Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / Expressions / IndexerReference.cs / 1305376 / IndexerReference.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities.Expressions { using System.Collections.ObjectModel; using System.ComponentModel; using System.Reflection; using System.Runtime; using System.Runtime.Collections; using System.Runtime.Serialization; using System.Windows.Markup; [ContentProperty("Indices")] public sealed class IndexerReference: CodeActivity > { Collection indices; MethodInfo getMethod; MethodInfo setMethod; [RequiredArgument] [DefaultValue(null)] public InArgument Operand { get; set; } [RequiredArgument] [DefaultValue(null)] public Collection Indices { get { if (this.indices == null) { this.indices = new ValidatingCollection { // disallow null values OnAddValidationCallback = item => { if (item == null) { throw FxTrace.Exception.ArgumentNull("item"); } }, }; } return this.indices; } } protected override void CacheMetadata(CodeActivityMetadata metadata) { if (typeof(TOperand).IsValueType) { metadata.AddValidationError(SR.TargetTypeIsValueType(this.GetType().Name, this.DisplayName)); } if (this.Indices.Count == 0) { metadata.AddValidationError(SR.IndicesAreNeeded(this.GetType().Name, this.DisplayName)); } else { IndexerHelper.CacheMethod (this.Indices, ref getMethod, ref setMethod); if (this.setMethod == null) { metadata.AddValidationError(SR.SpecialMethodNotFound("set_Item", typeof(TOperand).Name)); } } RuntimeArgument operandArgument = new RuntimeArgument("Operand", typeof(TOperand), ArgumentDirection.In, true); metadata.Bind(this.Operand, operandArgument); metadata.AddArgument(operandArgument); IndexerHelper.OnGetArguments (this.Indices, this.Result, metadata); } protected override Location Execute(CodeActivityContext context) { object[] indicesValue = new object[this.Indices.Count]; for (int i = 0; i < this.Indices.Count; i++) { indicesValue[i] = this.Indices[i].Get(context); } TOperand operandValue = this.Operand.Get(context); if (operandValue == null) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.MemberCannotBeNull("Operand", this.GetType().Name, this.DisplayName))); } return new IndexerLocation(operandValue, indicesValue, getMethod, setMethod); } [DataContract] class IndexerLocation : Location { [DataMember(EmitDefaultValue = false)] TOperand operand; [DataMember(EmitDefaultValue = false)] object[] indices; [DataMember(EmitDefaultValue = false)] object[] parameters; [DataMember(EmitDefaultValue = false)] MethodInfo getMethod; [DataMember(EmitDefaultValue = false)] MethodInfo setMethod; public IndexerLocation(TOperand operand, object[] indices, MethodInfo getMethod, MethodInfo setMethod) : base() { this.operand = operand; this.indices = indices; this.getMethod = getMethod; this.setMethod = setMethod; } public override TItem Value { get { Fx.Assert(this.operand != null, "operand must not be null"); Fx.Assert(this.indices != null, "indices must not be null"); if (this.getMethod == null) { throw FxTrace.Exception.AsError(new InvalidOperationException(SR.SpecialMethodNotFound("get_Item", typeof(TOperand).Name))); } return (TItem)this.getMethod.Invoke(this.operand, indices); } set { Fx.Assert(this.setMethod != null, "setMethod must not be null"); Fx.Assert(this.operand != null, "operand must not be null"); Fx.Assert(this.indices != null, "indices must not be null"); if (this.parameters == null) { this.parameters = new object[this.indices.Length + 1]; for (int i = 0; i < this.indices.Length; i++) { parameters[i] = this.indices[i]; } parameters[parameters.Length - 1] = value; } this.setMethod.Invoke(operand, parameters); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
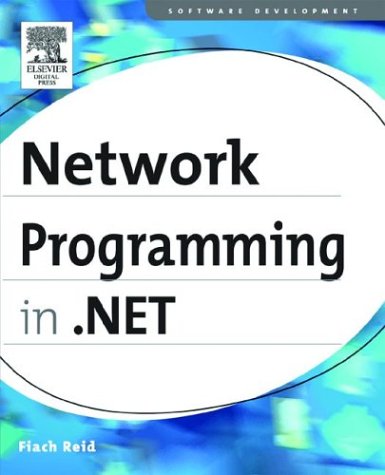
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RecordManager.cs
- SerialPinChanges.cs
- TextSelectionHelper.cs
- NodeLabelEditEvent.cs
- ImageFormatConverter.cs
- MenuItemBinding.cs
- ClientSettingsProvider.cs
- ClientEventManager.cs
- GridViewUpdatedEventArgs.cs
- Helpers.cs
- SafeNativeMethodsMilCoreApi.cs
- SQLSingle.cs
- XmlSchemaSimpleType.cs
- PixelFormats.cs
- UnsafeNativeMethods.cs
- IPAddressCollection.cs
- ContextMenuStripActionList.cs
- TraceAsyncResult.cs
- MonthChangedEventArgs.cs
- WeakReference.cs
- PresentationAppDomainManager.cs
- TripleDES.cs
- ReferenceEqualityComparer.cs
- StandardToolWindows.cs
- OdbcErrorCollection.cs
- AsyncCompletedEventArgs.cs
- ExpressionWriter.cs
- FileDialogCustomPlacesCollection.cs
- BasicExpressionVisitor.cs
- SeverityFilter.cs
- MetaForeignKeyColumn.cs
- EditorPartCollection.cs
- _DisconnectOverlappedAsyncResult.cs
- FormsAuthenticationConfiguration.cs
- XPathEmptyIterator.cs
- BitmapDownload.cs
- OperationContractGenerationContext.cs
- PeerDuplexChannelListener.cs
- ParagraphVisual.cs
- SelectionBorderGlyph.cs
- TypedAsyncResult.cs
- StorageSetMapping.cs
- Identifier.cs
- filewebresponse.cs
- Validator.cs
- CodeCommentStatement.cs
- UnsafeNativeMethods.cs
- XmlIncludeAttribute.cs
- InstanceKeyCompleteException.cs
- ProfileSettings.cs
- WrappedIUnknown.cs
- WorkflowStateRollbackService.cs
- MemberBinding.cs
- TemplateField.cs
- AuthenticationModuleElementCollection.cs
- FamilyMapCollection.cs
- WindowsFormsHost.cs
- CodeDomSerializerException.cs
- DriveInfo.cs
- sapiproxy.cs
- ImageButton.cs
- ConfigurationManager.cs
- ExceptionHelpers.cs
- LocalizableResourceBuilder.cs
- TextClipboardData.cs
- ResourcePart.cs
- ReachPageContentSerializerAsync.cs
- XmlEnumAttribute.cs
- DispatcherHookEventArgs.cs
- WindowsFormsDesignerOptionService.cs
- PermissionToken.cs
- TraceUtils.cs
- HtmlCommandAdapter.cs
- DataGridColumnCollectionEditor.cs
- ByteStreamGeometryContext.cs
- BindingListCollectionView.cs
- HttpCacheVaryByContentEncodings.cs
- DataGridRowClipboardEventArgs.cs
- HiddenField.cs
- HttpDebugHandler.cs
- HandlerBase.cs
- ParallelTimeline.cs
- XmlByteStreamReader.cs
- ObjectSecurityT.cs
- SelectorAutomationPeer.cs
- OleDbTransaction.cs
- NavigationWindowAutomationPeer.cs
- SecUtil.cs
- RegexMatch.cs
- UrlPath.cs
- UIElementParaClient.cs
- SortAction.cs
- WebPartZone.cs
- ObjectDataSourceChooseMethodsPanel.cs
- BigInt.cs
- SqlGenericUtil.cs
- WebEncodingValidatorAttribute.cs
- SmiGettersStream.cs
- _IPv6Address.cs
- SettingsProviderCollection.cs