Code:
/ 4.0 / 4.0 / untmp / DEVDIV_TFS / Dev10 / Releases / RTMRel / ndp / fx / src / WinForms / Managed / System / WinForms / ListViewInsertionMark.cs / 1305376 / ListViewInsertionMark.cs
//------------------------------------------------------------------------------ //// Copyright (c) Microsoft Corporation. All rights reserved. // //----------------------------------------------------------------------------- using System.Drawing; using System.Runtime.InteropServices; using System.Diagnostics; namespace System.Windows.Forms { ////// /// public sealed class ListViewInsertionMark { private ListView listView; private int index = 0; private Color color = Color.Empty; private bool appearsAfterItem = false; internal ListViewInsertionMark(ListView listView) { this.listView = listView; } ////// Encapsulates insertion-mark information /// ////// /// Specifies whether the insertion mark appears /// after the item - otherwise it appears /// before the item (the default). /// /// public bool AppearsAfterItem { get { return appearsAfterItem; } set { if (appearsAfterItem != value) { appearsAfterItem = value; if (listView.IsHandleCreated) { UpdateListView(); } } } } ////// /// Returns bounds of the insertion-mark. /// /// public Rectangle Bounds { get { NativeMethods.RECT rect = new NativeMethods.RECT(); listView.SendMessage(NativeMethods.LVM_GETINSERTMARKRECT, 0, ref rect); return Rectangle.FromLTRB(rect.left, rect.top, rect.right, rect.bottom); } } ////// /// The color of the insertion-mark. /// /// public Color Color { get { if (color.IsEmpty) { color = SafeNativeMethods.ColorFromCOLORREF((int)listView.SendMessage(NativeMethods.LVM_GETINSERTMARKCOLOR, 0, 0)); } return color; } set { if (color != value) { color = value; if (listView.IsHandleCreated) { listView.SendMessage(NativeMethods.LVM_SETINSERTMARKCOLOR, 0, SafeNativeMethods.ColorToCOLORREF(color)); } } } } ////// /// Item next to which the insertion-mark appears. /// /// public int Index { get { return index; } set { if (index != value) { index = value; if (listView.IsHandleCreated) { UpdateListView(); } } } } ////// /// Performs a hit-test at the specified insertion point /// and returns the closest item. /// /// public int NearestIndex(Point pt) { NativeMethods.POINT point = new NativeMethods.POINT(); point.x = pt.X; point.y = pt.Y; NativeMethods.LVINSERTMARK lvInsertMark = new NativeMethods.LVINSERTMARK(); UnsafeNativeMethods.SendMessage(new HandleRef(listView, listView.Handle), NativeMethods.LVM_INSERTMARKHITTEST, point, lvInsertMark); return lvInsertMark.iItem; } internal void UpdateListView() { Debug.Assert(listView.IsHandleCreated, "ApplySavedState Precondition: List-view handle must be created"); NativeMethods.LVINSERTMARK lvInsertMark = new NativeMethods.LVINSERTMARK(); lvInsertMark.dwFlags = appearsAfterItem ? NativeMethods.LVIM_AFTER : 0; lvInsertMark.iItem = index; UnsafeNativeMethods.SendMessage(new HandleRef(listView, listView.Handle), NativeMethods.LVM_SETINSERTMARK, 0, lvInsertMark); if (!color.IsEmpty) { listView.SendMessage(NativeMethods.LVM_SETINSERTMARKCOLOR, 0, SafeNativeMethods.ColorToCOLORREF(color)); } } } } // File provided for Reference Use Only by Microsoft Corporation (c) 2007.
Link Menu
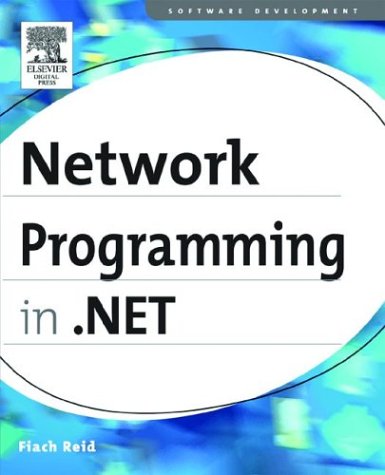
This book is available now!
