Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / ErrorActivity.cs / 1305376 / ErrorActivity.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation { using System; using System.Activities; using System.Activities.Presentation.View; using System.ComponentModel; using System.Runtime; using System.Xaml; using XamlDeferLoad = System.Windows.Markup.XamlDeferLoadAttribute; [Designer(typeof(ErrorActivity.ErrorActivityView))] internal class ErrorActivity : Activity { static readonly AttachableMemberIdentifier HasErrorActivitiesProperty = new AttachableMemberIdentifier(typeof(ErrorActivity), "HasErrorActivities"); internal const string ErrorNodesProperty = "ErrorNodes"; [Browsable(false)] [XamlDeferLoad(typeof(NodeListLoader), typeof(object))] public XamlNodeList ErrorNodes { get; set; } internal static bool GetHasErrorActivities(object target) { object result; if (AttachablePropertyServices.TryGetProperty(target, HasErrorActivitiesProperty, out result)) { return (bool)result; } return false; } internal static void SetHasErrorActivities(object target, bool value) { AttachablePropertyServices.SetProperty(target, HasErrorActivitiesProperty, value); } internal static void WriteNodeList(XamlWriter writer, XamlNodeList nodeList) { // We need to pass the ErrorNodes contents through as a NodeList, because XOW doesn't // currently support unknown types, even inside a DeferLoad block. // But if a NodeList is written to XOW as a Value, XOW will unpack, forcing us to re-buffer // the nodes in our deferring loader. So we wrap the NodeList value inside a dummy StartObject. writer.WriteStartObject(XamlLanguage.Object); writer.WriteStartMember(XamlLanguage.Initialization); writer.WriteValue(nodeList); writer.WriteEndMember(); writer.WriteEndObject(); } internal class NodeListLoader : XamlDeferringLoader { public override object Load(XamlReader xamlReader, IServiceProvider serviceProvider) { // Expects a nodestream produced by WriteNodesList xamlReader.Read(); xamlReader.Read(); xamlReader.Read(); Fx.Assert(xamlReader.NodeType == XamlNodeType.Value, "Expected Value node"); return (XamlNodeList)xamlReader.Value; } public override XamlReader Save(object value, IServiceProvider serviceProvider) { return ((XamlNodeList)value).GetReader(); } } internal class ErrorActivityView : WorkflowViewElement { public ErrorActivityView() { WorkflowViewService.ShowErrorInViewElement(this, SR.ActivityLoadError, null); } } } [Designer(typeof(ErrorActivity.ErrorActivityView))] internal class ErrorActivity: Activity { [Browsable(false)] [XamlDeferLoad(typeof(ErrorActivity.NodeListLoader), typeof(object))] public XamlNodeList ErrorNodes { get; set; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
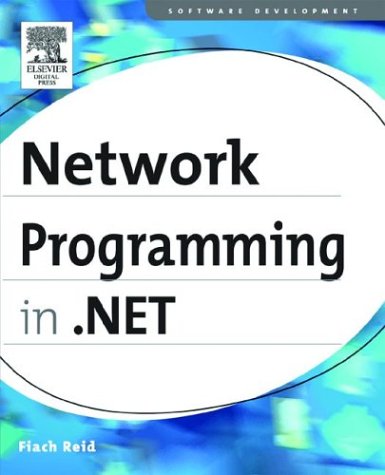
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- SparseMemoryStream.cs
- LongSumAggregationOperator.cs
- ServiceChannel.cs
- CharacterMetrics.cs
- Pkcs7Signer.cs
- CompilerScopeManager.cs
- StreamGeometry.cs
- WindowVisualStateTracker.cs
- SqlBooleanizer.cs
- ActivityTrace.cs
- NoneExcludedImageIndexConverter.cs
- XmlSchemaGroupRef.cs
- EventMap.cs
- ScrollChrome.cs
- LoopExpression.cs
- ValidationRuleCollection.cs
- UnsafeNativeMethods.cs
- LayoutEngine.cs
- DistinctQueryOperator.cs
- TemplatedWizardStep.cs
- EntityDataSourceState.cs
- coordinatorfactory.cs
- TdsValueSetter.cs
- TypeValidationEventArgs.cs
- PackagePart.cs
- HitTestParameters3D.cs
- ApplicationServiceHelper.cs
- DropDownButton.cs
- CssStyleCollection.cs
- Pens.cs
- PriorityBinding.cs
- MultiView.cs
- DebugView.cs
- FixedSOMContainer.cs
- RepeaterItemCollection.cs
- OutOfMemoryException.cs
- Pointer.cs
- LogReservationCollection.cs
- RepeatInfo.cs
- InputScopeNameConverter.cs
- ClientSettings.cs
- ColorInterpolationModeValidation.cs
- ISessionStateStore.cs
- Geometry.cs
- EncryptedType.cs
- ShapingWorkspace.cs
- TcpChannelFactory.cs
- DesignerRegion.cs
- tooltip.cs
- StorageEntityTypeMapping.cs
- MobileListItem.cs
- OdbcDataReader.cs
- ArrayList.cs
- ConcurrentBag.cs
- assemblycache.cs
- OracleSqlParser.cs
- CheckBox.cs
- SafeCryptHandles.cs
- ObjectSet.cs
- AssertValidation.cs
- BoolExpression.cs
- UnsafeNativeMethods.cs
- Constraint.cs
- WebPartConnectionsCancelEventArgs.cs
- ToolboxCategory.cs
- CapabilitiesSection.cs
- LambdaCompiler.Generated.cs
- ContainerActivationHelper.cs
- SwitchAttribute.cs
- BufferedStream.cs
- SharedStatics.cs
- Debug.cs
- ComponentManagerBroker.cs
- InstanceNameConverter.cs
- NativeObjectSecurity.cs
- HelpEvent.cs
- AffineTransform3D.cs
- EventProviderClassic.cs
- SMSvcHost.cs
- TransactionState.cs
- SourceFileInfo.cs
- AuthorizationRule.cs
- FacetChecker.cs
- MailMessage.cs
- FormViewRow.cs
- InkCanvasSelectionAdorner.cs
- XmlAnyAttributeAttribute.cs
- IntPtr.cs
- CommandBinding.cs
- AdapterDictionary.cs
- TextOutput.cs
- ErasingStroke.cs
- PathParser.cs
- OutputCacheProfileCollection.cs
- GlobalizationAssembly.cs
- ChangeBlockUndoRecord.cs
- StylusPointPropertyId.cs
- BufferAllocator.cs
- ConfigurationStrings.cs
- DataBoundLiteralControl.cs