Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / NetFx40 / Tools / System.Activities.Presentation / System / Activities / Presentation / View / WindowExtensionMethods.cs / 1305376 / WindowExtensionMethods.cs
//---------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------- namespace System.Activities.Presentation.View { using System.Runtime.InteropServices; using System.Windows; using System.Windows.Interop; static class WindowExtensionMethods { public static void ShowContextHelpButton(this Window window) { IntPtr hwnd = new WindowInteropHelper(window).Handle; IntPtr exStyle = Win32Interop.GetWindowLongPtr(hwnd, Win32Interop.GWL_EXSTYLE); if (IntPtr.Size == 4) { exStyle = new IntPtr(exStyle.ToInt32() | Win32Interop.WS_EX_CONTEXTHELP); } else { exStyle = new IntPtr(exStyle.ToInt64() | ((long)Win32Interop.WS_EX_CONTEXTHELP)); } Win32Interop.SetWindowLongPtr(new HandleRef(window, hwnd), Win32Interop.GWL_EXSTYLE, exStyle); } public static void HideMinMaxButton(this Window window) { IntPtr hwnd = new WindowInteropHelper(window).Handle; IntPtr style = Win32Interop.GetWindowLongPtr(hwnd, Win32Interop.GWL_STYLE); if (IntPtr.Size == 4) { int intValue = style.ToInt32(); intValue = SetBit(Win32Interop.WS_MAXIMIZEBOX, intValue, false); intValue = SetBit(Win32Interop.WS_MINIMIZEBOX, intValue, false); style = new IntPtr(intValue); } else { long longValue = style.ToInt64(); longValue = SetBit((long)Win32Interop.WS_MAXIMIZEBOX, longValue, false); longValue = SetBit((long)Win32Interop.WS_MINIMIZEBOX, longValue, false); style = new IntPtr(longValue); } Win32Interop.SetWindowLongPtr(new HandleRef(window, hwnd), Win32Interop.GWL_STYLE, style); } public static void AddWindowsHook(this Window window, HwndSourceHook wmHandler) { IntPtr hwnd = new WindowInteropHelper(window).Handle; HwndSource source = HwndSource.FromHwnd(hwnd); source.AddHook(wmHandler); } public static void RemoveWindowsHook(this Window window, HwndSourceHook wmHandler) { IntPtr hwnd = new WindowInteropHelper(window).Handle; HwndSource source = HwndSource.FromHwnd(hwnd); source.RemoveHook(wmHandler); } public static void HideIcon(this Window window) { IntPtr hwnd = new WindowInteropHelper(window).Handle; IntPtr exStyle = Win32Interop.GetWindowLongPtr(hwnd, Win32Interop.GWL_EXSTYLE); if (IntPtr.Size == 4) { exStyle = new IntPtr(exStyle.ToInt32() | Win32Interop.WS_EX_DLGMODALFRAME); } else { exStyle = new IntPtr(exStyle.ToInt64() | ((long)Win32Interop.WS_EX_DLGMODALFRAME)); } Win32Interop.SetWindowLongPtr(new HandleRef(window, hwnd), Win32Interop.GWL_EXSTYLE, exStyle); Win32Interop.SendMessage(hwnd, Win32Interop.WM_SETICON, new IntPtr(Win32Interop.ICON_SMALL), IntPtr.Zero); Win32Interop.SendMessage(hwnd, Win32Interop.WM_SETICON, new IntPtr(Win32Interop.ICON_BIG), IntPtr.Zero); } private static long SetBit(long mask, long value, bool flag) { if (flag) { return value | mask; } else { return value & ~mask; } } private static int SetBit(int mask, int value, bool flag) { if (flag) { return value | mask; } else { return value & ~mask; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
