Name)); } // Some types are handled differently in managed vs unmanaged - handle those here... if (value is AutomationElement) { // If this is a comparison against a Raw/LogicalElement, // save the runtime ID instead of the element so that we // can take it cross-proc if needed. value = ((AutomationElement)value).GetRuntimeId(); } else if (value is ControlType) { // If this is a control type, use the ID, not the CLR object value = ((ControlType)value).Id; } else if (value is Rect) { Rect rc = (Rect)value; value = new double[] { rc.Left, rc.Top, rc.Width, rc.Height }; } else if (value is Point) { Point pt = (Point)value; value = new double[] { pt.X, pt.Y }; } else if (value is CultureInfo) { value = ((CultureInfo)value).LCID; } return value; } #endregion Internal Methods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods static internal object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new ItemContainerPattern(el, hPattern); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved. //---------------------------------------------------------------------------- // //// Copyright (C) Microsoft Corporation. All rights reserved. // // // // Description: Client-side wrapper for ItemContainer Pattern // // History: // 10/22/2008 : [....] - created // //--------------------------------------------------------------------------- using System; using System.Windows.Automation.Provider; using MS.Internal.Automation; using System.Runtime.InteropServices; using System.Globalization; namespace System.Windows.Automation { ////// Represents Containers that maintains items and support item look up by propety value. /// #if (INTERNAL_COMPILE) internal class ItemContainerPattern: BasePattern #else public class ItemContainerPattern: BasePattern #endif { //----------------------------------------------------- // // Constructors // //----------------------------------------------------- #region Constructors private ItemContainerPattern(AutomationElement el, SafePatternHandle hPattern) : base(el, hPattern) { _hPattern = hPattern; } #endregion Constructors //------------------------------------------------------ // // Public Constants / Readonly Fields // //----------------------------------------------------- #region Public Constants and Readonly Fields ///ItemContainer pattern public static readonly AutomationPattern Pattern = ItemContainerPatternIdentifiers.Pattern; #endregion Public Constants and Readonly Fields //------------------------------------------------------ // // Public Methods // //------------------------------------------------------ #region Public Methods ////// Find item by specified property/value. It will return /// placeholder which depending upon it's virtualization state may /// or may not have the information of the complete peer/Wrapper. /// /// Throws ArgumentException if the property requested is not one that the /// container supports searching over. Supports Name property, AutomationId, /// IsSelected and ControlType. /// /// This method is expected to be relatively slow, since it may need to /// traverse multiple objects in order to find a matching one. /// When used in a loop to return multiple items, no specific order is /// defined so long as each item is returned only once (ie. loop should /// terminate). This method is also item-centric, not UI-centric, so items /// with multiple UI representations need only be returned once. /// /// A special propertyId of 0 means ‘match all items’. This can be used /// with startAfter=NULL to get the first item, and then to get successive /// items. /// /// this represents the item after which the container wants to begin search /// corresponds to property for whose value it want to search over. /// value to be searched for, for specified property ///The first item which matches the searched criterion, if no item matches, it returns null public AutomationElement FindItemByProperty(AutomationElement startAfter, AutomationProperty property, object value) { SafeNodeHandle hNode; // Invalidate the "value" passed against the "property" before passing it to UIACore, Don't invalidate if search is being done for "null" property // FindItemByProperty supports find for null property. if (property != null) { value = PropertyValueValidateAndMap(property, value); } if (startAfter != null) { if (property != null) hNode = UiaCoreApi.ItemContainerPattern_FindItemByProperty(_hPattern, startAfter.RawNode, property.Id, value); else hNode = UiaCoreApi.ItemContainerPattern_FindItemByProperty(_hPattern, startAfter.RawNode, 0, null); } else { if (property != null) hNode = UiaCoreApi.ItemContainerPattern_FindItemByProperty(_hPattern, new SafeNodeHandle(), property.Id, value); else hNode = UiaCoreApi.ItemContainerPattern_FindItemByProperty(_hPattern, new SafeNodeHandle(), 