Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Activities / System / ServiceModel / Activities / Receive.cs / 1305376 / Receive.cs
//------------------------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------------------------- namespace System.ServiceModel.Activities { using System.Activities; using System.Activities.Expressions; using System.Activities.Statements; using System.Collections.Generic; using System.Collections.ObjectModel; using System.ComponentModel; using System.Diagnostics.CodeAnalysis; using System.Net.Security; using System.Runtime; using System.ServiceModel.Activities.Description; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.ServiceModel.Dispatcher; using System.ServiceModel.XamlIntegration; using System.Windows.Markup; using System.Xml.Linq; [ContentProperty("Content")] public sealed class Receive : Activity { MessageQuerySet correlatesOn; CollectioncorrelationInitializers; Collection knownTypes; // Used by contract-inference to build the ServiceDescription IList followingReplies; IList followingFaults; // cached by base.Implementation invocation InternalReceiveMessage internalReceive; FromRequest requestFormatter; public Receive() : base() { base.Implementation = () => { // if CacheMetadata isn't called, bail early if (this.internalReceive == null) { return null; } // requestFormatter is null if we have an untyped message situation if (this.requestFormatter == null) { return this.internalReceive; } else { Variable request = new Variable { Name = "RequestMessage" }; Variable noPersistHandle = new Variable { Name = "ReceiveNoPersistHandle" }; this.internalReceive.Message = new OutArgument (request); this.requestFormatter.Message = new InOutArgument (request); this.internalReceive.NoPersistHandle = new InArgument (noPersistHandle); this.requestFormatter.NoPersistHandle = new InArgument (noPersistHandle); return new Sequence { Variables = { request, noPersistHandle }, Activities = { this.internalReceive, this.requestFormatter } }; } }; } [SuppressMessage(FxCop.Category.Usage, FxCop.Rule.CollectionPropertiesShouldBeReadOnly, Justification = "MessageQuerySet is a stand-alone class. We want to allow users to create their own.")] [SuppressMessage(FxCop.Category.Xaml, FxCop.Rule.PropertyExternalTypesMustBeKnown, Justification = "MessageQuerySet is a known XAML-serializable type in this assembly.")] public MessageQuerySet CorrelatesOn { get { if (this.correlatesOn == null) { this.correlatesOn = new MessageQuerySet(); } return this.correlatesOn; } set { this.correlatesOn = value; } } // the content to receive (either message or parameters-based) declared by the user [DefaultValue(null)] public ReceiveContent Content { get; set; } // Internally, we should always use InternalContent since this property may have default content that we added internal ReceiveContent InternalContent { get { return this.Content ?? ReceiveContent.DefaultReceiveContent; } } // For initializing additional correlations beyond the Correlation property for following // activiites. public Collection CorrelationInitializers { get { if (this.correlationInitializers == null) { this.correlationInitializers = new Collection (); } return this.correlationInitializers; } } // Allows the action for the message to be specified by the user/designer. // If not set, the value is constructed from the Name of the activity. // If specified on the Send side of a message, the Receive side needs to have the same value in order // for the message to be delivered correctly. [DefaultValue(null)] public string Action { get; set; } // If true, a new workflow instance is creted to process the message. If false, an existing workflow // instance is determined based on correlations. [DefaultValue(false)] public bool CanCreateInstance { get; set; } [DefaultValue(null)] public InArgument CorrelatesWith { get; set; } // This will be used to construct the default value for Action if the Action property is // not specifically set. It is also used in conjunction with CorrelatesWith and IsReply // to determine which Send and Receive activities make up a Request/Reply combination. // There