Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Bookmark.cs / 1305376 / Bookmark.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities { using System; using System.Activities.Hosting; using System.Activities.Runtime; using System.Collections.Generic; using System.Runtime; using System.Runtime.Serialization; using System.Globalization; [DataContract] [Fx.Tag.XamlVisible(false)] public class Bookmark : IEquatable{ static Bookmark asyncOperationCompletionBookmark = new Bookmark(-1); static IEqualityComparer comparer; //Used only when exclusive scopes are involved [DataMember(EmitDefaultValue = false, Order = 2)] ExclusiveHandleList exclusiveHandlesThatReferenceThis; [DataMember(EmitDefaultValue = false, Order = 0)] long id; [DataMember(EmitDefaultValue = false, Order = 1)] string externalName; Bookmark(long id) { Fx.Assert(id != 0, "id should not be zero"); this.id = id; } public Bookmark(string name) { if (string.IsNullOrEmpty(name)) { throw FxTrace.Exception.ArgumentNullOrEmpty("name"); } this.externalName = name; } internal static Bookmark AsyncOperationCompletionBookmark { get { return asyncOperationCompletionBookmark; } } internal static IEqualityComparer Comparer { get { if (comparer == null) { comparer = new BookmarkComparer(); } return comparer; } } [DataMember(EmitDefaultValue = false)] internal BookmarkScope Scope { get; set; } internal bool IsNamed { get { return this.id == 0; } } public string Name { get { if (this.IsNamed) { return this.externalName; } else { return string.Empty; } } } internal long Id { get { Fx.Assert(!this.IsNamed, "We should only get the id for unnamed bookmarks."); return this.id; } } internal ExclusiveHandleList ExclusiveHandles { get { return this.exclusiveHandlesThatReferenceThis; } set { this.exclusiveHandlesThatReferenceThis = value; } } internal static Bookmark Create(long id) { return new Bookmark(id); } internal BookmarkInfo GenerateBookmarkInfo(BookmarkCallbackWrapper bookmarkCallback) { Fx.Assert(this.IsNamed, "Can only generate BookmarkInfo for external bookmarks"); BookmarkScopeInfo scopeInfo = null; if (this.Scope != null) { scopeInfo = this.Scope.GenerateScopeInfo(); } return new BookmarkInfo(this.externalName, bookmarkCallback.ActivityInstance.Activity.DisplayName, scopeInfo); } public bool Equals(Bookmark other) { if (object.ReferenceEquals(other, null)) { return false; } if (this.IsNamed) { return other.IsNamed && this.externalName == other.externalName; } else { return this.id == other.id; } } public override bool Equals(object obj) { return this.Equals(obj as Bookmark); } public override int GetHashCode() { if (this.IsNamed) { return this.externalName.GetHashCode(); } else { return this.id.GetHashCode(); } } public override string ToString() { if (this.IsNamed) { return this.Name; } else { return this.Id.ToString(CultureInfo.InvariantCulture); } } [DataContract] class BookmarkComparer : IEqualityComparer { public BookmarkComparer() { } public bool Equals(Bookmark x, Bookmark y) { if (object.ReferenceEquals(x, null)) { return object.ReferenceEquals(y, null); } return x.Equals(y); } public int GetHashCode(Bookmark obj) { return obj.GetHashCode(); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
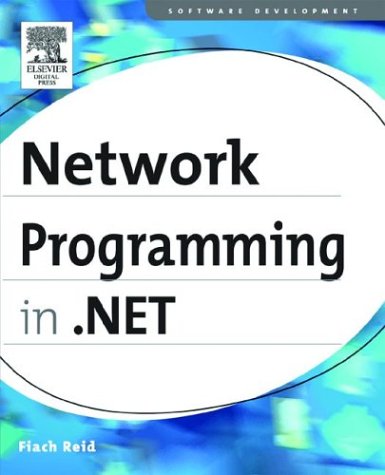
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StyleModeStack.cs
- RepeaterItemCollection.cs
- UserControlCodeDomTreeGenerator.cs
- OleDbConnection.cs
- Rotation3D.cs
- BasePropertyDescriptor.cs
- LocatorBase.cs
- XPathScanner.cs
- XmlSchemaAny.cs
- ChannelManager.cs
- SpellerError.cs
- StylusPointPropertyInfo.cs
- GridViewSelectEventArgs.cs
- RunClient.cs
- SoapServerMessage.cs
- DataGridViewRowCancelEventArgs.cs
- SpellerInterop.cs
- Buffer.cs
- ChangeDirector.cs
- FormsAuthenticationModule.cs
- DataTableMappingCollection.cs
- EntityConnectionStringBuilderItem.cs
- AutomationFocusChangedEventArgs.cs
- GradientStop.cs
- EndpointConfigContainer.cs
- httpapplicationstate.cs
- SerializationInfoEnumerator.cs
- StackBuilderSink.cs
- RoleBoolean.cs
- SEHException.cs
- DesignerAttribute.cs
- WebControlsSection.cs
- InvokeHandlers.cs
- EntryPointNotFoundException.cs
- ModuleBuilder.cs
- ProtocolsConfigurationHandler.cs
- ParamArrayAttribute.cs
- MenuBindingsEditor.cs
- Binding.cs
- Sql8ConformanceChecker.cs
- PageThemeParser.cs
- XhtmlTextWriter.cs
- ComplexTypeEmitter.cs
- UserControl.cs
- KeyboardDevice.cs
- VScrollProperties.cs
- WindowsListView.cs
- TdsParserStateObject.cs
- WebCategoryAttribute.cs
- AnimationClockResource.cs
- CanExecuteRoutedEventArgs.cs
- Merger.cs
- WebPartDescriptionCollection.cs
- WebBrowserBase.cs
- TextRunTypographyProperties.cs
- XPathSingletonIterator.cs
- ComAdminInterfaces.cs
- BuildManagerHost.cs
- Point4DConverter.cs
- ListItemCollection.cs
- ObservableDictionary.cs
- WizardPanelChangingEventArgs.cs
- TextBreakpoint.cs
- TaskDesigner.cs
- Point.cs
- DocumentGridPage.cs
- ListViewItem.cs
- PrintDialog.cs
- MenuEventArgs.cs
- InputLangChangeRequestEvent.cs
- AnonymousIdentificationModule.cs
- SmiGettersStream.cs
- TextDecorationLocationValidation.cs
- ImageInfo.cs
- ProjectionPlan.cs
- DesignerSerializationManager.cs
- XmlSchemaInfo.cs
- Point3DConverter.cs
- XmlTextReader.cs
- CacheSection.cs
- DataGrid.cs
- followingsibling.cs
- RTLAwareMessageBox.cs
- CFStream.cs
- XhtmlBasicControlAdapter.cs
- PassportAuthenticationEventArgs.cs
- PerfCounters.cs
- SmiSettersStream.cs
- ProxySimple.cs
- OdbcConnectionStringbuilder.cs
- EpmTargetTree.cs
- XmlArrayItemAttribute.cs
- LazyInitializer.cs
- BindingContext.cs
- RelationshipFixer.cs
- SelectionWordBreaker.cs
- HttpPostedFile.cs
- SortedList.cs
- Polygon.cs
- CompilerWrapper.cs