Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / CheckBoxRenderer.cs / 1305376 / CheckBoxRenderer.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- namespace System.Windows.Forms { using System; using System.Drawing; using System.Diagnostics.CodeAnalysis; using System.Windows.Forms.Internal; using System.Windows.Forms.VisualStyles; using Microsoft.Win32; ////// /// public sealed class CheckBoxRenderer { //Make this per-thread, so that different threads can safely use these methods. [ThreadStatic] private static VisualStyleRenderer visualStyleRenderer = null; private static readonly VisualStyleElement CheckBoxElement = VisualStyleElement.Button.CheckBox.UncheckedNormal; private static bool renderMatchingApplicationState = true; //cannot instantiate private CheckBoxRenderer() { } ////// This is a rendering class for the CheckBox control. It works downlevel too (obviously /// without visual styles applied.) /// ////// /// public static bool RenderMatchingApplicationState { get { return renderMatchingApplicationState; } set { renderMatchingApplicationState = value; } } private static bool RenderWithVisualStyles { get { return (!renderMatchingApplicationState || Application.RenderWithVisualStyles); } } ////// If this property is true, then the renderer will use the setting from Application.RenderWithVisualStyles to /// determine how to render. /// If this property is false, the renderer will always render with visualstyles. /// ////// /// public static bool IsBackgroundPartiallyTransparent(CheckBoxState state) { if (RenderWithVisualStyles) { InitializeRenderer((int)state); return visualStyleRenderer.IsBackgroundPartiallyTransparent(); } else { return false; //for downlevel, this is false } } ////// Returns true if the background corresponding to the given state is partially transparent, else false. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static void DrawParentBackground(Graphics g, Rectangle bounds, Control childControl) { if (RenderWithVisualStyles) { InitializeRenderer(0); visualStyleRenderer.DrawParentBackground(g, bounds, childControl); } } ////// This is just a convenience wrapper for VisualStyleRenderer.DrawThemeParentBackground. For downlevel, /// this isn't required and does nothing. /// ////// /// public static void DrawCheckBox(Graphics g, Point glyphLocation, CheckBoxState state) { Rectangle glyphBounds = new Rectangle(glyphLocation, GetGlyphSize(g, state)); if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, glyphBounds); } else { if (IsMixed(state)) { ControlPaint.DrawMixedCheckBox(g, glyphBounds, ConvertToButtonState(state)); } else { ControlPaint.DrawCheckBox(g, glyphBounds, ConvertToButtonState(state)); } } } ////// Renders a CheckBox control. /// ////// /// public static void DrawCheckBox(Graphics g, Point glyphLocation, Rectangle textBounds, string checkBoxText, Font font, bool focused, CheckBoxState state) { DrawCheckBox(g, glyphLocation, textBounds, checkBoxText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, focused, state); } ////// Renders a CheckBox control. /// ////// /// public static void DrawCheckBox(Graphics g, Point glyphLocation, Rectangle textBounds, string checkBoxText, Font font, TextFormatFlags flags, bool focused, CheckBoxState state) { Rectangle glyphBounds = new Rectangle(glyphLocation, GetGlyphSize(g, state)); Color textColor; if (RenderWithVisualStyles) { InitializeRenderer((int)state); visualStyleRenderer.DrawBackground(g, glyphBounds); textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); } else { if (IsMixed(state)) { ControlPaint.DrawMixedCheckBox(g, glyphBounds, ConvertToButtonState(state)); } else { ControlPaint.DrawCheckBox(g, glyphBounds, ConvertToButtonState(state)); } textColor = SystemColors.ControlText; } TextRenderer.DrawText(g, checkBoxText, font, textBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, textBounds); } } ////// Renders a CheckBox control. /// ////// /// public static void DrawCheckBox(Graphics g, Point glyphLocation, Rectangle textBounds, string checkBoxText, Font font, Image image, Rectangle imageBounds, bool focused, CheckBoxState state) { DrawCheckBox(g, glyphLocation, textBounds, checkBoxText, font, TextFormatFlags.HorizontalCenter | TextFormatFlags.VerticalCenter | TextFormatFlags.SingleLine, image, imageBounds, focused, state); } ////// Renders a CheckBox control. /// ////// /// public static void DrawCheckBox(Graphics g, Point glyphLocation, Rectangle textBounds, string checkBoxText, Font font, TextFormatFlags flags, Image image, Rectangle imageBounds, bool focused, CheckBoxState state) { Rectangle glyphBounds = new Rectangle(glyphLocation, GetGlyphSize(g, state)); Color textColor; if (RenderWithVisualStyles) { InitializeRenderer((int)state); //Keep this drawing order! It matches default drawing order. visualStyleRenderer.DrawImage(g, imageBounds, image); visualStyleRenderer.DrawBackground(g, glyphBounds); textColor = visualStyleRenderer.GetColor(ColorProperty.TextColor); } else { g.DrawImage(image, imageBounds); if (IsMixed(state)) { ControlPaint.DrawMixedCheckBox(g, glyphBounds, ConvertToButtonState(state)); } else { ControlPaint.DrawCheckBox(g, glyphBounds, ConvertToButtonState(state)); } textColor = SystemColors.ControlText; } TextRenderer.DrawText(g, checkBoxText, font, textBounds, textColor, flags); if (focused) { ControlPaint.DrawFocusRectangle(g, textBounds); } } ////// Renders a CheckBox control. /// ////// /// [ SuppressMessage("Microsoft.Design", "CA1011:ConsiderPassingBaseTypesAsParameters") // Using Graphics instead of IDeviceContext intentionally ] public static Size GetGlyphSize(Graphics g, CheckBoxState state) { if (RenderWithVisualStyles) { InitializeRenderer((int)state); return visualStyleRenderer.GetPartSize(g, ThemeSizeType.Draw); } return new Size(13, 13); } internal static ButtonState ConvertToButtonState(CheckBoxState state) { switch (state) { case CheckBoxState.CheckedNormal: case CheckBoxState.CheckedHot: return ButtonState.Checked; case CheckBoxState.CheckedPressed: return (ButtonState.Checked | ButtonState.Pushed); case CheckBoxState.CheckedDisabled: return (ButtonState.Checked | ButtonState.Inactive); case CheckBoxState.UncheckedPressed: return ButtonState.Pushed; case CheckBoxState.UncheckedDisabled: return ButtonState.Inactive; //Downlevel mixed drawing works only if ButtonState.Checked is set case CheckBoxState.MixedNormal: case CheckBoxState.MixedHot: return ButtonState.Checked; case CheckBoxState.MixedPressed: return (ButtonState.Checked | ButtonState.Pushed); case CheckBoxState.MixedDisabled: return (ButtonState.Checked | ButtonState.Inactive); default: return ButtonState.Normal; } } internal static CheckBoxState ConvertFromButtonState(ButtonState state, bool isMixed, bool isHot) { if (isMixed) { if ((state & ButtonState.Pushed) == ButtonState.Pushed) { return CheckBoxState.MixedPressed; } else if ((state & ButtonState.Inactive) == ButtonState.Inactive) { return CheckBoxState.MixedDisabled; } else if (isHot) { return CheckBoxState.MixedHot; } return CheckBoxState.MixedNormal; } else if ((state & ButtonState.Checked) == ButtonState.Checked) { if ((state & ButtonState.Pushed) == ButtonState.Pushed) { return CheckBoxState.CheckedPressed; } else if ((state & ButtonState.Inactive) == ButtonState.Inactive) { return CheckBoxState.CheckedDisabled; } else if (isHot) { return CheckBoxState.CheckedHot; } return CheckBoxState.CheckedNormal; } else { //unchecked if ((state & ButtonState.Pushed) == ButtonState.Pushed) { return CheckBoxState.UncheckedPressed; } else if ((state & ButtonState.Inactive) == ButtonState.Inactive) { return CheckBoxState.UncheckedDisabled; } else if (isHot) { return CheckBoxState.UncheckedHot; } return CheckBoxState.UncheckedNormal; } } private static bool IsMixed(CheckBoxState state) { switch (state) { case CheckBoxState.MixedNormal: case CheckBoxState.MixedHot: case CheckBoxState.MixedPressed: case CheckBoxState.MixedDisabled: return true; default: return false; } } private static void InitializeRenderer(int state) { if (visualStyleRenderer == null) { visualStyleRenderer = new VisualStyleRenderer(CheckBoxElement.ClassName, CheckBoxElement.Part, state); } else { visualStyleRenderer.SetParameters(CheckBoxElement.ClassName, CheckBoxElement.Part, state); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007./// Returns the size of the CheckBox glyph. /// ///
Link Menu
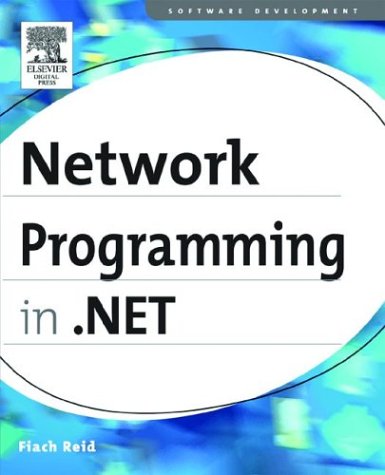
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- MarkerProperties.cs
- TextTreeTextNode.cs
- DeploymentSectionCache.cs
- BitSet.cs
- CompositeActivityTypeDescriptorProvider.cs
- UserControl.cs
- XmlComment.cs
- DataStreamFromComStream.cs
- DesignerCategoryAttribute.cs
- DefaultObjectMappingItemCollection.cs
- ToolStripGrip.cs
- DocumentViewerBaseAutomationPeer.cs
- XmlDocumentFragment.cs
- DeploymentExceptionMapper.cs
- DataRecordObjectView.cs
- InvalidateEvent.cs
- FunctionNode.cs
- SQLGuidStorage.cs
- _BasicClient.cs
- Mutex.cs
- NetCodeGroup.cs
- sqlcontext.cs
- CacheMode.cs
- ProfileManager.cs
- PrivilegedConfigurationManager.cs
- Config.cs
- Activity.cs
- NetCodeGroup.cs
- BaseParser.cs
- XmlSchemaGroup.cs
- InstanceKeyCompleteException.cs
- AlphaSortedEnumConverter.cs
- WebServiceEnumData.cs
- SynchronizedInputPattern.cs
- AutoFocusStyle.xaml.cs
- ServicesUtilities.cs
- Deserializer.cs
- DBParameter.cs
- GetPageCompletedEventArgs.cs
- ToolStripManager.cs
- OracleParameterBinding.cs
- MarkupExtensionReturnTypeAttribute.cs
- MobileControlPersister.cs
- EntityDescriptor.cs
- BindValidator.cs
- Environment.cs
- QueryContinueDragEvent.cs
- LambdaCompiler.Binary.cs
- PrintDialog.cs
- GlyphsSerializer.cs
- NativeMethods.cs
- FlatButtonAppearance.cs
- DynamicDocumentPaginator.cs
- DataGridViewComboBoxCell.cs
- CorrelationInitializer.cs
- XmlSchemaSimpleTypeList.cs
- XslVisitor.cs
- WindowPattern.cs
- DefaultMemberAttribute.cs
- AutomationPeer.cs
- _StreamFramer.cs
- NameValuePermission.cs
- SafeNativeMethods.cs
- SecurityKeyUsage.cs
- versioninfo.cs
- QueryStoreStatusRequest.cs
- DataSourceProvider.cs
- TreeNodeCollection.cs
- PermissionRequestEvidence.cs
- HtmlControl.cs
- SocketPermission.cs
- SyndicationFeedFormatter.cs
- ColumnClickEvent.cs
- BorderSidesEditor.cs
- PropertyChangeTracker.cs
- Duration.cs
- RequestCachingSection.cs
- DocumentEventArgs.cs
- EdmType.cs
- NativeMethods.cs
- cookiecollection.cs
- SmuggledIUnknown.cs
- COM2ComponentEditor.cs
- ToolStripDropDownMenu.cs
- httpstaticobjectscollection.cs
- SqlDataSourceFilteringEventArgs.cs
- MarkupWriter.cs
- SchemaMapping.cs
- BooleanAnimationBase.cs
- ContextBase.cs
- ViewLoader.cs
- QueryContinueDragEventArgs.cs
- DesignBindingConverter.cs
- JsonXmlDataContract.cs
- JournalEntry.cs
- EndGetFileNameFromUserRequest.cs
- CaretElement.cs
- AddInToken.cs
- OdbcEnvironment.cs
- CollectionDataContractAttribute.cs