is validation to make sure either this or Action has a value. // Used by IServiceDescriptionBuilder2 as well [DefaultValue(null)] public string OperationName { get; set; } // The protection level for the message. // If ValueType or Value.Expression.ExpressionType is MessageContract, the MessageContract definition may have additional settings. // Default if this is not specified is Sign. [DefaultValue(null)] public ProtectionLevel? ProtectionLevel { get; set; } [DefaultValue(SerializerOption.DataContractSerializer)] public SerializerOption SerializerOption { get; set; } // The service contract name. This allows the same workflow instance to implement multiple // servce "contracts". If not specified and this is the first Receive activity in the // workflow, contract inference uses this activity's Name as the service contract name. [DefaultValue(null)] [TypeConverter(typeof(ServiceXNameTypeConverter))] public XName ServiceContractName { get; set; } public Collection KnownTypes { get { if (this.knownTypes == null) { this.knownTypes = new Collection (); } return this.knownTypes; } } internal string OperationBookmarkName { get { Fx.Assert(this.internalReceive != null, "CacheMetadata must be called before this!"); return this.internalReceive.OperationBookmarkName; } } internal Collection InternalKnownTypes { get { // avoid the allocation if internal return this.knownTypes; } } internal bool HasCorrelatesOn { get { return this.correlatesOn != null && this.correlatesOn.Count > 0; } } internal bool HasCorrelationInitializers { get { return this.correlationInitializers != null && this.correlationInitializers.Count > 0; } } internal IList FollowingReplies { get { if (this.followingReplies == null) { this.followingReplies = new List (); } return this.followingReplies; } } internal IList FollowingFaults { get { if (this.followingFaults == null) { this.followingFaults = new List (); } return this.followingFaults; } } internal bool HasReply { get { return this.followingReplies != null && this.followingReplies.Count > 0; } } internal bool HasFault { get { return this.followingFaults != null && this.followingFaults.Count > 0; } } internal InternalReceiveMessage InternalReceive { get { return this.internalReceive; } } protected override void CacheMetadata(ActivityMetadata metadata) { if (string.IsNullOrEmpty(this.OperationName)) { metadata.AddValidationError(SR.MissingOperationName(this.DisplayName)); } // validate Correlation Initializers MessagingActivityHelper.ValidateCorrelationInitializer(metadata, this.correlationInitializers, false, this.DisplayName, this.OperationName); // Add runtime arguments MessagingActivityHelper.AddRuntimeArgument(this.CorrelatesWith, "CorrelatesWith", Constants.CorrelationHandleType, ArgumentDirection.In, metadata); // Validate Content this.InternalContent.CacheMetadata(metadata, this, this.OperationName); if (this.correlationInitializers != null) { for (int i = 0; i < this.correlationInitializers.Count; i++) { CorrelationInitializer initializer = this.correlationInitializers[i]; initializer.ArgumentName = Constants.Parameter + i; RuntimeArgument initializerArgument = new RuntimeArgument(initializer.ArgumentName, Constants.CorrelationHandleType, ArgumentDirection.In); metadata.Bind(initializer.CorrelationHandle, initializerArgument); metadata.AddArgument(initializerArgument); } } if (!metadata.HasViolations) { this.internalReceive = CreateInternalReceive(); this.InternalContent.ConfigureInternalReceive(this.internalReceive, out this.requestFormatter); } else { this.internalReceive = null; this.requestFormatter = null; } } InternalReceiveMessage CreateInternalReceive() { InternalReceiveMessage result = new InternalReceiveMessage { OperationName = this.OperationName, ServiceContractName = this.ServiceContractName, CorrelatesWith = new InArgument (new ArgumentValue { ArgumentName = "CorrelatesWith" }), IsOneWay = true // This will be updated by contract inference logic, }; if (this.correlationInitializers != null) { foreach (CorrelationInitializer correlation in this.correlationInitializers) { result.CorrelationInitializers.Add(correlation.Clone()); } } return