Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Activities / System / ServiceModel / Activities / WorkflowHostingEndpoint.cs / 1305376 / WorkflowHostingEndpoint.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Activities { using System.Activities; using System.Collections.Generic; using System.Collections.ObjectModel; using System.Diagnostics.CodeAnalysis; using System.Globalization; using System.Runtime; using System.ServiceModel.Activities.Description; using System.ServiceModel.Activities.Dispatcher; using System.ServiceModel.Channels; using System.ServiceModel.Description; using System.ServiceModel.Dispatcher; public abstract class WorkflowHostingEndpoint : ServiceEndpoint { CollectioncorrelationQueries; protected WorkflowHostingEndpoint(Type contractType) : this(contractType, null, null) { } protected WorkflowHostingEndpoint(Type contractType, Binding binding, EndpointAddress address) : base(ContractDescription.GetContract(contractType), binding, address) { this.IsSystemEndpoint = true; this.Contract.Behaviors.Add(new ServiceMetadataContractBehavior(false)); this.Contract.Behaviors.Add(new WorkflowHostingContractBehavior()); Fx.Assert(!this.Behaviors.Contains(typeof(CorrelationQueryBehavior)), "Must not contain correlation query!"); this.correlationQueries = new Collection (); this.Behaviors.Add(new CorrelationQueryBehavior(this.correlationQueries)); // If TransactionFlowOption.Allowed or TransactionFlowOption.Mandatory is defined on an operation, we will set // TransactionScopeRequired = true for that operation. The operation will become transacted (use transaction flow, // or create one locally). For usability reason, we assume this is the majority usage. User could opt out by // setting TransactionScopeRequired to false or remove the TransactionFlowAttribute from the operation. foreach (OperationDescription operationDescription in this.Contract.Operations) { TransactionFlowAttribute transactionFlow = operationDescription.Behaviors.Find (); if (transactionFlow != null && transactionFlow.Transactions != TransactionFlowOption.NotAllowed) { OperationBehaviorAttribute operationAttribute = operationDescription.Behaviors.Find (); operationAttribute.TransactionScopeRequired = true; } } } public Collection CorrelationQueries { get { return this.correlationQueries; } } // There are two main scenario that user will override this api. // - For ResumeBookmark, User explicitly put or know how to extract InstanceId from Message. This enables user to provide // customized and lighter-weight (no InstanceKeys indirection) correlation. // - For Workflow Creation, User could provide a preferred Id for newly created Workflow Instance. protected internal virtual Guid OnGetInstanceId(object[] inputs, OperationContext operationContext) { return Guid.Empty; } protected internal virtual WorkflowCreationContext OnGetCreationContext( object[] inputs, OperationContext operationContext, Guid instanceId, WorkflowHostingResponseContext responseContext) { return null; } [SuppressMessage(FxCop.Category.Design, FxCop.Rule.AvoidOutParameters, Justification = "By design, return both Bookmark and its payload.")] protected internal virtual Bookmark OnResolveBookmark(object[] inputs, OperationContext operationContext, WorkflowHostingResponseContext responseContext, out object value) { value = null; return null; } internal static FaultException CreateDispatchFaultException() { FaultCode code = new FaultCode(FaultCodeConstants.Codes.InternalServiceFault, FaultCodeConstants.Namespaces.NetDispatch); code = FaultCode.CreateReceiverFaultCode(code); MessageFault dispatchFault = MessageFault.CreateFault(code, new FaultReason(new FaultReasonText(SR.InternalServerError, CultureInfo.CurrentCulture))); return new FaultException(dispatchFault, FaultCodeConstants.Actions.NetDispatcher); } class WorkflowHostingContractBehavior : IContractBehavior { public void AddBindingParameters(ContractDescription contractDescription, ServiceEndpoint endpoint, BindingParameterCollection bindingParameters) { } public void ApplyClientBehavior(ContractDescription contractDescription, ServiceEndpoint endpoint, ClientRuntime