Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataEntity / System / Data / EntityModel / SchemaObjectModel / EntityKeyElement.cs / 1305376 / EntityKeyElement.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // // // @owner [....] // @backupOwner [....] //--------------------------------------------------------------------- using System; using System.Collections; using System.Collections.Generic; using System.Collections.Specialized; using System.Diagnostics; using System.Xml; using System.Data; using System.Data.Metadata.Edm; using System.Data.Entity; namespace System.Data.EntityModel.SchemaObjectModel { ////// Represents an Key element in an EntityType element. /// internal sealed class EntityKeyElement : SchemaElement { private List_keyProperties; /// /// Constructs an EntityContainerAssociationSetEnd /// /// Reference to the schema element. public EntityKeyElement( SchemaEntityType parentElement ) : base( parentElement ) { } public IListKeyProperties { get { if (_keyProperties == null) { _keyProperties = new List (); } return _keyProperties; } } protected override bool HandleAttribute(XmlReader reader) { return false; } protected override bool HandleElement(XmlReader reader) { if (base.HandleElement(reader)) { return true; } else if (CanHandleElement(reader, XmlConstants.PropertyRef)) { HandlePropertyRefElement(reader); return true; } return false; } /// /// /// /// private void HandlePropertyRefElement(XmlReader reader) { PropertyRefElement property = new PropertyRefElement((SchemaEntityType)ParentElement); property.Parse(reader); this.KeyProperties.Add(property); } ////// Used during the resolve phase to resolve the type name to the object that represents that type /// internal override void ResolveTopLevelNames() { Debug.Assert(_keyProperties != null, "xsd should have verified that there should be atleast one property ref element"); foreach (PropertyRefElement property in _keyProperties) { if (!property.ResolveNames((SchemaEntityType)this.ParentElement)) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyNoProperty(this.ParentElement.FQName, property.Name)); } } } ////// Validate all the key properties /// internal override void Validate() { Debug.Assert(_keyProperties != null, "xsd should have verified that there should be atleast one property ref element"); DictionarypropertyLookUp = new Dictionary (StringComparer.Ordinal); foreach (PropertyRefElement keyProperty in _keyProperties) { StructuredProperty property = keyProperty.Property; Debug.Assert(property != null, "This should never be null, since if we were not able to resolve, we should have never reached to this point"); if (propertyLookUp.ContainsKey(property.Name)) { AddError(ErrorCode.DuplicatePropertySpecifiedInEntityKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.DuplicatePropertyNameSpecifiedInEntityKey(this.ParentElement.FQName, property.Name)); continue; } propertyLookUp.Add(property.Name, keyProperty); if (property.Nullable) { AddError(ErrorCode.InvalidKey, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.InvalidKeyNullablePart(property.Name, this.ParentElement.Name)); } // currently we only support key properties of scalar type if ((!