Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / System.ServiceModel.Discovery / System / ServiceModel / Discovery / Configuration / ConfigurationUtility.cs / 1305376 / ConfigurationUtility.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.ServiceModel.Discovery.Configuration { using System.Configuration; using System.Runtime; using System.Security; using System.ServiceModel.Configuration; using System.ServiceModel.Description; using SR2 = System.ServiceModel.Discovery.SR; class ConfigurationUtility { public static ChannelEndpointElement GetDefaultDiscoveryEndpointElement() { return new ChannelEndpointElement() { Kind = ConfigurationStrings.UdpDiscoveryEndpoint }; } public static T LookupEndpoint(ChannelEndpointElement channelEndpointElement) where T : ServiceEndpoint { Fx.Assert(channelEndpointElement != null, "The parameter channelEndpointElement must be non null."); Fx.Assert(!string.IsNullOrEmpty(channelEndpointElement.Kind), "The Kind property of the specified channelEndpointElement parameter cannot be null or empty."); return ConfigLoader.LookupEndpoint(channelEndpointElement, null) as T; } internal static void InitializeAndValidateUdpChannelEndpointElement(ChannelEndpointElement channelEndpointElement) { if (!String.IsNullOrEmpty(channelEndpointElement.Binding)) { throw FxTrace.Exception.AsError(new ConfigurationErrorsException(SR2.DiscoveryConfigBindingSpecifiedForUdpDiscoveryEndpoint(channelEndpointElement.Kind)));; } if (!(channelEndpointElement.Address == null || String.IsNullOrEmpty(channelEndpointElement.Address.ToString()))) { throw FxTrace.Exception.AsError(new ConfigurationErrorsException(SR2.DiscoveryConfigAddressSpecifiedForUdpDiscoveryEndpoint(channelEndpointElement.Kind))); } channelEndpointElement.Address = null; } internal static void InitializeAndValidateUdpServiceEndpointElement(ServiceEndpointElement serviceEndpointElement) { if (!String.IsNullOrEmpty(serviceEndpointElement.Binding)) { throw FxTrace.Exception.AsError(new ConfigurationErrorsException(SR2.DiscoveryConfigBindingSpecifiedForUdpDiscoveryEndpoint(serviceEndpointElement.Kind)));; } if (!(serviceEndpointElement.Address == null || String.IsNullOrEmpty(serviceEndpointElement.Address.ToString()))) { throw FxTrace.Exception.AsError(new ConfigurationErrorsException(SR2.DiscoveryConfigAddressSpecifiedForUdpDiscoveryEndpoint(serviceEndpointElement.Kind))); } serviceEndpointElement.Address = null; if (serviceEndpointElement.ListenUri != null) { throw FxTrace.Exception.AsError(new ConfigurationErrorsException(SR2.DiscoveryConfigListenUriSpecifiedForUdpDiscoveryEndpoint(serviceEndpointElement.Kind))); } } [Fx.Tag.SecurityNote(Critical = "it is calling the SecurityCritical method to load client configuration section", Safe = "no configuration objects are leaked")] [SecuritySafeCritical] internal static TEndpoint LookupEndpointFromClientSection (string endpointConfigurationName) where TEndpoint : ServiceEndpoint { Fx.Assert(endpointConfigurationName != null, "The endpointConfigurationName parameter must be non null."); TEndpoint retval = default(TEndpoint); bool wildcard = string.Equals(endpointConfigurationName, "*", StringComparison.Ordinal); ClientSection clientSection = ClientSection.GetSection(); foreach (ChannelEndpointElement channelEndpointElement in clientSection.Endpoints) { if (string.IsNullOrEmpty(channelEndpointElement.Kind)) { continue; } if (endpointConfigurationName == channelEndpointElement.Name || wildcard) { TEndpoint endpoint = LookupEndpoint (channelEndpointElement); if (endpoint != null) { if (retval != null) { if (wildcard) { throw FxTrace.Exception.AsError( new InvalidOperationException( SR2.DiscoveryConfigMultipleEndpointsMatchWildcard( typeof(TEndpoint).FullName, clientSection.SectionInformation.SectionName))); } else { throw FxTrace.Exception.AsError( new InvalidOperationException( SR2.DiscoveryConfigMultipleEndpointsMatch( typeof(TEndpoint).FullName, endpointConfigurationName, clientSection.SectionInformation.SectionName))); } } else { retval = endpoint; } } } } if (retval == null) { if (wildcard) { throw FxTrace.Exception.AsError( new InvalidOperationException( SR2.DiscoveryConfigNoEndpointsMatchWildcard( typeof(TEndpoint).FullName, clientSection.SectionInformation.SectionName))); } else { throw FxTrace.Exception.AsError( new InvalidOperationException( SR2.DiscoveryConfigNoEndpointsMatch( typeof(TEndpoint).FullName, endpointConfigurationName, clientSection.SectionInformation.SectionName))); } } return retval; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
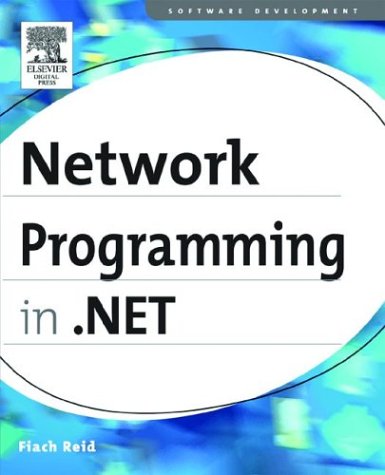
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RuntimeHandles.cs
- GAC.cs
- MimeMultiPart.cs
- ListDictionary.cs
- StreamReader.cs
- VirtualPathUtility.cs
- Int32Animation.cs
- CodeAttributeDeclarationCollection.cs
- BooleanSwitch.cs
- Image.cs
- UserControlParser.cs
- FontStyleConverter.cs
- DetailsViewUpdateEventArgs.cs
- unitconverter.cs
- IdleTimeoutMonitor.cs
- Slider.cs
- MessageSecurityOverTcpElement.cs
- EncoderParameters.cs
- GeneratedContractType.cs
- DbConnectionFactory.cs
- log.cs
- propertytag.cs
- RawUIStateInputReport.cs
- CompositeFontFamily.cs
- ScrollPattern.cs
- FormatPage.cs
- SafeNativeMethods.cs
- DocumentPageViewAutomationPeer.cs
- HwndTarget.cs
- ExpressionBindingCollection.cs
- AssemblyInfo.cs
- ControlBindingsCollection.cs
- IBuiltInEvidence.cs
- ParseHttpDate.cs
- ServicePointManagerElement.cs
- Stream.cs
- ContainerUIElement3D.cs
- XhtmlBasicImageAdapter.cs
- DragDrop.cs
- linebase.cs
- EncoderReplacementFallback.cs
- XamlTreeBuilder.cs
- RootProfilePropertySettingsCollection.cs
- MaskedTextBox.cs
- DiffuseMaterial.cs
- XmlWriterSettings.cs
- GridViewRowEventArgs.cs
- MinimizableAttributeTypeConverter.cs
- HttpClientCredentialType.cs
- PropertyChangedEventManager.cs
- AuthenticatedStream.cs
- ForceCopyBuildProvider.cs
- ImageIndexConverter.cs
- ScriptRegistrationManager.cs
- CallbackDebugElement.cs
- UIntPtr.cs
- InkCanvasFeedbackAdorner.cs
- XmlSiteMapProvider.cs
- RootProfilePropertySettingsCollection.cs
- SoapElementAttribute.cs
- ClaimSet.cs
- NavigationEventArgs.cs
- RequestCacheEntry.cs
- XmlDataLoader.cs
- RightsManagementInformation.cs
- DataContractSerializerSection.cs
- QilDataSource.cs
- GridViewPageEventArgs.cs
- BamlLocalizer.cs
- DefaultMemberAttribute.cs
- XmlBinaryWriterSession.cs
- XmlSchemaAnnotated.cs
- DataGridViewLinkColumn.cs
- MenuTracker.cs
- Highlights.cs
- Highlights.cs
- Command.cs
- InputElement.cs
- StatusBar.cs
- XmlProcessingInstruction.cs
- KeyConverter.cs
- Control.cs
- InheritanceContextHelper.cs
- XmlMemberMapping.cs
- TextCompositionEventArgs.cs
- WindowsStreamSecurityUpgradeProvider.cs
- DesignerSelectionListAdapter.cs
- ResourceDictionary.cs
- SoapElementAttribute.cs
- Rect.cs
- JapaneseCalendar.cs
- MenuItemStyle.cs
- BlockCollection.cs
- SetState.cs
- CodeSubDirectoriesCollection.cs
- SiteMapDataSource.cs
- HttpDateParse.cs
- XmlFormatExtensionPrefixAttribute.cs
- VisualCollection.cs
- SoapElementAttribute.cs