Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Core / System / Security / Cryptography / X509Certificates / AuthenticodeSignatureInformation.cs / 1305376 / AuthenticodeSignatureInformation.cs
// ==++== // // Copyright (c) Microsoft Corporation. All rights reserved. // // ==--== using System; using System.Diagnostics; using System.Runtime.InteropServices; using System.Security.Cryptography; using System.Security.Permissions; namespace System.Security.Cryptography.X509Certificates { ////// Details about the Authenticode signature of a manifest /// [System.Security.Permissions.HostProtection(MayLeakOnAbort = true)] public sealed class AuthenticodeSignatureInformation { private string m_description; private Uri m_descriptionUrl; private CapiNative.AlgorithmId m_hashAlgorithmId; private X509Chain m_signatureChain; private TimestampInformation m_timestamp; private SignatureVerificationResult m_verificationResult; private X509Certificate2 m_signingCertificate; internal AuthenticodeSignatureInformation(X509Native.AXL_AUTHENTICODE_SIGNER_INFO signer, X509Chain signatureChain, TimestampInformation timestamp) { m_verificationResult = (SignatureVerificationResult)signer.dwError; m_hashAlgorithmId = signer.algHash; if (signer.pwszDescription != IntPtr.Zero) { m_description = Marshal.PtrToStringUni(signer.pwszDescription); } if (signer.pwszDescriptionUrl != IntPtr.Zero) { string descriptionUrl = Marshal.PtrToStringUni(signer.pwszDescriptionUrl); Uri.TryCreate(descriptionUrl, UriKind.RelativeOrAbsolute, out m_descriptionUrl); } m_signatureChain = signatureChain; // If there was a timestamp, and it was not valid we need to invalidate the entire Authenticode // signature as well, since we cannot assume that the signature would have verified without // the timestamp. if (timestamp != null && timestamp.VerificationResult != SignatureVerificationResult.MissingSignature) { if (timestamp.IsValid) { m_timestamp = timestamp; } else { m_verificationResult = SignatureVerificationResult.InvalidTimestamp; } } else { m_timestamp = null; } } ////// Create an Authenticode signature information for a signature which is not valid /// internal AuthenticodeSignatureInformation(SignatureVerificationResult error) { Debug.Assert(error != SignatureVerificationResult.Valid); m_verificationResult = error; } ////// Description of the signing certificate /// public string Description { get { return m_description; } } ////// Description URL of the signing certificate /// public Uri DescriptionUrl { get { return m_descriptionUrl; } } ////// Hash algorithm the signature was computed with /// public string HashAlgorithm { get { return CapiNative.GetAlgorithmName(m_hashAlgorithmId); } } ////// HRESULT from verifying the signature /// public int HResult { get { return CapiNative.HResultForVerificationResult(m_verificationResult); } } ////// X509 chain used to verify the Authenticode signature /// public X509Chain SignatureChain { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { return m_signatureChain; } } ////// Certificate the manifest was signed with /// public X509Certificate2 SigningCertificate { [StorePermission(SecurityAction.Demand, OpenStore = true, EnumerateCertificates = true)] get { if (m_signingCertificate == null && SignatureChain != null) { Debug.Assert(SignatureChain.ChainElements.Count > 0, "SignatureChain.ChainElements.Count > 0"); m_signingCertificate = SignatureChain.ChainElements[0].Certificate; } return m_signingCertificate; } } ////// Timestamp, if any, applied to the Authenticode signature /// ////// Note that this is only available in the trusted publisher case /// public TimestampInformation Timestamp { get { return m_timestamp; } } ////// Trustworthiness of the Authenticode signature /// public TrustStatus TrustStatus { get { switch (VerificationResult) { case SignatureVerificationResult.Valid: return TrustStatus.Trusted; case SignatureVerificationResult.CertificateNotExplicitlyTrusted: return TrustStatus.KnownIdentity; case SignatureVerificationResult.CertificateExplicitlyDistrusted: return TrustStatus.Untrusted; default: return TrustStatus.UnknownIdentity; } } } ////// Result of verifying the Authenticode signature /// public SignatureVerificationResult VerificationResult { get { return m_verificationResult; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
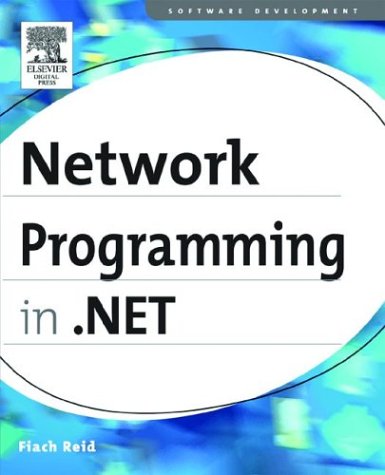
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- DataContext.cs
- Transform3DGroup.cs
- ChildTable.cs
- DbConnectionPool.cs
- ExpressionBinding.cs
- XsltLoader.cs
- HttpCapabilitiesBase.cs
- DecimalAnimation.cs
- DataSourceXmlSerializationAttribute.cs
- HandlerFactoryCache.cs
- NetCodeGroup.cs
- ConnectionManagementElementCollection.cs
- BitmapData.cs
- SqlRecordBuffer.cs
- QilName.cs
- CellTreeNodeVisitors.cs
- ChangeDirector.cs
- unsafeIndexingFilterStream.cs
- ExpressionStringBuilder.cs
- DurationConverter.cs
- ApplicationFileParser.cs
- SerializationAttributes.cs
- autovalidator.cs
- ObjectAnimationBase.cs
- Double.cs
- XMLUtil.cs
- Array.cs
- ValidatorCompatibilityHelper.cs
- Privilege.cs
- HelloMessage11.cs
- __Filters.cs
- TextTrailingCharacterEllipsis.cs
- SqlServer2KCompatibilityCheck.cs
- TableLayoutPanelCellPosition.cs
- Model3DGroup.cs
- SoapSchemaImporter.cs
- GroupByQueryOperator.cs
- XmlHierarchicalDataSourceView.cs
- DataListItemCollection.cs
- StackSpiller.Temps.cs
- ActivityScheduledRecord.cs
- ResourceDefaultValueAttribute.cs
- SQLBytesStorage.cs
- CursorConverter.cs
- Matrix3DStack.cs
- InstanceDataCollection.cs
- ModelItemExtensions.cs
- coordinatorscratchpad.cs
- DrawingAttributeSerializer.cs
- EventSourceCreationData.cs
- EntityDescriptor.cs
- XmlComplianceUtil.cs
- FunctionParameter.cs
- HostProtectionException.cs
- ValidationErrorCollection.cs
- ViewCellSlot.cs
- Paragraph.cs
- AccessorTable.cs
- DataGridState.cs
- ReferencedAssemblyResolver.cs
- UIElement3DAutomationPeer.cs
- Attributes.cs
- ControlLocalizer.cs
- TextDecorationUnitValidation.cs
- StateInitialization.cs
- XmlEventCache.cs
- CheckBoxRenderer.cs
- WebServiceMethodData.cs
- CalculatedColumn.cs
- DataGridViewColumnTypeEditor.cs
- FileDialogPermission.cs
- ParagraphResult.cs
- DictionaryItemsCollection.cs
- HtmlInputSubmit.cs
- UnaryOperationBinder.cs
- RuntimeHelpers.cs
- ProfileSection.cs
- InputElement.cs
- StreamWriter.cs
- FilteredDataSetHelper.cs
- OpacityConverter.cs
- DriveInfo.cs
- Vars.cs
- SplashScreen.cs
- _Connection.cs
- SqlBulkCopyColumnMappingCollection.cs
- RelationshipEndMember.cs
- CodeNamespace.cs
- Compiler.cs
- ConfigurationLoader.cs
- HandleRef.cs
- CodeGenHelper.cs
- ToolStripPanelRow.cs
- ExpandableObjectConverter.cs
- Vector3DConverter.cs
- InstanceOwnerException.cs
- VisualStyleElement.cs
- CollectionConverter.cs
- TreeView.cs
- ExpressionEditorAttribute.cs