Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Configuration / System / Configuration / ValidatorUtils.cs / 1305376 / ValidatorUtils.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System; using System.Collections; using System.IO; using System.Reflection; using System.Security.Permissions; using System.Xml; using System.Collections.Specialized; using System.Globalization; using System.ComponentModel; using System.Security; using System.Text; using System.Text.RegularExpressions; namespace System.Configuration { internal static class ValidatorUtils { public static void HelperParamValidation(object value, Type allowedType) { if (value == null) { return; } if (value.GetType() != allowedType) { throw new ArgumentException(SR.GetString(SR.Validator_value_type_invalid), String.Empty); } } public static void ValidateScalar(T value, T min, T max, T resolution, bool exclusiveRange) where T : IComparable { ValidateRangeImpl(value, min, max, exclusiveRange); // Validate the resolution ValidateResolution(resolution.ToString(), Convert.ToInt64(value, CultureInfo.InvariantCulture), Convert.ToInt64(resolution, CultureInfo.InvariantCulture)); } private static void ValidateRangeImpl ( T value, T min, T max, bool exclusiveRange ) where T : IComparable { IComparable itfValue = (IComparable )value; IComparable itfMax = (IComparable )max; bool valueIsInRange = false; // Check min range if ( itfValue.CompareTo( min ) >= 0 ) { // TRUE: value >= min valueIsInRange = true; } if ( valueIsInRange && ( itfValue.CompareTo( max ) > 0 ) ) { // TRUE: value > max valueIsInRange = false; } // Throw range validation error if ( !( valueIsInRange ^ exclusiveRange ) ) { string error = null; // First group of errors - the min and max range are the same. i.e. the valid value must be the same/equal to the min(max) if ( min.Equals( max ) ) { if ( exclusiveRange ) { // Valid values are outside of range. I.e has to be different then min( or max ) error = SR.GetString( SR.Validation_scalar_range_violation_not_different ); } else { // Valid values are inside the range. I.e. has to be equal to min ( or max ) error = SR.GetString( SR.Validation_scalar_range_violation_not_equal ); } } // Second group of errors: min != max. I.e. its a range else { if ( exclusiveRange ) { // Valid values are outside of range. error = SR.GetString( SR.Validation_scalar_range_violation_not_outside_range ); } else { // Valid values are inside the range. I.e. has to be equal to min ( or max ) error = SR.GetString( SR.Validation_scalar_range_violation_not_in_range ); } } throw new ArgumentException( String.Format( CultureInfo.InvariantCulture, error, min.ToString(), max.ToString() ) ); } } private static void ValidateResolution(string resolutionAsString, long value, long resolution) { Debug.Assert(resolution > 0, "resolution > 0"); if ((value % resolution) != 0) { throw new ArgumentException(SR.GetString(SR.Validator_scalar_resolution_violation, resolutionAsString)); } } public static void ValidateScalar(TimeSpan value, TimeSpan min, TimeSpan max, long resolutionInSeconds, bool exclusiveRange) { ValidateRangeImpl(value, min, max, exclusiveRange); // Validate the resolution if (resolutionInSeconds > 0) { ValidateResolution(TimeSpan.FromSeconds( resolutionInSeconds ).ToString(), value.Ticks, resolutionInSeconds * TimeSpan.TicksPerSecond); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
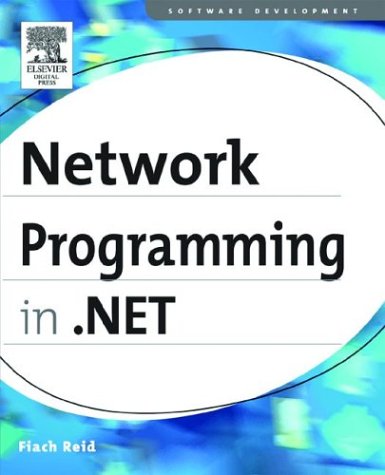
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- BufferModeSettings.cs
- ImageDesigner.cs
- XmlImplementation.cs
- QuaternionAnimation.cs
- UIElementIsland.cs
- PropertyEntry.cs
- RootBrowserWindowAutomationPeer.cs
- PrintingPermission.cs
- ObjectStateEntry.cs
- RoleGroup.cs
- CharacterBufferReference.cs
- Win32.cs
- CodeTypeReferenceExpression.cs
- RuleCache.cs
- HelpKeywordAttribute.cs
- Oid.cs
- LassoHelper.cs
- RadioButton.cs
- Accessible.cs
- MetafileHeader.cs
- VisualStyleElement.cs
- DataBoundControlActionList.cs
- tibetanshape.cs
- PriorityItem.cs
- _Semaphore.cs
- DeviceContexts.cs
- MenuRendererClassic.cs
- ListViewInsertEventArgs.cs
- WebPartMovingEventArgs.cs
- CurrencyManager.cs
- SoapFormatter.cs
- FieldCollectionEditor.cs
- PropertyCollection.cs
- HttpAsyncResult.cs
- BaseCodePageEncoding.cs
- DataGridDetailsPresenterAutomationPeer.cs
- FixedSOMImage.cs
- OneWayBindingElement.cs
- Int32AnimationBase.cs
- ControlValuePropertyAttribute.cs
- BufferBuilder.cs
- SqlFlattener.cs
- SiteIdentityPermission.cs
- NativeMethods.cs
- InteropEnvironment.cs
- HttpRuntimeSection.cs
- ColorKeyFrameCollection.cs
- TextEffectResolver.cs
- LogicalTreeHelper.cs
- ImageAutomationPeer.cs
- TableAdapterManagerMethodGenerator.cs
- DropAnimation.xaml.cs
- TraceHandlerErrorFormatter.cs
- indexingfiltermarshaler.cs
- QueryCacheManager.cs
- EventWaitHandle.cs
- RC2CryptoServiceProvider.cs
- ResourceDescriptionAttribute.cs
- PageVisual.cs
- RewritingValidator.cs
- OdbcConnectionPoolProviderInfo.cs
- DataServiceContext.cs
- _IPv4Address.cs
- Util.cs
- BufferedResponseStream.cs
- FormViewInsertEventArgs.cs
- DefaultAutoFieldGenerator.cs
- LineBreak.cs
- PlainXmlWriter.cs
- EditorOptionAttribute.cs
- ErrorHandler.cs
- InputMethodStateChangeEventArgs.cs
- AttachedAnnotationChangedEventArgs.cs
- ListComponentEditorPage.cs
- WebPartConnectVerb.cs
- ImpersonationOption.cs
- WSHttpBindingElement.cs
- DateBoldEvent.cs
- CommandManager.cs
- AutoSizeToolBoxItem.cs
- DynamicExpression.cs
- Base64Stream.cs
- BindingOperations.cs
- ComponentEditorPage.cs
- HashCodeCombiner.cs
- CacheMode.cs
- ContextStaticAttribute.cs
- OpenTypeCommon.cs
- OnOperation.cs
- EpmSyndicationContentSerializer.cs
- CodeIdentifier.cs
- Activity.cs
- StylusLogic.cs
- SystemIPGlobalStatistics.cs
- InfoCardRSAPKCS1KeyExchangeFormatter.cs
- SAPIEngineTypes.cs
- TextEditorThreadLocalStore.cs
- ColumnMapProcessor.cs
- XmlNamespaceMapping.cs
- DrawToolTipEventArgs.cs