Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / IdentityModel / System / IdentityModel / Selectors / SecurityTokenResolver.cs / 1305376 / SecurityTokenResolver.cs
//------------------------------------------------------------ // Copyright (c) Microsoft Corporation. All rights reserved. //----------------------------------------------------------- namespace System.IdentityModel.Selectors { using System.Collections.ObjectModel; using System.IdentityModel.Tokens; public abstract class SecurityTokenResolver { public SecurityToken ResolveToken(SecurityKeyIdentifier keyIdentifier) { if (keyIdentifier == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifier"); } SecurityToken token; if (!this.TryResolveTokenCore(keyIdentifier, out token)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperWarning(new InvalidOperationException(SR.GetString(SR.UnableToResolveTokenReference, keyIdentifier))); } return token; } public bool TryResolveToken(SecurityKeyIdentifier keyIdentifier, out SecurityToken token) { if (keyIdentifier == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifier"); } return TryResolveTokenCore(keyIdentifier, out token); } public SecurityToken ResolveToken(SecurityKeyIdentifierClause keyIdentifierClause) { if (keyIdentifierClause == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); } SecurityToken token; if (!this.TryResolveTokenCore(keyIdentifierClause, out token)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperWarning(new InvalidOperationException(SR.GetString(SR.UnableToResolveTokenReference, keyIdentifierClause))); } return token; } public bool TryResolveToken(SecurityKeyIdentifierClause keyIdentifierClause, out SecurityToken token) { if (keyIdentifierClause == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); } return this.TryResolveTokenCore(keyIdentifierClause, out token); } public SecurityKey ResolveSecurityKey(SecurityKeyIdentifierClause keyIdentifierClause) { if (keyIdentifierClause == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); } SecurityKey key; if (!this.TryResolveSecurityKeyCore(keyIdentifierClause, out key)) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperWarning(new InvalidOperationException(SR.GetString(SR.UnableToResolveKeyReference, keyIdentifierClause))); } return key; } public bool TryResolveSecurityKey(SecurityKeyIdentifierClause keyIdentifierClause, out SecurityKey key) { if (keyIdentifierClause == null) { throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); } return this.TryResolveSecurityKeyCore(keyIdentifierClause, out key); } // protected methods protected abstract bool TryResolveTokenCore(SecurityKeyIdentifier keyIdentifier, out SecurityToken token); protected abstract bool TryResolveTokenCore(SecurityKeyIdentifierClause keyIdentifierClause, out SecurityToken token); protected abstract bool TryResolveSecurityKeyCore(SecurityKeyIdentifierClause keyIdentifierClause, out SecurityKey key); public static SecurityTokenResolver CreateDefaultSecurityTokenResolver(ReadOnlyCollectiontokens, bool canMatchLocalId) { return new SimpleTokenResolver(tokens, canMatchLocalId); } class SimpleTokenResolver : SecurityTokenResolver { ReadOnlyCollection tokens; bool canMatchLocalId; public SimpleTokenResolver(ReadOnlyCollection tokens, bool canMatchLocalId) { if (tokens == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("tokens"); this.tokens = tokens; this.canMatchLocalId = canMatchLocalId; } protected override bool TryResolveSecurityKeyCore(SecurityKeyIdentifierClause keyIdentifierClause, out SecurityKey key) { if (keyIdentifierClause == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); key = null; for (int i = 0; i < this.tokens.Count; ++i) { SecurityKey securityKey = this.tokens[i].ResolveKeyIdentifierClause(keyIdentifierClause); if (securityKey != null) { key = securityKey; return true; } } if (keyIdentifierClause is EncryptedKeyIdentifierClause) { EncryptedKeyIdentifierClause keyClause = (EncryptedKeyIdentifierClause)keyIdentifierClause; SecurityKeyIdentifier keyIdentifier = keyClause.EncryptingKeyIdentifier; if (keyIdentifier != null && keyIdentifier.Count > 