Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / DataWeb / Client / System / Data / Services / Client / ProjectionPath.cs / 1305376 / ProjectionPath.cs
//---------------------------------------------------------------------- //// Copyright (c) Microsoft Corporation. All rights reserved. // //// Provides a class to represent an annotated path of segments // (each of which is a step in the parsed tree). // //--------------------------------------------------------------------- namespace System.Data.Services.Client { #region Namespaces. using System.Collections.Generic; using System.Diagnostics; using System.Linq.Expressions; using System.Text; #endregion Namespaces. ///Use this class to represent an annotated list of path segments. [DebuggerDisplay("{ToString()}")] internal class ProjectionPath : List{ #region Constructors. /// Initializes a new internal ProjectionPath() : base() { } ///instance. Initializes a new /// Root parameter for this path. /// Expression to get the expected root type in the target tree. /// Expression for the root entry. internal ProjectionPath(ParameterExpression root, Expression expectedRootType, Expression rootEntry) : base() { this.Root = root; this.RootEntry = rootEntry; this.ExpectedRootType = expectedRootType; } ///instance. Initializes a new /// Root parameter for this path. /// Expression to get the expected root type in the target tree. /// Expression for the root entry. /// Member to initialize the path with. internal ProjectionPath(ParameterExpression root, Expression expectedRootType, Expression rootEntry, IEnumerableinstance. members) : this(root, expectedRootType, rootEntry) { Debug.Assert(members != null, "members != null"); foreach (Expression member in members) { this.Add(new ProjectionPathSegment(this, ((MemberExpression)member).Member.Name, member.Type)); } } #endregion Constructors. #region Internal properties. /// Parameter expression in the source tree. internal ParameterExpression Root { get; private set; } ///Expression to get the entry for internal Expression RootEntry { get; private set; } ///in the target tree. Expression to get the expected root type in the target tree. internal Expression ExpectedRootType { get; private set; } #endregion Internal properties. #region Methods. ///Provides a string representation of this object. ///A string representation of this object, suitable for debugging. public override string ToString() { StringBuilder builder = new StringBuilder(); builder.Append(this.Root.ToString()); builder.Append("->"); for (int i = 0; i < this.Count; i++) { if (i > 0) { builder.Append('.'); } builder.Append(this[i].Member == null ? "*" : this[i].Member); } return builder.ToString(); } #endregion Methods. } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
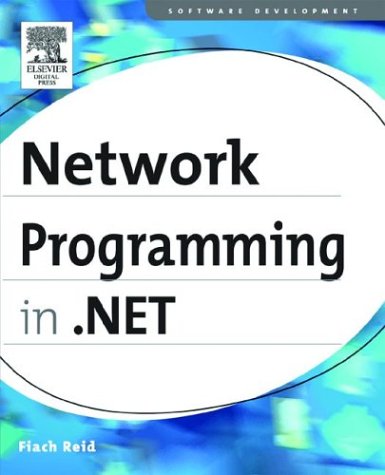
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- FixedSOMGroup.cs
- LinkClickEvent.cs
- SettingsSavedEventArgs.cs
- InstanceCreationEditor.cs
- ObjectDataSource.cs
- WorkflowServiceBehavior.cs
- AutoResetEvent.cs
- RedirectionProxy.cs
- TagPrefixCollection.cs
- UniformGrid.cs
- CategoryAttribute.cs
- _Rfc2616CacheValidators.cs
- WaitHandle.cs
- ActivityExecutorOperation.cs
- EdmEntityTypeAttribute.cs
- TemplateControlCodeDomTreeGenerator.cs
- Table.cs
- OdbcEnvironment.cs
- DeferredTextReference.cs
- HostedNamedPipeTransportManager.cs
- PropertyConverter.cs
- ProcessHostServerConfig.cs
- DataObjectPastingEventArgs.cs
- RightNameExpirationInfoPair.cs
- MDIClient.cs
- XmlSchemaNotation.cs
- LinqDataSource.cs
- LostFocusEventManager.cs
- DataGridRow.cs
- Int64Storage.cs
- EndEvent.cs
- EqualityComparer.cs
- CultureTableRecord.cs
- baseaxisquery.cs
- IdentityReference.cs
- Token.cs
- AutoResetEvent.cs
- XmlSchemaImport.cs
- SHA512CryptoServiceProvider.cs
- OracleTransaction.cs
- PasswordDeriveBytes.cs
- DoubleAnimationClockResource.cs
- IPEndPoint.cs
- ContainerUIElement3D.cs
- DoubleAnimationUsingKeyFrames.cs
- HttpHandlersSection.cs
- ColumnProvider.cs
- CacheEntry.cs
- ConfigXmlWhitespace.cs
- TreeNodeCollection.cs
- SqlXml.cs
- XamlPoint3DCollectionSerializer.cs
- WaveHeader.cs
- IdleTimeoutMonitor.cs
- ToolStripPanelDesigner.cs
- ChannelManagerHelpers.cs
- CodeNamespaceImport.cs
- MultiView.cs
- XmlnsDefinitionAttribute.cs
- ColumnProvider.cs
- WindowsTreeView.cs
- ObjectTag.cs
- CompressionTransform.cs
- GenericIdentity.cs
- ToolStripOverflowButton.cs
- WindowsListViewGroupHelper.cs
- DataRowCollection.cs
- HttpListenerRequest.cs
- ExpressionEditorAttribute.cs
- CodeChecksumPragma.cs
- ArrayMergeHelper.cs
- HttpResponseHeader.cs
- HttpServerUtilityBase.cs
- ExpressionsCollectionConverter.cs
- PointConverter.cs
- XPathArrayIterator.cs
- FontCollection.cs
- TransactionScopeDesigner.cs
- FileVersionInfo.cs
- CustomSignedXml.cs
- SQLByte.cs
- OutputScopeManager.cs
- ThrowHelper.cs
- Missing.cs
- DataConnectionHelper.cs
- DataGridViewAutoSizeColumnModeEventArgs.cs
- EntitySet.cs
- IdentityReference.cs
- OptimalTextSource.cs
- SecurityUtils.cs
- HttpHeaderCollection.cs
- ToolboxComponentsCreatingEventArgs.cs
- IssuanceLicense.cs
- Timer.cs
- SymmetricSecurityProtocolFactory.cs
- XmlDocument.cs
- ResponseStream.cs
- Exceptions.cs
- UpdateCompiler.cs
- WebPartsSection.cs