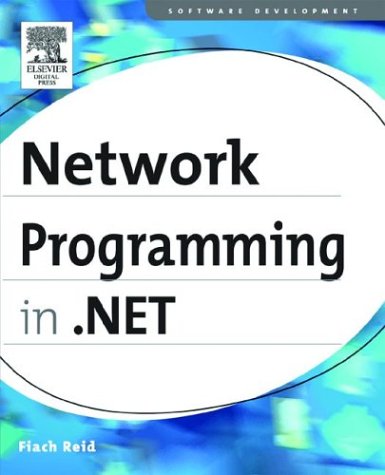
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- AxisAngleRotation3D.cs
- EntityDataSourceWrapper.cs
- OleServicesContext.cs
- ActiveXSite.cs
- Pen.cs
- XslTransform.cs
- MbpInfo.cs
- EntityClientCacheKey.cs
- DataBindingCollectionEditor.cs
- TemplateControlCodeDomTreeGenerator.cs
- ListViewHitTestInfo.cs
- LifetimeServices.cs
- AudioFileOut.cs
- HtmlControlPersistable.cs
- Util.cs
- ExpressionDumper.cs
- NavigationWindow.cs
- GuidelineSet.cs
- ToolStripDropDownMenu.cs
- CompiledRegexRunner.cs
- DataTrigger.cs
- GeneralTransform2DTo3D.cs
- CompilationSection.cs
- SerialPort.cs
- ExitEventArgs.cs
- TreeViewItem.cs
- FlowDocumentScrollViewer.cs
- ValidationResults.cs
- XmlSchemaAnyAttribute.cs
- MiniCustomAttributeInfo.cs
- PackageStore.cs
- MSAAEventDispatcher.cs
- SecurityContext.cs
- BrowserDefinitionCollection.cs
- DataGridViewAutoSizeModeEventArgs.cs
- ReadOnlyDataSource.cs
- ProcessHostFactoryHelper.cs
- SmiEventSink.cs
- LocalizabilityAttribute.cs
- VirtualDirectoryMapping.cs
- ConfigurationErrorsException.cs
- XmlSerializerSection.cs
- TableCellAutomationPeer.cs
- WebReferencesBuildProvider.cs
- SequenceDesignerAccessibleObject.cs
- CipherData.cs
- ExtensibleClassFactory.cs
- ProcessThreadCollection.cs
- ScaleTransform3D.cs
- KnownTypeDataContractResolver.cs
- GroupByExpressionRewriter.cs
- TextDecorationCollection.cs
- MultipartIdentifier.cs
- ManifestBasedResourceGroveler.cs
- Metafile.cs
- ITextView.cs
- RequestCachePolicy.cs
- ScriptingRoleServiceSection.cs
- SessionStateUtil.cs
- ListViewGroup.cs
- InvalidProgramException.cs
- BasicCellRelation.cs
- SignerInfo.cs
- DropShadowBitmapEffect.cs
- DefaultIfEmptyQueryOperator.cs
- ValueChangedEventManager.cs
- FlowDocumentReader.cs
- DataQuery.cs
- PeerCustomResolverElement.cs
- ValueSerializer.cs
- SqlBinder.cs
- _Win32.cs
- ValidatedControlConverter.cs
- RequestQueue.cs
- _CommandStream.cs
- NestPullup.cs
- ParseNumbers.cs
- PropertyCondition.cs
- Marshal.cs
- QuaternionIndependentAnimationStorage.cs
- DbgCompiler.cs
- IdentityNotMappedException.cs
- BinaryParser.cs
- FileCodeGroup.cs
- FrameworkContentElementAutomationPeer.cs
- XmlElementAttribute.cs
- ContainerSelectorActiveEvent.cs
- SqlSelectStatement.cs
- SafeNativeMethods.cs
- MetadataItem_Static.cs
- elementinformation.cs
- ImageSource.cs
- GeneralTransform3DTo2DTo3D.cs
- TextEditorContextMenu.cs
- CTreeGenerator.cs
- DataGridColumn.cs
- QueryOperationResponseOfT.cs
- OutKeywords.cs
- ContentElementAutomationPeer.cs
- XmlILOptimizerVisitor.cs