Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / Services / Messaging / System / Messaging / Interop / Columns.cs / 1305376 / Columns.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Messaging.Interop { using System.Runtime.InteropServices; using System.Diagnostics; using System; using System.ComponentModel; using System.Globalization; //for CultureInfo using Microsoft.Win32; internal class Columns { private int maxCount; private MQCOLUMNSET columnSet = new MQCOLUMNSET(); public Columns(int maxCount) { this.maxCount = maxCount; this.columnSet.columnIdentifiers = Marshal.AllocHGlobal(maxCount * 4); this.columnSet.columnCount = 0; } public virtual void AddColumnId(int columnId) { lock(this) { if (this.columnSet.columnCount >= this.maxCount) throw new InvalidOperationException(Res.GetString(Res.TooManyColumns, this.maxCount.ToString(CultureInfo.CurrentCulture))); ++ this.columnSet.columnCount; this.columnSet.SetId(columnId, this.columnSet.columnCount - 1); } } public virtual MQCOLUMNSET GetColumnsRef() { return this.columnSet; } [StructLayout(LayoutKind.Sequential)] public class MQCOLUMNSET{ public int columnCount; [System.Diagnostics.CodeAnalysis.SuppressMessage("Microsoft.Reliability", "CA2006:UseSafeHandleToEncapsulateNativeResources")] public IntPtr columnIdentifiers; ~MQCOLUMNSET() { if (this.columnIdentifiers != (IntPtr)0) { Marshal.FreeHGlobal(this.columnIdentifiers); this.columnIdentifiers = (IntPtr)0; } } public virtual void SetId(int columnId, int index) { Marshal.WriteInt32((IntPtr)((long)this.columnIdentifiers + (index * 4)), columnId); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
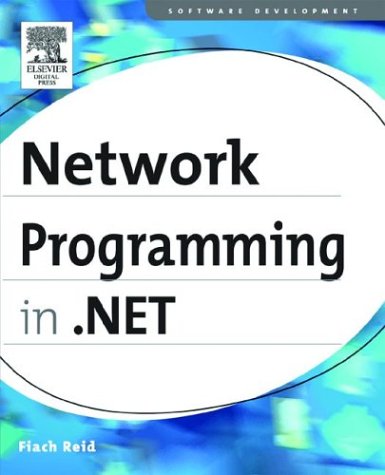
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- UnSafeCharBuffer.cs
- SystemDiagnosticsSection.cs
- ItemsControl.cs
- SuppressMessageAttribute.cs
- TiffBitmapEncoder.cs
- DaylightTime.cs
- GridItemPattern.cs
- Menu.cs
- XslAst.cs
- ManifestSignedXml.cs
- CodeArrayCreateExpression.cs
- ListView.cs
- SqlConnectionPoolGroupProviderInfo.cs
- GreenMethods.cs
- PasswordPropertyTextAttribute.cs
- FastEncoderWindow.cs
- TypedDatasetGenerator.cs
- LinqDataSourceStatusEventArgs.cs
- Thickness.cs
- XmlSerializerSection.cs
- Currency.cs
- TimersDescriptionAttribute.cs
- ManagedIStream.cs
- SapiAttributeParser.cs
- ContainerParagraph.cs
- ObjectAnimationUsingKeyFrames.cs
- MouseCaptureWithinProperty.cs
- FormViewInsertEventArgs.cs
- HtmlTable.cs
- RemoteArgument.cs
- FieldNameLookup.cs
- Compiler.cs
- TransactionState.cs
- NoClickablePointException.cs
- WebDescriptionAttribute.cs
- ListenerServiceInstallComponent.cs
- TemplatedMailWebEventProvider.cs
- OleDbFactory.cs
- DataGridViewRowPrePaintEventArgs.cs
- EventLogException.cs
- CorruptingExceptionCommon.cs
- TypeUsage.cs
- ViewStateChangedEventArgs.cs
- WebControl.cs
- BidPrivateBase.cs
- WinEventHandler.cs
- Assembly.cs
- XmlSchemaResource.cs
- ProxyWebPartManager.cs
- sqlser.cs
- TransformConverter.cs
- ResourceAssociationType.cs
- XmlQueryType.cs
- PerfService.cs
- DoubleIndependentAnimationStorage.cs
- AmbiguousMatchException.cs
- CopyOfAction.cs
- ReadOnlyHierarchicalDataSourceView.cs
- CryptoConfig.cs
- DataControlLinkButton.cs
- BitmapMetadataBlob.cs
- FillRuleValidation.cs
- XhtmlMobileTextWriter.cs
- XmlILModule.cs
- TextParentUndoUnit.cs
- SectionVisual.cs
- EntityDataSourceContainerNameItem.cs
- CodeDomComponentSerializationService.cs
- OdbcParameterCollection.cs
- GroupBoxAutomationPeer.cs
- MultiTrigger.cs
- FixedSOMImage.cs
- DataGridHeaderBorder.cs
- SoapFaultCodes.cs
- CheckBoxFlatAdapter.cs
- QuaternionRotation3D.cs
- ReflectPropertyDescriptor.cs
- StdRegProviderWrapper.cs
- DesignerActionListCollection.cs
- SoapHttpTransportImporter.cs
- BindingCompleteEventArgs.cs
- CultureTable.cs
- ObservableDictionary.cs
- ReaderWriterLockWrapper.cs
- DataRecordInternal.cs
- XmlArrayItemAttribute.cs
- HttpServerChannel.cs
- FixedSOMImage.cs
- ValuePatternIdentifiers.cs
- X509Certificate.cs
- Cursor.cs
- TableHeaderCell.cs
- DoubleLinkList.cs
- DataGridCaption.cs
- ByteKeyFrameCollection.cs
- XamlReader.cs
- HostingEnvironment.cs
- ListParaClient.cs
- RemotingClientProxy.cs
- FrameAutomationPeer.cs