result; } internal void SetIsOneWay(bool flag) { Fx.Assert(this.internalReceive != null, "InternalReceiveMessage cannot be null!"); this.internalReceive.IsOneWay = flag; // perf optimization if the receive is two way, null out the NoPersistHandle, // this optimization allows us not to access AEC in InternalReceiveMessage->Execute to null out the // NoPersistHandle in case of two-way. With this optimization, we just assert in InternalReceiveMessage if (!this.internalReceive.IsOneWay) { this.internalReceive.NoPersistHandle = null; if (this.requestFormatter != null) { this.requestFormatter.NoPersistHandle = null; } } else { // For oneway operations the FromRequest should close the message if (this.requestFormatter != null) { this.requestFormatter.CloseMessage = true; } } } internal void SetFormatter(IDispatchMessageFormatter formatter, IDispatchFaultFormatter faultFormatter, bool includeExceptionDetailInFaults) { Fx.Assert(this.requestFormatter != null, "FromRequest cannot be null!"); this.requestFormatter.Formatter = formatter; if (this.followingReplies != null) { for (int i = 0; i < this.followingReplies.Count; i++) { this.followingReplies[i].SetFormatter(formatter); } } if (this.followingFaults != null) { for (int i = 0; i < this.followingFaults.Count; i++) { this.followingFaults[i].SetFaultFormatter(faultFormatter, includeExceptionDetailInFaults); } } } [EditorBrowsable(EditorBrowsableState.Never)] public bool ShouldSerializeCorrelatesOn() { // don't serialize null nor default MessageQuerySet if (this.correlatesOn == null || (this.correlatesOn.Name == null && this.correlatesOn.Count == 0)) { return false; } return true; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
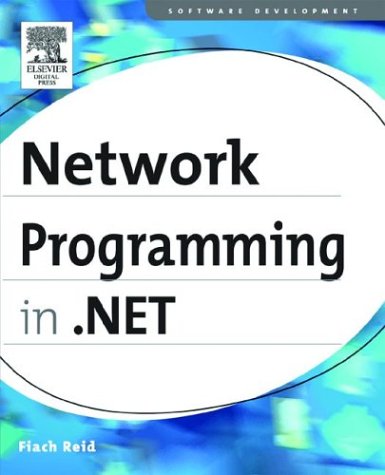
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- StaticSiteMapProvider.cs
- PresentationTraceSources.cs
- SmiSettersStream.cs
- ColumnHeaderConverter.cs
- ColumnClickEvent.cs
- LogicalExpr.cs
- ColorMap.cs
- SoapHttpTransportImporter.cs
- CustomWebEventKey.cs
- AuthenticatingEventArgs.cs
- GeneralTransform3DCollection.cs
- SystemIPAddressInformation.cs
- NativeCompoundFileAPIs.cs
- TemplateControl.cs
- DataListItemCollection.cs
- FlowDocument.cs
- BuildProvidersCompiler.cs
- Delay.cs
- PackageRelationshipCollection.cs
- AmbiguousMatchException.cs
- X509RawDataKeyIdentifierClause.cs
- ArrayWithOffset.cs
- Point3DCollection.cs
- AsyncResult.cs
- FixedBufferAttribute.cs
- MergePropertyDescriptor.cs
- InternalsVisibleToAttribute.cs
- Util.cs
- ApplicationInfo.cs
- EventlogProvider.cs
- HotSpot.cs
- IndexerNameAttribute.cs
- FontFamily.cs
- TreeNodeEventArgs.cs
- MenuAdapter.cs
- SqlWebEventProvider.cs
- OdbcException.cs
- SmtpReplyReaderFactory.cs
- ApplicationHost.cs
- BamlBinaryWriter.cs
- DodSequenceMerge.cs
- ContainsRowNumberChecker.cs
- Point3DValueSerializer.cs
- Rules.cs
- ProjectionRewriter.cs
- Grid.cs
- ImageAttributes.cs
- CultureData.cs
- DataGridViewDataConnection.cs
- ModelTreeEnumerator.cs
- Solver.cs
- CurrencyManager.cs
- QueryModel.cs
- ACL.cs
- ObjectParameterCollection.cs
- SqlUtils.cs
- XmlILStorageConverter.cs
- DataGridPagerStyle.cs
- PolyBezierSegment.cs
- BooleanToSelectiveScrollingOrientationConverter.cs
- TextSelectionHighlightLayer.cs
- UIElementIsland.cs
- BamlReader.cs
- Decoder.cs
- XPathConvert.cs
- ExpressionLexer.cs
- DataGridTextBox.cs
- XmlSchemaAttributeGroup.cs
- WebPartMenuStyle.cs
- contentDescriptor.cs
- HwndAppCommandInputProvider.cs
- TypedMessageConverter.cs
- ActivityExecutionFilter.cs
- HttpCacheVary.cs
- X509ChainPolicy.cs
- ArithmeticException.cs
- MethodBuilder.cs
- TreeNodeSelectionProcessor.cs
- Transform3D.cs
- MissingFieldException.cs
- ApplicationCommands.cs
- RuntimeIdentifierPropertyAttribute.cs
- HandoffBehavior.cs
- MarkupExtensionParser.cs
- HttpRuntimeSection.cs
- ReferencedCollectionType.cs
- Wizard.cs
- CellLabel.cs
- OleDbError.cs
- HostProtectionException.cs
- SerTrace.cs
- HttpRequest.cs
- HandlerBase.cs
- TrustLevelCollection.cs
- DataKeyCollection.cs
- SamlDoNotCacheCondition.cs
- LineSegment.cs
- ReadOnlyCollection.cs
- Label.cs
- DefaultPrintController.cs