Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Net / System / Net / DnsEndPoint.cs / 1305376 / DnsEndPoint.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Net.Sockets; namespace System.Net { public class DnsEndPoint : EndPoint { private string m_Host; private int m_Port; private AddressFamily m_Family; public DnsEndPoint(string host, int port) : this(host, port, AddressFamily.Unspecified) {} public DnsEndPoint(string host, int port, AddressFamily addressFamily) { if(host == null) { throw new ArgumentNullException("host"); } if (String.IsNullOrEmpty(host)) { throw new ArgumentException(SR.GetString(SR.net_emptystringcall, "host")); } if(port < IPEndPoint.MinPort || port > IPEndPoint.MaxPort) { throw new ArgumentOutOfRangeException("port"); } if (addressFamily != AddressFamily.InterNetwork && addressFamily != AddressFamily.InterNetworkV6 && addressFamily != AddressFamily.Unspecified) { throw new ArgumentException(SR.GetString(SR.net_sockets_invalid_optionValue_all), "addressFamily"); } m_Host = host; m_Port = port; m_Family = addressFamily; } public override bool Equals(object comparand) { DnsEndPoint dnsComparand = comparand as DnsEndPoint; if (dnsComparand == null) return false; return (m_Family == dnsComparand.m_Family && m_Port == dnsComparand.m_Port && m_Host == dnsComparand.m_Host); } public override int GetHashCode() { return StringComparer.InvariantCultureIgnoreCase.GetHashCode(ToString()); } public override string ToString() { return m_Family + "/" + m_Host + ":" + m_Port; } public string Host { get { return m_Host; } } public override AddressFamily AddressFamily { get { return m_Family; } } public int Port { get { return m_Port; } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
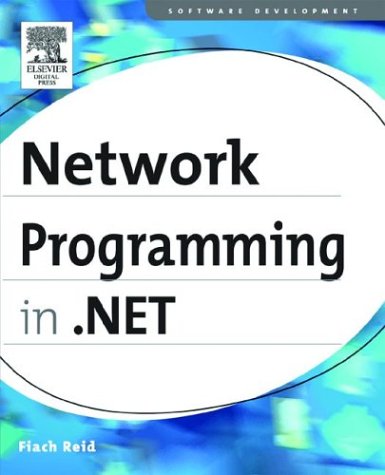
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- XamlWriter.cs
- DuplicateDetector.cs
- X509CertificateChain.cs
- FamilyCollection.cs
- MaskPropertyEditor.cs
- CutCopyPasteHelper.cs
- DataGridRowHeaderAutomationPeer.cs
- HostingPreferredMapPath.cs
- DateTimeFormat.cs
- Padding.cs
- InternalControlCollection.cs
- TabRenderer.cs
- RedirectionProxy.cs
- RequestCachingSection.cs
- AttributeSetAction.cs
- WindowCollection.cs
- UrlRoutingHandler.cs
- SoapFault.cs
- SignedXml.cs
- dtdvalidator.cs
- ALinqExpressionVisitor.cs
- AuthenticationModuleElementCollection.cs
- FrameworkContentElementAutomationPeer.cs
- DependencyPropertyValueSerializer.cs
- RadioButtonRenderer.cs
- Underline.cs
- LiteralDesigner.cs
- SqlRowUpdatedEvent.cs
- WebHttpDispatchOperationSelector.cs
- QueryResults.cs
- GeneralTransform3DGroup.cs
- EmptyCollection.cs
- EtwTrace.cs
- SortFieldComparer.cs
- StringCollection.cs
- AsyncWaitHandle.cs
- PlanCompilerUtil.cs
- XPathNode.cs
- InputReportEventArgs.cs
- HttpApplicationFactory.cs
- XmlSchemaCompilationSettings.cs
- SqlDeflator.cs
- DecoratedNameAttribute.cs
- BufferModesCollection.cs
- EngineSiteSapi.cs
- ErrorFormatter.cs
- CellCreator.cs
- Pair.cs
- CodeThrowExceptionStatement.cs
- QilBinary.cs
- DataGridBoundColumn.cs
- IpcClientChannel.cs
- FontInfo.cs
- InternalBase.cs
- MachinePropertyVariants.cs
- BooleanConverter.cs
- SystemNetHelpers.cs
- TypeDependencyAttribute.cs
- GridViewCancelEditEventArgs.cs
- ServiceNameElement.cs
- KnownColorTable.cs
- OledbConnectionStringbuilder.cs
- EventWaitHandle.cs
- SocketException.cs
- Function.cs
- XmlCharacterData.cs
- KeyGestureConverter.cs
- CompilerGlobalScopeAttribute.cs
- CommandEventArgs.cs
- AccessText.cs
- DetailsViewUpdatedEventArgs.cs
- VectorCollectionValueSerializer.cs
- Ray3DHitTestResult.cs
- PropVariant.cs
- Exceptions.cs
- SiteMapSection.cs
- AssertUtility.cs
- FileDialogCustomPlace.cs
- TransformerInfo.cs
- SmtpReplyReader.cs
- HttpModuleCollection.cs
- CompoundFileDeflateTransform.cs
- SR.cs
- ReflectionServiceProvider.cs
- ZipIOZip64EndOfCentralDirectoryBlock.cs
- _TransmitFileOverlappedAsyncResult.cs
- JapaneseCalendar.cs
- Latin1Encoding.cs
- HtmlMeta.cs
- TextDecorationCollectionConverter.cs
- XmlStreamStore.cs
- WebServiceBindingAttribute.cs
- BitmapEffectInput.cs
- SoapIncludeAttribute.cs
- HttpCapabilitiesSectionHandler.cs
- Margins.cs
- ZipArchive.cs
- MenuStrip.cs
- StylusPointPropertyInfoDefaults.cs
- UncommonField.cs