Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Base / Core / PropertyEditing / PropertyFilter.cs / 1305376 / PropertyFilter.cs
namespace System.Activities.Presentation.PropertyEditing { using System; using System.Collections.Generic; using System.Runtime; using System.Activities.Presentation; ////// This class is used as part of the searching/filtering functionality that may provided /// by the property editing host. It contains a list of predicates (i.e. strings to match against) /// [Fx.Tag.XamlVisible(false)] public class PropertyFilter { private List_predicates = new List (); /// /// Creates a PropertyFilter. /// /// String representation of predicates, space delimited public PropertyFilter(string filterText) { SetPredicates(filterText); } ////// Creates a PropertyFilter. /// /// IEnumerable collection of predicates public PropertyFilter(IEnumerablepredicates) { SetPredicates(predicates); } /// /// Readonly property that returns true if this PropertyFilter does not have any predicates /// public bool IsEmpty { get { return this._predicates == null || this._predicates.Count == 0; } } private void SetPredicates(string filterText) { if (string.IsNullOrEmpty(filterText)) return; string[] filterParts = filterText.Split(' '); for (int i=0; i < filterParts.Length; i++) { if (!string.IsNullOrEmpty(filterParts[i])) { _predicates.Add(new PropertyFilterPredicate(filterParts[i])); } } } private void SetPredicates(IEnumerablepredicates) { if (predicates == null) return; foreach (PropertyFilterPredicate predicate in predicates) { if (predicate != null) { _predicates.Add(predicate); } } } /// /// Matches this filter against a particular filter target. The /// filter returns true if there are no predicates or if one or more /// predicates match the filter target. /// /// Target to attempt matching ///True if there are no predicates or if one or more /// predicates match the filter target, false otherwise ///If target is null. public bool Match(IPropertyFilterTarget target) { if (target == null) throw FxTrace.Exception.ArgumentNull("target"); if (this.IsEmpty) return true; // Perform an OR over all predicates for (int i = 0; i < this._predicates.Count; i++) { if (target.MatchesPredicate(_predicates[i])) return true; } return false; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007. // Copyright (c) Microsoft Corporation. All rights reserved.
Link Menu
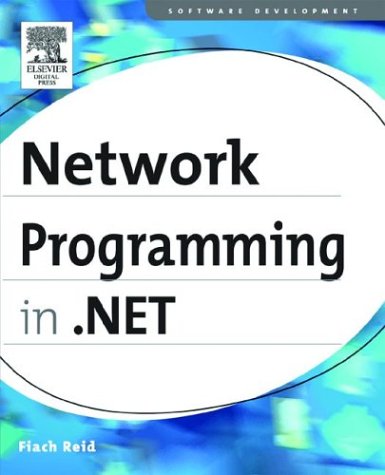
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SymbolTable.cs
- MultiByteCodec.cs
- SqlInternalConnection.cs
- ZipFileInfoCollection.cs
- RNGCryptoServiceProvider.cs
- ListViewUpdatedEventArgs.cs
- ListDataBindEventArgs.cs
- XmlElementCollection.cs
- Hyperlink.cs
- XmlSchemaFacet.cs
- WorkflowOperationAsyncResult.cs
- StateItem.cs
- MulticastIPAddressInformationCollection.cs
- TabPage.cs
- SqlConnectionHelper.cs
- SerializationStore.cs
- CopyOnWriteList.cs
- HttpModulesSection.cs
- CancelEventArgs.cs
- UserValidatedEventArgs.cs
- ConfigXmlCDataSection.cs
- HtmlImage.cs
- SkipQueryOptionExpression.cs
- UDPClient.cs
- FormsAuthenticationConfiguration.cs
- BuildProvidersCompiler.cs
- Triangle.cs
- ReadOnlyKeyedCollection.cs
- Splitter.cs
- WebProxyScriptElement.cs
- MethodCallConverter.cs
- DrawingVisualDrawingContext.cs
- xmlfixedPageInfo.cs
- TransactionCache.cs
- ParserExtension.cs
- CharUnicodeInfo.cs
- Bits.cs
- UInt64.cs
- UInt64Storage.cs
- RouteParametersHelper.cs
- VectorKeyFrameCollection.cs
- XmlSerializer.cs
- CodeDefaultValueExpression.cs
- DesignerTransaction.cs
- CodeMethodReturnStatement.cs
- OleDbCommandBuilder.cs
- InvalidCommandTreeException.cs
- FixedTextPointer.cs
- DataMemberAttribute.cs
- MessagingDescriptionAttribute.cs
- InvalidCastException.cs
- MSAAWinEventWrap.cs
- RoleServiceManager.cs
- ContextMenuService.cs
- Expression.DebuggerProxy.cs
- PagePropertiesChangingEventArgs.cs
- AdCreatedEventArgs.cs
- SystemTcpConnection.cs
- Int32CollectionConverter.cs
- VectorAnimationBase.cs
- WebPartRestoreVerb.cs
- SessionEndedEventArgs.cs
- SurrogateSelector.cs
- backend.cs
- SortDescriptionCollection.cs
- SliderAutomationPeer.cs
- ObjectItemCollectionAssemblyCacheEntry.cs
- BCLDebug.cs
- PatternMatchRules.cs
- XmlSchemaAttributeGroup.cs
- StringValueConverter.cs
- LineServices.cs
- Control.cs
- NullableDecimalMinMaxAggregationOperator.cs
- HandleCollector.cs
- StateWorkerRequest.cs
- FontCacheUtil.cs
- XPathEmptyIterator.cs
- CustomAttribute.cs
- InstanceDataCollection.cs
- SequenceDesignerAccessibleObject.cs
- ToolStripSeparatorRenderEventArgs.cs
- ReadOnlyHierarchicalDataSourceView.cs
- precedingquery.cs
- CustomErrorsSection.cs
- DoubleAnimationBase.cs
- TriState.cs
- BaseAsyncResult.cs
- ConfigurationSectionGroupCollection.cs
- CodeStatementCollection.cs
- CanonicalFormWriter.cs
- BamlResourceDeserializer.cs
- TriState.cs
- XmlILIndex.cs
- DynamicVirtualDiscoSearcher.cs
- DictionaryContent.cs
- XPathScanner.cs
- Events.cs
- Ray3DHitTestResult.cs
- BackgroundWorker.cs