(property.Type is ScalarType)) || (property.CollectionKind != CollectionKind.None)) { AddError(ErrorCode.EntityKeyMustBeScalar, EdmSchemaErrorSeverity.Error, System.Data.Entity.Strings.EntityKeyMustBeScalar(property.Name, this.ParentElement.Name)); continue; } Debug.Assert(property.TypeUsage != null, "For scalar type, typeusage must be initialized"); // Bug 484200: Binary type key properties are currently not supported. PrimitiveTypeKind kind = ((PrimitiveType)property.TypeUsage.EdmType).PrimitiveTypeKind; if (kind == PrimitiveTypeKind.Binary) { if (Schema.DataModel == SchemaDataModelOption.EntityDataModel) { // Binary keys are only supported for V2.0 CSDL if (Schema.SchemaVersion < XmlConstants.EdmVersionForV2) { AddError(ErrorCode.BinaryEntityKeyCurrentlyNotSupported, EdmSchemaErrorSeverity.Error, Strings.EntityKeyTypeCurrentlyNotSupported(property.Name, this.ParentElement.FQName, kind)); } } else { Debug.Assert(SchemaDataModelOption.ProviderDataModel == Schema.DataModel, "Invalid DataModel encountered"); // Binary keys are only supported for V2.0 SSDL if (Schema.SchemaVersion < XmlConstants.StoreVersionForV2) { AddError(ErrorCode.BinaryEntityKeyCurrentlyNotSupported, EdmSchemaErrorSeverity.Error, Strings.EntityKeyTypeCurrentlyNotSupportedInSSDL(property.Name, this.ParentElement.FQName, property.TypeUsage.EdmType.Name, property.TypeUsage.EdmType.BaseType.FullName, kind)); } } } } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
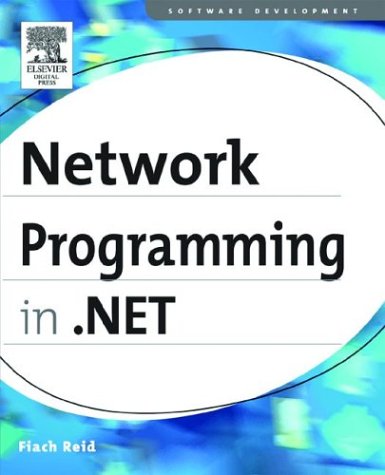
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- LogPolicy.cs
- IUnknownConstantAttribute.cs
- BitStream.cs
- RightsManagementEncryptionTransform.cs
- RoleManagerEventArgs.cs
- ClientApiGenerator.cs
- SpellerError.cs
- VerticalAlignConverter.cs
- XmlDictionaryString.cs
- XmlUrlResolver.cs
- DeferrableContentConverter.cs
- ArcSegment.cs
- PartialClassGenerationTaskInternal.cs
- PassportAuthenticationEventArgs.cs
- NavigationWindow.cs
- COM2ColorConverter.cs
- ScalarConstant.cs
- DirectoryInfo.cs
- CodeNamespace.cs
- XmlExpressionDumper.cs
- WebBrowserEvent.cs
- Themes.cs
- ToolStripDropDownDesigner.cs
- SynchronizedDispatch.cs
- DbUpdateCommandTree.cs
- UInt16Storage.cs
- DetailsViewUpdatedEventArgs.cs
- Propagator.cs
- AuthenticationSection.cs
- BindingNavigatorDesigner.cs
- UpDownEvent.cs
- GraphicsState.cs
- EntityChangedParams.cs
- GraphicsContainer.cs
- SqlUDTStorage.cs
- SparseMemoryStream.cs
- TextServicesDisplayAttribute.cs
- RangeValueProviderWrapper.cs
- ResourceExpressionBuilder.cs
- PropertyGroupDescription.cs
- OleDbException.cs
- SoapAttributeOverrides.cs
- FullTextLine.cs
- ProgressBar.cs
- XmlNamespaceManager.cs
- IncomingWebRequestContext.cs
- DropSource.cs
- WmlImageAdapter.cs
- mediaclock.cs
- XmlSignatureProperties.cs
- BamlBinaryWriter.cs
- PictureBox.cs
- AccessText.cs
- StorageMappingItemCollection.cs
- SharedPersonalizationStateInfo.cs
- SqlGenericUtil.cs
- DataTableNewRowEvent.cs
- XmlSchemaComplexContentRestriction.cs
- ToolStripContentPanel.cs
- SimpleBitVector32.cs
- LineSegment.cs
- Margins.cs
- RequestSecurityTokenResponseCollection.cs
- ConnectionStringsSection.cs
- IDReferencePropertyAttribute.cs
- ClonableStack.cs
- ResolveNameEventArgs.cs
- FragmentQueryKB.cs
- DataServiceRequestException.cs
- Avt.cs
- ListBoxAutomationPeer.cs
- ObjectListCommandsPage.cs
- ResourceCategoryAttribute.cs
- AutomationAttributeInfo.cs
- SchemaManager.cs
- PageContentCollection.cs
- DataSourceView.cs
- FormView.cs
- TemplateKeyConverter.cs
- SqlClientPermission.cs
- EdmProviderManifest.cs
- ResourceType.cs
- wmiprovider.cs
- AuthenticationManager.cs
- _ScatterGatherBuffers.cs
- Color.cs
- WeakReference.cs
- Itemizer.cs
- DataControlHelper.cs
- Message.cs
- DictionaryBase.cs
- TextOutput.cs
- CheckBoxBaseAdapter.cs
- MultiByteCodec.cs
- TagMapCollection.cs
- ListMarkerSourceInfo.cs
- DataSourceXmlSerializationAttribute.cs
- SqlMethods.cs
- DbConnectionPoolOptions.cs
- OdbcParameterCollection.cs