0, null); } AutomationElement wrappedElement = AutomationElement.Wrap(hNode); return wrappedElement; } #endregion Public Methods //----------------------------------------------------- // // Private Methods // //------------------------------------------------------ #region Private Methods private object PropertyValueValidateAndMap(AutomationProperty property, object value) { AutomationPropertyInfo info; if (!Schema.GetPropertyInfo(property, out info)) { throw new ArgumentException(SR.Get(SRID.UnsupportedProperty)); } // Check type is appropriate: NotSupported is allowed against any property, // null is allowed for any reference type (ie not for value types), otherwise // type must be assignable from expected type. Type expectedType = info.Type; if (value != AutomationElement.NotSupported && ((value == null && expectedType.IsValueType) || (value != null && !expectedType.IsAssignableFrom(value.GetType())))) { throw new ArgumentException(SR.Get(SRID.PropertyConditionIncorrectType, property.ProgrammaticName, expectedType.Name)); } // Some types are handled differently in managed vs unmanaged - handle those here... if (value is AutomationElement) { // If this is a comparison against a Raw/LogicalElement, // save the runtime ID instead of the element so that we // can take it cross-proc if needed. value = ((AutomationElement)value).GetRuntimeId(); } else if (value is ControlType) { // If this is a control type, use the ID, not the CLR object value = ((ControlType)value).Id; } else if (value is Rect) { Rect rc = (Rect)value; value = new double[] { rc.Left, rc.Top, rc.Width, rc.Height }; } else if (value is Point) { Point pt = (Point)value; value = new double[] { pt.X, pt.Y }; } else if (value is CultureInfo) { value = ((CultureInfo)value).LCID; } return value; } #endregion Internal Methods //----------------------------------------------------- // // Internal Methods // //----------------------------------------------------- #region Internal Methods static internal object Wrap(AutomationElement el, SafePatternHandle hPattern, bool cached) { return new ItemContainerPattern(el, hPattern); } #endregion Internal Methods //----------------------------------------------------- // // Private Fields // //------------------------------------------------------ #region Private Fields private SafePatternHandle _hPattern; #endregion Private Fields } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
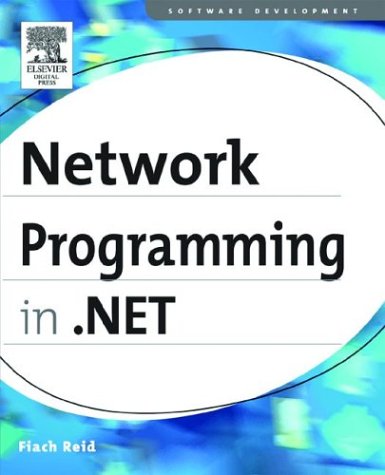
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- ContainerUtilities.cs
- OpenFileDialog.cs
- JournalEntry.cs
- SpecialFolderEnumConverter.cs
- GridPatternIdentifiers.cs
- RecommendedAsConfigurableAttribute.cs
- XmlSchemaType.cs
- DataGridViewCellValidatingEventArgs.cs
- LightweightCodeGenerator.cs
- PhonemeConverter.cs
- LogicalTreeHelper.cs
- ParallelTimeline.cs
- OutputBuffer.cs
- SharedStatics.cs
- EntityContainer.cs
- EdmFunction.cs
- ProcessThread.cs
- OleDbMetaDataFactory.cs
- FamilyTypefaceCollection.cs
- MarkupObject.cs
- ShaderRenderModeValidation.cs
- BigInt.cs
- DataContext.cs
- DataRow.cs
- IndexExpression.cs
- DispatcherHooks.cs
- PageTheme.cs
- Rectangle.cs
- LogReservationCollection.cs
- XmlAnyElementAttributes.cs
- MimeBasePart.cs
- _SpnDictionary.cs
- XamlBrushSerializer.cs
- TypeInfo.cs
- DocumentDesigner.cs
- MediaElement.cs
- ServerReliableChannelBinder.cs
- WebPartVerbCollection.cs
- LogLogRecord.cs
- MetricEntry.cs
- ManagementOptions.cs
- __ComObject.cs
- CodePageUtils.cs
- VectorKeyFrameCollection.cs
- FreeIndexList.cs
- WeakReferenceList.cs
- ParameterModifier.cs
- SharedPerformanceCounter.cs
- CharEnumerator.cs
- SpellerError.cs
- TableCellAutomationPeer.cs
- _FixedSizeReader.cs
- ContentIterators.cs
- OAVariantLib.cs
- CachedTypeface.cs
- Encoder.cs
- _SslStream.cs
- RelationshipEndCollection.cs
- ActivityBindForm.cs
- VisualTreeUtils.cs
- XmlDesignerDataSourceView.cs
- ObjectListField.cs
- FileSystemWatcher.cs
- SerializerProvider.cs
- Exception.cs
- RunInstallerAttribute.cs
- PackagePartCollection.cs
- Vector3DConverter.cs
- ModifierKeysConverter.cs
- EventMappingSettingsCollection.cs
- SupportsEventValidationAttribute.cs
- DecimalStorage.cs
- XmlUTF8TextReader.cs
- DispatcherExceptionFilterEventArgs.cs
- ConnectionPoint.cs
- TextRunCacheImp.cs
- WebProxyScriptElement.cs
- XPathQilFactory.cs
- CompilerErrorCollection.cs
- ExternalCalls.cs
- SectionInput.cs
- DynamicHyperLink.cs
- ReversePositionQuery.cs
- SettingsSavedEventArgs.cs
- UnsafeNativeMethods.cs
- Stylesheet.cs
- DeviceFilterEditorDialog.cs
- DrawingState.cs
- SmtpDateTime.cs
- ServiceMetadataBehavior.cs
- TextElementCollectionHelper.cs
- AnonymousIdentificationModule.cs
- HttpListenerContext.cs
- StoreConnection.cs
- DataBinding.cs
- ConfigLoader.cs
- CalloutQueueItem.cs
- SHA512Cng.cs
- SqlBuilder.cs
- Debugger.cs