0) { for (int i = 0; i < keyIdentifier.Count; i++) { SecurityKey unwrappingSecurityKey = null; if (TryResolveSecurityKey(keyIdentifier[i], out unwrappingSecurityKey)) { byte[] wrappedKey = keyClause.GetEncryptedKey(); string wrappingAlgorithm = keyClause.EncryptionMethod; byte[] unwrappedKey = unwrappingSecurityKey.DecryptKey(wrappingAlgorithm, wrappedKey); key = new InMemorySymmetricSecurityKey(unwrappedKey, false); return true; } } } } return key != null; } protected override bool TryResolveTokenCore(SecurityKeyIdentifier keyIdentifier, out SecurityToken token) { if (keyIdentifier == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifier"); token = null; for (int i = 0; i < keyIdentifier.Count; ++i) { SecurityToken securityToken = ResolveSecurityToken(keyIdentifier[i]); if (securityToken != null) { token = securityToken; break; } } return (token != null); } protected override bool TryResolveTokenCore(SecurityKeyIdentifierClause keyIdentifierClause, out SecurityToken token) { if (keyIdentifierClause == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); token = null; SecurityToken securityToken = ResolveSecurityToken(keyIdentifierClause); if (securityToken != null) token = securityToken; return (token != null); } SecurityToken ResolveSecurityToken(SecurityKeyIdentifierClause keyIdentifierClause) { if (keyIdentifierClause == null) throw DiagnosticUtility.ExceptionUtility.ThrowHelperArgumentNull("keyIdentifierClause"); if (!this.canMatchLocalId && keyIdentifierClause is LocalIdKeyIdentifierClause) return null; for (int i = 0; i < this.tokens.Count; ++i) { if (this.tokens[i].MatchesKeyIdentifierClause(keyIdentifierClause)) return this.tokens[i]; } return null; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
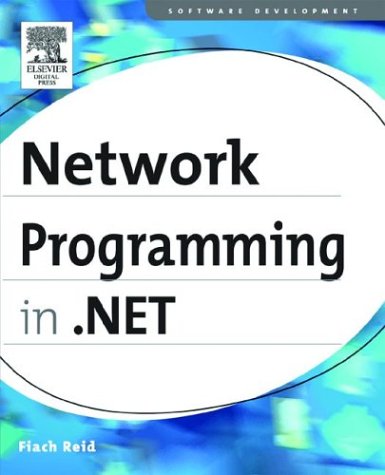
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- PersistChildrenAttribute.cs
- NavigateEvent.cs
- InkPresenter.cs
- DefinitionUpdate.cs
- ComEventsSink.cs
- FloatUtil.cs
- RTTypeWrapper.cs
- Button.cs
- MetadataItemEmitter.cs
- EntitySqlQueryBuilder.cs
- shaperfactoryquerycachekey.cs
- MouseActionValueSerializer.cs
- IIS7UserPrincipal.cs
- ImageUrlEditor.cs
- ActivationService.cs
- XmlSchemaInfo.cs
- oledbconnectionstring.cs
- ModelPropertyDescriptor.cs
- SystemIPInterfaceStatistics.cs
- Timeline.cs
- DesignerVerbCollection.cs
- Int64KeyFrameCollection.cs
- TypeUnloadedException.cs
- EncodingTable.cs
- ListenerElementsCollection.cs
- CatalogPart.cs
- IntSecurity.cs
- OracleSqlParser.cs
- BaseAsyncResult.cs
- SEHException.cs
- LifetimeManager.cs
- ReferencedCollectionType.cs
- CompensatableTransactionScopeActivityDesigner.cs
- TransformConverter.cs
- PerfService.cs
- ProfileGroupSettings.cs
- CorrelationKey.cs
- MappedMetaModel.cs
- HttpStreamXmlDictionaryReader.cs
- SerialStream.cs
- AssemblyBuilderData.cs
- sitestring.cs
- SelectionItemPattern.cs
- PointHitTestResult.cs
- HttpProcessUtility.cs
- ApplicationActivator.cs
- DataColumnChangeEvent.cs
- Coordinator.cs
- SecurityDocument.cs
- WebException.cs
- OneToOneMappingSerializer.cs
- XmlSchemaGroupRef.cs
- ProxyHwnd.cs
- DeflateInput.cs
- RectValueSerializer.cs
- KnownBoxes.cs
- ValidatedControlConverter.cs
- Help.cs
- PropertyTabChangedEvent.cs
- CompilerErrorCollection.cs
- IPEndPointCollection.cs
- DataDocumentXPathNavigator.cs
- AnimatedTypeHelpers.cs
- CompoundFileStreamReference.cs
- DocumentNUp.cs
- MULTI_QI.cs
- Material.cs
- SQLInt64Storage.cs
- GifBitmapEncoder.cs
- ExpressionWriter.cs
- FlowPosition.cs
- CreateParams.cs
- DictionaryItemsCollection.cs
- InkPresenter.cs
- COMException.cs
- MachineKeySection.cs
- ThemeDictionaryExtension.cs
- TreeBuilder.cs
- PenThreadWorker.cs
- TextBox.cs
- InputLanguage.cs
- SystemWebCachingSectionGroup.cs
- MimeWriter.cs
- ProcessModuleCollection.cs
- log.cs
- NavigationProperty.cs
- ReadOnlyCollectionBuilder.cs
- FrugalMap.cs
- LoopExpression.cs
- CodeDomExtensionMethods.cs
- BinaryConverter.cs
- SqlParameter.cs
- PersonalizationAdministration.cs
- ProgressBar.cs
- MetadataItemEmitter.cs
- CreateParams.cs
- PartManifestEntry.cs
- ProcessModule.cs
- WebConfigurationManager.cs
- Marshal.cs