Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.Activities / System / Activities / HybridCollection.cs / 1305376 / HybridCollection.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.Activities { using System; using System.Runtime.Serialization; using System.Collections.Generic; using System.Runtime; using System.Collections.ObjectModel; // used internally for performance in cases where a common usage pattern is a single item [DataContract] class HybridCollectionwhere T : class { [DataMember(EmitDefaultValue = false)] List multipleItems; [DataMember(EmitDefaultValue = false)] T singleItem; public HybridCollection() { } public HybridCollection(T initialItem) { Fx.Assert(initialItem != null, "null is used as a sentinal value and is not a valid item value for a hybrid collection"); this.singleItem = initialItem; } public T this[int index] { get { if (this.singleItem != null) { Fx.Assert(index == 0, "Out of range with a single item"); return this.singleItem; } else if (this.multipleItems != null) { Fx.Assert(index >= 0 && index < this.multipleItems.Count, "Out of range with multiple items."); return this.multipleItems[index]; } Fx.Assert("Out of range. There were no items in the HybridCollection."); return default(T); } } public int Count { get { if (this.singleItem != null) { return 1; } if (this.multipleItems != null) { return this.multipleItems.Count; } return 0; } } protected T SingleItem { get { return this.singleItem; } } protected IList MultipleItems { get { return this.multipleItems; } } public void Add(T item) { Fx.Assert(item != null, "null is used as a sentinal value and is not a valid item value for a hybrid collection"); if (this.multipleItems != null) { this.multipleItems.Add(item); } else if (this.singleItem != null) { this.multipleItems = new List (2); this.multipleItems.Add(this.singleItem); this.multipleItems.Add(item); this.singleItem = null; } else { this.singleItem = item; } } public ReadOnlyCollection AsReadOnly() { if (this.multipleItems != null) { return new ReadOnlyCollection (this.multipleItems); } else if (this.singleItem != null) { return new ReadOnlyCollection (new T[1] { this.singleItem }); } else { return new ReadOnlyCollection (new T[0]); } } // generally used for serialization purposes public void Compress() { if (this.multipleItems != null && this.multipleItems.Count == 1) { this.singleItem = this.multipleItems[0]; this.multipleItems = null; } } public void Remove(T item) { Remove(item, false); } internal void Remove(T item, bool searchingFromEnd) { if (this.singleItem != null) { Fx.Assert(object.Equals(item, this.singleItem), "The given item should be in this list. Something is wrong in our housekeeping."); this.singleItem = null; } else { Fx.Assert(this.multipleItems != null && this.multipleItems.Contains(item), "The given item should be in this list. Something is wrong in our housekeeping."); int position = (searchingFromEnd) ? this.multipleItems.LastIndexOf(item) : this.multipleItems.IndexOf(item); if (position != -1) { this.multipleItems.RemoveAt(position); } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
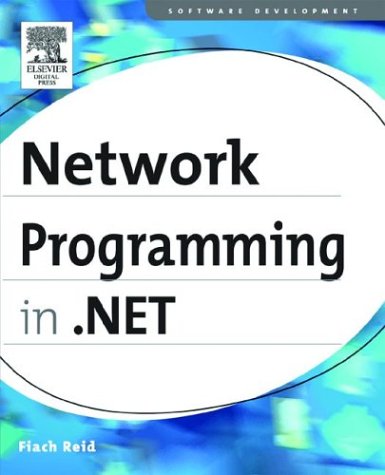
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TrackingMemoryStream.cs
- ColorContext.cs
- Msmq3PoisonHandler.cs
- HttpMethodAttribute.cs
- AtomMaterializer.cs
- CodeBinaryOperatorExpression.cs
- LoginName.cs
- SelectionList.cs
- RouteItem.cs
- SafeLibraryHandle.cs
- ContextProperty.cs
- SmtpLoginAuthenticationModule.cs
- SafeNativeMethods.cs
- DbXmlEnabledProviderManifest.cs
- PeerContact.cs
- VerificationAttribute.cs
- UIElementIsland.cs
- DataViewManager.cs
- StrokeNodeOperations.cs
- LineSegment.cs
- ExtensionFile.cs
- LocalizabilityAttribute.cs
- BatchParser.cs
- Misc.cs
- ScriptRef.cs
- SuppressMergeCheckAttribute.cs
- DoubleCollectionConverter.cs
- __Filters.cs
- AttachmentService.cs
- PropertyChangedEventManager.cs
- ConfigDefinitionUpdates.cs
- CollectionViewGroup.cs
- storepermission.cs
- SHA384CryptoServiceProvider.cs
- WebUtil.cs
- TextServicesHost.cs
- WebConfigurationHostFileChange.cs
- ExpandCollapseProviderWrapper.cs
- SqlDependencyListener.cs
- XmlSchemaObject.cs
- JobDuplex.cs
- ActiveXSerializer.cs
- DispatcherOperation.cs
- PrintPreviewGraphics.cs
- DataView.cs
- DataGridTableStyleMappingNameEditor.cs
- ImageSourceValueSerializer.cs
- HttpRuntime.cs
- BuildResult.cs
- FormClosedEvent.cs
- ImageAttributes.cs
- ByteFacetDescriptionElement.cs
- WebDisplayNameAttribute.cs
- BreakRecordTable.cs
- EventLogStatus.cs
- DynamicMetaObjectBinder.cs
- PriorityQueue.cs
- Storyboard.cs
- SerializerProvider.cs
- SqlCachedBuffer.cs
- _UncName.cs
- UdpDiscoveryEndpoint.cs
- CodePrimitiveExpression.cs
- PaginationProgressEventArgs.cs
- SchemaElementDecl.cs
- AllowedAudienceUriElement.cs
- WindowsFormsHost.cs
- AddInControllerImpl.cs
- PresentationSource.cs
- ObjectDataSourceMethodEventArgs.cs
- UriSection.cs
- filewebresponse.cs
- MessageBox.cs
- BulletDecorator.cs
- ConstrainedDataObject.cs
- Parsers.cs
- COAUTHINFO.cs
- TreeNode.cs
- SpellerHighlightLayer.cs
- ActivityExecutionWorkItem.cs
- ProcessRequestAsyncResult.cs
- SqlFunctionAttribute.cs
- MetadataArtifactLoaderComposite.cs
- BindStream.cs
- URLString.cs
- HashHelper.cs
- ValidatorCollection.cs
- SerializationInfo.cs
- X509CertificateStore.cs
- DefaultPropertyAttribute.cs
- DriveNotFoundException.cs
- SingleSelectRootGridEntry.cs
- WebPartChrome.cs
- FixedDocument.cs
- HashHelpers.cs
- EpmCustomContentWriterNodeData.cs
- ExpandoClass.cs
- ValueUtilsSmi.cs
- NativeMethods.cs
- ContractAdapter.cs