clientRuntime) { } public void ApplyDispatchBehavior(ContractDescription contractDescription, ServiceEndpoint endpoint, DispatchRuntime dispatchRuntime) { Fx.Assert(endpoint is WorkflowHostingEndpoint, "Must be hosting endpoint!"); foreach (OperationDescription operation in contractDescription.Operations) { if (operation.Behaviors.Find () == null) { operation.Behaviors.Add(new WorkflowHostingOperationBehavior((WorkflowHostingEndpoint)endpoint)); } } } public void Validate(ContractDescription contractDescription, ServiceEndpoint endpoint) { Fx.Assert(endpoint is WorkflowHostingEndpoint, "Must be hosting endpoint!"); } class WorkflowHostingOperationBehavior : WorkflowOperationBehavior { WorkflowHostingEndpoint hostingEndpoint; public WorkflowHostingOperationBehavior(WorkflowHostingEndpoint hostingEndpoint) : base(true) { this.hostingEndpoint = hostingEndpoint; } protected internal override Bookmark OnResolveBookmark(WorkflowOperationContext context, out BookmarkScope bookmarkScope, out object value) { CorrelationMessageProperty correlationMessageProperty; if (CorrelationMessageProperty.TryGet(context.OperationContext.IncomingMessageProperties, out correlationMessageProperty)) { bookmarkScope = new BookmarkScope(correlationMessageProperty.CorrelationKey.Value); } else { bookmarkScope = null; } WorkflowHostingResponseContext responseContext = new WorkflowHostingResponseContext(context); Bookmark bookmark = this.hostingEndpoint.OnResolveBookmark(context.Inputs, context.OperationContext, responseContext, out value); if (bookmark == null) { throw FxTrace.Exception.AsError(CreateDispatchFaultException()); } return bookmark; } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
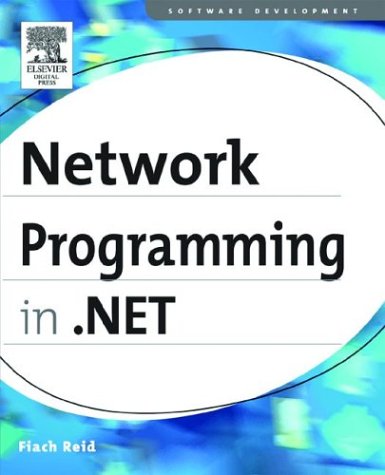
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AppDomainAttributes.cs
- DurationConverter.cs
- BezierSegment.cs
- StandardRuntimeEnumValidatorAttribute.cs
- HandlerBase.cs
- DataTableMapping.cs
- WebBrowsableAttribute.cs
- ExtensionFile.cs
- BrowsableAttribute.cs
- XmlSchemaObject.cs
- DateRangeEvent.cs
- SapiGrammar.cs
- TimeZone.cs
- HttpGetProtocolImporter.cs
- NetworkCredential.cs
- ListViewGroupItemCollection.cs
- DataRelation.cs
- ProcessProtocolHandler.cs
- ScriptResourceHandler.cs
- MetafileHeaderWmf.cs
- SelectionItemPattern.cs
- EnumerableRowCollection.cs
- TypeContext.cs
- CatchBlock.cs
- TryCatch.cs
- MultiSelectRootGridEntry.cs
- PreProcessor.cs
- SQLDateTime.cs
- Renderer.cs
- WSFederationHttpBinding.cs
- TextRangeEdit.cs
- COM2ColorConverter.cs
- Converter.cs
- MdiWindowListItemConverter.cs
- URLAttribute.cs
- Int16AnimationBase.cs
- SchemaCompiler.cs
- UTF7Encoding.cs
- MarkupObject.cs
- StylusButton.cs
- SignatureToken.cs
- XmlArrayItemAttribute.cs
- ArrayList.cs
- X509Certificate2.cs
- InProcStateClientManager.cs
- XmlDictionaryReader.cs
- TableLayoutPanel.cs
- RouteItem.cs
- ResourcePropertyMemberCodeDomSerializer.cs
- StrokeSerializer.cs
- GraphicsContainer.cs
- Image.cs
- InteropBitmapSource.cs
- DataViewListener.cs
- UrlPath.cs
- DataListComponentEditor.cs
- SupportingTokenDuplexChannel.cs
- LocationChangedEventArgs.cs
- BuildDependencySet.cs
- Vector3dCollection.cs
- ToolStripRenderer.cs
- ToolboxComponentsCreatingEventArgs.cs
- InkCanvasAutomationPeer.cs
- LogExtent.cs
- PerformanceCounterPermissionEntry.cs
- ClientTargetCollection.cs
- AttributeSetAction.cs
- GatewayDefinition.cs
- assemblycache.cs
- XComponentModel.cs
- CapabilitiesState.cs
- BCryptSafeHandles.cs
- NativeRecognizer.cs
- XmlCharacterData.cs
- CompatibleComparer.cs
- SmtpCommands.cs
- ResourcePermissionBase.cs
- RawStylusSystemGestureInputReport.cs
- StaticResourceExtension.cs
- InteropAutomationProvider.cs
- UrlMappingsModule.cs
- UrlMappingCollection.cs
- XmlRawWriter.cs
- ThreadStaticAttribute.cs
- ExtentJoinTreeNode.cs
- Pair.cs
- ListBase.cs
- WindowClosedEventArgs.cs
- X509RecipientCertificateServiceElement.cs
- ArrangedElementCollection.cs
- LayoutTableCell.cs
- SqlDataRecord.cs
- Utils.cs
- NumericUpDownAccelerationCollection.cs
- IBuiltInEvidence.cs
- MimeXmlReflector.cs
- PublishLicense.cs
- ResourceDefaultValueAttribute.cs
- MultipleViewProviderWrapper.cs
- ClientUIRequest.cs