Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / cdf / src / WCF / System.ServiceModel.Activation / System / ServiceModel / Activation / HostedTcpTransportManager.cs / 1305376 / HostedTcpTransportManager.cs
//---------------------------------------------------------------------------- // Copyright (c) Microsoft Corporation. All rights reserved. //--------------------------------------------------------------------------- namespace System.ServiceModel.Activation { using System.ServiceModel.Channels; using System.Collections.Generic; using System.Diagnostics; class HostedTcpTransportManager : SharedTcpTransportManager { bool settingsApplied; ActiononViaCallback; public HostedTcpTransportManager(BaseUriWithWildcard baseAddress) : base(baseAddress.BaseAddress) { this.HostNameComparisonMode = baseAddress.HostNameComparisonMode; this.onViaCallback = new Action (OnVia); } internal void Start(int queueId, Guid token, Action messageReceivedCallback) { SetMessageReceivedCallback(messageReceivedCallback); OnOpenInternal(queueId, token); } internal override void OnOpen() { // This is intentionally empty. } internal override void OnClose(TimeSpan timeout) { // This is intentionally empty. } internal override void OnAbort() { // This is intentionally empty. } internal void Stop(TimeSpan timeout) { CleanUp(false, timeout); settingsApplied = false; } protected override Action GetOnViaCallback() { return this.onViaCallback; } void OnVia(Uri address) { Debug.Print("HostedTcpTransportManager.OnVia() address: " + address + " calling EnsureServiceAvailable()"); ServiceHostingEnvironment.EnsureServiceAvailable(address.LocalPath); } protected override void OnSelecting(TcpChannelListener channelListener) { if (settingsApplied) { return; } lock (ThisLock) { if (settingsApplied) { // Use the first one. return; } this.ApplyListenerSettings(channelListener); settingsApplied = true; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
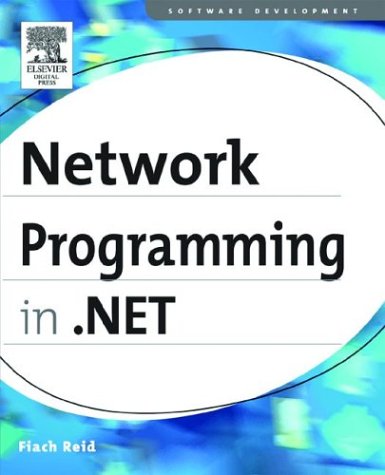
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MsmqReceiveParameters.cs
- ButtonChrome.cs
- UnmanagedMemoryStreamWrapper.cs
- DataGridViewRowEventArgs.cs
- SafeProcessHandle.cs
- ArrayElementGridEntry.cs
- EventSourceCreationData.cs
- DiscoveryRequestHandler.cs
- GcHandle.cs
- ReliabilityContractAttribute.cs
- ValidatedControlConverter.cs
- EventLogPermissionEntry.cs
- DetailsViewInsertedEventArgs.cs
- WindowsStreamSecurityElement.cs
- SrgsOneOf.cs
- FilterElement.cs
- FullTextLine.cs
- RuleDefinitions.cs
- ConcurrentQueue.cs
- BufferedOutputStream.cs
- NativeMethods.cs
- TextAdaptor.cs
- HtmlValidationSummaryAdapter.cs
- TreeNodeStyleCollection.cs
- RuntimeHandles.cs
- StringResourceManager.cs
- SelectionItemPattern.cs
- CompleteWizardStep.cs
- TreeBuilder.cs
- SqlException.cs
- DataSourceListEditor.cs
- ExceptionUtil.cs
- WorkflowMarkupSerializerMapping.cs
- DBSqlParserColumnCollection.cs
- SecurityHelper.cs
- SuppressMergeCheckAttribute.cs
- NameSpaceEvent.cs
- InfoCardConstants.cs
- _KerberosClient.cs
- TrackingDataItem.cs
- StopStoryboard.cs
- Crypto.cs
- XmlSecureResolver.cs
- CounterCreationDataCollection.cs
- RequestSecurityTokenForRemoteTokenFactory.cs
- ReadOnlyDictionary.cs
- FixedFindEngine.cs
- GPStream.cs
- PartialCachingAttribute.cs
- XmlSchemaComplexType.cs
- ResXFileRef.cs
- PrintDialog.cs
- PairComparer.cs
- FunctionUpdateCommand.cs
- HttpCachePolicyElement.cs
- ConstantProjectedSlot.cs
- Utils.cs
- FlowDocumentPageViewerAutomationPeer.cs
- DisplayNameAttribute.cs
- SqlClientMetaDataCollectionNames.cs
- SvcMapFile.cs
- HttpResponseBase.cs
- XmlSchemaAttribute.cs
- SetIterators.cs
- BaseParser.cs
- DescendantQuery.cs
- HtmlTableCell.cs
- LogEntrySerializationException.cs
- TableRow.cs
- URLString.cs
- X509Certificate2Collection.cs
- PartBasedPackageProperties.cs
- ToolStripItemImageRenderEventArgs.cs
- InlineCategoriesDocument.cs
- GeneralTransformCollection.cs
- DataSourceComponent.cs
- NetCodeGroup.cs
- MetadataFile.cs
- PassportPrincipal.cs
- IdentifierCreationService.cs
- XmlIgnoreAttribute.cs
- EntityDataSourceEntityTypeFilterItem.cs
- ZipIOBlockManager.cs
- CodeTypeParameterCollection.cs
- ScaleTransform.cs
- UserControlParser.cs
- Debug.cs
- ListControlStringCollectionEditor.cs
- ToolStripItemDesigner.cs
- CredentialCache.cs
- MatrixTransform3D.cs
- LinkedResourceCollection.cs
- COM2ColorConverter.cs
- RightsManagementInformation.cs
- OpCellTreeNode.cs
- SafeWaitHandle.cs
- TargetControlTypeCache.cs
- Identifier.cs
- HttpResponse.cs
- COMException.cs