Buy at Amazon US or
Buy at Amazon UK
- TextContainer.cs
- OutputCacheProviderCollection.cs
- TreeViewTemplateSelector.cs
- KeyValuePair.cs
- IMembershipProvider.cs
- Decoder.cs
- IdentitySection.cs
- TypefaceMap.cs
- ProcessHostConfigUtils.cs
- BrowserDefinitionCollection.cs
- SqlUtils.cs
- TableCell.cs
- Pool.cs
- ConfigurationConverterBase.cs
- ProxyGenerationError.cs
- RandomNumberGenerator.cs
- QueryContinueDragEventArgs.cs
- InternalTypeHelper.cs
- RequiredFieldValidator.cs
- WindowsRichEditRange.cs
- AppDomainUnloadedException.cs
- FlowLayoutPanel.cs
- ResourceDictionaryCollection.cs
- EnumMemberAttribute.cs
- PictureBox.cs
- VirtualDirectoryMappingCollection.cs
- Container.cs
- VirtualDirectoryMappingCollection.cs
- HttpClientCredentialType.cs
- ImmutableClientRuntime.cs
- ContentPlaceHolderDesigner.cs
- Rfc2898DeriveBytes.cs
- MemberDomainMap.cs
- SystemIPAddressInformation.cs
- StringReader.cs
- GZipStream.cs
- Binding.cs
- WebHeaderCollection.cs
- Pointer.cs
- HostedController.cs
- SponsorHelper.cs
- SafeRightsManagementQueryHandle.cs
- PhysicalAddress.cs
- DefaultAsyncDataDispatcher.cs
- AssociatedControlConverter.cs
- SectionRecord.cs
- SchemaImporter.cs
- WasAdminWrapper.cs
- AttributeQuery.cs
- SingleAnimationUsingKeyFrames.cs
- FontInfo.cs
- EdmItemCollection.cs
- DesignerPerfEventProvider.cs
- OleDbDataAdapter.cs
- TableItemStyle.cs
- MultilineStringConverter.cs
- ListViewSortEventArgs.cs
- ButtonFieldBase.cs
- GenericUriParser.cs
- ValueCollectionParameterReader.cs
- StatusBarItem.cs
- Vector3DCollection.cs
- BuildDependencySet.cs
- SqlDataSourceSelectingEventArgs.cs
- SignatureConfirmations.cs
- Solver.cs
- DataServiceEntityAttribute.cs
- DataSourceXmlClassAttribute.cs
- UnsafeNativeMethods.cs
- WebProxyScriptElement.cs
- WebPartHelpVerb.cs
- BamlRecordWriter.cs
- QilChoice.cs
- Cloud.cs
- GuidTagList.cs
- PointAnimationUsingPath.cs
- DynamicResourceExtension.cs
- ReachDocumentReferenceCollectionSerializer.cs
- EntryWrittenEventArgs.cs
- PlacementWorkspace.cs
- NaturalLanguageHyphenator.cs
- ByteKeyFrameCollection.cs
- ListControlBuilder.cs
- BamlRecordReader.cs
- Stacktrace.cs
- DynamicEntity.cs
- ToolStripStatusLabel.cs
- ApplicationInterop.cs
- Variable.cs
- WebPartDisplayMode.cs
- XmlLanguageConverter.cs
- IssuedTokenParametersElement.cs
- AssemblyHash.cs
- TableItemPatternIdentifiers.cs
- MsmqQueue.cs
- BuildResultCache.cs
- StackSpiller.Generated.cs
- BehaviorEditorPart.cs
- DetailsViewPageEventArgs.cs
- DynamicRendererThreadManager.cs