Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / Model / XamlUtilities.cs / 1305376 / XamlUtilities.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.Model { using System; using System.Collections; using System.ComponentModel; using System.Reflection; internal static class XamlUtilities { static Hashtable converterCache; static object converterCacheSyncObject = new object(); public static TypeConverter GetConverter(Type itemType) { TypeConverter converter = TypeDescriptor.GetConverter(itemType); if (converter == null || converter.GetType() == typeof(TypeConverter)) { // We got an invalid converter. WPF will do this if the converter // is internal, but we use internal converters all over the place // at design time. Detect this and build the converter ourselves. if (converterCache != null) { converter = (TypeConverter)converterCache[itemType]; if (converter != null) { return converter; } } AttributeCollection attrs = TypeDescriptor.GetAttributes(itemType); TypeConverterAttribute tca = attrs[typeof(TypeConverterAttribute)] as TypeConverterAttribute; if (tca != null && tca.ConverterTypeName != null) { Type type = Type.GetType(tca.ConverterTypeName); if (type != null && !type.IsPublic && typeof(TypeConverter).IsAssignableFrom(type)) { ConstructorInfo ctor = type.GetConstructor(new Type[] { typeof(Type) }); if (ctor != null) { converter = (TypeConverter)ctor.Invoke(new object[] { itemType }); } else { converter = (TypeConverter)Activator.CreateInstance(type); } lock (converterCacheSyncObject) { if (converterCache == null) { converterCache = new Hashtable(); // Listen to type changes and clear the cache. // This allows new metadata tables to be installed TypeDescriptor.Refreshed += delegate(RefreshEventArgs args) { converterCache.Remove(args.TypeChanged); }; } converterCache[itemType] = converter; } } } } return converter; } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
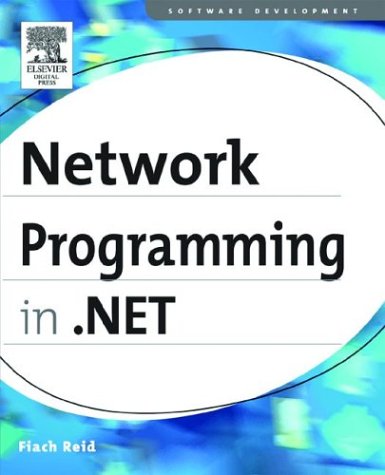
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- RegexNode.cs
- RootBuilder.cs
- DataPager.cs
- ResourcePermissionBase.cs
- ToolBarButtonClickEvent.cs
- SqlDelegatedTransaction.cs
- ResourceReferenceExpressionConverter.cs
- SimpleType.cs
- HttpPostedFile.cs
- SafeEventHandle.cs
- PolyBezierSegmentFigureLogic.cs
- TraceUtility.cs
- SafeFileMapViewHandle.cs
- UInt64.cs
- Operators.cs
- ListQueryResults.cs
- DbInsertCommandTree.cs
- EventSinkHelperWriter.cs
- MediaTimeline.cs
- DeviceFilterDictionary.cs
- SymmetricAlgorithm.cs
- KerberosRequestorSecurityToken.cs
- NotEqual.cs
- ReferenceSchema.cs
- CompiledQueryCacheKey.cs
- WebEventTraceProvider.cs
- TextEncodedRawTextWriter.cs
- HtmlControl.cs
- RSAPKCS1KeyExchangeDeformatter.cs
- ISCIIEncoding.cs
- RegexCharClass.cs
- WinEventWrap.cs
- COM2ExtendedUITypeEditor.cs
- TreeNodeStyle.cs
- JumpItem.cs
- DefaultBinder.cs
- ScaleTransform3D.cs
- DataBoundControlActionList.cs
- StrongNameHelpers.cs
- DeviceContext.cs
- CommandValueSerializer.cs
- NameValuePair.cs
- StringKeyFrameCollection.cs
- ObjectManager.cs
- CommittableTransaction.cs
- XmlSchemaSimpleContentExtension.cs
- InProcStateClientManager.cs
- DelegatingHeader.cs
- DictionarySurrogate.cs
- UdpDiscoveryEndpoint.cs
- ImplicitInputBrush.cs
- ExpressionList.cs
- CombinedGeometry.cs
- SqlCrossApplyToCrossJoin.cs
- SqlParameter.cs
- HtmlInputFile.cs
- TableRowsCollectionEditor.cs
- ActivityCollectionMarkupSerializer.cs
- ScriptingSectionGroup.cs
- IndentedWriter.cs
- BaseDataListComponentEditor.cs
- EdmEntityTypeAttribute.cs
- SymbolMethod.cs
- ByteKeyFrameCollection.cs
- AutomationEventArgs.cs
- ReadOnlyDataSource.cs
- MemberCollection.cs
- DesignerTransaction.cs
- BoundingRectTracker.cs
- ByteKeyFrameCollection.cs
- Timer.cs
- AutomationIdentifier.cs
- UriScheme.cs
- CodeAttributeDeclaration.cs
- XmlAttributeProperties.cs
- TransformCollection.cs
- Substitution.cs
- OleDbSchemaGuid.cs
- ConfigPathUtility.cs
- MostlySingletonList.cs
- RequestCachePolicy.cs
- TemplateControlBuildProvider.cs
- FunctionDetailsReader.cs
- ServiceModelDictionary.cs
- AffineTransform3D.cs
- GridSplitterAutomationPeer.cs
- SocketPermission.cs
- SelectionProcessor.cs
- QueryOutputWriter.cs
- ObjectDataSourceSelectingEventArgs.cs
- TagPrefixAttribute.cs
- MetaDataInfo.cs
- KeyConstraint.cs
- AttachedAnnotationChangedEventArgs.cs
- QuaternionAnimationBase.cs
- XmlStreamStore.cs
- StandardBindingImporter.cs
- OutputBuffer.cs
- Vector3dCollection.cs
- CapabilitiesState.cs