Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / CommonUI / System / Drawing / Advanced / EncoderParameter.cs / 1305376 / EncoderParameter.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Drawing.Imaging { using System; using System.Text; using System.Diagnostics; using System.Drawing; using System.Runtime.InteropServices; using System.Drawing.Internal; ////// /// [StructLayout(LayoutKind.Sequential)] public sealed class EncoderParameter : IDisposable { [MarshalAs(UnmanagedType.Struct)] Guid parameterGuid; // GUID of the parameter int numberOfValues; // Number of the parameter values int parameterValueType; // Value type, like ValueTypeLONG etc. IntPtr parameterValue; // A pointer to the parameter values ///[To be supplied.] ///~EncoderParameter() { Dispose(false); } /// /// /// Gets/Sets the Encoder for the EncoderPameter. /// public Encoder Encoder { get { return new Encoder(parameterGuid); } set { parameterGuid = value.Guid; } } ////// /// Gets the EncoderParameterValueType object from the EncoderParameter. /// public EncoderParameterValueType Type { get { return (EncoderParameterValueType)parameterValueType; } } ////// /// Gets the EncoderParameterValueType object from the EncoderParameter. /// public EncoderParameterValueType ValueType { get { return (EncoderParameterValueType)parameterValueType; } } ////// /// Gets the NumberOfValues from the EncoderParameter. /// public int NumberOfValues { get { return numberOfValues; } } ////// /// public void Dispose() { Dispose(true); GC.KeepAlive(this); GC.SuppressFinalize(this); } void Dispose(bool disposing) { if (parameterValue != IntPtr.Zero) Marshal.FreeHGlobal(parameterValue); parameterValue = IntPtr.Zero; } ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, byte value) { parameterGuid = encoder.Guid; parameterValueType = (int)EncoderParameterValueType.ValueTypeByte; numberOfValues = 1; parameterValue = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(Byte))); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); Marshal.WriteByte(parameterValue, value); GC.KeepAlive(this); } ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, byte value, bool undefined) { parameterGuid = encoder.Guid; if (undefined == true) parameterValueType = (int)EncoderParameterValueType.ValueTypeUndefined; else parameterValueType = (int)EncoderParameterValueType.ValueTypeByte; numberOfValues = 1; parameterValue = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(Byte))); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); Marshal.WriteByte(parameterValue, value); GC.KeepAlive(this); } ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, short value) { parameterGuid = encoder.Guid; parameterValueType = (int)EncoderParameterValueType.ValueTypeShort; numberOfValues = 1; parameterValue = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(Int16))); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); Marshal.WriteInt16(parameterValue, value); GC.KeepAlive(this); } ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, long value) { parameterGuid = encoder.Guid; parameterValueType = (int)EncoderParameterValueType.ValueTypeLong; numberOfValues = 1; parameterValue = Marshal.AllocHGlobal(Marshal.SizeOf(typeof(Int32))); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); Marshal.WriteInt32(parameterValue, (int)value); GC.KeepAlive(this); } // Consider supporting a 'float' and converting to numerator/denominator ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, int numerator, int demoninator) { parameterGuid = encoder.Guid; parameterValueType = (int)EncoderParameterValueType.ValueTypeRational; numberOfValues = 1; int size = Marshal.SizeOf(typeof(Int32)); parameterValue = Marshal.AllocHGlobal(2*size); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); Marshal.WriteInt32(parameterValue, numerator); Marshal.WriteInt32(Add(parameterValue, size), demoninator); GC.KeepAlive(this); } // Consider supporting a 'float' and converting to numerator/denominator ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, long rangebegin, long rangeend) { parameterGuid = encoder.Guid; parameterValueType = (int)EncoderParameterValueType.ValueTypeLongRange; numberOfValues = 1; int size = Marshal.SizeOf(typeof(Int32)); parameterValue = Marshal.AllocHGlobal(2*size); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); Marshal.WriteInt32(parameterValue, (int)rangebegin); Marshal.WriteInt32(Add(parameterValue, size), (int)rangeend); GC.KeepAlive(this); } // Consider supporting a 'float' and converting to numerator/denominator ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, int numerator1, int demoninator1, int numerator2, int demoninator2) { parameterGuid = encoder.Guid; parameterValueType = (int)EncoderParameterValueType.ValueTypeRationalRange; numberOfValues = 1; int size = Marshal.SizeOf(typeof(Int32)); parameterValue = Marshal.AllocHGlobal(4*size); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); Marshal.WriteInt32(parameterValue, numerator1); Marshal.WriteInt32(Add(parameterValue, size), demoninator1); Marshal.WriteInt32(Add(parameterValue, 2*size), numerator2); Marshal.WriteInt32(Add(parameterValue, 3*size), demoninator2); GC.KeepAlive(this); } ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, string value) { parameterGuid = encoder.Guid; parameterValueType = (int)EncoderParameterValueType.ValueTypeAscii; numberOfValues = value.Length; parameterValue = Marshal.StringToHGlobalAnsi(value); GC.KeepAlive(this); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); } ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, byte[] value) { parameterGuid = encoder.Guid; parameterValueType = (int)EncoderParameterValueType.ValueTypeByte; numberOfValues = value.Length; parameterValue = Marshal.AllocHGlobal(numberOfValues); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); Marshal.Copy(value, 0, parameterValue, numberOfValues); GC.KeepAlive(this); } ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, byte[] value, bool undefined) { parameterGuid = encoder.Guid; if (undefined == true) parameterValueType = (int)EncoderParameterValueType.ValueTypeUndefined; else parameterValueType = (int)EncoderParameterValueType.ValueTypeByte; numberOfValues = value.Length; parameterValue = Marshal.AllocHGlobal(numberOfValues); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); Marshal.Copy(value, 0, parameterValue, numberOfValues); GC.KeepAlive(this); } ///[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, short[] value) { parameterGuid = encoder.Guid; parameterValueType = (int)EncoderParameterValueType.ValueTypeShort; numberOfValues = value.Length; int size = Marshal.SizeOf(typeof(short)); parameterValue = Marshal.AllocHGlobal(numberOfValues*size); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); Marshal.Copy(value, 0, parameterValue, numberOfValues); GC.KeepAlive(this); } ///[To be supplied.] ////// /// public unsafe EncoderParameter(Encoder encoder, long[] value) { parameterGuid = encoder.Guid; parameterValueType = (int)EncoderParameterValueType.ValueTypeLong; numberOfValues = value.Length; int size = Marshal.SizeOf(typeof(Int32)); parameterValue = Marshal.AllocHGlobal(numberOfValues*size); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); int* dest = (int*)parameterValue; fixed (long* source = value) { for (int i=0; i[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, int[] numerator, int[] denominator) { parameterGuid = encoder.Guid; if (numerator.Length != denominator.Length) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); parameterValueType = (int)EncoderParameterValueType.ValueTypeRational; numberOfValues = numerator.Length; int size = Marshal.SizeOf(typeof(Int32)); parameterValue = Marshal.AllocHGlobal(numberOfValues*2*size); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); for (int i=0; i[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, long[] rangebegin, long[] rangeend) { parameterGuid = encoder.Guid; if (rangebegin.Length != rangeend.Length) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); parameterValueType = (int)EncoderParameterValueType.ValueTypeLongRange; numberOfValues = rangebegin.Length; int size = Marshal.SizeOf(typeof(Int32)); parameterValue = Marshal.AllocHGlobal(numberOfValues*2*size); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); for (int i=0; i[To be supplied.] ////// /// public EncoderParameter(Encoder encoder, int[] numerator1, int[] denominator1, int[] numerator2, int[] denominator2) { parameterGuid = encoder.Guid; if (numerator1.Length != denominator1.Length || numerator1.Length != denominator2.Length || denominator1.Length != denominator2.Length) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.InvalidParameter); parameterValueType = (int)EncoderParameterValueType.ValueTypeRationalRange; numberOfValues = numerator1.Length; int size = Marshal.SizeOf(typeof(Int32)); parameterValue = Marshal.AllocHGlobal(numberOfValues*4*size); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); for (int i=0; i[To be supplied.] ////// /// [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Naming", "CA1704:IdentifiersShouldBeSpelledCorrectly")] public EncoderParameter(Encoder encoder, int NumberOfValues, int Type, int Value) { int size; switch((EncoderParameterValueType)Type) { case EncoderParameterValueType.ValueTypeByte: case EncoderParameterValueType.ValueTypeAscii: size = 1; break; case EncoderParameterValueType.ValueTypeShort: size = 2; break; case EncoderParameterValueType.ValueTypeLong: size = 4; break; case EncoderParameterValueType.ValueTypeRational: case EncoderParameterValueType.ValueTypeLongRange: size = 2*4; break; case EncoderParameterValueType.ValueTypeUndefined: size = 1; break; case EncoderParameterValueType.ValueTypeRationalRange: size = 2*2*4; break; default: throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.WrongState); } int bytes = size*NumberOfValues; parameterValue = Marshal.AllocHGlobal(bytes); if (parameterValue == IntPtr.Zero) throw SafeNativeMethods.Gdip.StatusException(SafeNativeMethods.Gdip.OutOfMemory); for (int i=0; i[To be supplied.] ///
Link Menu
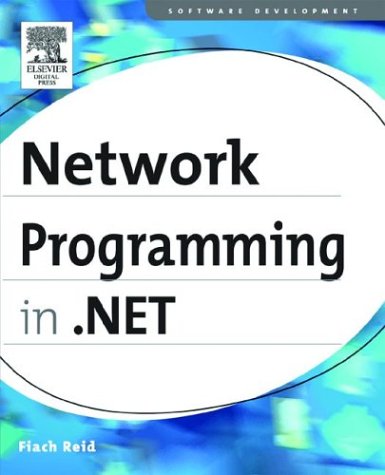
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- OdbcConnectionFactory.cs
- XmlObjectSerializerContext.cs
- HyperLinkColumn.cs
- EntityDataSourceValidationException.cs
- MdiWindowListStrip.cs
- GridViewDeletedEventArgs.cs
- WS2007FederationHttpBinding.cs
- RenderData.cs
- ComponentDispatcher.cs
- FormViewInsertEventArgs.cs
- HMACSHA256.cs
- DataTrigger.cs
- ColorEditor.cs
- DataTableTypeConverter.cs
- MaskedTextBoxDesigner.cs
- Size3DValueSerializer.cs
- QilGenerator.cs
- StylusShape.cs
- AbstractDataSvcMapFileLoader.cs
- StreamInfo.cs
- DataSourceXmlSerializationAttribute.cs
- ToolboxComponentsCreatingEventArgs.cs
- TableRowGroup.cs
- DSACryptoServiceProvider.cs
- AsyncPostBackErrorEventArgs.cs
- GeneralTransform3D.cs
- CustomError.cs
- MissingFieldException.cs
- DataListDesigner.cs
- InfoCardKeyedHashAlgorithm.cs
- ObjectReferenceStack.cs
- InstanceHandleConflictException.cs
- OracleConnectionFactory.cs
- HtmlContainerControl.cs
- SqlCharStream.cs
- SubqueryRules.cs
- PrintDialog.cs
- ImageCollectionCodeDomSerializer.cs
- SqlDuplicator.cs
- Int32KeyFrameCollection.cs
- TextServicesCompartmentContext.cs
- SQLBytes.cs
- FontInfo.cs
- BitmapEffectDrawingContent.cs
- ScriptControlManager.cs
- CharConverter.cs
- CallbackValidator.cs
- EntityPropertyMappingAttribute.cs
- _KerberosClient.cs
- validation.cs
- FontStretchConverter.cs
- TableHeaderCell.cs
- Rotation3DAnimationBase.cs
- ScriptControl.cs
- RemotingServices.cs
- WebControlParameterProxy.cs
- TripleDESCryptoServiceProvider.cs
- CodeMemberProperty.cs
- DocumentPage.cs
- StringInfo.cs
- SHA384Cng.cs
- WebPageTraceListener.cs
- LeftCellWrapper.cs
- WebResourceAttribute.cs
- StateDesignerConnector.cs
- MemoryPressure.cs
- DnsPermission.cs
- BasicExpressionVisitor.cs
- ResourcesChangeInfo.cs
- SelectionItemPattern.cs
- IntranetCredentialPolicy.cs
- XmlSerializationWriter.cs
- SectionRecord.cs
- Trace.cs
- MediaContext.cs
- CodeTypeParameterCollection.cs
- OracleFactory.cs
- DictionarySectionHandler.cs
- safex509handles.cs
- HttpCacheParams.cs
- ControlCollection.cs
- ResourceExpression.cs
- CompilerParameters.cs
- DefaultBinder.cs
- LateBoundBitmapDecoder.cs
- RectKeyFrameCollection.cs
- XmlSerializerAssemblyAttribute.cs
- MetadataItemSerializer.cs
- FunctionCommandText.cs
- SortedSet.cs
- InstallerTypeAttribute.cs
- BindingManagerDataErrorEventArgs.cs
- Certificate.cs
- StringValidatorAttribute.cs
- CodeChecksumPragma.cs
- ProcessHostConfigUtils.cs
- HtmlInputReset.cs
- DesigntimeLicenseContextSerializer.cs
- AbandonedMutexException.cs
- MeasureData.cs