Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx35 / System.WorkflowServices / System / ServiceModel / Persistence / PersistenceProvider.cs / 1305376 / PersistenceProvider.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.ServiceModel.Persistence { using System; using System.ServiceModel.Channels; public abstract class PersistenceProvider : CommunicationObject { internal static readonly TimeSpan DefaultOpenClosePersistenceTimout = TimeSpan.FromSeconds(15); Guid id; protected PersistenceProvider(Guid id) { this.id = id; } public Guid Id { get { return this.id; } } public abstract IAsyncResult BeginCreate(object instance, TimeSpan timeout, AsyncCallback callback, object state); public abstract IAsyncResult BeginDelete(object instance, TimeSpan timeout, AsyncCallback callback, object state); public abstract IAsyncResult BeginLoad(TimeSpan timeout, AsyncCallback callback, object state); public virtual IAsyncResult BeginLoadIfChanged(TimeSpan timeout, object instanceToken, AsyncCallback callback, object state) { return this.BeginLoad(timeout, callback, state); } public abstract IAsyncResult BeginUpdate(object instance, TimeSpan timeout, AsyncCallback callback, object state); public abstract object Create(object instance, TimeSpan timeout); public abstract void Delete(object instance, TimeSpan timeout); public abstract object EndCreate(IAsyncResult result); public abstract void EndDelete(IAsyncResult result); public abstract object EndLoad(IAsyncResult result); [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1021")] public virtual bool EndLoadIfChanged(IAsyncResult result, out object instance) { instance = this.EndLoad(result); return true; } public abstract object EndUpdate(IAsyncResult result); public abstract object Load(TimeSpan timeout); [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Design", "CA1021")] public virtual bool LoadIfChanged(TimeSpan timeout, object instanceToken, out object instance) { instance = this.Load(timeout); return true; } public abstract object Update(object instance, TimeSpan timeout); } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
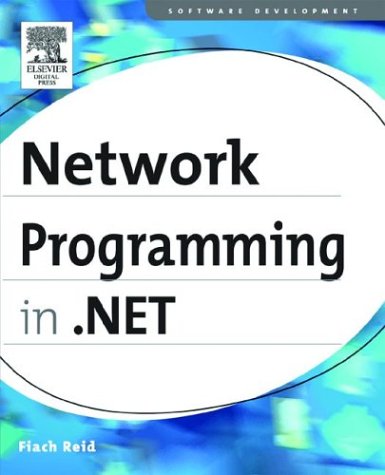
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataGridViewColumn.cs
- MulticastDelegate.cs
- Atom10FormatterFactory.cs
- Processor.cs
- PageCatalogPart.cs
- Timer.cs
- ToolStripDropTargetManager.cs
- SubMenuStyleCollection.cs
- RelationshipEntry.cs
- Icon.cs
- Scene3D.cs
- TextDecorationCollectionConverter.cs
- CharacterMetrics.cs
- WorkflowMessageEventHandler.cs
- DataGridViewBindingCompleteEventArgs.cs
- ErrorStyle.cs
- SettingsBindableAttribute.cs
- PeerObject.cs
- Control.cs
- RelationshipWrapper.cs
- SpeechUI.cs
- AssemblyNameProxy.cs
- EllipseGeometry.cs
- HostSecurityManager.cs
- RemoteCryptoSignHashRequest.cs
- DiagnosticsConfiguration.cs
- CopyNamespacesAction.cs
- DataKeyArray.cs
- AssertSection.cs
- WindowsToolbarItemAsMenuItem.cs
- GridViewColumn.cs
- Debugger.cs
- MetadataItemCollectionFactory.cs
- HwndHostAutomationPeer.cs
- ObjectListItemCollection.cs
- ScriptingSectionGroup.cs
- GenericTextProperties.cs
- Pkcs9Attribute.cs
- BamlLocalizabilityResolver.cs
- validationstate.cs
- StatusBarAutomationPeer.cs
- PenThread.cs
- MeasureData.cs
- SystemEvents.cs
- SafeRightsManagementQueryHandle.cs
- ChineseLunisolarCalendar.cs
- DBCommandBuilder.cs
- LongValidatorAttribute.cs
- FileNotFoundException.cs
- X509Utils.cs
- HttpDictionary.cs
- MeasurementDCInfo.cs
- ContentControl.cs
- SecurityUtils.cs
- UTF8Encoding.cs
- AttributeEmitter.cs
- LogEntrySerializationException.cs
- EarlyBoundInfo.cs
- XmlNodeChangedEventArgs.cs
- X509Utils.cs
- SiteMap.cs
- MultipleViewPattern.cs
- DataRowChangeEvent.cs
- TraversalRequest.cs
- EnterpriseServicesHelper.cs
- nulltextnavigator.cs
- ProcessManager.cs
- SafeNativeMethods.cs
- ConfigXmlAttribute.cs
- PhoneCallDesigner.cs
- AssemblyAttributesGoHere.cs
- XNodeNavigator.cs
- FontUnitConverter.cs
- ClipboardData.cs
- XPathNodeIterator.cs
- StandardCommands.cs
- DataTableTypeConverter.cs
- QueryConverter.cs
- CollectionViewSource.cs
- CompModSwitches.cs
- LocatorPart.cs
- EmptyElement.cs
- ManagedIStream.cs
- TextParagraphCache.cs
- FindCompletedEventArgs.cs
- SqlDelegatedTransaction.cs
- XmlILAnnotation.cs
- Helper.cs
- Floater.cs
- JsonFormatWriterGenerator.cs
- XhtmlBasicLabelAdapter.cs
- StrongTypingException.cs
- TypeUsageBuilder.cs
- DocumentAutomationPeer.cs
- UrlAuthFailedErrorFormatter.cs
- MobileContainerDesigner.cs
- CodeComment.cs
- ChannelSinkStacks.cs
- Transform.cs
- VariableExpressionConverter.cs